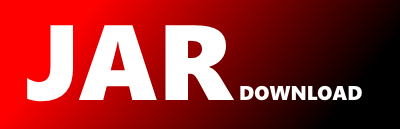
org.jnario.impl.ShouldThrowImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/**
*
*
*
* $Id$
*/
package org.jnario.impl;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.xtext.common.types.JvmTypeReference;
import org.eclipse.xtext.xbase.XExpression;
import org.eclipse.xtext.xbase.impl.XExpressionImpl;
import org.jnario.JnarioPackage;
import org.jnario.ShouldThrow;
/**
*
* An implementation of the model object 'Should Throw'.
*
*
* The following features are implemented:
*
* - {@link org.jnario.impl.ShouldThrowImpl#getType Type}
* - {@link org.jnario.impl.ShouldThrowImpl#getExpression Expression}
*
*
*
* @generated
*/
public class ShouldThrowImpl extends XExpressionImpl implements ShouldThrow {
/**
* The cached value of the '{@link #getType() Type}' containment reference.
*
*
* @see #getType()
* @generated
* @ordered
*/
protected JvmTypeReference type;
/**
* The cached value of the '{@link #getExpression() Expression}' containment reference.
*
*
* @see #getExpression()
* @generated
* @ordered
*/
protected XExpression expression;
/**
*
*
* @generated
*/
protected ShouldThrowImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return JnarioPackage.Literals.SHOULD_THROW;
}
/**
*
*
* @generated
*/
public JvmTypeReference getType() {
return type;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetType(JvmTypeReference newType, NotificationChain msgs) {
JvmTypeReference oldType = type;
type = newType;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, JnarioPackage.SHOULD_THROW__TYPE, oldType, newType);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setType(JvmTypeReference newType) {
if (newType != type) {
NotificationChain msgs = null;
if (type != null)
msgs = ((InternalEObject)type).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - JnarioPackage.SHOULD_THROW__TYPE, null, msgs);
if (newType != null)
msgs = ((InternalEObject)newType).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - JnarioPackage.SHOULD_THROW__TYPE, null, msgs);
msgs = basicSetType(newType, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, JnarioPackage.SHOULD_THROW__TYPE, newType, newType));
}
/**
*
*
* @generated
*/
public XExpression getExpression() {
return expression;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetExpression(XExpression newExpression, NotificationChain msgs) {
XExpression oldExpression = expression;
expression = newExpression;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, JnarioPackage.SHOULD_THROW__EXPRESSION, oldExpression, newExpression);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setExpression(XExpression newExpression) {
if (newExpression != expression) {
NotificationChain msgs = null;
if (expression != null)
msgs = ((InternalEObject)expression).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - JnarioPackage.SHOULD_THROW__EXPRESSION, null, msgs);
if (newExpression != null)
msgs = ((InternalEObject)newExpression).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - JnarioPackage.SHOULD_THROW__EXPRESSION, null, msgs);
msgs = basicSetExpression(newExpression, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, JnarioPackage.SHOULD_THROW__EXPRESSION, newExpression, newExpression));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case JnarioPackage.SHOULD_THROW__TYPE:
return basicSetType(null, msgs);
case JnarioPackage.SHOULD_THROW__EXPRESSION:
return basicSetExpression(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType) {
switch (featureID) {
case JnarioPackage.SHOULD_THROW__TYPE:
return getType();
case JnarioPackage.SHOULD_THROW__EXPRESSION:
return getExpression();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue) {
switch (featureID) {
case JnarioPackage.SHOULD_THROW__TYPE:
setType((JvmTypeReference)newValue);
return;
case JnarioPackage.SHOULD_THROW__EXPRESSION:
setExpression((XExpression)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID) {
switch (featureID) {
case JnarioPackage.SHOULD_THROW__TYPE:
setType((JvmTypeReference)null);
return;
case JnarioPackage.SHOULD_THROW__EXPRESSION:
setExpression((XExpression)null);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID) {
switch (featureID) {
case JnarioPackage.SHOULD_THROW__TYPE:
return type != null;
case JnarioPackage.SHOULD_THROW__EXPRESSION:
return expression != null;
}
return super.eIsSet(featureID);
}
} //ShouldThrowImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy