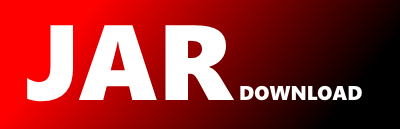
org.jnario.lib.Assert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
package org.jnario.lib;
/*
* copied from org.junit
*/
public class Assert {
/**
* Asserts that a condition is true. If it isn't it throws an
* {@link AssertionError} with the given message.
*
* @param message
* the identifying message for the {@link AssertionError} (null
* okay)
* @param condition
* condition to be checked
*/
static public void assertTrue(String message, boolean condition) {
if (!condition)
fail(message);
}
/**
* Asserts that a condition is false. If it isn't it throws an
* {@link AssertionError} with the given message.
*
* @param message
* the identifying message for the {@link AssertionError} (null
* okay)
* @param condition
* condition to be checked
*/
static public void assertFalse(String message, boolean condition) {
assertTrue(message, !condition);
}
/**
* Fails a test with the given message.
*
* @param message
* the identifying message for the {@link AssertionError} (null
* okay)
* @see AssertionError
*/
static public void fail(String message) {
if (message == null)
throw new AssertionError();
throw new AssertionError(message);
}
/**
* Asserts that an object isn't null. If it is an {@link AssertionError} is
* thrown with the given message.
*
* @param message
* the identifying message for the {@link AssertionError} (null
* okay)
* @param object
* Object to check or null
*/
static public void assertNotNull(String message, Object object) {
assertTrue(message, object != null);
}
/**
* Asserts that an object isn't null. If it is an {@link AssertionError} is
* thrown.
*
* @param object
* Object to check or null
*/
static public void assertNotNull(Object object) {
assertNotNull(null, object);
}
/**
* Asserts that an object is null. If it is not, an {@link AssertionError}
* is thrown with the given message.
*
* @param message
* the identifying message for the {@link AssertionError} (null
* okay)
* @param object
* Object to check or null
*/
static public void assertNull(String message, Object object) {
assertTrue(message, object == null);
}
/**
* Asserts that an object is null. If it isn't an {@link AssertionError} is
* thrown.
*
* @param object
* Object to check or null
*/
static public void assertNull(Object object) {
assertNull(null, object);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy