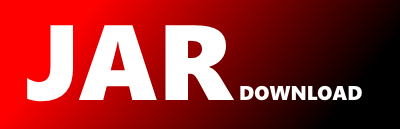
org.jnario.spec.doc.SpecDocGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/**
* Copyright (c) 2012 BMW Car IT and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.jnario.spec.doc;
import com.google.common.base.Objects;
import com.google.inject.Inject;
import java.util.Arrays;
import java.util.List;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtend.core.xtend.XtendClass;
import org.eclipse.xtend.core.xtend.XtendMember;
import org.eclipse.xtend.core.xtend.XtendPackage;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.XExpression;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
import org.jnario.ExampleTable;
import org.jnario.doc.AbstractDocGenerator;
import org.jnario.doc.Filter;
import org.jnario.doc.FilterExtractor;
import org.jnario.doc.FilteringResult;
import org.jnario.doc.HtmlFile;
import org.jnario.spec.naming.ExampleNameProvider;
import org.jnario.spec.spec.Example;
import org.jnario.spec.spec.ExampleGroup;
@SuppressWarnings("all")
public class SpecDocGenerator extends AbstractDocGenerator {
@Inject
@Extension
private ExampleNameProvider _exampleNameProvider;
@Inject
@Extension
private FilterExtractor _filterExtractor;
public HtmlFile createHtmlFile(final XtendClass xtendClass) {
HtmlFile _xblockexpression = null;
{
if ((!(xtendClass instanceof ExampleGroup))) {
return HtmlFile.EMPTY_FILE;
}
final ExampleGroup exampleGroup = ((ExampleGroup) xtendClass);
final Procedure1 _function = new Procedure1() {
public void apply(final HtmlFile it) {
String _javaClassName = SpecDocGenerator.this._exampleNameProvider.toJavaClassName(exampleGroup);
it.setName(_javaClassName);
String _asTitle = SpecDocGenerator.this.asTitle(exampleGroup);
it.setTitle(_asTitle);
CharSequence _generateContent = SpecDocGenerator.this.generateContent(exampleGroup);
it.setContent(_generateContent);
String _root = SpecDocGenerator.this.root(exampleGroup);
it.setRootFolder(_root);
EObject _eContainer = xtendClass.eContainer();
CharSequence _pre = SpecDocGenerator.this.pre(_eContainer, "lang-spec");
it.setSourceCode(_pre);
String _fileName = SpecDocGenerator.this.fileName(xtendClass);
it.setFileName(_fileName);
String _executionStateClass = SpecDocGenerator.this.executionStateClass(exampleGroup);
it.setExecutionStatus(_executionStateClass);
}
};
_xblockexpression = HtmlFile.newHtmlFile(_function);
}
return _xblockexpression;
}
private CharSequence generateContent(final ExampleGroup exampleGroup) {
StringConcatenation _builder = new StringConcatenation();
CharSequence _generateDoc = this.generateDoc(exampleGroup);
_builder.append(_generateDoc, "");
_builder.newLineIfNotEmpty();
StringConcatenation _generateMembers = this.generateMembers(exampleGroup, 1);
_builder.append(_generateMembers, "");
_builder.newLineIfNotEmpty();
return _builder;
}
private StringConcatenation generateMembers(final ExampleGroup exampleGroup, final int level) {
final StringConcatenation result = new StringConcatenation();
boolean inList = false;
EList _members = exampleGroup.getMembers();
final Function1 _function = new Function1() {
public Boolean apply(final XtendMember it) {
boolean _or = false;
if (((it instanceof Example) || (it instanceof ExampleGroup))) {
_or = true;
} else {
_or = (it instanceof ExampleTable);
}
return Boolean.valueOf(_or);
}
};
final Iterable members = IterableExtensions.filter(_members, _function);
for (final XtendMember member : members) {
{
final boolean isExampleGroup = (member instanceof ExampleGroup);
if ((inList && (!isExampleGroup))) {
result.append("");
CharSequence _generate = this.generate(member, level);
result.append(_generate);
result.append(" ");
} else {
if (((!inList) && (!isExampleGroup))) {
result.append("");
result.append("- ");
CharSequence _generate_1 = this.generate(member, level);
result.append(_generate_1);
result.append("
");
inList = true;
} else {
boolean _and = false;
if (!inList) {
_and = false;
} else {
_and = isExampleGroup;
}
if (_and) {
result.append("
");
CharSequence _generate_2 = this.generate(member, level);
result.append(_generate_2);
inList = false;
} else {
boolean _and_1 = false;
if (!(!inList)) {
_and_1 = false;
} else {
_and_1 = isExampleGroup;
}
if (_and_1) {
CharSequence _generate_3 = this.generate(member, level);
result.append(_generate_3);
}
}
}
}
}
}
if (inList) {
result.append("");
}
return result;
}
private CharSequence generateDoc(final EObject eObject) {
StringConcatenation _builder = new StringConcatenation();
{
String _documentation = this.documentation(eObject);
boolean _notEquals = (!Objects.equal(_documentation, null));
if (_notEquals) {
String _documentation_1 = this.documentation(eObject);
String _markdown2Html = this.markdown2Html(_documentation_1);
_builder.append(_markdown2Html, "");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
protected CharSequence _generate(final XtendMember member, final int level) {
StringConcatenation _builder = new StringConcatenation();
return _builder;
}
protected CharSequence _generate(final Example example, final int level) {
CharSequence _xblockexpression = null;
{
String docString = this.documentation(example);
List filters = CollectionLiterals.emptyList();
boolean _notEquals = (!Objects.equal(docString, null));
if (_notEquals) {
final FilteringResult filterResult = this._filterExtractor.apply(docString);
List _filters = filterResult.getFilters();
filters = _filters;
String _string = filterResult.getString();
docString = _string;
String _markdown2Html = this.markdown2Html(docString);
docString = _markdown2Html;
}
StringConcatenation _builder = new StringConcatenation();
{
String _name = example.getName();
boolean _notEquals_1 = (!Objects.equal(_name, null));
if (_notEquals_1) {
_builder.append("");
String _describe = this._exampleNameProvider.describe(example);
String _decode = this.decode(_describe);
_builder.append(_decode, "");
_builder.append("
");
_builder.newLineIfNotEmpty();
}
}
_builder.append(docString, "");
_builder.newLineIfNotEmpty();
{
boolean _and = false;
boolean _isPending = example.isPending();
boolean _not = (!_isPending);
if (!_not) {
_and = false;
} else {
boolean _eIsSet = example.eIsSet(XtendPackage.Literals.XTEND_EXECUTABLE__EXPRESSION);
_and = _eIsSet;
}
if (_and) {
CharSequence _codeBlock = this.toCodeBlock(example, filters);
_builder.append(_codeBlock, "");
_builder.newLineIfNotEmpty();
}
}
String _errorMessage = this.errorMessage(example);
_builder.append(_errorMessage, "");
_builder.newLineIfNotEmpty();
_xblockexpression = _builder;
}
return _xblockexpression;
}
public CharSequence toCodeBlock(final Example example, final List filters) {
CharSequence _xblockexpression = null;
{
String prefix = "";
String _apply = this.apply(filters, prefix);
prefix = _apply;
XExpression _expression = example.getExpression();
final String code = this.serialize(_expression, filters);
int _length = code.length();
boolean _equals = (_length == 0);
if (_equals) {
return "";
}
StringConcatenation _builder = new StringConcatenation();
_builder.append(prefix, "");
_builder.newLineIfNotEmpty();
_builder.append(code, "");
_builder.append("
");
_xblockexpression = _builder;
}
return _xblockexpression;
}
protected CharSequence _generate(final ExampleTable table, final int level) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
String _fieldName_1 = this._exampleNameProvider.toFieldName(table);
String _title = this.toTitle(_fieldName_1);
_builder.append(_title, "");
_builder.append("
");
_builder.newLineIfNotEmpty();
CharSequence _generateDoc = this.generateDoc(table);
_builder.append(_generateDoc, "");
_builder.newLineIfNotEmpty();
CharSequence _generate = super.generate(table);
_builder.append(_generate, "");
_builder.newLineIfNotEmpty();
return _builder;
}
protected CharSequence _generate(final ExampleGroup exampleGroup, final int level) {
StringConcatenation _builder = new StringConcatenation();
{
if ((level > 1)) {
_builder.append("");
_builder.newLine();
}
}
_builder.append("");
String _asTitle = this.asTitle(exampleGroup);
_builder.append(_asTitle, "");
_builder.append(" ");
_builder.newLineIfNotEmpty();
CharSequence _generateDoc = this.generateDoc(exampleGroup);
_builder.append(_generateDoc, "");
_builder.newLineIfNotEmpty();
StringConcatenation _generateMembers = this.generateMembers(exampleGroup, (level + 1));
_builder.append(_generateMembers, "");
_builder.newLineIfNotEmpty();
{
if ((level > 1)) {
_builder.append("");
_builder.newLine();
}
}
return _builder;
}
private String header(final ExampleGroup exampleGroup, final int level) {
String _xifexpression = null;
if ((level <= 1)) {
_xifexpression = "3";
} else {
String _xifexpression_1 = null;
if ((level == 2)) {
_xifexpression_1 = "4";
} else {
_xifexpression_1 = "5";
}
_xifexpression = _xifexpression_1;
}
return _xifexpression;
}
protected String _asTitle(final ExampleGroup exampleGroup) {
String _describe = this._exampleNameProvider.describe(exampleGroup);
return this.toTitle(_describe);
}
protected String _asTitle(final Example example) {
String _describe = this._exampleNameProvider.describe(example);
return this.toTitle(_describe);
}
public CharSequence generate(final XtendMember example, final int level) {
if (example instanceof Example) {
return _generate((Example)example, level);
} else if (example instanceof ExampleGroup) {
return _generate((ExampleGroup)example, level);
} else if (example instanceof ExampleTable) {
return _generate((ExampleTable)example, level);
} else if (example != null) {
return _generate(example, level);
} else {
throw new IllegalArgumentException("Unhandled parameter types: " +
Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy