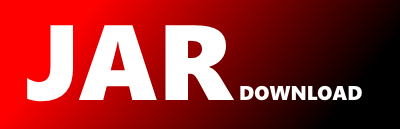
org.jnario.impl.JnarioPackageImpl Maven / Gradle / Ivy
Show all versions of org.jnario.standalone Show documentation
/*******************************************************************************
* Copyright (c) 2012 BMW Car IT and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.jnario.impl;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
import org.eclipse.emf.ecore.impl.EPackageImpl;
import org.eclipse.xtend.core.xtend.XtendPackage;
import org.eclipse.xtext.common.types.TypesPackage;
import org.eclipse.xtext.xbase.XbasePackage;
import org.eclipse.xtext.xtype.XtypePackage;
import org.jnario.Assertion;
import org.jnario.ExampleCell;
import org.jnario.ExampleColumn;
import org.jnario.ExampleRow;
import org.jnario.ExampleTable;
import org.jnario.Executable;
import org.jnario.JnarioFactory;
import org.jnario.JnarioPackage;
import org.jnario.MockLiteral;
import org.jnario.Should;
import org.jnario.ShouldThrow;
import org.jnario.Specification;
/**
*
* An implementation of the model Package.
*
* @generated
*/
public class JnarioPackageImpl extends EPackageImpl implements JnarioPackage {
/**
*
*
* @generated
*/
private EClass exampleTableEClass = null;
/**
*
*
* @generated
*/
private EClass exampleRowEClass = null;
/**
*
*
* @generated
*/
private EClass assertionEClass = null;
/**
*
*
* @generated
*/
private EClass exampleColumnEClass = null;
/**
*
*
* @generated
*/
private EClass shouldEClass = null;
/**
*
*
* @generated
*/
private EClass shouldThrowEClass = null;
/**
*
*
* @generated
*/
private EClass specificationEClass = null;
/**
*
*
* @generated
*/
private EClass executableEClass = null;
/**
*
*
* @generated
*/
private EClass mockLiteralEClass = null;
/**
*
*
* @generated
*/
private EClass exampleCellEClass = null;
/**
* Creates an instance of the model Package, registered with
* {@link org.eclipse.emf.ecore.EPackage.Registry EPackage.Registry} by the package
* package URI value.
* Note: the correct way to create the package is via the static
* factory method {@link #init init()}, which also performs
* initialization of the package, or returns the registered package,
* if one already exists.
*
*
* @see org.eclipse.emf.ecore.EPackage.Registry
* @see org.jnario.JnarioPackage#eNS_URI
* @see #init()
* @generated
*/
private JnarioPackageImpl() {
super(eNS_URI, JnarioFactory.eINSTANCE);
}
/**
*
*
* @generated
*/
private static boolean isInited = false;
/**
* Creates, registers, and initializes the Package for this model, and for any others upon which it depends.
*
*
This method is used to initialize {@link JnarioPackage#eINSTANCE} when that field is accessed.
* Clients should not invoke it directly. Instead, they should simply access that field to obtain the package.
*
*
* @see #eNS_URI
* @see #createPackageContents()
* @see #initializePackageContents()
* @generated
*/
public static JnarioPackage init() {
if (isInited) return (JnarioPackage)EPackage.Registry.INSTANCE.getEPackage(JnarioPackage.eNS_URI);
// Obtain or create and register package
JnarioPackageImpl theJnarioPackage = (JnarioPackageImpl)(EPackage.Registry.INSTANCE.get(eNS_URI) instanceof JnarioPackageImpl ? EPackage.Registry.INSTANCE.get(eNS_URI) : new JnarioPackageImpl());
isInited = true;
// Initialize simple dependencies
XtendPackage.eINSTANCE.eClass();
XtypePackage.eINSTANCE.eClass();
// Create package meta-data objects
theJnarioPackage.createPackageContents();
// Initialize created meta-data
theJnarioPackage.initializePackageContents();
// Mark meta-data to indicate it can't be changed
theJnarioPackage.freeze();
// Update the registry and return the package
EPackage.Registry.INSTANCE.put(JnarioPackage.eNS_URI, theJnarioPackage);
return theJnarioPackage;
}
/**
*
*
* @generated
*/
public EClass getExampleTable() {
return exampleTableEClass;
}
/**
*
*
* @generated
*/
public EAttribute getExampleTable_Name() {
return (EAttribute)exampleTableEClass.getEStructuralFeatures().get(0);
}
/**
*
*
* @generated
*/
public EReference getExampleTable_Rows() {
return (EReference)exampleTableEClass.getEStructuralFeatures().get(1);
}
/**
*
*
* @generated
*/
public EReference getExampleTable_Columns() {
return (EReference)exampleTableEClass.getEStructuralFeatures().get(2);
}
/**
*
*
* @generated
*/
public EClass getExampleRow() {
return exampleRowEClass;
}
/**
*
*
* @generated
*/
public EReference getExampleRow_Cells() {
return (EReference)exampleRowEClass.getEStructuralFeatures().get(0);
}
/**
*
*
* @generated
*/
public EReference getExampleRow_Table() {
return (EReference)exampleRowEClass.getEStructuralFeatures().get(1);
}
/**
*
*
* @generated
*/
public EClass getAssertion() {
return assertionEClass;
}
/**
*
*
* @generated
*/
public EReference getAssertion_Expression() {
return (EReference)assertionEClass.getEStructuralFeatures().get(0);
}
/**
*
*
* @generated
*/
public EClass getExampleColumn() {
return exampleColumnEClass;
}
/**
*
*
* @generated
*/
public EReference getExampleColumn_Table() {
return (EReference)exampleColumnEClass.getEStructuralFeatures().get(0);
}
/**
*
*
* @generated
*/
public EReference getExampleColumn_Cells() {
return (EReference)exampleColumnEClass.getEStructuralFeatures().get(1);
}
/**
*
*
* @generated
*/
public EAttribute getExampleColumn_Name() {
return (EAttribute)exampleColumnEClass.getEStructuralFeatures().get(2);
}
/**
*
*
* @generated
*/
public EReference getExampleColumn_Type() {
return (EReference)exampleColumnEClass.getEStructuralFeatures().get(3);
}
/**
*
*
* @generated
*/
public EClass getShould() {
return shouldEClass;
}
/**
*
*
* @generated
*/
public EAttribute getShould_Not() {
return (EAttribute)shouldEClass.getEStructuralFeatures().get(0);
}
/**
*
*
* @generated
*/
public EClass getShouldThrow() {
return shouldThrowEClass;
}
/**
*
*
* @generated
*/
public EReference getShouldThrow_Type() {
return (EReference)shouldThrowEClass.getEStructuralFeatures().get(0);
}
/**
*
*
* @generated
*/
public EReference getShouldThrow_Expression() {
return (EReference)shouldThrowEClass.getEStructuralFeatures().get(1);
}
/**
*
*
* @generated
*/
public EClass getSpecification() {
return specificationEClass;
}
/**
*
*
* @generated
*/
public EClass getExecutable() {
return executableEClass;
}
/**
*
*
* @generated
*/
public EClass getMockLiteral() {
return mockLiteralEClass;
}
/**
*
*
* @generated
*/
public EClass getExampleCell() {
return exampleCellEClass;
}
/**
*
*
* @generated
*/
public JnarioFactory getJnarioFactory() {
return (JnarioFactory)getEFactoryInstance();
}
/**
*
*
* @generated
*/
private boolean isCreated = false;
/**
* Creates the meta-model objects for the package. This method is
* guarded to have no affect on any invocation but its first.
*
*
* @generated
*/
public void createPackageContents() {
if (isCreated) return;
isCreated = true;
// Create classes and their features
exampleTableEClass = createEClass(EXAMPLE_TABLE);
createEAttribute(exampleTableEClass, EXAMPLE_TABLE__NAME);
createEReference(exampleTableEClass, EXAMPLE_TABLE__ROWS);
createEReference(exampleTableEClass, EXAMPLE_TABLE__COLUMNS);
exampleRowEClass = createEClass(EXAMPLE_ROW);
createEReference(exampleRowEClass, EXAMPLE_ROW__CELLS);
createEReference(exampleRowEClass, EXAMPLE_ROW__TABLE);
assertionEClass = createEClass(ASSERTION);
createEReference(assertionEClass, ASSERTION__EXPRESSION);
exampleColumnEClass = createEClass(EXAMPLE_COLUMN);
createEReference(exampleColumnEClass, EXAMPLE_COLUMN__TABLE);
createEReference(exampleColumnEClass, EXAMPLE_COLUMN__CELLS);
createEAttribute(exampleColumnEClass, EXAMPLE_COLUMN__NAME);
createEReference(exampleColumnEClass, EXAMPLE_COLUMN__TYPE);
shouldEClass = createEClass(SHOULD);
createEAttribute(shouldEClass, SHOULD__NOT);
shouldThrowEClass = createEClass(SHOULD_THROW);
createEReference(shouldThrowEClass, SHOULD_THROW__TYPE);
createEReference(shouldThrowEClass, SHOULD_THROW__EXPRESSION);
specificationEClass = createEClass(SPECIFICATION);
executableEClass = createEClass(EXECUTABLE);
mockLiteralEClass = createEClass(MOCK_LITERAL);
exampleCellEClass = createEClass(EXAMPLE_CELL);
}
/**
*
*
* @generated
*/
private boolean isInitialized = false;
/**
* Complete the initialization of the package and its meta-model. This
* method is guarded to have no affect on any invocation but its first.
*
*
* @generated
*/
public void initializePackageContents() {
if (isInitialized) return;
isInitialized = true;
// Initialize package
setName(eNAME);
setNsPrefix(eNS_PREFIX);
setNsURI(eNS_URI);
// Obtain other dependent packages
XtendPackage theXtendPackage = (XtendPackage)EPackage.Registry.INSTANCE.getEPackage(XtendPackage.eNS_URI);
XbasePackage theXbasePackage = (XbasePackage)EPackage.Registry.INSTANCE.getEPackage(XbasePackage.eNS_URI);
TypesPackage theTypesPackage = (TypesPackage)EPackage.Registry.INSTANCE.getEPackage(TypesPackage.eNS_URI);
// Create type parameters
// Set bounds for type parameters
// Add supertypes to classes
exampleTableEClass.getESuperTypes().add(theXtendPackage.getXtendMember());
exampleTableEClass.getESuperTypes().add(theXbasePackage.getXExpression());
assertionEClass.getESuperTypes().add(theXbasePackage.getXExpression());
shouldEClass.getESuperTypes().add(theXbasePackage.getXBinaryOperation());
shouldThrowEClass.getESuperTypes().add(theXbasePackage.getXExpression());
specificationEClass.getESuperTypes().add(theXtendPackage.getXtendClass());
specificationEClass.getESuperTypes().add(this.getExecutable());
mockLiteralEClass.getESuperTypes().add(theXbasePackage.getXTypeLiteral());
exampleCellEClass.getESuperTypes().add(theXtendPackage.getXtendFunction());
// Initialize classes and features; add operations and parameters
initEClass(exampleTableEClass, ExampleTable.class, "ExampleTable", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEAttribute(getExampleTable_Name(), ecorePackage.getEString(), "name", null, 0, 1, ExampleTable.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, !IS_UNSETTABLE, !IS_ID, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEReference(getExampleTable_Rows(), this.getExampleRow(), this.getExampleRow_Table(), "rows", null, 0, -1, ExampleTable.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEReference(getExampleTable_Columns(), this.getExampleColumn(), this.getExampleColumn_Table(), "columns", null, 0, -1, ExampleTable.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
addEOperation(exampleTableEClass, ecorePackage.getEBoolean(), "isValid", 0, 1, IS_UNIQUE, IS_ORDERED);
initEClass(exampleRowEClass, ExampleRow.class, "ExampleRow", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEReference(getExampleRow_Cells(), this.getExampleCell(), null, "cells", null, 0, -1, ExampleRow.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEReference(getExampleRow_Table(), this.getExampleTable(), this.getExampleTable_Rows(), "table", null, 0, 1, ExampleRow.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, !IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEClass(assertionEClass, Assertion.class, "Assertion", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEReference(getAssertion_Expression(), theXbasePackage.getXExpression(), null, "expression", null, 0, 1, Assertion.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEClass(exampleColumnEClass, ExampleColumn.class, "ExampleColumn", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEReference(getExampleColumn_Table(), this.getExampleTable(), this.getExampleTable_Columns(), "table", null, 0, 1, ExampleColumn.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, !IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEReference(getExampleColumn_Cells(), this.getExampleCell(), null, "cells", null, 0, -1, ExampleColumn.class, IS_TRANSIENT, IS_VOLATILE, IS_CHANGEABLE, !IS_COMPOSITE, IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, IS_DERIVED, IS_ORDERED);
initEAttribute(getExampleColumn_Name(), ecorePackage.getEString(), "name", null, 0, 1, ExampleColumn.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, !IS_UNSETTABLE, !IS_ID, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEReference(getExampleColumn_Type(), theTypesPackage.getJvmTypeReference(), null, "type", null, 0, 1, ExampleColumn.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEClass(shouldEClass, Should.class, "Should", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEAttribute(getShould_Not(), ecorePackage.getEBoolean(), "not", null, 0, 1, Should.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, !IS_UNSETTABLE, !IS_ID, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEClass(shouldThrowEClass, ShouldThrow.class, "ShouldThrow", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEReference(getShouldThrow_Type(), theTypesPackage.getJvmTypeReference(), null, "type", null, 1, 1, ShouldThrow.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEReference(getShouldThrow_Expression(), theXbasePackage.getXExpression(), null, "expression", null, 1, 1, ShouldThrow.class, !IS_TRANSIENT, !IS_VOLATILE, IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
initEClass(specificationEClass, Specification.class, "Specification", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEClass(executableEClass, Executable.class, "Executable", IS_ABSTRACT, IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
addEOperation(executableEClass, ecorePackage.getEBoolean(), "isPending", 0, 1, IS_UNIQUE, IS_ORDERED);
initEClass(mockLiteralEClass, MockLiteral.class, "MockLiteral", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
initEClass(exampleCellEClass, ExampleCell.class, "ExampleCell", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
// Create resource
createResource(eNS_URI);
}
} //JnarioPackageImpl