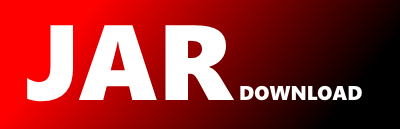
org.jnario.impl.JnarioFactoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/*******************************************************************************
* Copyright (c) 2012 BMW Car IT and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.jnario.impl;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.impl.EFactoryImpl;
import org.eclipse.emf.ecore.plugin.EcorePlugin;
import org.jnario.*;
import org.jnario.Assertion;
import org.jnario.ExampleColumn;
import org.jnario.ExampleRow;
import org.jnario.ExampleTable;
import org.jnario.JnarioFactory;
import org.jnario.JnarioPackage;
import org.jnario.MockLiteral;
import org.jnario.Should;
import org.jnario.ShouldThrow;
/**
*
* An implementation of the model Factory.
*
* @generated
*/
public class JnarioFactoryImpl extends EFactoryImpl implements JnarioFactory {
/**
* Creates the default factory implementation.
*
*
* @generated
*/
public static JnarioFactory init() {
try {
JnarioFactory theJnarioFactory = (JnarioFactory)EPackage.Registry.INSTANCE.getEFactory(JnarioPackage.eNS_URI);
if (theJnarioFactory != null) {
return theJnarioFactory;
}
}
catch (Exception exception) {
EcorePlugin.INSTANCE.log(exception);
}
return new JnarioFactoryImpl();
}
/**
* Creates an instance of the factory.
*
*
* @generated
*/
public JnarioFactoryImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
public EObject create(EClass eClass) {
switch (eClass.getClassifierID()) {
case JnarioPackage.EXAMPLE_TABLE: return createExampleTable();
case JnarioPackage.EXAMPLE_ROW: return createExampleRow();
case JnarioPackage.ASSERTION: return createAssertion();
case JnarioPackage.EXAMPLE_COLUMN: return createExampleColumn();
case JnarioPackage.SHOULD: return createShould();
case JnarioPackage.SHOULD_THROW: return createShouldThrow();
case JnarioPackage.SPECIFICATION: return createSpecification();
case JnarioPackage.MOCK_LITERAL: return createMockLiteral();
case JnarioPackage.EXAMPLE_CELL: return createExampleCell();
default:
throw new IllegalArgumentException("The class '" + eClass.getName() + "' is not a valid classifier");
}
}
/**
*
*
* @generated
*/
public ExampleTable createExampleTable() {
ExampleTableImpl exampleTable = new ExampleTableImpl();
return exampleTable;
}
/**
*
*
* @generated
*/
public ExampleRow createExampleRow() {
ExampleRowImpl exampleRow = new ExampleRowImpl();
return exampleRow;
}
/**
*
*
* @generated
*/
public Assertion createAssertion() {
AssertionImpl assertion = new AssertionImpl();
return assertion;
}
/**
*
*
* @generated
*/
public ExampleColumn createExampleColumn() {
ExampleColumnImpl exampleColumn = new ExampleColumnImpl();
return exampleColumn;
}
/**
*
*
* @generated
*/
public Should createShould() {
ShouldImpl should = new ShouldImpl();
return should;
}
/**
*
*
* @generated
*/
public ShouldThrow createShouldThrow() {
ShouldThrowImpl shouldThrow = new ShouldThrowImpl();
return shouldThrow;
}
/**
*
*
* @generated
*/
public Specification createSpecification() {
SpecificationImpl specification = new SpecificationImpl();
return specification;
}
/**
*
*
* @generated
*/
public MockLiteral createMockLiteral() {
MockLiteralImpl mockLiteral = new MockLiteralImpl();
return mockLiteral;
}
/**
*
*
* @generated
*/
public ExampleCell createExampleCell() {
ExampleCellImpl exampleCell = new ExampleCellImpl();
return exampleCell;
}
/**
*
*
* @generated
*/
public JnarioPackage getJnarioPackage() {
return (JnarioPackage)getEPackage();
}
/**
*
*
* @deprecated
* @generated
*/
@Deprecated
public static JnarioPackage getPackage() {
return JnarioPackage.eINSTANCE;
}
} //JnarioFactoryImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy