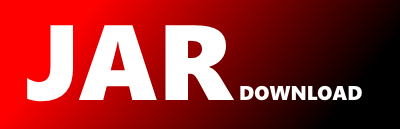
org.jnario.lib.StringConversions Maven / Gradle / Ivy
Show all versions of org.jnario.standalone Show documentation
/*******************************************************************************
* Copyright (c) 2012 BMW Car IT and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.jnario.lib;
import static com.google.common.collect.Lists.transform;
import static java.util.Arrays.asList;
import java.math.BigDecimal;
import java.util.List;
import com.google.common.base.Function;
/**
* Jnario extensions to convert string values into other formats.
* Useful when converting {@link StepArguments}.
*
* @author Sebastian Benz - Initial contribution and API
*/
public class StringConversions {
/**
* Parses the string argument as a signed decimal integer. The
* characters in the string must all be decimal digits, except that
* the first character may be an ASCII minus sign '-'
* ('\u002D'
) to indicate a negative value. The resulting
* integer value is returned, exactly as if the argument and the radix
* 10 were given as arguments to the {@link Integer#parseInt(java.lang.String, int)} method.
*
* @param s a String
containing the int
* representation to be parsed
* @return the integer value represented by the argument in decimal.
* @exception NumberFormatException if the string does not contain a
* parsable integer.
* @see java.lang.Integer#parseInt(String)
*/
public static int toInt(String s) {
return Integer.parseInt(s);
}
/**
* Parses the string argument as a signed decimal
* long
. The characters in the string must all be
* decimal digits, except that the first character may be an ASCII
* minus sign '-'
(\u002D'
) to
* indicate a negative value. The resulting long
* value is returned, exactly as if the argument and the radix
* 10
were given as arguments to the {@link
* Long#parseLong(java.lang.String, int)} method.
*
* Note that neither the character L
* ('\u004C'
) nor l
* ('\u006C'
) is permitted to appear at the end
* of the string as a type indicator, as would be permitted in
* Java programming language source code.
*
* @param s a String
containing the long
* representation to be parsed
* @return the long
represented by the argument in
* decimal.
* @exception NumberFormatException if the string does not contain a
* parsable long
.
* @see java.lang.Long#parseLong(String)
*/
public static long toLong(String s) {
return Long.parseLong(s);
}
/**
* Returns a new double
initialized to the value
* represented by the specified String
, as performed
* by the valueOf
method of class
* Double
.
*
* @param s the string to be parsed.
* @return the double
value represented by the string
* argument.
* @exception NumberFormatException if the string does not contain
* a parsable double
.
* @see java.lang.Double#parseDouble(String)
*/
public static double toDouble(String s) {
return Double.parseDouble(s);
}
/**
* Returns a Boolean
with a value represented by the
* specified string. The Boolean
returned represents a
* true value if the string argument is not null
* and is equal, ignoring case, to the string {@code "true"}.
*
* @param s a string.
* @return the Boolean
value represented by the string.
* @see java.lang.Boolean#parseBoolean(String)
*/
public static boolean toBool(String s) {
return Boolean.parseBoolean(s);
}
/**
* Translates the string representation of a {@code BigDecimal}
* into a {@code BigDecimal}. The string representation consists
* of an optional sign, {@code '+'} ( '\u002B') or
* {@code '-'} ('\u002D'), followed by a sequence of
* zero or more decimal digits ("the integer"), optionally
* followed by a fraction, optionally followed by an exponent.
*/
public static BigDecimal toBigDecimal(String s) {
if("0".equals(s)){
return BigDecimal.ZERO;
}
if("1".equals(s)){
return BigDecimal.ONE;
}
if("10".equals(s)){
return BigDecimal.TEN;
}
return new BigDecimal(s);
}
/**
* Convert a comma separated value string into a {@link List}.
*
* @param s a list with comma separated values
* @return a list with the trimmed values
*/
public static List toList(String s){
return transform(asList(s.split(",\\s*")), new Function() {
public String apply(String input){
return input.trim();
}
});
}
}