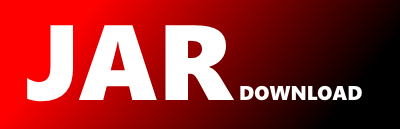
org.jnario.spec.services.SpecGrammarAccess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/*
* generated by Xtext
*/
package org.jnario.spec.services;
import com.google.inject.Singleton;
import com.google.inject.Inject;
import java.util.List;
import org.eclipse.xtext.*;
import org.eclipse.xtext.service.GrammarProvider;
import org.eclipse.xtext.service.AbstractElementFinder.*;
import org.eclipse.xtend.core.services.XtendGrammarAccess;
import org.eclipse.xtext.xbase.annotations.services.XbaseWithAnnotationsGrammarAccess;
import org.eclipse.xtext.xbase.services.XbaseGrammarAccess;
import org.eclipse.xtext.xbase.services.XtypeGrammarAccess;
@Singleton
public class SpecGrammarAccess extends AbstractGrammarElementFinder {
public class SpecFileElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "SpecFile");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Action cSpecFileAction_0 = (Action)cGroup.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cPackageKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cPackageAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cPackageQualifiedNameParserRuleCall_1_1_0 = (RuleCall)cPackageAssignment_1_1.eContents().get(0);
private final Assignment cImportSectionAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cImportSectionXImportSectionParserRuleCall_2_0 = (RuleCall)cImportSectionAssignment_2.eContents().get(0);
private final Assignment cXtendTypesAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cXtendTypesTypeParserRuleCall_3_0 = (RuleCall)cXtendTypesAssignment_3.eContents().get(0);
//SpecFile:
// {SpecFile} ("package" package=QualifiedName)? importSection=XImportSection? xtendTypes+=Type*;
public ParserRule getRule() { return rule; }
//{SpecFile} ("package" package=QualifiedName)? importSection=XImportSection? xtendTypes+=Type*
public Group getGroup() { return cGroup; }
//{SpecFile}
public Action getSpecFileAction_0() { return cSpecFileAction_0; }
//("package" package=QualifiedName)?
public Group getGroup_1() { return cGroup_1; }
//"package"
public Keyword getPackageKeyword_1_0() { return cPackageKeyword_1_0; }
//package=QualifiedName
public Assignment getPackageAssignment_1_1() { return cPackageAssignment_1_1; }
//QualifiedName
public RuleCall getPackageQualifiedNameParserRuleCall_1_1_0() { return cPackageQualifiedNameParserRuleCall_1_1_0; }
//importSection=XImportSection?
public Assignment getImportSectionAssignment_2() { return cImportSectionAssignment_2; }
//XImportSection
public RuleCall getImportSectionXImportSectionParserRuleCall_2_0() { return cImportSectionXImportSectionParserRuleCall_2_0; }
//xtendTypes+=Type*
public Assignment getXtendTypesAssignment_3() { return cXtendTypesAssignment_3; }
//Type
public RuleCall getXtendTypesTypeParserRuleCall_3_0() { return cXtendTypesTypeParserRuleCall_3_0; }
}
public class TypeElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Type");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Action cXtendTypeDeclarationAction_0 = (Action)cGroup.eContents().get(0);
private final Assignment cAnnotationsAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cAnnotationsXAnnotationParserRuleCall_1_0 = (RuleCall)cAnnotationsAssignment_1.eContents().get(0);
private final Alternatives cAlternatives_2 = (Alternatives)cGroup.eContents().get(2);
private final Group cGroup_2_0 = (Group)cAlternatives_2.eContents().get(0);
private final Action cXtendClassAnnotationInfoAction_2_0_0 = (Action)cGroup_2_0.eContents().get(0);
private final Assignment cModifiersAssignment_2_0_1 = (Assignment)cGroup_2_0.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_0_1_0 = (RuleCall)cModifiersAssignment_2_0_1.eContents().get(0);
private final Keyword cClassKeyword_2_0_2 = (Keyword)cGroup_2_0.eContents().get(2);
private final Assignment cNameAssignment_2_0_3 = (Assignment)cGroup_2_0.eContents().get(3);
private final RuleCall cNameValidIDParserRuleCall_2_0_3_0 = (RuleCall)cNameAssignment_2_0_3.eContents().get(0);
private final Group cGroup_2_0_4 = (Group)cGroup_2_0.eContents().get(4);
private final Keyword cLessThanSignKeyword_2_0_4_0 = (Keyword)cGroup_2_0_4.eContents().get(0);
private final Assignment cTypeParametersAssignment_2_0_4_1 = (Assignment)cGroup_2_0_4.eContents().get(1);
private final RuleCall cTypeParametersJvmTypeParameterParserRuleCall_2_0_4_1_0 = (RuleCall)cTypeParametersAssignment_2_0_4_1.eContents().get(0);
private final Group cGroup_2_0_4_2 = (Group)cGroup_2_0_4.eContents().get(2);
private final Keyword cCommaKeyword_2_0_4_2_0 = (Keyword)cGroup_2_0_4_2.eContents().get(0);
private final Assignment cTypeParametersAssignment_2_0_4_2_1 = (Assignment)cGroup_2_0_4_2.eContents().get(1);
private final RuleCall cTypeParametersJvmTypeParameterParserRuleCall_2_0_4_2_1_0 = (RuleCall)cTypeParametersAssignment_2_0_4_2_1.eContents().get(0);
private final Keyword cGreaterThanSignKeyword_2_0_4_3 = (Keyword)cGroup_2_0_4.eContents().get(3);
private final Group cGroup_2_0_5 = (Group)cGroup_2_0.eContents().get(5);
private final Keyword cExtendsKeyword_2_0_5_0 = (Keyword)cGroup_2_0_5.eContents().get(0);
private final Assignment cExtendsAssignment_2_0_5_1 = (Assignment)cGroup_2_0_5.eContents().get(1);
private final RuleCall cExtendsJvmParameterizedTypeReferenceParserRuleCall_2_0_5_1_0 = (RuleCall)cExtendsAssignment_2_0_5_1.eContents().get(0);
private final Group cGroup_2_0_6 = (Group)cGroup_2_0.eContents().get(6);
private final Keyword cImplementsKeyword_2_0_6_0 = (Keyword)cGroup_2_0_6.eContents().get(0);
private final Assignment cImplementsAssignment_2_0_6_1 = (Assignment)cGroup_2_0_6.eContents().get(1);
private final RuleCall cImplementsJvmParameterizedTypeReferenceParserRuleCall_2_0_6_1_0 = (RuleCall)cImplementsAssignment_2_0_6_1.eContents().get(0);
private final Group cGroup_2_0_6_2 = (Group)cGroup_2_0_6.eContents().get(2);
private final Keyword cCommaKeyword_2_0_6_2_0 = (Keyword)cGroup_2_0_6_2.eContents().get(0);
private final Assignment cImplementsAssignment_2_0_6_2_1 = (Assignment)cGroup_2_0_6_2.eContents().get(1);
private final RuleCall cImplementsJvmParameterizedTypeReferenceParserRuleCall_2_0_6_2_1_0 = (RuleCall)cImplementsAssignment_2_0_6_2_1.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_0_7 = (Keyword)cGroup_2_0.eContents().get(7);
private final Assignment cMembersAssignment_2_0_8 = (Assignment)cGroup_2_0.eContents().get(8);
private final RuleCall cMembersMemberParserRuleCall_2_0_8_0 = (RuleCall)cMembersAssignment_2_0_8.eContents().get(0);
private final Keyword cRightCurlyBracketKeyword_2_0_9 = (Keyword)cGroup_2_0.eContents().get(9);
private final Group cGroup_2_1 = (Group)cAlternatives_2.eContents().get(1);
private final Action cXtendInterfaceAnnotationInfoAction_2_1_0 = (Action)cGroup_2_1.eContents().get(0);
private final Assignment cModifiersAssignment_2_1_1 = (Assignment)cGroup_2_1.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_1_1_0 = (RuleCall)cModifiersAssignment_2_1_1.eContents().get(0);
private final Keyword cInterfaceKeyword_2_1_2 = (Keyword)cGroup_2_1.eContents().get(2);
private final Assignment cNameAssignment_2_1_3 = (Assignment)cGroup_2_1.eContents().get(3);
private final RuleCall cNameValidIDParserRuleCall_2_1_3_0 = (RuleCall)cNameAssignment_2_1_3.eContents().get(0);
private final Group cGroup_2_1_4 = (Group)cGroup_2_1.eContents().get(4);
private final Keyword cLessThanSignKeyword_2_1_4_0 = (Keyword)cGroup_2_1_4.eContents().get(0);
private final Assignment cTypeParametersAssignment_2_1_4_1 = (Assignment)cGroup_2_1_4.eContents().get(1);
private final RuleCall cTypeParametersJvmTypeParameterParserRuleCall_2_1_4_1_0 = (RuleCall)cTypeParametersAssignment_2_1_4_1.eContents().get(0);
private final Group cGroup_2_1_4_2 = (Group)cGroup_2_1_4.eContents().get(2);
private final Keyword cCommaKeyword_2_1_4_2_0 = (Keyword)cGroup_2_1_4_2.eContents().get(0);
private final Assignment cTypeParametersAssignment_2_1_4_2_1 = (Assignment)cGroup_2_1_4_2.eContents().get(1);
private final RuleCall cTypeParametersJvmTypeParameterParserRuleCall_2_1_4_2_1_0 = (RuleCall)cTypeParametersAssignment_2_1_4_2_1.eContents().get(0);
private final Keyword cGreaterThanSignKeyword_2_1_4_3 = (Keyword)cGroup_2_1_4.eContents().get(3);
private final Group cGroup_2_1_5 = (Group)cGroup_2_1.eContents().get(5);
private final Keyword cExtendsKeyword_2_1_5_0 = (Keyword)cGroup_2_1_5.eContents().get(0);
private final Assignment cExtendsAssignment_2_1_5_1 = (Assignment)cGroup_2_1_5.eContents().get(1);
private final RuleCall cExtendsJvmParameterizedTypeReferenceParserRuleCall_2_1_5_1_0 = (RuleCall)cExtendsAssignment_2_1_5_1.eContents().get(0);
private final Group cGroup_2_1_5_2 = (Group)cGroup_2_1_5.eContents().get(2);
private final Keyword cCommaKeyword_2_1_5_2_0 = (Keyword)cGroup_2_1_5_2.eContents().get(0);
private final Assignment cExtendsAssignment_2_1_5_2_1 = (Assignment)cGroup_2_1_5_2.eContents().get(1);
private final RuleCall cExtendsJvmParameterizedTypeReferenceParserRuleCall_2_1_5_2_1_0 = (RuleCall)cExtendsAssignment_2_1_5_2_1.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_1_6 = (Keyword)cGroup_2_1.eContents().get(6);
private final Assignment cMembersAssignment_2_1_7 = (Assignment)cGroup_2_1.eContents().get(7);
private final RuleCall cMembersMemberParserRuleCall_2_1_7_0 = (RuleCall)cMembersAssignment_2_1_7.eContents().get(0);
private final Keyword cRightCurlyBracketKeyword_2_1_8 = (Keyword)cGroup_2_1.eContents().get(8);
private final Group cGroup_2_2 = (Group)cAlternatives_2.eContents().get(2);
private final Action cXtendEnumAnnotationInfoAction_2_2_0 = (Action)cGroup_2_2.eContents().get(0);
private final Assignment cModifiersAssignment_2_2_1 = (Assignment)cGroup_2_2.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_2_1_0 = (RuleCall)cModifiersAssignment_2_2_1.eContents().get(0);
private final Keyword cEnumKeyword_2_2_2 = (Keyword)cGroup_2_2.eContents().get(2);
private final Assignment cNameAssignment_2_2_3 = (Assignment)cGroup_2_2.eContents().get(3);
private final RuleCall cNameValidIDParserRuleCall_2_2_3_0 = (RuleCall)cNameAssignment_2_2_3.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_2_4 = (Keyword)cGroup_2_2.eContents().get(4);
private final Group cGroup_2_2_5 = (Group)cGroup_2_2.eContents().get(5);
private final Assignment cMembersAssignment_2_2_5_0 = (Assignment)cGroup_2_2_5.eContents().get(0);
private final RuleCall cMembersXtendEnumLiteralParserRuleCall_2_2_5_0_0 = (RuleCall)cMembersAssignment_2_2_5_0.eContents().get(0);
private final Group cGroup_2_2_5_1 = (Group)cGroup_2_2_5.eContents().get(1);
private final Keyword cCommaKeyword_2_2_5_1_0 = (Keyword)cGroup_2_2_5_1.eContents().get(0);
private final Assignment cMembersAssignment_2_2_5_1_1 = (Assignment)cGroup_2_2_5_1.eContents().get(1);
private final RuleCall cMembersXtendEnumLiteralParserRuleCall_2_2_5_1_1_0 = (RuleCall)cMembersAssignment_2_2_5_1_1.eContents().get(0);
private final Keyword cSemicolonKeyword_2_2_6 = (Keyword)cGroup_2_2.eContents().get(6);
private final Keyword cRightCurlyBracketKeyword_2_2_7 = (Keyword)cGroup_2_2.eContents().get(7);
private final Group cGroup_2_3 = (Group)cAlternatives_2.eContents().get(3);
private final Action cXtendAnnotationTypeAnnotationInfoAction_2_3_0 = (Action)cGroup_2_3.eContents().get(0);
private final Assignment cModifiersAssignment_2_3_1 = (Assignment)cGroup_2_3.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_3_1_0 = (RuleCall)cModifiersAssignment_2_3_1.eContents().get(0);
private final Keyword cAnnotationKeyword_2_3_2 = (Keyword)cGroup_2_3.eContents().get(2);
private final Assignment cNameAssignment_2_3_3 = (Assignment)cGroup_2_3.eContents().get(3);
private final RuleCall cNameValidIDParserRuleCall_2_3_3_0 = (RuleCall)cNameAssignment_2_3_3.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_3_4 = (Keyword)cGroup_2_3.eContents().get(4);
private final Assignment cMembersAssignment_2_3_5 = (Assignment)cGroup_2_3.eContents().get(5);
private final RuleCall cMembersAnnotationFieldParserRuleCall_2_3_5_0 = (RuleCall)cMembersAssignment_2_3_5.eContents().get(0);
private final Keyword cRightCurlyBracketKeyword_2_3_6 = (Keyword)cGroup_2_3.eContents().get(6);
private final Group cGroup_2_4 = (Group)cAlternatives_2.eContents().get(4);
private final Action cExampleGroupAnnotationInfoAction_2_4_0 = (Action)cGroup_2_4.eContents().get(0);
private final Assignment cPreambleAssignment_2_4_1 = (Assignment)cGroup_2_4.eContents().get(1);
private final Keyword cPreambleDescribeKeyword_2_4_1_0 = (Keyword)cPreambleAssignment_2_4_1.eContents().get(0);
private final Assignment cTargetTypeAssignment_2_4_2 = (Assignment)cGroup_2_4.eContents().get(2);
private final RuleCall cTargetTypeJvmTypeReferenceParserRuleCall_2_4_2_0 = (RuleCall)cTargetTypeAssignment_2_4_2.eContents().get(0);
private final Assignment cNameAssignment_2_4_3 = (Assignment)cGroup_2_4.eContents().get(3);
private final RuleCall cNameSTRINGTerminalRuleCall_2_4_3_0 = (RuleCall)cNameAssignment_2_4_3.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_4_4 = (Keyword)cGroup_2_4.eContents().get(4);
private final Assignment cMembersAssignment_2_4_5 = (Assignment)cGroup_2_4.eContents().get(5);
private final RuleCall cMembersMemberParserRuleCall_2_4_5_0 = (RuleCall)cMembersAssignment_2_4_5.eContents().get(0);
private final Keyword cRightCurlyBracketKeyword_2_4_6 = (Keyword)cGroup_2_4.eContents().get(6);
//Type returns xtend::XtendTypeDeclaration:
// {xtend::XtendTypeDeclaration} annotations+=XAnnotation* ({xtend::XtendClass.annotationInfo=current}
// modifiers+=CommonModifier* "class" name=ValidID ("<" typeParameters+=JvmTypeParameter (","
// typeParameters+=JvmTypeParameter)* ">")? ("extends" extends=JvmParameterizedTypeReference)? ("implements"
// implements+=JvmParameterizedTypeReference ("," implements+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}"
// | {xtend::XtendInterface.annotationInfo=current} modifiers+=CommonModifier* "interface" name=ValidID ("<"
// typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? ("extends"
// extends+=JvmParameterizedTypeReference ("," extends+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" |
// {xtend::XtendEnum.annotationInfo=current} modifiers+=CommonModifier* "enum" name=ValidID "{"
// (members+=XtendEnumLiteral ("," members+=XtendEnumLiteral)*)? ";"? "}" |
// {xtend::XtendAnnotationType.annotationInfo=current} modifiers+=CommonModifier* "annotation" name=ValidID "{"
// members+=AnnotationField* "}" | {ExampleGroup.annotationInfo=current} preamble="describe" targetType=JvmTypeReference?
// name=STRING? "{" members+=Member* "}");
public ParserRule getRule() { return rule; }
//{xtend::XtendTypeDeclaration} annotations+=XAnnotation* ({xtend::XtendClass.annotationInfo=current}
//modifiers+=CommonModifier* "class" name=ValidID ("<" typeParameters+=JvmTypeParameter (","
//typeParameters+=JvmTypeParameter)* ">")? ("extends" extends=JvmParameterizedTypeReference)? ("implements"
//implements+=JvmParameterizedTypeReference ("," implements+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" |
//{xtend::XtendInterface.annotationInfo=current} modifiers+=CommonModifier* "interface" name=ValidID ("<"
//typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? ("extends"
//extends+=JvmParameterizedTypeReference ("," extends+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" |
//{xtend::XtendEnum.annotationInfo=current} modifiers+=CommonModifier* "enum" name=ValidID "{" (members+=XtendEnumLiteral
//("," members+=XtendEnumLiteral)*)? ";"? "}" | {xtend::XtendAnnotationType.annotationInfo=current}
//modifiers+=CommonModifier* "annotation" name=ValidID "{" members+=AnnotationField* "}" |
//{ExampleGroup.annotationInfo=current} preamble="describe" targetType=JvmTypeReference? name=STRING? "{"
//members+=Member* "}")
public Group getGroup() { return cGroup; }
//{xtend::XtendTypeDeclaration}
public Action getXtendTypeDeclarationAction_0() { return cXtendTypeDeclarationAction_0; }
//annotations+=XAnnotation*
public Assignment getAnnotationsAssignment_1() { return cAnnotationsAssignment_1; }
//XAnnotation
public RuleCall getAnnotationsXAnnotationParserRuleCall_1_0() { return cAnnotationsXAnnotationParserRuleCall_1_0; }
//{xtend::XtendClass.annotationInfo=current} modifiers+=CommonModifier* "class" name=ValidID ("<"
//typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? ("extends"
//extends=JvmParameterizedTypeReference)? ("implements" implements+=JvmParameterizedTypeReference (","
//implements+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" | {xtend::XtendInterface.annotationInfo=current}
//modifiers+=CommonModifier* "interface" name=ValidID ("<" typeParameters+=JvmTypeParameter (","
//typeParameters+=JvmTypeParameter)* ">")? ("extends" extends+=JvmParameterizedTypeReference (","
//extends+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" | {xtend::XtendEnum.annotationInfo=current}
//modifiers+=CommonModifier* "enum" name=ValidID "{" (members+=XtendEnumLiteral ("," members+=XtendEnumLiteral)*)? ";"?
//"}" | {xtend::XtendAnnotationType.annotationInfo=current} modifiers+=CommonModifier* "annotation" name=ValidID "{"
//members+=AnnotationField* "}" | {ExampleGroup.annotationInfo=current} preamble="describe" targetType=JvmTypeReference?
//name=STRING? "{" members+=Member* "}"
public Alternatives getAlternatives_2() { return cAlternatives_2; }
//{xtend::XtendClass.annotationInfo=current} modifiers+=CommonModifier* "class" name=ValidID ("<"
//typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? ("extends"
//extends=JvmParameterizedTypeReference)? ("implements" implements+=JvmParameterizedTypeReference (","
//implements+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}"
public Group getGroup_2_0() { return cGroup_2_0; }
//{xtend::XtendClass.annotationInfo=current}
public Action getXtendClassAnnotationInfoAction_2_0_0() { return cXtendClassAnnotationInfoAction_2_0_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_0_1() { return cModifiersAssignment_2_0_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_0_1_0() { return cModifiersCommonModifierParserRuleCall_2_0_1_0; }
//"class"
public Keyword getClassKeyword_2_0_2() { return cClassKeyword_2_0_2; }
//name=ValidID
public Assignment getNameAssignment_2_0_3() { return cNameAssignment_2_0_3; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_0_3_0() { return cNameValidIDParserRuleCall_2_0_3_0; }
//("<" typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")?
public Group getGroup_2_0_4() { return cGroup_2_0_4; }
//"<"
public Keyword getLessThanSignKeyword_2_0_4_0() { return cLessThanSignKeyword_2_0_4_0; }
//typeParameters+=JvmTypeParameter
public Assignment getTypeParametersAssignment_2_0_4_1() { return cTypeParametersAssignment_2_0_4_1; }
//JvmTypeParameter
public RuleCall getTypeParametersJvmTypeParameterParserRuleCall_2_0_4_1_0() { return cTypeParametersJvmTypeParameterParserRuleCall_2_0_4_1_0; }
//("," typeParameters+=JvmTypeParameter)*
public Group getGroup_2_0_4_2() { return cGroup_2_0_4_2; }
//","
public Keyword getCommaKeyword_2_0_4_2_0() { return cCommaKeyword_2_0_4_2_0; }
//typeParameters+=JvmTypeParameter
public Assignment getTypeParametersAssignment_2_0_4_2_1() { return cTypeParametersAssignment_2_0_4_2_1; }
//JvmTypeParameter
public RuleCall getTypeParametersJvmTypeParameterParserRuleCall_2_0_4_2_1_0() { return cTypeParametersJvmTypeParameterParserRuleCall_2_0_4_2_1_0; }
//">"
public Keyword getGreaterThanSignKeyword_2_0_4_3() { return cGreaterThanSignKeyword_2_0_4_3; }
//("extends" extends=JvmParameterizedTypeReference)?
public Group getGroup_2_0_5() { return cGroup_2_0_5; }
//"extends"
public Keyword getExtendsKeyword_2_0_5_0() { return cExtendsKeyword_2_0_5_0; }
//extends=JvmParameterizedTypeReference
public Assignment getExtendsAssignment_2_0_5_1() { return cExtendsAssignment_2_0_5_1; }
//JvmParameterizedTypeReference
public RuleCall getExtendsJvmParameterizedTypeReferenceParserRuleCall_2_0_5_1_0() { return cExtendsJvmParameterizedTypeReferenceParserRuleCall_2_0_5_1_0; }
//("implements" implements+=JvmParameterizedTypeReference ("," implements+=JvmParameterizedTypeReference)*)?
public Group getGroup_2_0_6() { return cGroup_2_0_6; }
//"implements"
public Keyword getImplementsKeyword_2_0_6_0() { return cImplementsKeyword_2_0_6_0; }
//implements+=JvmParameterizedTypeReference
public Assignment getImplementsAssignment_2_0_6_1() { return cImplementsAssignment_2_0_6_1; }
//JvmParameterizedTypeReference
public RuleCall getImplementsJvmParameterizedTypeReferenceParserRuleCall_2_0_6_1_0() { return cImplementsJvmParameterizedTypeReferenceParserRuleCall_2_0_6_1_0; }
//("," implements+=JvmParameterizedTypeReference)*
public Group getGroup_2_0_6_2() { return cGroup_2_0_6_2; }
//","
public Keyword getCommaKeyword_2_0_6_2_0() { return cCommaKeyword_2_0_6_2_0; }
//implements+=JvmParameterizedTypeReference
public Assignment getImplementsAssignment_2_0_6_2_1() { return cImplementsAssignment_2_0_6_2_1; }
//JvmParameterizedTypeReference
public RuleCall getImplementsJvmParameterizedTypeReferenceParserRuleCall_2_0_6_2_1_0() { return cImplementsJvmParameterizedTypeReferenceParserRuleCall_2_0_6_2_1_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_0_7() { return cLeftCurlyBracketKeyword_2_0_7; }
//members+=Member*
public Assignment getMembersAssignment_2_0_8() { return cMembersAssignment_2_0_8; }
//Member
public RuleCall getMembersMemberParserRuleCall_2_0_8_0() { return cMembersMemberParserRuleCall_2_0_8_0; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_0_9() { return cRightCurlyBracketKeyword_2_0_9; }
//{xtend::XtendInterface.annotationInfo=current} modifiers+=CommonModifier* "interface" name=ValidID ("<"
//typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? ("extends"
//extends+=JvmParameterizedTypeReference ("," extends+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}"
public Group getGroup_2_1() { return cGroup_2_1; }
//{xtend::XtendInterface.annotationInfo=current}
public Action getXtendInterfaceAnnotationInfoAction_2_1_0() { return cXtendInterfaceAnnotationInfoAction_2_1_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_1_1() { return cModifiersAssignment_2_1_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_1_1_0() { return cModifiersCommonModifierParserRuleCall_2_1_1_0; }
//"interface"
public Keyword getInterfaceKeyword_2_1_2() { return cInterfaceKeyword_2_1_2; }
//name=ValidID
public Assignment getNameAssignment_2_1_3() { return cNameAssignment_2_1_3; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_1_3_0() { return cNameValidIDParserRuleCall_2_1_3_0; }
//("<" typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")?
public Group getGroup_2_1_4() { return cGroup_2_1_4; }
//"<"
public Keyword getLessThanSignKeyword_2_1_4_0() { return cLessThanSignKeyword_2_1_4_0; }
//typeParameters+=JvmTypeParameter
public Assignment getTypeParametersAssignment_2_1_4_1() { return cTypeParametersAssignment_2_1_4_1; }
//JvmTypeParameter
public RuleCall getTypeParametersJvmTypeParameterParserRuleCall_2_1_4_1_0() { return cTypeParametersJvmTypeParameterParserRuleCall_2_1_4_1_0; }
//("," typeParameters+=JvmTypeParameter)*
public Group getGroup_2_1_4_2() { return cGroup_2_1_4_2; }
//","
public Keyword getCommaKeyword_2_1_4_2_0() { return cCommaKeyword_2_1_4_2_0; }
//typeParameters+=JvmTypeParameter
public Assignment getTypeParametersAssignment_2_1_4_2_1() { return cTypeParametersAssignment_2_1_4_2_1; }
//JvmTypeParameter
public RuleCall getTypeParametersJvmTypeParameterParserRuleCall_2_1_4_2_1_0() { return cTypeParametersJvmTypeParameterParserRuleCall_2_1_4_2_1_0; }
//">"
public Keyword getGreaterThanSignKeyword_2_1_4_3() { return cGreaterThanSignKeyword_2_1_4_3; }
//("extends" extends+=JvmParameterizedTypeReference ("," extends+=JvmParameterizedTypeReference)*)?
public Group getGroup_2_1_5() { return cGroup_2_1_5; }
//"extends"
public Keyword getExtendsKeyword_2_1_5_0() { return cExtendsKeyword_2_1_5_0; }
//extends+=JvmParameterizedTypeReference
public Assignment getExtendsAssignment_2_1_5_1() { return cExtendsAssignment_2_1_5_1; }
//JvmParameterizedTypeReference
public RuleCall getExtendsJvmParameterizedTypeReferenceParserRuleCall_2_1_5_1_0() { return cExtendsJvmParameterizedTypeReferenceParserRuleCall_2_1_5_1_0; }
//("," extends+=JvmParameterizedTypeReference)*
public Group getGroup_2_1_5_2() { return cGroup_2_1_5_2; }
//","
public Keyword getCommaKeyword_2_1_5_2_0() { return cCommaKeyword_2_1_5_2_0; }
//extends+=JvmParameterizedTypeReference
public Assignment getExtendsAssignment_2_1_5_2_1() { return cExtendsAssignment_2_1_5_2_1; }
//JvmParameterizedTypeReference
public RuleCall getExtendsJvmParameterizedTypeReferenceParserRuleCall_2_1_5_2_1_0() { return cExtendsJvmParameterizedTypeReferenceParserRuleCall_2_1_5_2_1_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_1_6() { return cLeftCurlyBracketKeyword_2_1_6; }
//members+=Member*
public Assignment getMembersAssignment_2_1_7() { return cMembersAssignment_2_1_7; }
//Member
public RuleCall getMembersMemberParserRuleCall_2_1_7_0() { return cMembersMemberParserRuleCall_2_1_7_0; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_1_8() { return cRightCurlyBracketKeyword_2_1_8; }
//{xtend::XtendEnum.annotationInfo=current} modifiers+=CommonModifier* "enum" name=ValidID "{" (members+=XtendEnumLiteral
//("," members+=XtendEnumLiteral)*)? ";"? "}"
public Group getGroup_2_2() { return cGroup_2_2; }
//{xtend::XtendEnum.annotationInfo=current}
public Action getXtendEnumAnnotationInfoAction_2_2_0() { return cXtendEnumAnnotationInfoAction_2_2_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_2_1() { return cModifiersAssignment_2_2_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_2_1_0() { return cModifiersCommonModifierParserRuleCall_2_2_1_0; }
//"enum"
public Keyword getEnumKeyword_2_2_2() { return cEnumKeyword_2_2_2; }
//name=ValidID
public Assignment getNameAssignment_2_2_3() { return cNameAssignment_2_2_3; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_2_3_0() { return cNameValidIDParserRuleCall_2_2_3_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_2_4() { return cLeftCurlyBracketKeyword_2_2_4; }
//(members+=XtendEnumLiteral ("," members+=XtendEnumLiteral)*)?
public Group getGroup_2_2_5() { return cGroup_2_2_5; }
//members+=XtendEnumLiteral
public Assignment getMembersAssignment_2_2_5_0() { return cMembersAssignment_2_2_5_0; }
//XtendEnumLiteral
public RuleCall getMembersXtendEnumLiteralParserRuleCall_2_2_5_0_0() { return cMembersXtendEnumLiteralParserRuleCall_2_2_5_0_0; }
//("," members+=XtendEnumLiteral)*
public Group getGroup_2_2_5_1() { return cGroup_2_2_5_1; }
//","
public Keyword getCommaKeyword_2_2_5_1_0() { return cCommaKeyword_2_2_5_1_0; }
//members+=XtendEnumLiteral
public Assignment getMembersAssignment_2_2_5_1_1() { return cMembersAssignment_2_2_5_1_1; }
//XtendEnumLiteral
public RuleCall getMembersXtendEnumLiteralParserRuleCall_2_2_5_1_1_0() { return cMembersXtendEnumLiteralParserRuleCall_2_2_5_1_1_0; }
//";"?
public Keyword getSemicolonKeyword_2_2_6() { return cSemicolonKeyword_2_2_6; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_2_7() { return cRightCurlyBracketKeyword_2_2_7; }
//{xtend::XtendAnnotationType.annotationInfo=current} modifiers+=CommonModifier* "annotation" name=ValidID "{"
//members+=AnnotationField* "}"
public Group getGroup_2_3() { return cGroup_2_3; }
//{xtend::XtendAnnotationType.annotationInfo=current}
public Action getXtendAnnotationTypeAnnotationInfoAction_2_3_0() { return cXtendAnnotationTypeAnnotationInfoAction_2_3_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_3_1() { return cModifiersAssignment_2_3_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_3_1_0() { return cModifiersCommonModifierParserRuleCall_2_3_1_0; }
//"annotation"
public Keyword getAnnotationKeyword_2_3_2() { return cAnnotationKeyword_2_3_2; }
//name=ValidID
public Assignment getNameAssignment_2_3_3() { return cNameAssignment_2_3_3; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_3_3_0() { return cNameValidIDParserRuleCall_2_3_3_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_3_4() { return cLeftCurlyBracketKeyword_2_3_4; }
//members+=AnnotationField*
public Assignment getMembersAssignment_2_3_5() { return cMembersAssignment_2_3_5; }
//AnnotationField
public RuleCall getMembersAnnotationFieldParserRuleCall_2_3_5_0() { return cMembersAnnotationFieldParserRuleCall_2_3_5_0; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_3_6() { return cRightCurlyBracketKeyword_2_3_6; }
//{ExampleGroup.annotationInfo=current} preamble="describe" targetType=JvmTypeReference? name=STRING? "{" members+=Member*
//"}"
public Group getGroup_2_4() { return cGroup_2_4; }
//{ExampleGroup.annotationInfo=current}
public Action getExampleGroupAnnotationInfoAction_2_4_0() { return cExampleGroupAnnotationInfoAction_2_4_0; }
//preamble="describe"
public Assignment getPreambleAssignment_2_4_1() { return cPreambleAssignment_2_4_1; }
//"describe"
public Keyword getPreambleDescribeKeyword_2_4_1_0() { return cPreambleDescribeKeyword_2_4_1_0; }
//targetType=JvmTypeReference?
public Assignment getTargetTypeAssignment_2_4_2() { return cTargetTypeAssignment_2_4_2; }
//JvmTypeReference
public RuleCall getTargetTypeJvmTypeReferenceParserRuleCall_2_4_2_0() { return cTargetTypeJvmTypeReferenceParserRuleCall_2_4_2_0; }
//name=STRING?
public Assignment getNameAssignment_2_4_3() { return cNameAssignment_2_4_3; }
//STRING
public RuleCall getNameSTRINGTerminalRuleCall_2_4_3_0() { return cNameSTRINGTerminalRuleCall_2_4_3_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_4_4() { return cLeftCurlyBracketKeyword_2_4_4; }
//members+=Member*
public Assignment getMembersAssignment_2_4_5() { return cMembersAssignment_2_4_5; }
//Member
public RuleCall getMembersMemberParserRuleCall_2_4_5_0() { return cMembersMemberParserRuleCall_2_4_5_0; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_4_6() { return cRightCurlyBracketKeyword_2_4_6; }
}
public class MethodElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Method");
private final Group cGroup = (Group)rule.eContents().get(1);
private final RuleCall cIDTerminalRuleCall_0 = (RuleCall)cGroup.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Group cGroup_1_1 = (Group)cGroup_1.eContents().get(1);
private final RuleCall cTypeNameParserRuleCall_1_1_0 = (RuleCall)cGroup_1_1.eContents().get(0);
private final Group cGroup_1_1_1 = (Group)cGroup_1_1.eContents().get(1);
private final Keyword cCommaKeyword_1_1_1_0 = (Keyword)cGroup_1_1_1.eContents().get(0);
private final RuleCall cTypeNameParserRuleCall_1_1_1_1 = (RuleCall)cGroup_1_1_1.eContents().get(1);
private final Keyword cRightParenthesisKeyword_1_2 = (Keyword)cGroup_1.eContents().get(2);
//Method:
// ID ("(" (TypeName ("," TypeName)*)? ")")?;
public ParserRule getRule() { return rule; }
//ID ("(" (TypeName ("," TypeName)*)? ")")?
public Group getGroup() { return cGroup; }
//ID
public RuleCall getIDTerminalRuleCall_0() { return cIDTerminalRuleCall_0; }
//("(" (TypeName ("," TypeName)*)? ")")?
public Group getGroup_1() { return cGroup_1; }
//"("
public Keyword getLeftParenthesisKeyword_1_0() { return cLeftParenthesisKeyword_1_0; }
//(TypeName ("," TypeName)*)?
public Group getGroup_1_1() { return cGroup_1_1; }
//TypeName
public RuleCall getTypeNameParserRuleCall_1_1_0() { return cTypeNameParserRuleCall_1_1_0; }
//("," TypeName)*
public Group getGroup_1_1_1() { return cGroup_1_1_1; }
//","
public Keyword getCommaKeyword_1_1_1_0() { return cCommaKeyword_1_1_1_0; }
//TypeName
public RuleCall getTypeNameParserRuleCall_1_1_1_1() { return cTypeNameParserRuleCall_1_1_1_1; }
//")"
public Keyword getRightParenthesisKeyword_1_2() { return cRightParenthesisKeyword_1_2; }
}
public class TypeNameElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "TypeName");
private final Group cGroup = (Group)rule.eContents().get(1);
private final RuleCall cIDTerminalRuleCall_0 = (RuleCall)cGroup.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cLessThanSignKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final RuleCall cTypeRefParserRuleCall_1_1 = (RuleCall)cGroup_1.eContents().get(1);
private final Group cGroup_1_2 = (Group)cGroup_1.eContents().get(2);
private final Keyword cCommaKeyword_1_2_0 = (Keyword)cGroup_1_2.eContents().get(0);
private final RuleCall cTypeRefParserRuleCall_1_2_1 = (RuleCall)cGroup_1_2.eContents().get(1);
private final Keyword cGreaterThanSignKeyword_1_3 = (Keyword)cGroup_1.eContents().get(3);
private final Group cGroup_2 = (Group)cGroup.eContents().get(2);
private final Keyword cLeftSquareBracketKeyword_2_0 = (Keyword)cGroup_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_2_1 = (Keyword)cGroup_2.eContents().get(1);
//TypeName:
// ID ("<" TypeRef ("," TypeRef)* ">")? ("[" "]")?;
public ParserRule getRule() { return rule; }
//ID ("<" TypeRef ("," TypeRef)* ">")? ("[" "]")?
public Group getGroup() { return cGroup; }
//ID
public RuleCall getIDTerminalRuleCall_0() { return cIDTerminalRuleCall_0; }
//("<" TypeRef ("," TypeRef)* ">")?
public Group getGroup_1() { return cGroup_1; }
//"<"
public Keyword getLessThanSignKeyword_1_0() { return cLessThanSignKeyword_1_0; }
//TypeRef
public RuleCall getTypeRefParserRuleCall_1_1() { return cTypeRefParserRuleCall_1_1; }
//("," TypeRef)*
public Group getGroup_1_2() { return cGroup_1_2; }
//","
public Keyword getCommaKeyword_1_2_0() { return cCommaKeyword_1_2_0; }
//TypeRef
public RuleCall getTypeRefParserRuleCall_1_2_1() { return cTypeRefParserRuleCall_1_2_1; }
//">"
public Keyword getGreaterThanSignKeyword_1_3() { return cGreaterThanSignKeyword_1_3; }
//("[" "]")?
public Group getGroup_2() { return cGroup_2; }
//"["
public Keyword getLeftSquareBracketKeyword_2_0() { return cLeftSquareBracketKeyword_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_2_1() { return cRightSquareBracketKeyword_2_1; }
}
public class TypeRefElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "TypeRef");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cQuestionMarkKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Alternatives cAlternatives_1 = (Alternatives)cGroup.eContents().get(1);
private final Keyword cExtendsKeyword_1_0 = (Keyword)cAlternatives_1.eContents().get(0);
private final Keyword cSuperKeyword_1_1 = (Keyword)cAlternatives_1.eContents().get(1);
private final RuleCall cTypeNameParserRuleCall_2 = (RuleCall)cGroup.eContents().get(2);
//TypeRef:
// "?"? ("extends" | "super")? TypeName;
public ParserRule getRule() { return rule; }
//"?"? ("extends" | "super")? TypeName
public Group getGroup() { return cGroup; }
//"?"?
public Keyword getQuestionMarkKeyword_0() { return cQuestionMarkKeyword_0; }
//("extends" | "super")?
public Alternatives getAlternatives_1() { return cAlternatives_1; }
//"extends"
public Keyword getExtendsKeyword_1_0() { return cExtendsKeyword_1_0; }
//"super"
public Keyword getSuperKeyword_1_1() { return cSuperKeyword_1_1; }
//TypeName
public RuleCall getTypeNameParserRuleCall_2() { return cTypeNameParserRuleCall_2; }
}
public class MemberElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Member");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Action cXtendMemberAction_0 = (Action)cGroup.eContents().get(0);
private final Assignment cAnnotationsAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cAnnotationsXAnnotationParserRuleCall_1_0 = (RuleCall)cAnnotationsAssignment_1.eContents().get(0);
private final Alternatives cAlternatives_2 = (Alternatives)cGroup.eContents().get(2);
private final Group cGroup_2_0 = (Group)cAlternatives_2.eContents().get(0);
private final Action cExampleAnnotationInfoAction_2_0_0 = (Action)cGroup_2_0.eContents().get(0);
private final Group cGroup_2_0_1 = (Group)cGroup_2_0.eContents().get(1);
private final Assignment cPendingAssignment_2_0_1_0 = (Assignment)cGroup_2_0_1.eContents().get(0);
private final Keyword cPendingPendingKeyword_2_0_1_0_0 = (Keyword)cPendingAssignment_2_0_1_0.eContents().get(0);
private final Alternatives cAlternatives_2_0_1_1 = (Alternatives)cGroup_2_0_1.eContents().get(1);
private final Keyword cFactKeyword_2_0_1_1_0 = (Keyword)cAlternatives_2_0_1_1.eContents().get(0);
private final Keyword cFactsKeyword_2_0_1_1_1 = (Keyword)cAlternatives_2_0_1_1.eContents().get(1);
private final Assignment cExprAssignment_2_0_1_2 = (Assignment)cGroup_2_0_1.eContents().get(2);
private final RuleCall cExprXExpressionParserRuleCall_2_0_1_2_0 = (RuleCall)cExprAssignment_2_0_1_2.eContents().get(0);
private final Assignment cExpressionAssignment_2_0_1_3 = (Assignment)cGroup_2_0_1.eContents().get(3);
private final RuleCall cExpressionXBlockExpressionParserRuleCall_2_0_1_3_0 = (RuleCall)cExpressionAssignment_2_0_1_3.eContents().get(0);
private final Group cGroup_2_1 = (Group)cAlternatives_2.eContents().get(1);
private final Action cExampleGroupAnnotationInfoAction_2_1_0 = (Action)cGroup_2_1.eContents().get(0);
private final Group cGroup_2_1_1 = (Group)cGroup_2_1.eContents().get(1);
private final Assignment cPreambleAssignment_2_1_1_0 = (Assignment)cGroup_2_1_1.eContents().get(0);
private final Keyword cPreambleDescribeKeyword_2_1_1_0_0 = (Keyword)cPreambleAssignment_2_1_1_0.eContents().get(0);
private final Assignment cTargetTypeAssignment_2_1_1_1 = (Assignment)cGroup_2_1_1.eContents().get(1);
private final RuleCall cTargetTypeJvmTypeReferenceParserRuleCall_2_1_1_1_0 = (RuleCall)cTargetTypeAssignment_2_1_1_1.eContents().get(0);
private final Assignment cNameAssignment_2_1_1_2 = (Assignment)cGroup_2_1_1.eContents().get(2);
private final RuleCall cNameSTRINGTerminalRuleCall_2_1_1_2_0 = (RuleCall)cNameAssignment_2_1_1_2.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_1_1_3 = (Keyword)cGroup_2_1_1.eContents().get(3);
private final Assignment cMembersAssignment_2_1_1_4 = (Assignment)cGroup_2_1_1.eContents().get(4);
private final RuleCall cMembersMemberParserRuleCall_2_1_1_4_0 = (RuleCall)cMembersAssignment_2_1_1_4.eContents().get(0);
private final Keyword cRightCurlyBracketKeyword_2_1_1_5 = (Keyword)cGroup_2_1_1.eContents().get(5);
private final Group cGroup_2_2 = (Group)cAlternatives_2.eContents().get(2);
private final Action cBeforeAnnotationInfoAction_2_2_0 = (Action)cGroup_2_2.eContents().get(0);
private final Group cGroup_2_2_1 = (Group)cGroup_2_2.eContents().get(1);
private final Keyword cBeforeKeyword_2_2_1_0 = (Keyword)cGroup_2_2_1.eContents().get(0);
private final Alternatives cAlternatives_2_2_1_1 = (Alternatives)cGroup_2_2_1.eContents().get(1);
private final Assignment cModifiersAssignment_2_2_1_1_0 = (Assignment)cAlternatives_2_2_1_1.eContents().get(0);
private final Keyword cModifiersAllKeyword_2_2_1_1_0_0 = (Keyword)cModifiersAssignment_2_2_1_1_0.eContents().get(0);
private final Keyword cEachKeyword_2_2_1_1_1 = (Keyword)cAlternatives_2_2_1_1.eContents().get(1);
private final Assignment cNameAssignment_2_2_1_2 = (Assignment)cGroup_2_2_1.eContents().get(2);
private final RuleCall cNameSTRINGTerminalRuleCall_2_2_1_2_0 = (RuleCall)cNameAssignment_2_2_1_2.eContents().get(0);
private final Assignment cExpressionAssignment_2_2_1_3 = (Assignment)cGroup_2_2_1.eContents().get(3);
private final RuleCall cExpressionXExpressionParserRuleCall_2_2_1_3_0 = (RuleCall)cExpressionAssignment_2_2_1_3.eContents().get(0);
private final Group cGroup_2_3 = (Group)cAlternatives_2.eContents().get(3);
private final Action cAfterAnnotationInfoAction_2_3_0 = (Action)cGroup_2_3.eContents().get(0);
private final Group cGroup_2_3_1 = (Group)cGroup_2_3.eContents().get(1);
private final Keyword cAfterKeyword_2_3_1_0 = (Keyword)cGroup_2_3_1.eContents().get(0);
private final Alternatives cAlternatives_2_3_1_1 = (Alternatives)cGroup_2_3_1.eContents().get(1);
private final Assignment cModifiersAssignment_2_3_1_1_0 = (Assignment)cAlternatives_2_3_1_1.eContents().get(0);
private final Keyword cModifiersAllKeyword_2_3_1_1_0_0 = (Keyword)cModifiersAssignment_2_3_1_1_0.eContents().get(0);
private final Keyword cEachKeyword_2_3_1_1_1 = (Keyword)cAlternatives_2_3_1_1.eContents().get(1);
private final Assignment cNameAssignment_2_3_1_2 = (Assignment)cGroup_2_3_1.eContents().get(2);
private final RuleCall cNameSTRINGTerminalRuleCall_2_3_1_2_0 = (RuleCall)cNameAssignment_2_3_1_2.eContents().get(0);
private final Assignment cExpressionAssignment_2_3_1_3 = (Assignment)cGroup_2_3_1.eContents().get(3);
private final RuleCall cExpressionXExpressionParserRuleCall_2_3_1_3_0 = (RuleCall)cExpressionAssignment_2_3_1_3.eContents().get(0);
private final Group cGroup_2_4 = (Group)cAlternatives_2.eContents().get(4);
private final Action cExampleGroupAnnotationInfoAction_2_4_0 = (Action)cGroup_2_4.eContents().get(0);
private final Group cGroup_2_4_1 = (Group)cGroup_2_4.eContents().get(1);
private final Assignment cPreambleAssignment_2_4_1_0 = (Assignment)cGroup_2_4_1.eContents().get(0);
private final Keyword cPreambleContextKeyword_2_4_1_0_0 = (Keyword)cPreambleAssignment_2_4_1_0.eContents().get(0);
private final Assignment cTargetOperationAssignment_2_4_1_1 = (Assignment)cGroup_2_4_1.eContents().get(1);
private final CrossReference cTargetOperationJvmOperationCrossReference_2_4_1_1_0 = (CrossReference)cTargetOperationAssignment_2_4_1_1.eContents().get(0);
private final RuleCall cTargetOperationJvmOperationMethodParserRuleCall_2_4_1_1_0_1 = (RuleCall)cTargetOperationJvmOperationCrossReference_2_4_1_1_0.eContents().get(1);
private final Assignment cNameAssignment_2_4_1_2 = (Assignment)cGroup_2_4_1.eContents().get(2);
private final RuleCall cNameSTRINGTerminalRuleCall_2_4_1_2_0 = (RuleCall)cNameAssignment_2_4_1_2.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_4_1_3 = (Keyword)cGroup_2_4_1.eContents().get(3);
private final Assignment cMembersAssignment_2_4_1_4 = (Assignment)cGroup_2_4_1.eContents().get(4);
private final RuleCall cMembersMemberParserRuleCall_2_4_1_4_0 = (RuleCall)cMembersAssignment_2_4_1_4.eContents().get(0);
private final Keyword cRightCurlyBracketKeyword_2_4_1_5 = (Keyword)cGroup_2_4_1.eContents().get(5);
private final Group cGroup_2_5 = (Group)cAlternatives_2.eContents().get(5);
private final Action cExampleTableAnnotationInfoAction_2_5_0 = (Action)cGroup_2_5.eContents().get(0);
private final Group cGroup_2_5_1 = (Group)cGroup_2_5.eContents().get(1);
private final Keyword cDefKeyword_2_5_1_0 = (Keyword)cGroup_2_5_1.eContents().get(0);
private final Assignment cNameAssignment_2_5_1_1 = (Assignment)cGroup_2_5_1.eContents().get(1);
private final RuleCall cNameIDTerminalRuleCall_2_5_1_1_0 = (RuleCall)cNameAssignment_2_5_1_1.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_2_5_1_2 = (Keyword)cGroup_2_5_1.eContents().get(2);
private final Group cGroup_2_5_1_3 = (Group)cGroup_2_5_1.eContents().get(3);
private final Keyword cVerticalLineKeyword_2_5_1_3_0 = (Keyword)cGroup_2_5_1_3.eContents().get(0);
private final Assignment cColumnsAssignment_2_5_1_3_1 = (Assignment)cGroup_2_5_1_3.eContents().get(1);
private final RuleCall cColumnsExampleColumnParserRuleCall_2_5_1_3_1_0 = (RuleCall)cColumnsAssignment_2_5_1_3_1.eContents().get(0);
private final Assignment cRowsAssignment_2_5_1_3_2 = (Assignment)cGroup_2_5_1_3.eContents().get(2);
private final RuleCall cRowsExampleRowParserRuleCall_2_5_1_3_2_0 = (RuleCall)cRowsAssignment_2_5_1_3_2.eContents().get(0);
private final Keyword cRightCurlyBracketKeyword_2_5_1_4 = (Keyword)cGroup_2_5_1.eContents().get(4);
private final Group cGroup_2_6 = (Group)cAlternatives_2.eContents().get(6);
private final Action cXtendFieldAnnotationInfoAction_2_6_0 = (Action)cGroup_2_6.eContents().get(0);
private final Assignment cModifiersAssignment_2_6_1 = (Assignment)cGroup_2_6.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_6_1_0 = (RuleCall)cModifiersAssignment_2_6_1.eContents().get(0);
private final Alternatives cAlternatives_2_6_2 = (Alternatives)cGroup_2_6.eContents().get(2);
private final Group cGroup_2_6_2_0 = (Group)cAlternatives_2_6_2.eContents().get(0);
private final Assignment cModifiersAssignment_2_6_2_0_0 = (Assignment)cGroup_2_6_2_0.eContents().get(0);
private final RuleCall cModifiersFieldModifierParserRuleCall_2_6_2_0_0_0 = (RuleCall)cModifiersAssignment_2_6_2_0_0.eContents().get(0);
private final Assignment cModifiersAssignment_2_6_2_0_1 = (Assignment)cGroup_2_6_2_0.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_6_2_0_1_0 = (RuleCall)cModifiersAssignment_2_6_2_0_1.eContents().get(0);
private final Assignment cTypeAssignment_2_6_2_0_2 = (Assignment)cGroup_2_6_2_0.eContents().get(2);
private final RuleCall cTypeJvmTypeReferenceParserRuleCall_2_6_2_0_2_0 = (RuleCall)cTypeAssignment_2_6_2_0_2.eContents().get(0);
private final Assignment cNameAssignment_2_6_2_0_3 = (Assignment)cGroup_2_6_2_0.eContents().get(3);
private final RuleCall cNameValidIDParserRuleCall_2_6_2_0_3_0 = (RuleCall)cNameAssignment_2_6_2_0_3.eContents().get(0);
private final Group cGroup_2_6_2_1 = (Group)cAlternatives_2_6_2.eContents().get(1);
private final Assignment cModifiersAssignment_2_6_2_1_0 = (Assignment)cGroup_2_6_2_1.eContents().get(0);
private final Keyword cModifiersExtensionKeyword_2_6_2_1_0_0 = (Keyword)cModifiersAssignment_2_6_2_1_0.eContents().get(0);
private final Alternatives cAlternatives_2_6_2_1_1 = (Alternatives)cGroup_2_6_2_1.eContents().get(1);
private final Assignment cModifiersAssignment_2_6_2_1_1_0 = (Assignment)cAlternatives_2_6_2_1_1.eContents().get(0);
private final RuleCall cModifiersFieldModifierParserRuleCall_2_6_2_1_1_0_0 = (RuleCall)cModifiersAssignment_2_6_2_1_1_0.eContents().get(0);
private final Assignment cModifiersAssignment_2_6_2_1_1_1 = (Assignment)cAlternatives_2_6_2_1_1.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_6_2_1_1_1_0 = (RuleCall)cModifiersAssignment_2_6_2_1_1_1.eContents().get(0);
private final Assignment cTypeAssignment_2_6_2_1_2 = (Assignment)cGroup_2_6_2_1.eContents().get(2);
private final RuleCall cTypeJvmTypeReferenceParserRuleCall_2_6_2_1_2_0 = (RuleCall)cTypeAssignment_2_6_2_1_2.eContents().get(0);
private final Assignment cNameAssignment_2_6_2_1_3 = (Assignment)cGroup_2_6_2_1.eContents().get(3);
private final RuleCall cNameValidIDParserRuleCall_2_6_2_1_3_0 = (RuleCall)cNameAssignment_2_6_2_1_3.eContents().get(0);
private final Group cGroup_2_6_2_2 = (Group)cAlternatives_2_6_2.eContents().get(2);
private final Assignment cModifiersAssignment_2_6_2_2_0 = (Assignment)cGroup_2_6_2_2.eContents().get(0);
private final RuleCall cModifiersFieldModifierParserRuleCall_2_6_2_2_0_0 = (RuleCall)cModifiersAssignment_2_6_2_2_0.eContents().get(0);
private final Assignment cModifiersAssignment_2_6_2_2_1 = (Assignment)cGroup_2_6_2_2.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_6_2_2_1_0 = (RuleCall)cModifiersAssignment_2_6_2_2_1.eContents().get(0);
private final Assignment cModifiersAssignment_2_6_2_2_2 = (Assignment)cGroup_2_6_2_2.eContents().get(2);
private final Keyword cModifiersExtensionKeyword_2_6_2_2_2_0 = (Keyword)cModifiersAssignment_2_6_2_2_2.eContents().get(0);
private final Assignment cModifiersAssignment_2_6_2_2_3 = (Assignment)cGroup_2_6_2_2.eContents().get(3);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_6_2_2_3_0 = (RuleCall)cModifiersAssignment_2_6_2_2_3.eContents().get(0);
private final Assignment cTypeAssignment_2_6_2_2_4 = (Assignment)cGroup_2_6_2_2.eContents().get(4);
private final RuleCall cTypeJvmTypeReferenceParserRuleCall_2_6_2_2_4_0 = (RuleCall)cTypeAssignment_2_6_2_2_4.eContents().get(0);
private final Assignment cNameAssignment_2_6_2_2_5 = (Assignment)cGroup_2_6_2_2.eContents().get(5);
private final RuleCall cNameValidIDParserRuleCall_2_6_2_2_5_0 = (RuleCall)cNameAssignment_2_6_2_2_5.eContents().get(0);
private final Group cGroup_2_6_2_3 = (Group)cAlternatives_2_6_2.eContents().get(3);
private final Assignment cTypeAssignment_2_6_2_3_0 = (Assignment)cGroup_2_6_2_3.eContents().get(0);
private final RuleCall cTypeJvmTypeReferenceParserRuleCall_2_6_2_3_0_0 = (RuleCall)cTypeAssignment_2_6_2_3_0.eContents().get(0);
private final Assignment cNameAssignment_2_6_2_3_1 = (Assignment)cGroup_2_6_2_3.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_2_6_2_3_1_0 = (RuleCall)cNameAssignment_2_6_2_3_1.eContents().get(0);
private final Group cGroup_2_6_3 = (Group)cGroup_2_6.eContents().get(3);
private final Keyword cEqualsSignKeyword_2_6_3_0 = (Keyword)cGroup_2_6_3.eContents().get(0);
private final Assignment cInitialValueAssignment_2_6_3_1 = (Assignment)cGroup_2_6_3.eContents().get(1);
private final RuleCall cInitialValueXExpressionParserRuleCall_2_6_3_1_0 = (RuleCall)cInitialValueAssignment_2_6_3_1.eContents().get(0);
private final Keyword cSemicolonKeyword_2_6_4 = (Keyword)cGroup_2_6.eContents().get(4);
private final Group cGroup_2_7 = (Group)cAlternatives_2.eContents().get(7);
private final Action cXtendFunctionAnnotationInfoAction_2_7_0 = (Action)cGroup_2_7.eContents().get(0);
private final Assignment cModifiersAssignment_2_7_1 = (Assignment)cGroup_2_7.eContents().get(1);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_7_1_0 = (RuleCall)cModifiersAssignment_2_7_1.eContents().get(0);
private final Assignment cModifiersAssignment_2_7_2 = (Assignment)cGroup_2_7.eContents().get(2);
private final RuleCall cModifiersMethodModifierParserRuleCall_2_7_2_0 = (RuleCall)cModifiersAssignment_2_7_2.eContents().get(0);
private final Alternatives cAlternatives_2_7_3 = (Alternatives)cGroup_2_7.eContents().get(3);
private final Assignment cModifiersAssignment_2_7_3_0 = (Assignment)cAlternatives_2_7_3.eContents().get(0);
private final RuleCall cModifiersCommonModifierParserRuleCall_2_7_3_0_0 = (RuleCall)cModifiersAssignment_2_7_3_0.eContents().get(0);
private final Assignment cModifiersAssignment_2_7_3_1 = (Assignment)cAlternatives_2_7_3.eContents().get(1);
private final RuleCall cModifiersMethodModifierParserRuleCall_2_7_3_1_0 = (RuleCall)cModifiersAssignment_2_7_3_1.eContents().get(0);
private final Group cGroup_2_7_4 = (Group)cGroup_2_7.eContents().get(4);
private final Keyword cLessThanSignKeyword_2_7_4_0 = (Keyword)cGroup_2_7_4.eContents().get(0);
private final Assignment cTypeParametersAssignment_2_7_4_1 = (Assignment)cGroup_2_7_4.eContents().get(1);
private final RuleCall cTypeParametersJvmTypeParameterParserRuleCall_2_7_4_1_0 = (RuleCall)cTypeParametersAssignment_2_7_4_1.eContents().get(0);
private final Group cGroup_2_7_4_2 = (Group)cGroup_2_7_4.eContents().get(2);
private final Keyword cCommaKeyword_2_7_4_2_0 = (Keyword)cGroup_2_7_4_2.eContents().get(0);
private final Assignment cTypeParametersAssignment_2_7_4_2_1 = (Assignment)cGroup_2_7_4_2.eContents().get(1);
private final RuleCall cTypeParametersJvmTypeParameterParserRuleCall_2_7_4_2_1_0 = (RuleCall)cTypeParametersAssignment_2_7_4_2_1.eContents().get(0);
private final Keyword cGreaterThanSignKeyword_2_7_4_3 = (Keyword)cGroup_2_7_4.eContents().get(3);
private final Alternatives cAlternatives_2_7_5 = (Alternatives)cGroup_2_7.eContents().get(5);
private final Group cGroup_2_7_5_0 = (Group)cAlternatives_2_7_5.eContents().get(0);
private final Group cGroup_2_7_5_0_0 = (Group)cGroup_2_7_5_0.eContents().get(0);
private final Assignment cReturnTypeAssignment_2_7_5_0_0_0 = (Assignment)cGroup_2_7_5_0_0.eContents().get(0);
private final RuleCall cReturnTypeJvmTypeReferenceParserRuleCall_2_7_5_0_0_0_0 = (RuleCall)cReturnTypeAssignment_2_7_5_0_0_0.eContents().get(0);
private final Assignment cCreateExtensionInfoAssignment_2_7_5_0_0_1 = (Assignment)cGroup_2_7_5_0_0.eContents().get(1);
private final RuleCall cCreateExtensionInfoCreateExtensionInfoParserRuleCall_2_7_5_0_0_1_0 = (RuleCall)cCreateExtensionInfoAssignment_2_7_5_0_0_1.eContents().get(0);
private final Assignment cNameAssignment_2_7_5_0_0_2 = (Assignment)cGroup_2_7_5_0_0.eContents().get(2);
private final RuleCall cNameValidIDParserRuleCall_2_7_5_0_0_2_0 = (RuleCall)cNameAssignment_2_7_5_0_0_2.eContents().get(0);
private final Keyword cLeftParenthesisKeyword_2_7_5_0_0_3 = (Keyword)cGroup_2_7_5_0_0.eContents().get(3);
private final Group cGroup_2_7_5_1 = (Group)cAlternatives_2_7_5.eContents().get(1);
private final Group cGroup_2_7_5_1_0 = (Group)cGroup_2_7_5_1.eContents().get(0);
private final Assignment cReturnTypeAssignment_2_7_5_1_0_0 = (Assignment)cGroup_2_7_5_1_0.eContents().get(0);
private final RuleCall cReturnTypeJvmTypeReferenceParserRuleCall_2_7_5_1_0_0_0 = (RuleCall)cReturnTypeAssignment_2_7_5_1_0_0.eContents().get(0);
private final Assignment cNameAssignment_2_7_5_1_0_1 = (Assignment)cGroup_2_7_5_1_0.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_2_7_5_1_0_1_0 = (RuleCall)cNameAssignment_2_7_5_1_0_1.eContents().get(0);
private final Keyword cLeftParenthesisKeyword_2_7_5_1_0_2 = (Keyword)cGroup_2_7_5_1_0.eContents().get(2);
private final Group cGroup_2_7_5_2 = (Group)cAlternatives_2_7_5.eContents().get(2);
private final Group cGroup_2_7_5_2_0 = (Group)cGroup_2_7_5_2.eContents().get(0);
private final Assignment cCreateExtensionInfoAssignment_2_7_5_2_0_0 = (Assignment)cGroup_2_7_5_2_0.eContents().get(0);
private final RuleCall cCreateExtensionInfoCreateExtensionInfoParserRuleCall_2_7_5_2_0_0_0 = (RuleCall)cCreateExtensionInfoAssignment_2_7_5_2_0_0.eContents().get(0);
private final Assignment cNameAssignment_2_7_5_2_0_1 = (Assignment)cGroup_2_7_5_2_0.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_2_7_5_2_0_1_0 = (RuleCall)cNameAssignment_2_7_5_2_0_1.eContents().get(0);
private final Keyword cLeftParenthesisKeyword_2_7_5_2_0_2 = (Keyword)cGroup_2_7_5_2_0.eContents().get(2);
private final Group cGroup_2_7_5_3 = (Group)cAlternatives_2_7_5.eContents().get(3);
private final Assignment cNameAssignment_2_7_5_3_0 = (Assignment)cGroup_2_7_5_3.eContents().get(0);
private final RuleCall cNameValidIDParserRuleCall_2_7_5_3_0_0 = (RuleCall)cNameAssignment_2_7_5_3_0.eContents().get(0);
private final Keyword cLeftParenthesisKeyword_2_7_5_3_1 = (Keyword)cGroup_2_7_5_3.eContents().get(1);
private final Group cGroup_2_7_6 = (Group)cGroup_2_7.eContents().get(6);
private final Assignment cParametersAssignment_2_7_6_0 = (Assignment)cGroup_2_7_6.eContents().get(0);
private final RuleCall cParametersParameterParserRuleCall_2_7_6_0_0 = (RuleCall)cParametersAssignment_2_7_6_0.eContents().get(0);
private final Group cGroup_2_7_6_1 = (Group)cGroup_2_7_6.eContents().get(1);
private final Keyword cCommaKeyword_2_7_6_1_0 = (Keyword)cGroup_2_7_6_1.eContents().get(0);
private final Assignment cParametersAssignment_2_7_6_1_1 = (Assignment)cGroup_2_7_6_1.eContents().get(1);
private final RuleCall cParametersParameterParserRuleCall_2_7_6_1_1_0 = (RuleCall)cParametersAssignment_2_7_6_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_2_7_7 = (Keyword)cGroup_2_7.eContents().get(7);
private final Group cGroup_2_7_8 = (Group)cGroup_2_7.eContents().get(8);
private final Keyword cThrowsKeyword_2_7_8_0 = (Keyword)cGroup_2_7_8.eContents().get(0);
private final Assignment cExceptionsAssignment_2_7_8_1 = (Assignment)cGroup_2_7_8.eContents().get(1);
private final RuleCall cExceptionsJvmTypeReferenceParserRuleCall_2_7_8_1_0 = (RuleCall)cExceptionsAssignment_2_7_8_1.eContents().get(0);
private final Group cGroup_2_7_8_2 = (Group)cGroup_2_7_8.eContents().get(2);
private final Keyword cCommaKeyword_2_7_8_2_0 = (Keyword)cGroup_2_7_8_2.eContents().get(0);
private final Assignment cExceptionsAssignment_2_7_8_2_1 = (Assignment)cGroup_2_7_8_2.eContents().get(1);
private final RuleCall cExceptionsJvmTypeReferenceParserRuleCall_2_7_8_2_1_0 = (RuleCall)cExceptionsAssignment_2_7_8_2_1.eContents().get(0);
private final Alternatives cAlternatives_2_7_9 = (Alternatives)cGroup_2_7.eContents().get(9);
private final Assignment cExpressionAssignment_2_7_9_0 = (Assignment)cAlternatives_2_7_9.eContents().get(0);
private final RuleCall cExpressionXBlockExpressionParserRuleCall_2_7_9_0_0 = (RuleCall)cExpressionAssignment_2_7_9_0.eContents().get(0);
private final Assignment cExpressionAssignment_2_7_9_1 = (Assignment)cAlternatives_2_7_9.eContents().get(1);
private final RuleCall cExpressionRichStringParserRuleCall_2_7_9_1_0 = (RuleCall)cExpressionAssignment_2_7_9_1.eContents().get(0);
private final Keyword cSemicolonKeyword_2_7_9_2 = (Keyword)cAlternatives_2_7_9.eContents().get(2);
//Member returns xtend::XtendMember:
// {xtend::XtendMember} annotations+=XAnnotation* ({Example.annotationInfo=current} (pending?="pending"? ("fact" |
// "facts") expr=XExpression expression=XBlockExpression?) | {ExampleGroup.annotationInfo=current} (preamble="describe"
// targetType=JvmTypeReference? name=STRING? "{" members+=Member* "}") | {Before.annotationInfo=current} ("before"
// (modifiers+="all" | "each")? => name=STRING? expression=XExpression) | {After.annotationInfo=current} ("after"
// (modifiers+="all" | "each")? => name=STRING? expression=XExpression) | {ExampleGroup.annotationInfo=current}
// (preamble="context" targetOperation=[types::JvmOperation|Method]? name=STRING? "{" members+=Member* "}") |
// {ExampleTable.annotationInfo=current} ("def" name=ID? "{" ("|" columns+=ExampleColumn* rows+=ExampleRow*)? "}") |
// {xtend::XtendField.annotationInfo=current} modifiers+=CommonModifier* (modifiers+=FieldModifier
// modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID | modifiers+="extension" (modifiers+=FieldModifier |
// modifiers+=CommonModifier)* type=JvmTypeReference name=ValidID? | modifiers+=FieldModifier modifiers+=CommonModifier*
// modifiers+="extension" modifiers+=CommonModifier* type=JvmTypeReference name=ValidID? | type=JvmTypeReference
// name=ValidID) ("=" initialValue=XExpression)? ";"? | {xtend::XtendFunction.annotationInfo=current}
// modifiers+=CommonModifier* modifiers+=MethodModifier (modifiers+=CommonModifier | modifiers+=MethodModifier)* ("<"
// typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? (=> (returnType=JvmTypeReference
// createExtensionInfo=CreateExtensionInfo name=ValidID "(") | => (returnType=JvmTypeReference name=ValidID "(") | =>
// (createExtensionInfo=CreateExtensionInfo name=ValidID "(") | name=ValidID "(") (parameters+=Parameter (","
// parameters+=Parameter)*)? ")" ("throws" exceptions+=JvmTypeReference ("," exceptions+=JvmTypeReference)*)?
// (expression=XBlockExpression | expression=RichString | ";")?);
public ParserRule getRule() { return rule; }
//{xtend::XtendMember} annotations+=XAnnotation* ({Example.annotationInfo=current} (pending?="pending"? ("fact" | "facts")
//expr=XExpression expression=XBlockExpression?) | {ExampleGroup.annotationInfo=current} (preamble="describe"
//targetType=JvmTypeReference? name=STRING? "{" members+=Member* "}") | {Before.annotationInfo=current} ("before"
//(modifiers+="all" | "each")? => name=STRING? expression=XExpression) | {After.annotationInfo=current} ("after"
//(modifiers+="all" | "each")? => name=STRING? expression=XExpression) | {ExampleGroup.annotationInfo=current}
//(preamble="context" targetOperation=[types::JvmOperation|Method]? name=STRING? "{" members+=Member* "}") |
//{ExampleTable.annotationInfo=current} ("def" name=ID? "{" ("|" columns+=ExampleColumn* rows+=ExampleRow*)? "}") |
//{xtend::XtendField.annotationInfo=current} modifiers+=CommonModifier* (modifiers+=FieldModifier
//modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID | modifiers+="extension" (modifiers+=FieldModifier |
//modifiers+=CommonModifier)* type=JvmTypeReference name=ValidID? | modifiers+=FieldModifier modifiers+=CommonModifier*
//modifiers+="extension" modifiers+=CommonModifier* type=JvmTypeReference name=ValidID? | type=JvmTypeReference
//name=ValidID) ("=" initialValue=XExpression)? ";"? | {xtend::XtendFunction.annotationInfo=current}
//modifiers+=CommonModifier* modifiers+=MethodModifier (modifiers+=CommonModifier | modifiers+=MethodModifier)* ("<"
//typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? (=> (returnType=JvmTypeReference
//createExtensionInfo=CreateExtensionInfo name=ValidID "(") | => (returnType=JvmTypeReference name=ValidID "(") | =>
//(createExtensionInfo=CreateExtensionInfo name=ValidID "(") | name=ValidID "(") (parameters+=Parameter (","
//parameters+=Parameter)*)? ")" ("throws" exceptions+=JvmTypeReference ("," exceptions+=JvmTypeReference)*)?
//(expression=XBlockExpression | expression=RichString | ";")?)
public Group getGroup() { return cGroup; }
//{xtend::XtendMember}
public Action getXtendMemberAction_0() { return cXtendMemberAction_0; }
//annotations+=XAnnotation*
public Assignment getAnnotationsAssignment_1() { return cAnnotationsAssignment_1; }
//XAnnotation
public RuleCall getAnnotationsXAnnotationParserRuleCall_1_0() { return cAnnotationsXAnnotationParserRuleCall_1_0; }
//{Example.annotationInfo=current} (pending?="pending"? ("fact" | "facts") expr=XExpression expression=XBlockExpression?)
//| {ExampleGroup.annotationInfo=current} (preamble="describe" targetType=JvmTypeReference? name=STRING? "{"
//members+=Member* "}") | {Before.annotationInfo=current} ("before" (modifiers+="all" | "each")? => name=STRING?
//expression=XExpression) | {After.annotationInfo=current} ("after" (modifiers+="all" | "each")? => name=STRING?
//expression=XExpression) | {ExampleGroup.annotationInfo=current} (preamble="context"
//targetOperation=[types::JvmOperation|Method]? name=STRING? "{" members+=Member* "}") |
//{ExampleTable.annotationInfo=current} ("def" name=ID? "{" ("|" columns+=ExampleColumn* rows+=ExampleRow*)? "}") |
//{xtend::XtendField.annotationInfo=current} modifiers+=CommonModifier* (modifiers+=FieldModifier
//modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID | modifiers+="extension" (modifiers+=FieldModifier |
//modifiers+=CommonModifier)* type=JvmTypeReference name=ValidID? | modifiers+=FieldModifier modifiers+=CommonModifier*
//modifiers+="extension" modifiers+=CommonModifier* type=JvmTypeReference name=ValidID? | type=JvmTypeReference
//name=ValidID) ("=" initialValue=XExpression)? ";"? | {xtend::XtendFunction.annotationInfo=current}
//modifiers+=CommonModifier* modifiers+=MethodModifier (modifiers+=CommonModifier | modifiers+=MethodModifier)* ("<"
//typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? (=> (returnType=JvmTypeReference
//createExtensionInfo=CreateExtensionInfo name=ValidID "(") | => (returnType=JvmTypeReference name=ValidID "(") | =>
//(createExtensionInfo=CreateExtensionInfo name=ValidID "(") | name=ValidID "(") (parameters+=Parameter (","
//parameters+=Parameter)*)? ")" ("throws" exceptions+=JvmTypeReference ("," exceptions+=JvmTypeReference)*)?
//(expression=XBlockExpression | expression=RichString | ";")?
public Alternatives getAlternatives_2() { return cAlternatives_2; }
//{Example.annotationInfo=current} (pending?="pending"? ("fact" | "facts") expr=XExpression expression=XBlockExpression?)
public Group getGroup_2_0() { return cGroup_2_0; }
//{Example.annotationInfo=current}
public Action getExampleAnnotationInfoAction_2_0_0() { return cExampleAnnotationInfoAction_2_0_0; }
//pending?="pending"? ("fact" | "facts") expr=XExpression expression=XBlockExpression?
public Group getGroup_2_0_1() { return cGroup_2_0_1; }
//pending?="pending"?
public Assignment getPendingAssignment_2_0_1_0() { return cPendingAssignment_2_0_1_0; }
//"pending"
public Keyword getPendingPendingKeyword_2_0_1_0_0() { return cPendingPendingKeyword_2_0_1_0_0; }
//"fact" | "facts"
public Alternatives getAlternatives_2_0_1_1() { return cAlternatives_2_0_1_1; }
//"fact"
public Keyword getFactKeyword_2_0_1_1_0() { return cFactKeyword_2_0_1_1_0; }
//"facts"
public Keyword getFactsKeyword_2_0_1_1_1() { return cFactsKeyword_2_0_1_1_1; }
//expr=XExpression
public Assignment getExprAssignment_2_0_1_2() { return cExprAssignment_2_0_1_2; }
//XExpression
public RuleCall getExprXExpressionParserRuleCall_2_0_1_2_0() { return cExprXExpressionParserRuleCall_2_0_1_2_0; }
//expression=XBlockExpression?
public Assignment getExpressionAssignment_2_0_1_3() { return cExpressionAssignment_2_0_1_3; }
//XBlockExpression
public RuleCall getExpressionXBlockExpressionParserRuleCall_2_0_1_3_0() { return cExpressionXBlockExpressionParserRuleCall_2_0_1_3_0; }
//{ExampleGroup.annotationInfo=current} (preamble="describe" targetType=JvmTypeReference? name=STRING? "{"
//members+=Member* "}")
public Group getGroup_2_1() { return cGroup_2_1; }
//{ExampleGroup.annotationInfo=current}
public Action getExampleGroupAnnotationInfoAction_2_1_0() { return cExampleGroupAnnotationInfoAction_2_1_0; }
//preamble="describe" targetType=JvmTypeReference? name=STRING? "{" members+=Member* "}"
public Group getGroup_2_1_1() { return cGroup_2_1_1; }
//preamble="describe"
public Assignment getPreambleAssignment_2_1_1_0() { return cPreambleAssignment_2_1_1_0; }
//"describe"
public Keyword getPreambleDescribeKeyword_2_1_1_0_0() { return cPreambleDescribeKeyword_2_1_1_0_0; }
//targetType=JvmTypeReference?
public Assignment getTargetTypeAssignment_2_1_1_1() { return cTargetTypeAssignment_2_1_1_1; }
//JvmTypeReference
public RuleCall getTargetTypeJvmTypeReferenceParserRuleCall_2_1_1_1_0() { return cTargetTypeJvmTypeReferenceParserRuleCall_2_1_1_1_0; }
//name=STRING?
public Assignment getNameAssignment_2_1_1_2() { return cNameAssignment_2_1_1_2; }
//STRING
public RuleCall getNameSTRINGTerminalRuleCall_2_1_1_2_0() { return cNameSTRINGTerminalRuleCall_2_1_1_2_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_1_1_3() { return cLeftCurlyBracketKeyword_2_1_1_3; }
//members+=Member*
public Assignment getMembersAssignment_2_1_1_4() { return cMembersAssignment_2_1_1_4; }
//Member
public RuleCall getMembersMemberParserRuleCall_2_1_1_4_0() { return cMembersMemberParserRuleCall_2_1_1_4_0; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_1_1_5() { return cRightCurlyBracketKeyword_2_1_1_5; }
//{Before.annotationInfo=current} ("before" (modifiers+="all" | "each")? => name=STRING? expression=XExpression)
public Group getGroup_2_2() { return cGroup_2_2; }
//{Before.annotationInfo=current}
public Action getBeforeAnnotationInfoAction_2_2_0() { return cBeforeAnnotationInfoAction_2_2_0; }
//"before" (modifiers+="all" | "each")? => name=STRING? expression=XExpression
public Group getGroup_2_2_1() { return cGroup_2_2_1; }
//"before"
public Keyword getBeforeKeyword_2_2_1_0() { return cBeforeKeyword_2_2_1_0; }
//(modifiers+="all" | "each")?
public Alternatives getAlternatives_2_2_1_1() { return cAlternatives_2_2_1_1; }
//modifiers+="all"
public Assignment getModifiersAssignment_2_2_1_1_0() { return cModifiersAssignment_2_2_1_1_0; }
//"all"
public Keyword getModifiersAllKeyword_2_2_1_1_0_0() { return cModifiersAllKeyword_2_2_1_1_0_0; }
//"each"
public Keyword getEachKeyword_2_2_1_1_1() { return cEachKeyword_2_2_1_1_1; }
//=> name=STRING?
public Assignment getNameAssignment_2_2_1_2() { return cNameAssignment_2_2_1_2; }
//STRING
public RuleCall getNameSTRINGTerminalRuleCall_2_2_1_2_0() { return cNameSTRINGTerminalRuleCall_2_2_1_2_0; }
//expression=XExpression
public Assignment getExpressionAssignment_2_2_1_3() { return cExpressionAssignment_2_2_1_3; }
//XExpression
public RuleCall getExpressionXExpressionParserRuleCall_2_2_1_3_0() { return cExpressionXExpressionParserRuleCall_2_2_1_3_0; }
//{After.annotationInfo=current} ("after" (modifiers+="all" | "each")? => name=STRING? expression=XExpression)
public Group getGroup_2_3() { return cGroup_2_3; }
//{After.annotationInfo=current}
public Action getAfterAnnotationInfoAction_2_3_0() { return cAfterAnnotationInfoAction_2_3_0; }
//"after" (modifiers+="all" | "each")? => name=STRING? expression=XExpression
public Group getGroup_2_3_1() { return cGroup_2_3_1; }
//"after"
public Keyword getAfterKeyword_2_3_1_0() { return cAfterKeyword_2_3_1_0; }
//(modifiers+="all" | "each")?
public Alternatives getAlternatives_2_3_1_1() { return cAlternatives_2_3_1_1; }
//modifiers+="all"
public Assignment getModifiersAssignment_2_3_1_1_0() { return cModifiersAssignment_2_3_1_1_0; }
//"all"
public Keyword getModifiersAllKeyword_2_3_1_1_0_0() { return cModifiersAllKeyword_2_3_1_1_0_0; }
//"each"
public Keyword getEachKeyword_2_3_1_1_1() { return cEachKeyword_2_3_1_1_1; }
//=> name=STRING?
public Assignment getNameAssignment_2_3_1_2() { return cNameAssignment_2_3_1_2; }
//STRING
public RuleCall getNameSTRINGTerminalRuleCall_2_3_1_2_0() { return cNameSTRINGTerminalRuleCall_2_3_1_2_0; }
//expression=XExpression
public Assignment getExpressionAssignment_2_3_1_3() { return cExpressionAssignment_2_3_1_3; }
//XExpression
public RuleCall getExpressionXExpressionParserRuleCall_2_3_1_3_0() { return cExpressionXExpressionParserRuleCall_2_3_1_3_0; }
//{ExampleGroup.annotationInfo=current} (preamble="context" targetOperation=[types::JvmOperation|Method]? name=STRING? "{"
//members+=Member* "}")
public Group getGroup_2_4() { return cGroup_2_4; }
//{ExampleGroup.annotationInfo=current}
public Action getExampleGroupAnnotationInfoAction_2_4_0() { return cExampleGroupAnnotationInfoAction_2_4_0; }
//preamble="context" targetOperation=[types::JvmOperation|Method]? name=STRING? "{" members+=Member* "}"
public Group getGroup_2_4_1() { return cGroup_2_4_1; }
//preamble="context"
public Assignment getPreambleAssignment_2_4_1_0() { return cPreambleAssignment_2_4_1_0; }
//"context"
public Keyword getPreambleContextKeyword_2_4_1_0_0() { return cPreambleContextKeyword_2_4_1_0_0; }
//targetOperation=[types::JvmOperation|Method]?
public Assignment getTargetOperationAssignment_2_4_1_1() { return cTargetOperationAssignment_2_4_1_1; }
//[types::JvmOperation|Method]
public CrossReference getTargetOperationJvmOperationCrossReference_2_4_1_1_0() { return cTargetOperationJvmOperationCrossReference_2_4_1_1_0; }
//Method
public RuleCall getTargetOperationJvmOperationMethodParserRuleCall_2_4_1_1_0_1() { return cTargetOperationJvmOperationMethodParserRuleCall_2_4_1_1_0_1; }
//name=STRING?
public Assignment getNameAssignment_2_4_1_2() { return cNameAssignment_2_4_1_2; }
//STRING
public RuleCall getNameSTRINGTerminalRuleCall_2_4_1_2_0() { return cNameSTRINGTerminalRuleCall_2_4_1_2_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_4_1_3() { return cLeftCurlyBracketKeyword_2_4_1_3; }
//members+=Member*
public Assignment getMembersAssignment_2_4_1_4() { return cMembersAssignment_2_4_1_4; }
//Member
public RuleCall getMembersMemberParserRuleCall_2_4_1_4_0() { return cMembersMemberParserRuleCall_2_4_1_4_0; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_4_1_5() { return cRightCurlyBracketKeyword_2_4_1_5; }
//{ExampleTable.annotationInfo=current} ("def" name=ID? "{" ("|" columns+=ExampleColumn* rows+=ExampleRow*)? "}")
public Group getGroup_2_5() { return cGroup_2_5; }
//{ExampleTable.annotationInfo=current}
public Action getExampleTableAnnotationInfoAction_2_5_0() { return cExampleTableAnnotationInfoAction_2_5_0; }
//"def" name=ID? "{" ("|" columns+=ExampleColumn* rows+=ExampleRow*)? "}"
public Group getGroup_2_5_1() { return cGroup_2_5_1; }
//"def"
public Keyword getDefKeyword_2_5_1_0() { return cDefKeyword_2_5_1_0; }
//name=ID?
public Assignment getNameAssignment_2_5_1_1() { return cNameAssignment_2_5_1_1; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_5_1_1_0() { return cNameIDTerminalRuleCall_2_5_1_1_0; }
//"{"
public Keyword getLeftCurlyBracketKeyword_2_5_1_2() { return cLeftCurlyBracketKeyword_2_5_1_2; }
//("|" columns+=ExampleColumn* rows+=ExampleRow*)?
public Group getGroup_2_5_1_3() { return cGroup_2_5_1_3; }
//"|"
public Keyword getVerticalLineKeyword_2_5_1_3_0() { return cVerticalLineKeyword_2_5_1_3_0; }
//columns+=ExampleColumn*
public Assignment getColumnsAssignment_2_5_1_3_1() { return cColumnsAssignment_2_5_1_3_1; }
//ExampleColumn
public RuleCall getColumnsExampleColumnParserRuleCall_2_5_1_3_1_0() { return cColumnsExampleColumnParserRuleCall_2_5_1_3_1_0; }
//rows+=ExampleRow*
public Assignment getRowsAssignment_2_5_1_3_2() { return cRowsAssignment_2_5_1_3_2; }
//ExampleRow
public RuleCall getRowsExampleRowParserRuleCall_2_5_1_3_2_0() { return cRowsExampleRowParserRuleCall_2_5_1_3_2_0; }
//"}"
public Keyword getRightCurlyBracketKeyword_2_5_1_4() { return cRightCurlyBracketKeyword_2_5_1_4; }
//{xtend::XtendField.annotationInfo=current} modifiers+=CommonModifier* (modifiers+=FieldModifier
//modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID | modifiers+="extension" (modifiers+=FieldModifier |
//modifiers+=CommonModifier)* type=JvmTypeReference name=ValidID? | modifiers+=FieldModifier modifiers+=CommonModifier*
//modifiers+="extension" modifiers+=CommonModifier* type=JvmTypeReference name=ValidID? | type=JvmTypeReference
//name=ValidID) ("=" initialValue=XExpression)? ";"?
public Group getGroup_2_6() { return cGroup_2_6; }
//{xtend::XtendField.annotationInfo=current}
public Action getXtendFieldAnnotationInfoAction_2_6_0() { return cXtendFieldAnnotationInfoAction_2_6_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_6_1() { return cModifiersAssignment_2_6_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_6_1_0() { return cModifiersCommonModifierParserRuleCall_2_6_1_0; }
//modifiers+=FieldModifier modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID | modifiers+="extension"
//(modifiers+=FieldModifier | modifiers+=CommonModifier)* type=JvmTypeReference name=ValidID? | modifiers+=FieldModifier
//modifiers+=CommonModifier* modifiers+="extension" modifiers+=CommonModifier* type=JvmTypeReference name=ValidID? |
//type=JvmTypeReference name=ValidID
public Alternatives getAlternatives_2_6_2() { return cAlternatives_2_6_2; }
//modifiers+=FieldModifier modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID
public Group getGroup_2_6_2_0() { return cGroup_2_6_2_0; }
//modifiers+=FieldModifier
public Assignment getModifiersAssignment_2_6_2_0_0() { return cModifiersAssignment_2_6_2_0_0; }
//FieldModifier
public RuleCall getModifiersFieldModifierParserRuleCall_2_6_2_0_0_0() { return cModifiersFieldModifierParserRuleCall_2_6_2_0_0_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_6_2_0_1() { return cModifiersAssignment_2_6_2_0_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_6_2_0_1_0() { return cModifiersCommonModifierParserRuleCall_2_6_2_0_1_0; }
//type=JvmTypeReference?
public Assignment getTypeAssignment_2_6_2_0_2() { return cTypeAssignment_2_6_2_0_2; }
//JvmTypeReference
public RuleCall getTypeJvmTypeReferenceParserRuleCall_2_6_2_0_2_0() { return cTypeJvmTypeReferenceParserRuleCall_2_6_2_0_2_0; }
//name=ValidID
public Assignment getNameAssignment_2_6_2_0_3() { return cNameAssignment_2_6_2_0_3; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_6_2_0_3_0() { return cNameValidIDParserRuleCall_2_6_2_0_3_0; }
//modifiers+="extension" (modifiers+=FieldModifier | modifiers+=CommonModifier)* type=JvmTypeReference name=ValidID?
public Group getGroup_2_6_2_1() { return cGroup_2_6_2_1; }
//modifiers+="extension"
public Assignment getModifiersAssignment_2_6_2_1_0() { return cModifiersAssignment_2_6_2_1_0; }
//"extension"
public Keyword getModifiersExtensionKeyword_2_6_2_1_0_0() { return cModifiersExtensionKeyword_2_6_2_1_0_0; }
//(modifiers+=FieldModifier | modifiers+=CommonModifier)*
public Alternatives getAlternatives_2_6_2_1_1() { return cAlternatives_2_6_2_1_1; }
//modifiers+=FieldModifier
public Assignment getModifiersAssignment_2_6_2_1_1_0() { return cModifiersAssignment_2_6_2_1_1_0; }
//FieldModifier
public RuleCall getModifiersFieldModifierParserRuleCall_2_6_2_1_1_0_0() { return cModifiersFieldModifierParserRuleCall_2_6_2_1_1_0_0; }
//modifiers+=CommonModifier
public Assignment getModifiersAssignment_2_6_2_1_1_1() { return cModifiersAssignment_2_6_2_1_1_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_6_2_1_1_1_0() { return cModifiersCommonModifierParserRuleCall_2_6_2_1_1_1_0; }
//type=JvmTypeReference
public Assignment getTypeAssignment_2_6_2_1_2() { return cTypeAssignment_2_6_2_1_2; }
//JvmTypeReference
public RuleCall getTypeJvmTypeReferenceParserRuleCall_2_6_2_1_2_0() { return cTypeJvmTypeReferenceParserRuleCall_2_6_2_1_2_0; }
//name=ValidID?
public Assignment getNameAssignment_2_6_2_1_3() { return cNameAssignment_2_6_2_1_3; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_6_2_1_3_0() { return cNameValidIDParserRuleCall_2_6_2_1_3_0; }
//modifiers+=FieldModifier modifiers+=CommonModifier* modifiers+="extension" modifiers+=CommonModifier*
//type=JvmTypeReference name=ValidID?
public Group getGroup_2_6_2_2() { return cGroup_2_6_2_2; }
//modifiers+=FieldModifier
public Assignment getModifiersAssignment_2_6_2_2_0() { return cModifiersAssignment_2_6_2_2_0; }
//FieldModifier
public RuleCall getModifiersFieldModifierParserRuleCall_2_6_2_2_0_0() { return cModifiersFieldModifierParserRuleCall_2_6_2_2_0_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_6_2_2_1() { return cModifiersAssignment_2_6_2_2_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_6_2_2_1_0() { return cModifiersCommonModifierParserRuleCall_2_6_2_2_1_0; }
//modifiers+="extension"
public Assignment getModifiersAssignment_2_6_2_2_2() { return cModifiersAssignment_2_6_2_2_2; }
//"extension"
public Keyword getModifiersExtensionKeyword_2_6_2_2_2_0() { return cModifiersExtensionKeyword_2_6_2_2_2_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_6_2_2_3() { return cModifiersAssignment_2_6_2_2_3; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_6_2_2_3_0() { return cModifiersCommonModifierParserRuleCall_2_6_2_2_3_0; }
//type=JvmTypeReference
public Assignment getTypeAssignment_2_6_2_2_4() { return cTypeAssignment_2_6_2_2_4; }
//JvmTypeReference
public RuleCall getTypeJvmTypeReferenceParserRuleCall_2_6_2_2_4_0() { return cTypeJvmTypeReferenceParserRuleCall_2_6_2_2_4_0; }
//name=ValidID?
public Assignment getNameAssignment_2_6_2_2_5() { return cNameAssignment_2_6_2_2_5; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_6_2_2_5_0() { return cNameValidIDParserRuleCall_2_6_2_2_5_0; }
//type=JvmTypeReference name=ValidID
public Group getGroup_2_6_2_3() { return cGroup_2_6_2_3; }
//type=JvmTypeReference
public Assignment getTypeAssignment_2_6_2_3_0() { return cTypeAssignment_2_6_2_3_0; }
//JvmTypeReference
public RuleCall getTypeJvmTypeReferenceParserRuleCall_2_6_2_3_0_0() { return cTypeJvmTypeReferenceParserRuleCall_2_6_2_3_0_0; }
//name=ValidID
public Assignment getNameAssignment_2_6_2_3_1() { return cNameAssignment_2_6_2_3_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_6_2_3_1_0() { return cNameValidIDParserRuleCall_2_6_2_3_1_0; }
//("=" initialValue=XExpression)?
public Group getGroup_2_6_3() { return cGroup_2_6_3; }
//"="
public Keyword getEqualsSignKeyword_2_6_3_0() { return cEqualsSignKeyword_2_6_3_0; }
//initialValue=XExpression
public Assignment getInitialValueAssignment_2_6_3_1() { return cInitialValueAssignment_2_6_3_1; }
//XExpression
public RuleCall getInitialValueXExpressionParserRuleCall_2_6_3_1_0() { return cInitialValueXExpressionParserRuleCall_2_6_3_1_0; }
//";"?
public Keyword getSemicolonKeyword_2_6_4() { return cSemicolonKeyword_2_6_4; }
//{xtend::XtendFunction.annotationInfo=current} modifiers+=CommonModifier* modifiers+=MethodModifier
//(modifiers+=CommonModifier | modifiers+=MethodModifier)* ("<" typeParameters+=JvmTypeParameter (","
//typeParameters+=JvmTypeParameter)* ">")? (=> (returnType=JvmTypeReference createExtensionInfo=CreateExtensionInfo
//name=ValidID "(") | => (returnType=JvmTypeReference name=ValidID "(") | => (createExtensionInfo=CreateExtensionInfo
//name=ValidID "(") | name=ValidID "(") (parameters+=Parameter ("," parameters+=Parameter)*)? ")" ("throws"
//exceptions+=JvmTypeReference ("," exceptions+=JvmTypeReference)*)? (expression=XBlockExpression | expression=RichString
//| ";")?
public Group getGroup_2_7() { return cGroup_2_7; }
//{xtend::XtendFunction.annotationInfo=current}
public Action getXtendFunctionAnnotationInfoAction_2_7_0() { return cXtendFunctionAnnotationInfoAction_2_7_0; }
//modifiers+=CommonModifier*
public Assignment getModifiersAssignment_2_7_1() { return cModifiersAssignment_2_7_1; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_7_1_0() { return cModifiersCommonModifierParserRuleCall_2_7_1_0; }
//modifiers+=MethodModifier
public Assignment getModifiersAssignment_2_7_2() { return cModifiersAssignment_2_7_2; }
//MethodModifier
public RuleCall getModifiersMethodModifierParserRuleCall_2_7_2_0() { return cModifiersMethodModifierParserRuleCall_2_7_2_0; }
//(modifiers+=CommonModifier | modifiers+=MethodModifier)*
public Alternatives getAlternatives_2_7_3() { return cAlternatives_2_7_3; }
//modifiers+=CommonModifier
public Assignment getModifiersAssignment_2_7_3_0() { return cModifiersAssignment_2_7_3_0; }
//CommonModifier
public RuleCall getModifiersCommonModifierParserRuleCall_2_7_3_0_0() { return cModifiersCommonModifierParserRuleCall_2_7_3_0_0; }
//modifiers+=MethodModifier
public Assignment getModifiersAssignment_2_7_3_1() { return cModifiersAssignment_2_7_3_1; }
//MethodModifier
public RuleCall getModifiersMethodModifierParserRuleCall_2_7_3_1_0() { return cModifiersMethodModifierParserRuleCall_2_7_3_1_0; }
//("<" typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")?
public Group getGroup_2_7_4() { return cGroup_2_7_4; }
//"<"
public Keyword getLessThanSignKeyword_2_7_4_0() { return cLessThanSignKeyword_2_7_4_0; }
//typeParameters+=JvmTypeParameter
public Assignment getTypeParametersAssignment_2_7_4_1() { return cTypeParametersAssignment_2_7_4_1; }
//JvmTypeParameter
public RuleCall getTypeParametersJvmTypeParameterParserRuleCall_2_7_4_1_0() { return cTypeParametersJvmTypeParameterParserRuleCall_2_7_4_1_0; }
//("," typeParameters+=JvmTypeParameter)*
public Group getGroup_2_7_4_2() { return cGroup_2_7_4_2; }
//","
public Keyword getCommaKeyword_2_7_4_2_0() { return cCommaKeyword_2_7_4_2_0; }
//typeParameters+=JvmTypeParameter
public Assignment getTypeParametersAssignment_2_7_4_2_1() { return cTypeParametersAssignment_2_7_4_2_1; }
//JvmTypeParameter
public RuleCall getTypeParametersJvmTypeParameterParserRuleCall_2_7_4_2_1_0() { return cTypeParametersJvmTypeParameterParserRuleCall_2_7_4_2_1_0; }
//">"
public Keyword getGreaterThanSignKeyword_2_7_4_3() { return cGreaterThanSignKeyword_2_7_4_3; }
//=> (returnType=JvmTypeReference createExtensionInfo=CreateExtensionInfo name=ValidID "(") | =>
//(returnType=JvmTypeReference name=ValidID "(") | => (createExtensionInfo=CreateExtensionInfo name=ValidID "(") |
//name=ValidID "("
public Alternatives getAlternatives_2_7_5() { return cAlternatives_2_7_5; }
//=> (returnType=JvmTypeReference createExtensionInfo=CreateExtensionInfo name=ValidID "(")
public Group getGroup_2_7_5_0() { return cGroup_2_7_5_0; }
//returnType=JvmTypeReference createExtensionInfo=CreateExtensionInfo name=ValidID "("
public Group getGroup_2_7_5_0_0() { return cGroup_2_7_5_0_0; }
//returnType=JvmTypeReference
public Assignment getReturnTypeAssignment_2_7_5_0_0_0() { return cReturnTypeAssignment_2_7_5_0_0_0; }
//JvmTypeReference
public RuleCall getReturnTypeJvmTypeReferenceParserRuleCall_2_7_5_0_0_0_0() { return cReturnTypeJvmTypeReferenceParserRuleCall_2_7_5_0_0_0_0; }
//createExtensionInfo=CreateExtensionInfo
public Assignment getCreateExtensionInfoAssignment_2_7_5_0_0_1() { return cCreateExtensionInfoAssignment_2_7_5_0_0_1; }
//CreateExtensionInfo
public RuleCall getCreateExtensionInfoCreateExtensionInfoParserRuleCall_2_7_5_0_0_1_0() { return cCreateExtensionInfoCreateExtensionInfoParserRuleCall_2_7_5_0_0_1_0; }
//name=ValidID
public Assignment getNameAssignment_2_7_5_0_0_2() { return cNameAssignment_2_7_5_0_0_2; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_7_5_0_0_2_0() { return cNameValidIDParserRuleCall_2_7_5_0_0_2_0; }
//"("
public Keyword getLeftParenthesisKeyword_2_7_5_0_0_3() { return cLeftParenthesisKeyword_2_7_5_0_0_3; }
//=> (returnType=JvmTypeReference name=ValidID "(")
public Group getGroup_2_7_5_1() { return cGroup_2_7_5_1; }
//returnType=JvmTypeReference name=ValidID "("
public Group getGroup_2_7_5_1_0() { return cGroup_2_7_5_1_0; }
//returnType=JvmTypeReference
public Assignment getReturnTypeAssignment_2_7_5_1_0_0() { return cReturnTypeAssignment_2_7_5_1_0_0; }
//JvmTypeReference
public RuleCall getReturnTypeJvmTypeReferenceParserRuleCall_2_7_5_1_0_0_0() { return cReturnTypeJvmTypeReferenceParserRuleCall_2_7_5_1_0_0_0; }
//name=ValidID
public Assignment getNameAssignment_2_7_5_1_0_1() { return cNameAssignment_2_7_5_1_0_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_7_5_1_0_1_0() { return cNameValidIDParserRuleCall_2_7_5_1_0_1_0; }
//"("
public Keyword getLeftParenthesisKeyword_2_7_5_1_0_2() { return cLeftParenthesisKeyword_2_7_5_1_0_2; }
//=> (createExtensionInfo=CreateExtensionInfo name=ValidID "(")
public Group getGroup_2_7_5_2() { return cGroup_2_7_5_2; }
//createExtensionInfo=CreateExtensionInfo name=ValidID "("
public Group getGroup_2_7_5_2_0() { return cGroup_2_7_5_2_0; }
//createExtensionInfo=CreateExtensionInfo
public Assignment getCreateExtensionInfoAssignment_2_7_5_2_0_0() { return cCreateExtensionInfoAssignment_2_7_5_2_0_0; }
//CreateExtensionInfo
public RuleCall getCreateExtensionInfoCreateExtensionInfoParserRuleCall_2_7_5_2_0_0_0() { return cCreateExtensionInfoCreateExtensionInfoParserRuleCall_2_7_5_2_0_0_0; }
//name=ValidID
public Assignment getNameAssignment_2_7_5_2_0_1() { return cNameAssignment_2_7_5_2_0_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_7_5_2_0_1_0() { return cNameValidIDParserRuleCall_2_7_5_2_0_1_0; }
//"("
public Keyword getLeftParenthesisKeyword_2_7_5_2_0_2() { return cLeftParenthesisKeyword_2_7_5_2_0_2; }
//name=ValidID "("
public Group getGroup_2_7_5_3() { return cGroup_2_7_5_3; }
//name=ValidID
public Assignment getNameAssignment_2_7_5_3_0() { return cNameAssignment_2_7_5_3_0; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_7_5_3_0_0() { return cNameValidIDParserRuleCall_2_7_5_3_0_0; }
//"("
public Keyword getLeftParenthesisKeyword_2_7_5_3_1() { return cLeftParenthesisKeyword_2_7_5_3_1; }
//(parameters+=Parameter ("," parameters+=Parameter)*)?
public Group getGroup_2_7_6() { return cGroup_2_7_6; }
//parameters+=Parameter
public Assignment getParametersAssignment_2_7_6_0() { return cParametersAssignment_2_7_6_0; }
//Parameter
public RuleCall getParametersParameterParserRuleCall_2_7_6_0_0() { return cParametersParameterParserRuleCall_2_7_6_0_0; }
//("," parameters+=Parameter)*
public Group getGroup_2_7_6_1() { return cGroup_2_7_6_1; }
//","
public Keyword getCommaKeyword_2_7_6_1_0() { return cCommaKeyword_2_7_6_1_0; }
//parameters+=Parameter
public Assignment getParametersAssignment_2_7_6_1_1() { return cParametersAssignment_2_7_6_1_1; }
//Parameter
public RuleCall getParametersParameterParserRuleCall_2_7_6_1_1_0() { return cParametersParameterParserRuleCall_2_7_6_1_1_0; }
//")"
public Keyword getRightParenthesisKeyword_2_7_7() { return cRightParenthesisKeyword_2_7_7; }
//("throws" exceptions+=JvmTypeReference ("," exceptions+=JvmTypeReference)*)?
public Group getGroup_2_7_8() { return cGroup_2_7_8; }
//"throws"
public Keyword getThrowsKeyword_2_7_8_0() { return cThrowsKeyword_2_7_8_0; }
//exceptions+=JvmTypeReference
public Assignment getExceptionsAssignment_2_7_8_1() { return cExceptionsAssignment_2_7_8_1; }
//JvmTypeReference
public RuleCall getExceptionsJvmTypeReferenceParserRuleCall_2_7_8_1_0() { return cExceptionsJvmTypeReferenceParserRuleCall_2_7_8_1_0; }
//("," exceptions+=JvmTypeReference)*
public Group getGroup_2_7_8_2() { return cGroup_2_7_8_2; }
//","
public Keyword getCommaKeyword_2_7_8_2_0() { return cCommaKeyword_2_7_8_2_0; }
//exceptions+=JvmTypeReference
public Assignment getExceptionsAssignment_2_7_8_2_1() { return cExceptionsAssignment_2_7_8_2_1; }
//JvmTypeReference
public RuleCall getExceptionsJvmTypeReferenceParserRuleCall_2_7_8_2_1_0() { return cExceptionsJvmTypeReferenceParserRuleCall_2_7_8_2_1_0; }
//(expression=XBlockExpression | expression=RichString | ";")?
public Alternatives getAlternatives_2_7_9() { return cAlternatives_2_7_9; }
//expression=XBlockExpression
public Assignment getExpressionAssignment_2_7_9_0() { return cExpressionAssignment_2_7_9_0; }
//XBlockExpression
public RuleCall getExpressionXBlockExpressionParserRuleCall_2_7_9_0_0() { return cExpressionXBlockExpressionParserRuleCall_2_7_9_0_0; }
//expression=RichString
public Assignment getExpressionAssignment_2_7_9_1() { return cExpressionAssignment_2_7_9_1; }
//RichString
public RuleCall getExpressionRichStringParserRuleCall_2_7_9_1_0() { return cExpressionRichStringParserRuleCall_2_7_9_1_0; }
//";"
public Keyword getSemicolonKeyword_2_7_9_2() { return cSemicolonKeyword_2_7_9_2; }
}
public class XPrimaryExpressionElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "XPrimaryExpression");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final RuleCall cXConstructorCallParserRuleCall_0 = (RuleCall)cAlternatives.eContents().get(0);
private final RuleCall cXBlockExpressionParserRuleCall_1 = (RuleCall)cAlternatives.eContents().get(1);
private final RuleCall cXSwitchExpressionParserRuleCall_2 = (RuleCall)cAlternatives.eContents().get(2);
private final RuleCall cXSynchronizedExpressionParserRuleCall_3 = (RuleCall)cAlternatives.eContents().get(3);
private final RuleCall cXFeatureCallParserRuleCall_4 = (RuleCall)cAlternatives.eContents().get(4);
private final RuleCall cXLiteralParserRuleCall_5 = (RuleCall)cAlternatives.eContents().get(5);
private final RuleCall cXIfExpressionParserRuleCall_6 = (RuleCall)cAlternatives.eContents().get(6);
private final RuleCall cXForLoopExpressionParserRuleCall_7 = (RuleCall)cAlternatives.eContents().get(7);
private final RuleCall cXBasicForLoopExpressionParserRuleCall_8 = (RuleCall)cAlternatives.eContents().get(8);
private final RuleCall cXWhileExpressionParserRuleCall_9 = (RuleCall)cAlternatives.eContents().get(9);
private final RuleCall cXDoWhileExpressionParserRuleCall_10 = (RuleCall)cAlternatives.eContents().get(10);
private final RuleCall cXThrowExpressionParserRuleCall_11 = (RuleCall)cAlternatives.eContents().get(11);
private final RuleCall cXReturnExpressionParserRuleCall_12 = (RuleCall)cAlternatives.eContents().get(12);
private final RuleCall cXTryCatchFinallyExpressionParserRuleCall_13 = (RuleCall)cAlternatives.eContents().get(13);
private final RuleCall cXParenthesizedExpressionParserRuleCall_14 = (RuleCall)cAlternatives.eContents().get(14);
private final RuleCall cAssertionParserRuleCall_15 = (RuleCall)cAlternatives.eContents().get(15);
//XPrimaryExpression returns xbase::XExpression:
// XConstructorCall | XBlockExpression | XSwitchExpression | XSynchronizedExpression | XFeatureCall | XLiteral |
// XIfExpression | XForLoopExpression | XBasicForLoopExpression | XWhileExpression | XDoWhileExpression |
// XThrowExpression | XReturnExpression | XTryCatchFinallyExpression | XParenthesizedExpression | Assertion;
public ParserRule getRule() { return rule; }
//XConstructorCall | XBlockExpression | XSwitchExpression | XSynchronizedExpression | XFeatureCall | XLiteral |
//XIfExpression | XForLoopExpression | XBasicForLoopExpression | XWhileExpression | XDoWhileExpression | XThrowExpression
//| XReturnExpression | XTryCatchFinallyExpression | XParenthesizedExpression | Assertion
public Alternatives getAlternatives() { return cAlternatives; }
//XConstructorCall
public RuleCall getXConstructorCallParserRuleCall_0() { return cXConstructorCallParserRuleCall_0; }
//XBlockExpression
public RuleCall getXBlockExpressionParserRuleCall_1() { return cXBlockExpressionParserRuleCall_1; }
//XSwitchExpression
public RuleCall getXSwitchExpressionParserRuleCall_2() { return cXSwitchExpressionParserRuleCall_2; }
//XSynchronizedExpression
public RuleCall getXSynchronizedExpressionParserRuleCall_3() { return cXSynchronizedExpressionParserRuleCall_3; }
//XFeatureCall
public RuleCall getXFeatureCallParserRuleCall_4() { return cXFeatureCallParserRuleCall_4; }
//XLiteral
public RuleCall getXLiteralParserRuleCall_5() { return cXLiteralParserRuleCall_5; }
//XIfExpression
public RuleCall getXIfExpressionParserRuleCall_6() { return cXIfExpressionParserRuleCall_6; }
//XForLoopExpression
public RuleCall getXForLoopExpressionParserRuleCall_7() { return cXForLoopExpressionParserRuleCall_7; }
//XBasicForLoopExpression
public RuleCall getXBasicForLoopExpressionParserRuleCall_8() { return cXBasicForLoopExpressionParserRuleCall_8; }
//XWhileExpression
public RuleCall getXWhileExpressionParserRuleCall_9() { return cXWhileExpressionParserRuleCall_9; }
//XDoWhileExpression
public RuleCall getXDoWhileExpressionParserRuleCall_10() { return cXDoWhileExpressionParserRuleCall_10; }
//XThrowExpression
public RuleCall getXThrowExpressionParserRuleCall_11() { return cXThrowExpressionParserRuleCall_11; }
//XReturnExpression
public RuleCall getXReturnExpressionParserRuleCall_12() { return cXReturnExpressionParserRuleCall_12; }
//XTryCatchFinallyExpression
public RuleCall getXTryCatchFinallyExpressionParserRuleCall_13() { return cXTryCatchFinallyExpressionParserRuleCall_13; }
//XParenthesizedExpression
public RuleCall getXParenthesizedExpressionParserRuleCall_14() { return cXParenthesizedExpressionParserRuleCall_14; }
//Assertion
public RuleCall getAssertionParserRuleCall_15() { return cAssertionParserRuleCall_15; }
}
public class XRelationalExpressionElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "XRelationalExpression");
private final Group cGroup = (Group)rule.eContents().get(1);
private final RuleCall cXOtherOperatorExpressionParserRuleCall_0 = (RuleCall)cGroup.eContents().get(0);
private final Alternatives cAlternatives_1 = (Alternatives)cGroup.eContents().get(1);
private final Group cGroup_1_0 = (Group)cAlternatives_1.eContents().get(0);
private final Group cGroup_1_0_0 = (Group)cGroup_1_0.eContents().get(0);
private final Group cGroup_1_0_0_0 = (Group)cGroup_1_0_0.eContents().get(0);
private final Action cShouldLeftOperandAction_1_0_0_0_0 = (Action)cGroup_1_0_0_0.eContents().get(0);
private final Assignment cFeatureAssignment_1_0_0_0_1 = (Assignment)cGroup_1_0_0_0.eContents().get(1);
private final CrossReference cFeatureJvmIdentifiableElementCrossReference_1_0_0_0_1_0 = (CrossReference)cFeatureAssignment_1_0_0_0_1.eContents().get(0);
private final RuleCall cFeatureJvmIdentifiableElementShouldParserRuleCall_1_0_0_0_1_0_1 = (RuleCall)cFeatureJvmIdentifiableElementCrossReference_1_0_0_0_1_0.eContents().get(1);
private final Assignment cRightOperandAssignment_1_0_1 = (Assignment)cGroup_1_0.eContents().get(1);
private final RuleCall cRightOperandXOtherOperatorExpressionParserRuleCall_1_0_1_0 = (RuleCall)cRightOperandAssignment_1_0_1.eContents().get(0);
private final Group cGroup_1_1 = (Group)cAlternatives_1.eContents().get(1);
private final Group cGroup_1_1_0 = (Group)cGroup_1_1.eContents().get(0);
private final Group cGroup_1_1_0_0 = (Group)cGroup_1_1_0.eContents().get(0);
private final Action cShouldThrowExpressionAction_1_1_0_0_0 = (Action)cGroup_1_1_0_0.eContents().get(0);
private final Alternatives cAlternatives_1_1_0_0_1 = (Alternatives)cGroup_1_1_0_0.eContents().get(1);
private final Group cGroup_1_1_0_0_1_0 = (Group)cAlternatives_1_1_0_0_1.eContents().get(0);
private final Keyword cShouldKeyword_1_1_0_0_1_0_0 = (Keyword)cGroup_1_1_0_0_1_0.eContents().get(0);
private final Keyword cThrowKeyword_1_1_0_0_1_0_1 = (Keyword)cGroup_1_1_0_0_1_0.eContents().get(1);
private final Keyword cThrowsKeyword_1_1_0_0_1_1 = (Keyword)cAlternatives_1_1_0_0_1.eContents().get(1);
private final Assignment cTypeAssignment_1_1_1 = (Assignment)cGroup_1_1.eContents().get(1);
private final RuleCall cTypeJvmTypeReferenceParserRuleCall_1_1_1_0 = (RuleCall)cTypeAssignment_1_1_1.eContents().get(0);
private final Group cGroup_1_2 = (Group)cAlternatives_1.eContents().get(2);
private final Group cGroup_1_2_0 = (Group)cGroup_1_2.eContents().get(0);
private final Group cGroup_1_2_0_0 = (Group)cGroup_1_2_0.eContents().get(0);
private final Action cXInstanceOfExpressionExpressionAction_1_2_0_0_0 = (Action)cGroup_1_2_0_0.eContents().get(0);
private final Keyword cInstanceofKeyword_1_2_0_0_1 = (Keyword)cGroup_1_2_0_0.eContents().get(1);
private final Assignment cTypeAssignment_1_2_1 = (Assignment)cGroup_1_2.eContents().get(1);
private final RuleCall cTypeJvmTypeReferenceParserRuleCall_1_2_1_0 = (RuleCall)cTypeAssignment_1_2_1.eContents().get(0);
private final Group cGroup_1_3 = (Group)cAlternatives_1.eContents().get(3);
private final Group cGroup_1_3_0 = (Group)cGroup_1_3.eContents().get(0);
private final Group cGroup_1_3_0_0 = (Group)cGroup_1_3_0.eContents().get(0);
private final Action cXBinaryOperationLeftOperandAction_1_3_0_0_0 = (Action)cGroup_1_3_0_0.eContents().get(0);
private final Assignment cFeatureAssignment_1_3_0_0_1 = (Assignment)cGroup_1_3_0_0.eContents().get(1);
private final CrossReference cFeatureJvmIdentifiableElementCrossReference_1_3_0_0_1_0 = (CrossReference)cFeatureAssignment_1_3_0_0_1.eContents().get(0);
private final RuleCall cFeatureJvmIdentifiableElementOpCompareParserRuleCall_1_3_0_0_1_0_1 = (RuleCall)cFeatureJvmIdentifiableElementCrossReference_1_3_0_0_1_0.eContents().get(1);
private final Assignment cRightOperandAssignment_1_3_1 = (Assignment)cGroup_1_3.eContents().get(1);
private final RuleCall cRightOperandXOtherOperatorExpressionParserRuleCall_1_3_1_0 = (RuleCall)cRightOperandAssignment_1_3_1.eContents().get(0);
//XRelationalExpression returns xbase::XExpression:
// XOtherOperatorExpression (=> ({Should.leftOperand=current} feature=[types::JvmIdentifiableElement|Should]) =>
// rightOperand=XOtherOperatorExpression? | => ({ShouldThrow.expression=current} ("should" "throw" | "throws"))
// type=JvmTypeReference | => ({xbase::XInstanceOfExpression.expression=current} "instanceof") type=JvmTypeReference | =>
// ({xbase::XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpCompare])
// rightOperand=XOtherOperatorExpression)*;
public ParserRule getRule() { return rule; }
//XOtherOperatorExpression (=> ({Should.leftOperand=current} feature=[types::JvmIdentifiableElement|Should]) =>
//rightOperand=XOtherOperatorExpression? | => ({ShouldThrow.expression=current} ("should" "throw" | "throws"))
//type=JvmTypeReference | => ({xbase::XInstanceOfExpression.expression=current} "instanceof") type=JvmTypeReference | =>
//({xbase::XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpCompare])
//rightOperand=XOtherOperatorExpression)*
public Group getGroup() { return cGroup; }
//XOtherOperatorExpression
public RuleCall getXOtherOperatorExpressionParserRuleCall_0() { return cXOtherOperatorExpressionParserRuleCall_0; }
//(=> ({Should.leftOperand=current} feature=[types::JvmIdentifiableElement|Should]) =>
//rightOperand=XOtherOperatorExpression? | => ({ShouldThrow.expression=current} ("should" "throw" | "throws"))
//type=JvmTypeReference | => ({xbase::XInstanceOfExpression.expression=current} "instanceof") type=JvmTypeReference | =>
//({xbase::XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpCompare])
//rightOperand=XOtherOperatorExpression)*
public Alternatives getAlternatives_1() { return cAlternatives_1; }
//=> ({Should.leftOperand=current} feature=[types::JvmIdentifiableElement|Should]) =>
//rightOperand=XOtherOperatorExpression?
public Group getGroup_1_0() { return cGroup_1_0; }
//=> ({Should.leftOperand=current} feature=[types::JvmIdentifiableElement|Should])
public Group getGroup_1_0_0() { return cGroup_1_0_0; }
//{Should.leftOperand=current} feature=[types::JvmIdentifiableElement|Should]
public Group getGroup_1_0_0_0() { return cGroup_1_0_0_0; }
//{Should.leftOperand=current}
public Action getShouldLeftOperandAction_1_0_0_0_0() { return cShouldLeftOperandAction_1_0_0_0_0; }
//feature=[types::JvmIdentifiableElement|Should]
public Assignment getFeatureAssignment_1_0_0_0_1() { return cFeatureAssignment_1_0_0_0_1; }
//[types::JvmIdentifiableElement|Should]
public CrossReference getFeatureJvmIdentifiableElementCrossReference_1_0_0_0_1_0() { return cFeatureJvmIdentifiableElementCrossReference_1_0_0_0_1_0; }
//Should
public RuleCall getFeatureJvmIdentifiableElementShouldParserRuleCall_1_0_0_0_1_0_1() { return cFeatureJvmIdentifiableElementShouldParserRuleCall_1_0_0_0_1_0_1; }
//=> rightOperand=XOtherOperatorExpression?
public Assignment getRightOperandAssignment_1_0_1() { return cRightOperandAssignment_1_0_1; }
//XOtherOperatorExpression
public RuleCall getRightOperandXOtherOperatorExpressionParserRuleCall_1_0_1_0() { return cRightOperandXOtherOperatorExpressionParserRuleCall_1_0_1_0; }
//=> ({ShouldThrow.expression=current} ("should" "throw" | "throws")) type=JvmTypeReference
public Group getGroup_1_1() { return cGroup_1_1; }
//=> ({ShouldThrow.expression=current} ("should" "throw" | "throws"))
public Group getGroup_1_1_0() { return cGroup_1_1_0; }
//{ShouldThrow.expression=current} ("should" "throw" | "throws")
public Group getGroup_1_1_0_0() { return cGroup_1_1_0_0; }
//{ShouldThrow.expression=current}
public Action getShouldThrowExpressionAction_1_1_0_0_0() { return cShouldThrowExpressionAction_1_1_0_0_0; }
//"should" "throw" | "throws"
public Alternatives getAlternatives_1_1_0_0_1() { return cAlternatives_1_1_0_0_1; }
//"should" "throw"
public Group getGroup_1_1_0_0_1_0() { return cGroup_1_1_0_0_1_0; }
//"should"
public Keyword getShouldKeyword_1_1_0_0_1_0_0() { return cShouldKeyword_1_1_0_0_1_0_0; }
//"throw"
public Keyword getThrowKeyword_1_1_0_0_1_0_1() { return cThrowKeyword_1_1_0_0_1_0_1; }
//"throws"
public Keyword getThrowsKeyword_1_1_0_0_1_1() { return cThrowsKeyword_1_1_0_0_1_1; }
//type=JvmTypeReference
public Assignment getTypeAssignment_1_1_1() { return cTypeAssignment_1_1_1; }
//JvmTypeReference
public RuleCall getTypeJvmTypeReferenceParserRuleCall_1_1_1_0() { return cTypeJvmTypeReferenceParserRuleCall_1_1_1_0; }
//=> ({xbase::XInstanceOfExpression.expression=current} "instanceof") type=JvmTypeReference
public Group getGroup_1_2() { return cGroup_1_2; }
//=> ({xbase::XInstanceOfExpression.expression=current} "instanceof")
public Group getGroup_1_2_0() { return cGroup_1_2_0; }
//{xbase::XInstanceOfExpression.expression=current} "instanceof"
public Group getGroup_1_2_0_0() { return cGroup_1_2_0_0; }
//{xbase::XInstanceOfExpression.expression=current}
public Action getXInstanceOfExpressionExpressionAction_1_2_0_0_0() { return cXInstanceOfExpressionExpressionAction_1_2_0_0_0; }
//"instanceof"
public Keyword getInstanceofKeyword_1_2_0_0_1() { return cInstanceofKeyword_1_2_0_0_1; }
//type=JvmTypeReference
public Assignment getTypeAssignment_1_2_1() { return cTypeAssignment_1_2_1; }
//JvmTypeReference
public RuleCall getTypeJvmTypeReferenceParserRuleCall_1_2_1_0() { return cTypeJvmTypeReferenceParserRuleCall_1_2_1_0; }
//=> ({xbase::XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpCompare])
//rightOperand=XOtherOperatorExpression
public Group getGroup_1_3() { return cGroup_1_3; }
//=> ({xbase::XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpCompare])
public Group getGroup_1_3_0() { return cGroup_1_3_0; }
//{xbase::XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpCompare]
public Group getGroup_1_3_0_0() { return cGroup_1_3_0_0; }
//{xbase::XBinaryOperation.leftOperand=current}
public Action getXBinaryOperationLeftOperandAction_1_3_0_0_0() { return cXBinaryOperationLeftOperandAction_1_3_0_0_0; }
//feature=[types::JvmIdentifiableElement|OpCompare]
public Assignment getFeatureAssignment_1_3_0_0_1() { return cFeatureAssignment_1_3_0_0_1; }
//[types::JvmIdentifiableElement|OpCompare]
public CrossReference getFeatureJvmIdentifiableElementCrossReference_1_3_0_0_1_0() { return cFeatureJvmIdentifiableElementCrossReference_1_3_0_0_1_0; }
//OpCompare
public RuleCall getFeatureJvmIdentifiableElementOpCompareParserRuleCall_1_3_0_0_1_0_1() { return cFeatureJvmIdentifiableElementOpCompareParserRuleCall_1_3_0_0_1_0_1; }
//rightOperand=XOtherOperatorExpression
public Assignment getRightOperandAssignment_1_3_1() { return cRightOperandAssignment_1_3_1; }
//XOtherOperatorExpression
public RuleCall getRightOperandXOtherOperatorExpressionParserRuleCall_1_3_1_0() { return cRightOperandXOtherOperatorExpressionParserRuleCall_1_3_1_0; }
}
public class ShouldElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Should");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cShouldKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Keyword cNotKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final RuleCall cIDTerminalRuleCall_2 = (RuleCall)cGroup.eContents().get(2);
//Should:
// "should" "not"? ID;
public ParserRule getRule() { return rule; }
//"should" "not"? ID
public Group getGroup() { return cGroup; }
//"should"
public Keyword getShouldKeyword_0() { return cShouldKeyword_0; }
//"not"?
public Keyword getNotKeyword_1() { return cNotKeyword_1; }
//ID
public RuleCall getIDTerminalRuleCall_2() { return cIDTerminalRuleCall_2; }
}
public class AssertionElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Assertion");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Action cAssertionAction_0 = (Action)cGroup.eContents().get(0);
private final Keyword cAssertKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cExpressionAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cExpressionXExpressionParserRuleCall_2_0 = (RuleCall)cExpressionAssignment_2.eContents().get(0);
//Assertion returns xbase::XExpression:
// {Assertion} "assert" expression=XExpression;
public ParserRule getRule() { return rule; }
//{Assertion} "assert" expression=XExpression
public Group getGroup() { return cGroup; }
//{Assertion}
public Action getAssertionAction_0() { return cAssertionAction_0; }
//"assert"
public Keyword getAssertKeyword_1() { return cAssertKeyword_1; }
//expression=XExpression
public Assignment getExpressionAssignment_2() { return cExpressionAssignment_2; }
//XExpression
public RuleCall getExpressionXExpressionParserRuleCall_2_0() { return cExpressionXExpressionParserRuleCall_2_0; }
}
public class ExampleColumnElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "ExampleColumn");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cTypeAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cTypeJvmTypeReferenceParserRuleCall_0_0 = (RuleCall)cTypeAssignment_0.eContents().get(0);
private final Assignment cNameAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_1_0 = (RuleCall)cNameAssignment_1.eContents().get(0);
private final Keyword cVerticalLineKeyword_2 = (Keyword)cGroup.eContents().get(2);
//ExampleColumn:
// type=JvmTypeReference? name=ValidID "|";
public ParserRule getRule() { return rule; }
//type=JvmTypeReference? name=ValidID "|"
public Group getGroup() { return cGroup; }
//type=JvmTypeReference?
public Assignment getTypeAssignment_0() { return cTypeAssignment_0; }
//JvmTypeReference
public RuleCall getTypeJvmTypeReferenceParserRuleCall_0_0() { return cTypeJvmTypeReferenceParserRuleCall_0_0; }
//name=ValidID
public Assignment getNameAssignment_1() { return cNameAssignment_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_1_0() { return cNameValidIDParserRuleCall_1_0; }
//"|"
public Keyword getVerticalLineKeyword_2() { return cVerticalLineKeyword_2; }
}
public class ExampleRowElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "ExampleRow");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cVerticalLineKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Action cExampleRowAction_1 = (Action)cGroup.eContents().get(1);
private final Group cGroup_2 = (Group)cGroup.eContents().get(2);
private final Assignment cCellsAssignment_2_0 = (Assignment)cGroup_2.eContents().get(0);
private final RuleCall cCellsExampleCellParserRuleCall_2_0_0 = (RuleCall)cCellsAssignment_2_0.eContents().get(0);
private final Keyword cVerticalLineKeyword_2_1 = (Keyword)cGroup_2.eContents().get(1);
//ExampleRow:
// "|" {ExampleRow} (cells+=ExampleCell "|")*;
public ParserRule getRule() { return rule; }
//"|" {ExampleRow} (cells+=ExampleCell "|")*
public Group getGroup() { return cGroup; }
//"|"
public Keyword getVerticalLineKeyword_0() { return cVerticalLineKeyword_0; }
//{ExampleRow}
public Action getExampleRowAction_1() { return cExampleRowAction_1; }
//(cells+=ExampleCell "|")*
public Group getGroup_2() { return cGroup_2; }
//cells+=ExampleCell
public Assignment getCellsAssignment_2_0() { return cCellsAssignment_2_0; }
//ExampleCell
public RuleCall getCellsExampleCellParserRuleCall_2_0_0() { return cCellsExampleCellParserRuleCall_2_0_0; }
//"|"
public Keyword getVerticalLineKeyword_2_1() { return cVerticalLineKeyword_2_1; }
}
public class ExampleCellElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "ExampleCell");
private final Assignment cExpressionAssignment = (Assignment)rule.eContents().get(1);
private final RuleCall cExpressionXExpressionParserRuleCall_0 = (RuleCall)cExpressionAssignment.eContents().get(0);
//ExampleCell:
// expression=XExpression;
public ParserRule getRule() { return rule; }
//expression=XExpression
public Assignment getExpressionAssignment() { return cExpressionAssignment; }
//XExpression
public RuleCall getExpressionXExpressionParserRuleCall_0() { return cExpressionXExpressionParserRuleCall_0; }
}
private SpecFileElements pSpecFile;
private TypeElements pType;
private MethodElements pMethod;
private TypeNameElements pTypeName;
private TypeRefElements pTypeRef;
private MemberElements pMember;
private XPrimaryExpressionElements pXPrimaryExpression;
private XRelationalExpressionElements pXRelationalExpression;
private ShouldElements pShould;
private AssertionElements pAssertion;
private ExampleColumnElements pExampleColumn;
private ExampleRowElements pExampleRow;
private ExampleCellElements pExampleCell;
private TerminalRule tML_COMMENT;
private TerminalRule tRICH_TEXT;
private TerminalRule tRICH_TEXT_START;
private TerminalRule tRICH_TEXT_END;
private TerminalRule tRICH_TEXT_INBETWEEN;
private TerminalRule tCOMMENT_RICH_TEXT_INBETWEEN;
private TerminalRule tCOMMENT_RICH_TEXT_END;
private TerminalRule tIN_RICH_STRING;
private final Grammar grammar;
private XtendGrammarAccess gaXtend;
@Inject
public SpecGrammarAccess(GrammarProvider grammarProvider,
XtendGrammarAccess gaXtend) {
this.grammar = internalFindGrammar(grammarProvider);
this.gaXtend = gaXtend;
}
protected Grammar internalFindGrammar(GrammarProvider grammarProvider) {
Grammar grammar = grammarProvider.getGrammar(this);
while (grammar != null) {
if ("org.jnario.spec.Spec".equals(grammar.getName())) {
return grammar;
}
List grammars = grammar.getUsedGrammars();
if (!grammars.isEmpty()) {
grammar = grammars.iterator().next();
} else {
return null;
}
}
return grammar;
}
public Grammar getGrammar() {
return grammar;
}
public XtendGrammarAccess getXtendGrammarAccess() {
return gaXtend;
}
//SpecFile:
// {SpecFile} ("package" package=QualifiedName)? importSection=XImportSection? xtendTypes+=Type*;
public SpecFileElements getSpecFileAccess() {
return (pSpecFile != null) ? pSpecFile : (pSpecFile = new SpecFileElements());
}
public ParserRule getSpecFileRule() {
return getSpecFileAccess().getRule();
}
//Type returns xtend::XtendTypeDeclaration:
// {xtend::XtendTypeDeclaration} annotations+=XAnnotation* ({xtend::XtendClass.annotationInfo=current}
// modifiers+=CommonModifier* "class" name=ValidID ("<" typeParameters+=JvmTypeParameter (","
// typeParameters+=JvmTypeParameter)* ">")? ("extends" extends=JvmParameterizedTypeReference)? ("implements"
// implements+=JvmParameterizedTypeReference ("," implements+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}"
// | {xtend::XtendInterface.annotationInfo=current} modifiers+=CommonModifier* "interface" name=ValidID ("<"
// typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? ("extends"
// extends+=JvmParameterizedTypeReference ("," extends+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" |
// {xtend::XtendEnum.annotationInfo=current} modifiers+=CommonModifier* "enum" name=ValidID "{"
// (members+=XtendEnumLiteral ("," members+=XtendEnumLiteral)*)? ";"? "}" |
// {xtend::XtendAnnotationType.annotationInfo=current} modifiers+=CommonModifier* "annotation" name=ValidID "{"
// members+=AnnotationField* "}" | {ExampleGroup.annotationInfo=current} preamble="describe" targetType=JvmTypeReference?
// name=STRING? "{" members+=Member* "}");
public TypeElements getTypeAccess() {
return (pType != null) ? pType : (pType = new TypeElements());
}
public ParserRule getTypeRule() {
return getTypeAccess().getRule();
}
//Method:
// ID ("(" (TypeName ("," TypeName)*)? ")")?;
public MethodElements getMethodAccess() {
return (pMethod != null) ? pMethod : (pMethod = new MethodElements());
}
public ParserRule getMethodRule() {
return getMethodAccess().getRule();
}
//TypeName:
// ID ("<" TypeRef ("," TypeRef)* ">")? ("[" "]")?;
public TypeNameElements getTypeNameAccess() {
return (pTypeName != null) ? pTypeName : (pTypeName = new TypeNameElements());
}
public ParserRule getTypeNameRule() {
return getTypeNameAccess().getRule();
}
//TypeRef:
// "?"? ("extends" | "super")? TypeName;
public TypeRefElements getTypeRefAccess() {
return (pTypeRef != null) ? pTypeRef : (pTypeRef = new TypeRefElements());
}
public ParserRule getTypeRefRule() {
return getTypeRefAccess().getRule();
}
//Member returns xtend::XtendMember:
// {xtend::XtendMember} annotations+=XAnnotation* ({Example.annotationInfo=current} (pending?="pending"? ("fact" |
// "facts") expr=XExpression expression=XBlockExpression?) | {ExampleGroup.annotationInfo=current} (preamble="describe"
// targetType=JvmTypeReference? name=STRING? "{" members+=Member* "}") | {Before.annotationInfo=current} ("before"
// (modifiers+="all" | "each")? => name=STRING? expression=XExpression) | {After.annotationInfo=current} ("after"
// (modifiers+="all" | "each")? => name=STRING? expression=XExpression) | {ExampleGroup.annotationInfo=current}
// (preamble="context" targetOperation=[types::JvmOperation|Method]? name=STRING? "{" members+=Member* "}") |
// {ExampleTable.annotationInfo=current} ("def" name=ID? "{" ("|" columns+=ExampleColumn* rows+=ExampleRow*)? "}") |
// {xtend::XtendField.annotationInfo=current} modifiers+=CommonModifier* (modifiers+=FieldModifier
// modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID | modifiers+="extension" (modifiers+=FieldModifier |
// modifiers+=CommonModifier)* type=JvmTypeReference name=ValidID? | modifiers+=FieldModifier modifiers+=CommonModifier*
// modifiers+="extension" modifiers+=CommonModifier* type=JvmTypeReference name=ValidID? | type=JvmTypeReference
// name=ValidID) ("=" initialValue=XExpression)? ";"? | {xtend::XtendFunction.annotationInfo=current}
// modifiers+=CommonModifier* modifiers+=MethodModifier (modifiers+=CommonModifier | modifiers+=MethodModifier)* ("<"
// typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? (=> (returnType=JvmTypeReference
// createExtensionInfo=CreateExtensionInfo name=ValidID "(") | => (returnType=JvmTypeReference name=ValidID "(") | =>
// (createExtensionInfo=CreateExtensionInfo name=ValidID "(") | name=ValidID "(") (parameters+=Parameter (","
// parameters+=Parameter)*)? ")" ("throws" exceptions+=JvmTypeReference ("," exceptions+=JvmTypeReference)*)?
// (expression=XBlockExpression | expression=RichString | ";")?);
public MemberElements getMemberAccess() {
return (pMember != null) ? pMember : (pMember = new MemberElements());
}
public ParserRule getMemberRule() {
return getMemberAccess().getRule();
}
//XPrimaryExpression returns xbase::XExpression:
// XConstructorCall | XBlockExpression | XSwitchExpression | XSynchronizedExpression | XFeatureCall | XLiteral |
// XIfExpression | XForLoopExpression | XBasicForLoopExpression | XWhileExpression | XDoWhileExpression |
// XThrowExpression | XReturnExpression | XTryCatchFinallyExpression | XParenthesizedExpression | Assertion;
public XPrimaryExpressionElements getXPrimaryExpressionAccess() {
return (pXPrimaryExpression != null) ? pXPrimaryExpression : (pXPrimaryExpression = new XPrimaryExpressionElements());
}
public ParserRule getXPrimaryExpressionRule() {
return getXPrimaryExpressionAccess().getRule();
}
//XRelationalExpression returns xbase::XExpression:
// XOtherOperatorExpression (=> ({Should.leftOperand=current} feature=[types::JvmIdentifiableElement|Should]) =>
// rightOperand=XOtherOperatorExpression? | => ({ShouldThrow.expression=current} ("should" "throw" | "throws"))
// type=JvmTypeReference | => ({xbase::XInstanceOfExpression.expression=current} "instanceof") type=JvmTypeReference | =>
// ({xbase::XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpCompare])
// rightOperand=XOtherOperatorExpression)*;
public XRelationalExpressionElements getXRelationalExpressionAccess() {
return (pXRelationalExpression != null) ? pXRelationalExpression : (pXRelationalExpression = new XRelationalExpressionElements());
}
public ParserRule getXRelationalExpressionRule() {
return getXRelationalExpressionAccess().getRule();
}
//Should:
// "should" "not"? ID;
public ShouldElements getShouldAccess() {
return (pShould != null) ? pShould : (pShould = new ShouldElements());
}
public ParserRule getShouldRule() {
return getShouldAccess().getRule();
}
//Assertion returns xbase::XExpression:
// {Assertion} "assert" expression=XExpression;
public AssertionElements getAssertionAccess() {
return (pAssertion != null) ? pAssertion : (pAssertion = new AssertionElements());
}
public ParserRule getAssertionRule() {
return getAssertionAccess().getRule();
}
//ExampleColumn:
// type=JvmTypeReference? name=ValidID "|";
public ExampleColumnElements getExampleColumnAccess() {
return (pExampleColumn != null) ? pExampleColumn : (pExampleColumn = new ExampleColumnElements());
}
public ParserRule getExampleColumnRule() {
return getExampleColumnAccess().getRule();
}
//ExampleRow:
// "|" {ExampleRow} (cells+=ExampleCell "|")*;
public ExampleRowElements getExampleRowAccess() {
return (pExampleRow != null) ? pExampleRow : (pExampleRow = new ExampleRowElements());
}
public ParserRule getExampleRowRule() {
return getExampleRowAccess().getRule();
}
//ExampleCell:
// expression=XExpression;
public ExampleCellElements getExampleCellAccess() {
return (pExampleCell != null) ? pExampleCell : (pExampleCell = new ExampleCellElements());
}
public ParserRule getExampleCellRule() {
return getExampleCellAccess().getRule();
}
//terminal ML_COMMENT:
// "/ *"->(!"\\" "* /");
public TerminalRule getML_COMMENTRule() {
return (tML_COMMENT != null) ? tML_COMMENT : (tML_COMMENT = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "ML_COMMENT"));
}
//terminal RICH_TEXT:
// "\'\'\'" IN_RICH_STRING* ("\'\'\'" | ("\'" "\'"?)? EOF);
public TerminalRule getRICH_TEXTRule() {
return (tRICH_TEXT != null) ? tRICH_TEXT : (tRICH_TEXT = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "RICH_TEXT"));
}
//terminal RICH_TEXT_START:
// "\'\'\'" IN_RICH_STRING* ("\'" "\'"?)? "«";
public TerminalRule getRICH_TEXT_STARTRule() {
return (tRICH_TEXT_START != null) ? tRICH_TEXT_START : (tRICH_TEXT_START = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "RICH_TEXT_START"));
}
//terminal RICH_TEXT_END:
// "»" IN_RICH_STRING* ("\'\'\'" | ("\'" "\'"?)? EOF);
public TerminalRule getRICH_TEXT_ENDRule() {
return (tRICH_TEXT_END != null) ? tRICH_TEXT_END : (tRICH_TEXT_END = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "RICH_TEXT_END"));
}
//terminal RICH_TEXT_INBETWEEN:
// "»" IN_RICH_STRING* ("\'" "\'"?)? "«";
public TerminalRule getRICH_TEXT_INBETWEENRule() {
return (tRICH_TEXT_INBETWEEN != null) ? tRICH_TEXT_INBETWEEN : (tRICH_TEXT_INBETWEEN = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "RICH_TEXT_INBETWEEN"));
}
//terminal COMMENT_RICH_TEXT_INBETWEEN:
// "««" !("\n" | "\r")* ("\r"? "\n" IN_RICH_STRING* ("\'" "\'"?)? "«")?;
public TerminalRule getCOMMENT_RICH_TEXT_INBETWEENRule() {
return (tCOMMENT_RICH_TEXT_INBETWEEN != null) ? tCOMMENT_RICH_TEXT_INBETWEEN : (tCOMMENT_RICH_TEXT_INBETWEEN = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "COMMENT_RICH_TEXT_INBETWEEN"));
}
//terminal COMMENT_RICH_TEXT_END:
// "««" !("\n" | "\r")* ("\r"? "\n" IN_RICH_STRING* ("\'\'\'" | ("\'" "\'"?)? EOF) | EOF);
public TerminalRule getCOMMENT_RICH_TEXT_ENDRule() {
return (tCOMMENT_RICH_TEXT_END != null) ? tCOMMENT_RICH_TEXT_END : (tCOMMENT_RICH_TEXT_END = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "COMMENT_RICH_TEXT_END"));
}
//terminal fragment IN_RICH_STRING:
// "\'\'" !("«" | "\'") | "\'" !("«" | "\'") | !("«" | "\'");
public TerminalRule getIN_RICH_STRINGRule() {
return (tIN_RICH_STRING != null) ? tIN_RICH_STRING : (tIN_RICH_STRING = (TerminalRule) GrammarUtil.findRuleForName(getGrammar(), "IN_RICH_STRING"));
}
//File returns XtendFile:
// ("package" package=QualifiedName ";"?)? importSection=XImportSection? xtendTypes+=Type*;
public XtendGrammarAccess.FileElements getFileAccess() {
return gaXtend.getFileAccess();
}
public ParserRule getFileRule() {
return getFileAccess().getRule();
}
//AnnotationField returns XtendMember:
// {XtendMember} annotations+=XAnnotation* (({XtendField.annotationInfo=current} modifiers+=CommonModifier*
// modifiers+=FieldModifier modifiers+=CommonModifier* type=JvmTypeReference? name=ValidID |
// {XtendField.annotationInfo=current} modifiers+=CommonModifier* type=JvmTypeReference name=ValidID) ("="
// initialValue=XAnnotationElementValue)? ";"? | {XtendClass.annotationInfo=current} modifiers+=CommonModifier* "class"
// name=ValidID ("<" typeParameters+=JvmTypeParameter ("," typeParameters+=JvmTypeParameter)* ">")? ("extends"
// extends=JvmParameterizedTypeReference)? ("implements" implements+=JvmParameterizedTypeReference (","
// implements+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" | {XtendInterface.annotationInfo=current}
// modifiers+=CommonModifier* "interface" name=ValidID ("<" typeParameters+=JvmTypeParameter (","
// typeParameters+=JvmTypeParameter)* ">")? ("extends" extends+=JvmParameterizedTypeReference (","
// extends+=JvmParameterizedTypeReference)*)? "{" members+=Member* "}" | {XtendEnum.annotationInfo=current}
// modifiers+=CommonModifier* "enum" name=ValidID "{" (members+=XtendEnumLiteral ("," members+=XtendEnumLiteral)*)? ";"?
// "}" | {XtendAnnotationType.annotationInfo=current} modifiers+=CommonModifier* "annotation" name=ValidID "{"
// members+=AnnotationField* "}");
public XtendGrammarAccess.AnnotationFieldElements getAnnotationFieldAccess() {
return gaXtend.getAnnotationFieldAccess();
}
public ParserRule getAnnotationFieldRule() {
return getAnnotationFieldAccess().getRule();
}
//TypeReferenceNoTypeArgs returns types::JvmParameterizedTypeReference:
// type=[types::JvmType|QualifiedName];
public XtendGrammarAccess.TypeReferenceNoTypeArgsElements getTypeReferenceNoTypeArgsAccess() {
return gaXtend.getTypeReferenceNoTypeArgsAccess();
}
public ParserRule getTypeReferenceNoTypeArgsRule() {
return getTypeReferenceNoTypeArgsAccess().getRule();
}
//FunctionID:
// ValidID | Operators;
public XtendGrammarAccess.FunctionIDElements getFunctionIDAccess() {
return gaXtend.getFunctionIDAccess();
}
public ParserRule getFunctionIDRule() {
return getFunctionIDAccess().getRule();
}
//Operators:
// OpMultiAssign // | OpAdd completely contained in OpUnary
// | OpOr | OpAnd | OpEquality | OpCompare | OpOther | OpMulti | OpUnary | OpPostfix;
public XtendGrammarAccess.OperatorsElements getOperatorsAccess() {
return gaXtend.getOperatorsAccess();
}
public ParserRule getOperatorsRule() {
return getOperatorsAccess().getRule();
}
//XtendEnumLiteral:
// name=ValidID;
public XtendGrammarAccess.XtendEnumLiteralElements getXtendEnumLiteralAccess() {
return gaXtend.getXtendEnumLiteralAccess();
}
public ParserRule getXtendEnumLiteralRule() {
return getXtendEnumLiteralAccess().getRule();
}
//CommonModifier:
// "public" | "private" | "protected" | "package" | "abstract" | "static" | "dispatch" | "final" | "strictfp" | "native"
// | "volatile" | "synchronized" | "transient";
public XtendGrammarAccess.CommonModifierElements getCommonModifierAccess() {
return gaXtend.getCommonModifierAccess();
}
public ParserRule getCommonModifierRule() {
return getCommonModifierAccess().getRule();
}
//FieldModifier:
// "val" | "var";
public XtendGrammarAccess.FieldModifierElements getFieldModifierAccess() {
return gaXtend.getFieldModifierAccess();
}
public ParserRule getFieldModifierRule() {
return getFieldModifierAccess().getRule();
}
//MethodModifier:
// "def" | "override";
public XtendGrammarAccess.MethodModifierElements getMethodModifierAccess() {
return gaXtend.getMethodModifierAccess();
}
public ParserRule getMethodModifierRule() {
return getMethodModifierAccess().getRule();
}
//CreateExtensionInfo:
// "create" (name=ValidID ":")? createExpression=XExpression;
public XtendGrammarAccess.CreateExtensionInfoElements getCreateExtensionInfoAccess() {
return gaXtend.getCreateExtensionInfoAccess();
}
public ParserRule getCreateExtensionInfoRule() {
return getCreateExtensionInfoAccess().getRule();
}
//ValidID:
// ID | "create" | "annotation" | "AFTER" | "BEFORE" | "SEPARATOR";
public XtendGrammarAccess.ValidIDElements getValidIDAccess() {
return gaXtend.getValidIDAccess();
}
public ParserRule getValidIDRule() {
return getValidIDAccess().getRule();
}
//// For feature calls we add 'extension' since there are method such as 'isExtension' or 'getExtension' out there.
//FeatureCallID:
// InnerVarID | "extension";
public XtendGrammarAccess.FeatureCallIDElements getFeatureCallIDAccess() {
return gaXtend.getFeatureCallIDAccess();
}
public ParserRule getFeatureCallIDRule() {
return getFeatureCallIDAccess().getRule();
}
//InnerVarID:
// ID | "abstract" | "annotation" | "class" | "create" | "def" | "dispatch" | "enum" | "extends" | "final" | "implements"
// | "import" | "interface" | "override" | "package" | "public" | "private" | "protected" | "static" | "throws" |
// "strictfp" | "native" | "volatile" | "synchronized" | "transient" | "AFTER" | "BEFORE" | "SEPARATOR";
public XtendGrammarAccess.InnerVarIDElements getInnerVarIDAccess() {
return gaXtend.getInnerVarIDAccess();
}
public ParserRule getInnerVarIDRule() {
return getInnerVarIDAccess().getRule();
}
//Parameter returns XtendParameter:
// annotations+=XAnnotation* (extension?="extension" annotations+=XAnnotation*)? parameterType=JvmTypeReference
// varArg?="..."? name=ValidID;
public XtendGrammarAccess.ParameterElements getParameterAccess() {
return gaXtend.getParameterAccess();
}
public ParserRule getParameterRule() {
return getParameterAccess().getRule();
}
//XVariableDeclaration returns xbase::XExpression:
// => ({XtendVariableDeclaration} ((writeable?="var" | "val") extension?="extension"? | extension?="extension"
// (writeable?="var" | "val"))) (=> (type=JvmTypeReference name=InnerVarID) | name=InnerVarID) ("=" right=XExpression)?;
public XtendGrammarAccess.XVariableDeclarationElements getXVariableDeclarationAccess() {
return gaXtend.getXVariableDeclarationAccess();
}
public ParserRule getXVariableDeclarationRule() {
return getXVariableDeclarationAccess().getRule();
}
//XConstructorCall returns xbase::XExpression:
// XbaseConstructorCall (=> ({AnonymousClass.constructorCall=current} "{") members+=Member* "}")?;
public XtendGrammarAccess.XConstructorCallElements getXConstructorCallAccess() {
return gaXtend.getXConstructorCallAccess();
}
public ParserRule getXConstructorCallRule() {
return getXConstructorCallAccess().getRule();
}
//XbaseConstructorCall returns xbase::XConstructorCall:
// {xbase::XConstructorCall} "new" constructor=[types::JvmConstructor|QualifiedName] ("<"
// typeArguments+=JvmArgumentTypeReference ("," typeArguments+=JvmArgumentTypeReference)* ">")? (=>
// explicitConstructorCall?="(" (arguments+=XShortClosure | arguments+=XExpression ("," arguments+=XExpression)*)? ")")?
// arguments+=XClosure?;
public XtendGrammarAccess.XbaseConstructorCallElements getXbaseConstructorCallAccess() {
return gaXtend.getXbaseConstructorCallAccess();
}
public ParserRule getXbaseConstructorCallRule() {
return getXbaseConstructorCallAccess().getRule();
}
//JvmFormalParameter returns XtendFormalParameter:
// extension?="extension"? parameterType=JvmTypeReference? name=InnerVarID;
public XtendGrammarAccess.JvmFormalParameterElements getJvmFormalParameterAccess() {
return gaXtend.getJvmFormalParameterAccess();
}
public ParserRule getJvmFormalParameterRule() {
return getJvmFormalParameterAccess().getRule();
}
//FullJvmFormalParameter returns XtendFormalParameter:
// extension?="extension"? parameterType=JvmTypeReference name=InnerVarID;
public XtendGrammarAccess.FullJvmFormalParameterElements getFullJvmFormalParameterAccess() {
return gaXtend.getFullJvmFormalParameterAccess();
}
public ParserRule getFullJvmFormalParameterRule() {
return getFullJvmFormalParameterAccess().getRule();
}
//XStringLiteral returns xbase::XExpression:
// SimpleStringLiteral | RichString;
public XtendGrammarAccess.XStringLiteralElements getXStringLiteralAccess() {
return gaXtend.getXStringLiteralAccess();
}
public ParserRule getXStringLiteralRule() {
return getXStringLiteralAccess().getRule();
}
//XSwitchExpression returns xbase::XExpression:
// {xbase::XSwitchExpression} "switch" (=> ("(" declaredParam=JvmFormalParameter ":") switch=XExpression ")" | =>
// (declaredParam=JvmFormalParameter ":")? switch=XExpressionOrSimpleConstructorCall) "{" cases+=XCasePart* ("default"
// ":" default=XExpression)? "}";
public XtendGrammarAccess.XSwitchExpressionElements getXSwitchExpressionAccess() {
return gaXtend.getXSwitchExpressionAccess();
}
public ParserRule getXSwitchExpressionRule() {
return getXSwitchExpressionAccess().getRule();
}
//XExpressionOrSimpleConstructorCall returns xbase::XExpression:
// XbaseConstructorCall | XExpression;
public XtendGrammarAccess.XExpressionOrSimpleConstructorCallElements getXExpressionOrSimpleConstructorCallAccess() {
return gaXtend.getXExpressionOrSimpleConstructorCallAccess();
}
public ParserRule getXExpressionOrSimpleConstructorCallRule() {
return getXExpressionOrSimpleConstructorCallAccess().getRule();
}
//SimpleStringLiteral returns xbase::XExpression:
// {xbase::XStringLiteral} value=STRING;
public XtendGrammarAccess.SimpleStringLiteralElements getSimpleStringLiteralAccess() {
return gaXtend.getSimpleStringLiteralAccess();
}
public ParserRule getSimpleStringLiteralRule() {
return getSimpleStringLiteralAccess().getRule();
}
//RichString returns xbase::XExpression:
// {RichString} (expressions+=RichStringLiteral | expressions+=RichStringLiteralStart expressions+=RichStringPart?
// (expressions+=RichStringLiteralInbetween expressions+=RichStringPart?)* expressions+=RichStringLiteralEnd);
public XtendGrammarAccess.RichStringElements getRichStringAccess() {
return gaXtend.getRichStringAccess();
}
public ParserRule getRichStringRule() {
return getRichStringAccess().getRule();
}
//RichStringLiteral returns xbase::XExpression:
// {RichStringLiteral} value=RICH_TEXT;
public XtendGrammarAccess.RichStringLiteralElements getRichStringLiteralAccess() {
return gaXtend.getRichStringLiteralAccess();
}
public ParserRule getRichStringLiteralRule() {
return getRichStringLiteralAccess().getRule();
}
//RichStringLiteralStart returns xbase::XExpression:
// {RichStringLiteral} value=RICH_TEXT_START;
public XtendGrammarAccess.RichStringLiteralStartElements getRichStringLiteralStartAccess() {
return gaXtend.getRichStringLiteralStartAccess();
}
public ParserRule getRichStringLiteralStartRule() {
return getRichStringLiteralStartAccess().getRule();
}
//RichStringLiteralInbetween returns xbase::XExpression:
// {RichStringLiteral} (value=RICH_TEXT_INBETWEEN | value=COMMENT_RICH_TEXT_INBETWEEN);
public XtendGrammarAccess.RichStringLiteralInbetweenElements getRichStringLiteralInbetweenAccess() {
return gaXtend.getRichStringLiteralInbetweenAccess();
}
public ParserRule getRichStringLiteralInbetweenRule() {
return getRichStringLiteralInbetweenAccess().getRule();
}
//RichStringLiteralEnd returns xbase::XExpression:
// {RichStringLiteral} (value=RICH_TEXT_END | value=COMMENT_RICH_TEXT_END);
public XtendGrammarAccess.RichStringLiteralEndElements getRichStringLiteralEndAccess() {
return gaXtend.getRichStringLiteralEndAccess();
}
public ParserRule getRichStringLiteralEndRule() {
return getRichStringLiteralEndAccess().getRule();
}
//InternalRichString returns xbase::XExpression:
// {RichString} (expressions+=RichStringLiteralInbetween (expressions+=RichStringPart?
// expressions+=RichStringLiteralInbetween)*);
public XtendGrammarAccess.InternalRichStringElements getInternalRichStringAccess() {
return gaXtend.getInternalRichStringAccess();
}
public ParserRule getInternalRichStringRule() {
return getInternalRichStringAccess().getRule();
}
//RichStringPart returns xbase::XExpression:
// XExpressionOrVarDeclaration | RichStringForLoop | RichStringIf;
public XtendGrammarAccess.RichStringPartElements getRichStringPartAccess() {
return gaXtend.getRichStringPartAccess();
}
public ParserRule getRichStringPartRule() {
return getRichStringPartAccess().getRule();
}
//RichStringForLoop returns xbase::XExpression:
// {RichStringForLoop} "FOR" declaredParam=JvmFormalParameter ":" forExpression=XExpression ("BEFORE"
// before=XExpression)? ("SEPARATOR" separator=XExpression)? ("AFTER" after=XExpression)?
// eachExpression=InternalRichString "ENDFOR";
public XtendGrammarAccess.RichStringForLoopElements getRichStringForLoopAccess() {
return gaXtend.getRichStringForLoopAccess();
}
public ParserRule getRichStringForLoopRule() {
return getRichStringForLoopAccess().getRule();
}
//RichStringIf returns xbase::XExpression:
// {RichStringIf} "IF" if=XExpression then=InternalRichString elseIfs+=RichStringElseIf* ("ELSE"
// else=InternalRichString)? "ENDIF";
public XtendGrammarAccess.RichStringIfElements getRichStringIfAccess() {
return gaXtend.getRichStringIfAccess();
}
public ParserRule getRichStringIfRule() {
return getRichStringIfAccess().getRule();
}
//RichStringElseIf:
// "ELSEIF" if=XExpression then=InternalRichString;
public XtendGrammarAccess.RichStringElseIfElements getRichStringElseIfAccess() {
return gaXtend.getRichStringElseIfAccess();
}
public ParserRule getRichStringElseIfRule() {
return getRichStringElseIfAccess().getRule();
}
//terminal ID:
// "^"? (IDENTIFIER_START | UNICODE_ESCAPE) (IDENTIFIER_PART | UNICODE_ESCAPE)*;
public TerminalRule getIDRule() {
return gaXtend.getIDRule();
}
//terminal fragment HEX_DIGIT:
// "0".."9" | "a".."f" | "A".."F";
public TerminalRule getHEX_DIGITRule() {
return gaXtend.getHEX_DIGITRule();
}
//terminal fragment UNICODE_ESCAPE:
// "\\" "u" (HEX_DIGIT (HEX_DIGIT (HEX_DIGIT HEX_DIGIT?)?)?)?;
public TerminalRule getUNICODE_ESCAPERule() {
return gaXtend.getUNICODE_ESCAPERule();
}
//terminal fragment IDENTIFIER_START:
// "$" | "A".."Z" | "_" | "a".."z" | "¢".."¥" | "ª" | "µ" | "º" | "À".."Ö" | "Ø".."ö" | "ø".."ȶ" | "ɐ".."ˁ" | "ˆ".."ˑ" |
// "ˠ".."ˤ" | "ˮ" | "ͺ" | "Ά" | "Έ".."Ί" | "Ό" | "Ύ".."Ρ" | "Σ".."ώ" | "ϐ".."ϵ" | "Ϸ".."ϻ" | "Ѐ".."ҁ" | "Ҋ".."ӎ" |
// "Ӑ".."ӵ" | "Ӹ".."ӹ" | "Ԁ".."ԏ" | "Ա".."Ֆ" | "ՙ" | "ա".."և" | "א".."ת" | "װ".."ײ" | "ء".."غ" | "ـ".."ي" | "ٮ".."ٯ" |
// "ٱ".."ۓ" | "ە" | "ۥ".."ۦ" | "ۮ".."ۯ" | "ۺ".."ۼ" | "ۿ" | "ܐ" | "ܒ".."ܯ" | "ݍ".."ݏ" | "ހ".."ޥ" | "ޱ" | "ऄ".."ह" | "ऽ" |
// "ॐ" | "क़".."ॡ" | "অ".."ঌ" | "এ".."ঐ" | "ও".."ন" | "প".."র" | "ল" | "শ".."হ" | "ঽ" | "ড়".."ঢ়" | "য়".."ৡ" | "ৰ".."৳" |
// "ਅ".."ਊ" | "ਏ".."ਐ" | "ਓ".."ਨ" | "ਪ".."ਰ" | "ਲ".."ਲ਼" | "ਵ".."ਸ਼" | "ਸ".."ਹ" | "ਖ਼".."ੜ" | "ਫ਼" | "ੲ".."ੴ" | "અ".."ઍ" |
// "એ".."ઑ" | "ઓ".."ન" | "પ".."ર" | "લ".."ળ" | "વ".."હ" | "ઽ" | "ૐ" | "ૠ".."ૡ" | "૱" | "ଅ".."ଌ" | "ଏ".."ଐ" | "ଓ".."ନ" |
// "ପ".."ର" | "ଲ".."ଳ" | "ଵ".."ହ" | "ଽ" | "ଡ଼".."ଢ଼" | "ୟ".."ୡ" | "ୱ" | "ஃ" | "அ".."ஊ" | "எ".."ஐ" | "ஒ".."க" | "ங".."ச" |
// "ஜ" | "ஞ".."ட" | "ண".."த" | "ந".."ப" | "ம".."வ" | "ஷ".."ஹ" | "௹" | "అ".."ఌ" | "ఎ".."ఐ" | "ఒ".."న" | "ప".."ళ" |
// "వ".."హ" | "ౠ".."ౡ" | "ಅ".."ಌ" | "ಎ".."ಐ" | "ಒ".."ನ" | "ಪ".."ಳ" | "ವ".."ಹ" | "ಽ" | "ೞ" | "ೠ".."ೡ" | "അ".."ഌ" |
// "എ".."ഐ" | "ഒ".."ന" | "പ".."ഹ" | "ൠ".."ൡ" | "අ".."ඖ" | "ක".."න" | "ඳ".."ර" | "ල" | "ව".."ෆ" | "ก".."ะ" | "า".."ำ" |
// "฿".."ๆ" | "ກ".."ຂ" | "ຄ" | "ງ".."ຈ" | "ຊ" | "ຍ" | "ດ".."ທ" | "ນ".."ຟ" | "ມ".."ຣ" | "ລ" | "ວ" | "ສ".."ຫ" | "ອ".."ະ" |
// "າ".."ຳ" | "ຽ" | "ເ".."ໄ" | "ໆ" | "ໜ".."ໝ" | "ༀ" | "ཀ".."ཇ" | "ཉ".."ཪ" | "ྈ".."ྋ" | "က".."အ" | "ဣ".."ဧ" | "ဩ".."ဪ" |
// "ၐ".."ၕ" | "Ⴀ".."Ⴥ" | "ა".."ჸ" | "ᄀ".."ᅙ" | "ᅟ".."ᆢ" | "ᆨ".."ᇹ" | "ሀ".."ሆ" | "ለ".."ቆ" | "ቈ" | "ቊ".."ቍ" | "ቐ".."ቖ" |
// "ቘ" | "ቚ".."ቝ" | "በ".."ኆ" | "ኈ" | "ኊ".."ኍ" | "ነ".."ኮ" | "ኰ" | "ኲ".."ኵ" | "ኸ".."ኾ" | "ዀ" | "ዂ".."ዅ" | "ወ".."ዎ" |
// "ዐ".."ዖ" | "ዘ".."ዮ" | "ደ".."ጎ" | "ጐ" | "ጒ".."ጕ" | "ጘ".."ጞ" | "ጠ".."ፆ" | "ፈ".."ፚ" | "Ꭰ".."Ᏼ" | "ᐁ".."ᙬ" | "ᙯ".."ᙶ" |
// "ᚁ".."ᚚ" | "ᚠ".."ᛪ" | "ᛮ".."ᛰ" | "ᜀ".."ᜌ" | "ᜎ".."ᜑ" | "ᜠ".."ᜱ" | "ᝀ".."ᝑ" | "ᝠ".."ᝬ" | "ᝮ".."ᝰ" | "ក".."ឳ" | "ៗ" |
// "៛".."ៜ" | "ᠠ".."ᡷ" | "ᢀ".."ᢨ" | "ᤀ".."ᤜ" | "ᥐ".."ᥭ" | "ᥰ".."ᥴ" | "ᴀ".."ᵫ" | "Ḁ".."ẛ" | "Ạ".."ỹ" | "ἀ".."ἕ" | "Ἐ".."Ἕ"
// | "ἠ".."ὅ" | "Ὀ".."Ὅ" | "ὐ".."ὗ" | "Ὑ" | "Ὓ" | "Ὕ" | "Ὗ".."ώ" | "ᾀ".."ᾴ" | "ᾶ".."ᾼ" | "ι" | "ῂ".."ῄ" | "ῆ".."ῌ" |
// "ῐ".."ΐ" | "ῖ".."Ί" | "ῠ".."Ῥ" | "ῲ".."ῴ" | "ῶ".."ῼ" | "‿".."⁀" | "⁔" | "ⁱ" | "ⁿ" | "₠".."₱" | "ℂ" | "ℇ" | "ℊ".."ℓ" |
// "ℕ" | "ℙ".."ℝ" | "ℤ" | "Ω" | "ℨ" | "K".."ℭ" | "ℯ".."ℱ" | "ℳ".."ℹ" | "ℽ".."ℿ" | "ⅅ".."ⅉ" | "Ⅰ".."Ↄ" | "々".."〇" |
// "〡".."〩" | "〱".."〵" | "〸".."〼" | "ぁ".."ゖ" | "ゝ".."ゟ" | "ァ".."ヿ" | "ㄅ".."ㄬ" | "ㄱ".."ㆎ" | "ㆠ".."ㆷ" | "ㇰ".."ㇿ" | "㐀".."䶵"
// | "一".."龥" | "ꀀ".."ꒌ" | "가".."힣" | "豈".."鶴" | "侮".."頻" | "ff".."st" | "ﬓ".."ﬗ" | "יִ" | "ײַ".."ﬨ" | "שׁ".."זּ" | "טּ".."לּ" |
// "מּ" | "נּ".."סּ" | "ףּ".."פּ" | "צּ".."ﮱ" | "ﯓ".."ﴽ" | "ﵐ".."ﶏ" | "ﶒ".."ﷇ" | "ﷰ".."﷼" | "︳".."︴" | "﹍".."﹏" | "﹩" |
// "ﹰ".."ﹴ" | "ﹶ".."ﻼ" | "$" | "A".."Z" | "_" | "a".."z" | "・".."ᄒ" | "ᅡ".."ᅦ" | "ᅧ".."ᅬ" | "ᅭ".."ᅲ" | "ᅳ".."ᅵ" |
// "¢".."£" | "¥".."₩";
public TerminalRule getIDENTIFIER_STARTRule() {
return gaXtend.getIDENTIFIER_STARTRule();
}
//terminal fragment IDENTIFIER_PART:
// IDENTIFIER_START | IDENTIFIER_PART_IMPL;
public TerminalRule getIDENTIFIER_PARTRule() {
return gaXtend.getIDENTIFIER_PARTRule();
}
//terminal fragment IDENTIFIER_PART_IMPL:
// " ".."\b" | "".."" | "0".."9" | "".."" | "" | "̀".."͗" | "͝".."ͯ" | "҃".."҆" | "֑".."֡" | "֣".."ֹ" | "ֻ".."ֽ" |
// "ֿ" | "ׁ".."ׂ" | "ׄ" | "".."" | "ؐ".."ؕ" | "ً".."٘" | "٠".."٩" | "ٰ" | "ۖ".."" | "۟".."ۤ" | "ۧ".."ۨ" | "۪".."ۭ" |
// "۰".."۹" | "" | "ܑ" | "ܰ".."݊" | "ަ".."ް" | "ँ".."ः" | "़" | "ा".."्" | "॑".."॔" | "ॢ".."ॣ" | "०".."९" | "ঁ".."ঃ" |
// "়" | "া".."ৄ" | "ে".."ৈ" | "ো".."্" | "ৗ" | "ৢ".."ৣ" | "০".."৯" | "ਁ".."ਃ" | "਼" | "ਾ".."ੂ" | "ੇ".."ੈ" | "ੋ".."੍" |
// "੦".."ੱ" | "ઁ".."ઃ" | "઼" | "ા".."ૅ" | "ે".."ૉ" | "ો".."્" | "ૢ".."ૣ" | "૦".."૯" | "ଁ".."ଃ" | "଼" | "ା".."ୃ" |
// "େ".."ୈ" | "ୋ".."୍" | "ୖ".."ୗ" | "୦".."୯" | "ஂ" | "ா".."ூ" | "ெ".."ை" | "ொ".."்" | "ௗ" | "௧".."௯" | "ఁ".."ః" |
// "ా".."ౄ" | "ె".."ై" | "ొ".."్" | "ౕ".."ౖ" | "౦".."౯" | "ಂ".."ಃ" | "಼" | "ಾ".."ೄ" | "ೆ".."ೈ" | "ೊ".."್" | "ೕ".."ೖ" |
// "೦".."೯" | "ം".."ഃ" | "ാ".."ൃ" | "െ".."ൈ" | "ൊ".."്" | "ൗ" | "൦".."൯" | "ං".."ඃ" | "්" | "ා".."ු" | "ූ" | "ෘ".."ෟ" |
// "ෲ".."ෳ" | "ั" | "ิ".."ฺ" | "็".."๎" | "๐".."๙" | "ັ" | "ິ".."ູ" | "ົ".."ຼ" | "່".."ໍ" | "໐".."໙" | "༘".."༙" |
// "༠".."༩" | "༵" | "༷" | "༹" | "༾".."༿" | "ཱ".."྄" | "྆".."྇" | "ྐ".."ྗ" | "ྙ".."ྼ" | "࿆" | "ာ".."ဲ" | "ံ".."္" |
// "၀".."၉" | "ၖ".."ၙ" | "፩".."፱" | "ᜒ".."᜔" | "ᜲ".."᜴" | "ᝒ".."ᝓ" | "ᝲ".."ᝳ" | "឴".."៓" | "៝" | "០".."៩" | "᠋".."᠍" |
// "᠐".."᠙" | "ᢩ" | "ᤠ".."ᤫ" | "ᤰ".."᤻" | "᥆".."᥏" | "".."" | "".."" | "".."" | "".."" | "⃐".."⃜" | "⃡" |
// "⃥".."⃪" | "〪".."〯" | "゙".."゚" | "ﬞ" | "︀".."️" | "︠".."︣" | "" | "0".."9" | "".."";
public TerminalRule getIDENTIFIER_PART_IMPLRule() {
return gaXtend.getIDENTIFIER_PART_IMPLRule();
}
//XAnnotation:
// {XAnnotation} "@" annotationType=[types::JvmAnnotationType|QualifiedName] ("("
// (elementValuePairs+=XAnnotationElementValuePair ("," elementValuePairs+=XAnnotationElementValuePair)* |
// value=XAnnotationElementValueOrCommaList)? ")")?;
public XbaseWithAnnotationsGrammarAccess.XAnnotationElements getXAnnotationAccess() {
return gaXtend.getXAnnotationAccess();
}
public ParserRule getXAnnotationRule() {
return getXAnnotationAccess().getRule();
}
//XAnnotationElementValuePair:
// => (element=[types::JvmOperation|ValidID] "=") value=XAnnotationElementValue;
public XbaseWithAnnotationsGrammarAccess.XAnnotationElementValuePairElements getXAnnotationElementValuePairAccess() {
return gaXtend.getXAnnotationElementValuePairAccess();
}
public ParserRule getXAnnotationElementValuePairRule() {
return getXAnnotationElementValuePairAccess().getRule();
}
//XAnnotationElementValueOrCommaList returns xbase::XExpression:
// => ({xbase::XListLiteral} "#" "[") (elements+=XAnnotationOrExpression ("," elements+=XAnnotationOrExpression)*)? "]" |
// XAnnotationOrExpression ({xbase::XListLiteral.elements+=current} ("," elements+=XAnnotationOrExpression)+)?;
public XbaseWithAnnotationsGrammarAccess.XAnnotationElementValueOrCommaListElements getXAnnotationElementValueOrCommaListAccess() {
return gaXtend.getXAnnotationElementValueOrCommaListAccess();
}
public ParserRule getXAnnotationElementValueOrCommaListRule() {
return getXAnnotationElementValueOrCommaListAccess().getRule();
}
//XAnnotationElementValue returns xbase::XExpression:
// => ({xbase::XListLiteral} "#" "[") (elements+=XAnnotationOrExpression ("," elements+=XAnnotationOrExpression)*)? "]" |
// XAnnotationOrExpression;
public XbaseWithAnnotationsGrammarAccess.XAnnotationElementValueElements getXAnnotationElementValueAccess() {
return gaXtend.getXAnnotationElementValueAccess();
}
public ParserRule getXAnnotationElementValueRule() {
return getXAnnotationElementValueAccess().getRule();
}
//XAnnotationOrExpression returns xbase::XExpression:
// XAnnotation | XExpression;
public XbaseWithAnnotationsGrammarAccess.XAnnotationOrExpressionElements getXAnnotationOrExpressionAccess() {
return gaXtend.getXAnnotationOrExpressionAccess();
}
public ParserRule getXAnnotationOrExpressionRule() {
return getXAnnotationOrExpressionAccess().getRule();
}
//XExpression:
// XAssignment;
public XbaseGrammarAccess.XExpressionElements getXExpressionAccess() {
return gaXtend.getXExpressionAccess();
}
public ParserRule getXExpressionRule() {
return getXExpressionAccess().getRule();
}
//XAssignment returns XExpression:
// {XAssignment} feature=[types::JvmIdentifiableElement|FeatureCallID] OpSingleAssign value=XAssignment | XOrExpression
// (=> ({XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpMultiAssign])
// rightOperand=XAssignment)?;
public XbaseGrammarAccess.XAssignmentElements getXAssignmentAccess() {
return gaXtend.getXAssignmentAccess();
}
public ParserRule getXAssignmentRule() {
return getXAssignmentAccess().getRule();
}
//OpSingleAssign:
// "=";
public XbaseGrammarAccess.OpSingleAssignElements getOpSingleAssignAccess() {
return gaXtend.getOpSingleAssignAccess();
}
public ParserRule getOpSingleAssignRule() {
return getOpSingleAssignAccess().getRule();
}
//OpMultiAssign:
// "+=" | "-=" | "*=" | "/=" | "%=" | "<" "<" "=" | ">" ">"? ">=";
public XbaseGrammarAccess.OpMultiAssignElements getOpMultiAssignAccess() {
return gaXtend.getOpMultiAssignAccess();
}
public ParserRule getOpMultiAssignRule() {
return getOpMultiAssignAccess().getRule();
}
//XOrExpression returns XExpression:
// XAndExpression (=> ({XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpOr])
// rightOperand=XAndExpression)*;
public XbaseGrammarAccess.XOrExpressionElements getXOrExpressionAccess() {
return gaXtend.getXOrExpressionAccess();
}
public ParserRule getXOrExpressionRule() {
return getXOrExpressionAccess().getRule();
}
//OpOr:
// "||";
public XbaseGrammarAccess.OpOrElements getOpOrAccess() {
return gaXtend.getOpOrAccess();
}
public ParserRule getOpOrRule() {
return getOpOrAccess().getRule();
}
//XAndExpression returns XExpression:
// XEqualityExpression (=> ({XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpAnd])
// rightOperand=XEqualityExpression)*;
public XbaseGrammarAccess.XAndExpressionElements getXAndExpressionAccess() {
return gaXtend.getXAndExpressionAccess();
}
public ParserRule getXAndExpressionRule() {
return getXAndExpressionAccess().getRule();
}
//OpAnd:
// "&&";
public XbaseGrammarAccess.OpAndElements getOpAndAccess() {
return gaXtend.getOpAndAccess();
}
public ParserRule getOpAndRule() {
return getOpAndAccess().getRule();
}
//XEqualityExpression returns XExpression:
// XRelationalExpression (=> ({XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpEquality])
// rightOperand=XRelationalExpression)*;
public XbaseGrammarAccess.XEqualityExpressionElements getXEqualityExpressionAccess() {
return gaXtend.getXEqualityExpressionAccess();
}
public ParserRule getXEqualityExpressionRule() {
return getXEqualityExpressionAccess().getRule();
}
//OpEquality:
// "==" | "!=" | "===" | "!==";
public XbaseGrammarAccess.OpEqualityElements getOpEqualityAccess() {
return gaXtend.getOpEqualityAccess();
}
public ParserRule getOpEqualityRule() {
return getOpEqualityAccess().getRule();
}
//OpCompare:
// ">=" | "<" "=" | ">" | "<";
public XbaseGrammarAccess.OpCompareElements getOpCompareAccess() {
return gaXtend.getOpCompareAccess();
}
public ParserRule getOpCompareRule() {
return getOpCompareAccess().getRule();
}
//XOtherOperatorExpression returns XExpression:
// XAdditiveExpression (=> ({XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpOther])
// rightOperand=XAdditiveExpression)*;
public XbaseGrammarAccess.XOtherOperatorExpressionElements getXOtherOperatorExpressionAccess() {
return gaXtend.getXOtherOperatorExpressionAccess();
}
public ParserRule getXOtherOperatorExpressionRule() {
return getXOtherOperatorExpressionAccess().getRule();
}
//OpOther:
// "->" | "..<" | ">" ".." | ".." | "=>" | ">" (=> (">" ">") | ">") | "<" (=> ("<" "<") | "<" | "=>") | "<>" | "?:";
public XbaseGrammarAccess.OpOtherElements getOpOtherAccess() {
return gaXtend.getOpOtherAccess();
}
public ParserRule getOpOtherRule() {
return getOpOtherAccess().getRule();
}
//XAdditiveExpression returns XExpression:
// XMultiplicativeExpression (=> ({XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpAdd])
// rightOperand=XMultiplicativeExpression)*;
public XbaseGrammarAccess.XAdditiveExpressionElements getXAdditiveExpressionAccess() {
return gaXtend.getXAdditiveExpressionAccess();
}
public ParserRule getXAdditiveExpressionRule() {
return getXAdditiveExpressionAccess().getRule();
}
//OpAdd:
// "+" | "-";
public XbaseGrammarAccess.OpAddElements getOpAddAccess() {
return gaXtend.getOpAddAccess();
}
public ParserRule getOpAddRule() {
return getOpAddAccess().getRule();
}
//XMultiplicativeExpression returns XExpression:
// XUnaryOperation (=> ({XBinaryOperation.leftOperand=current} feature=[types::JvmIdentifiableElement|OpMulti])
// rightOperand=XUnaryOperation)*;
public XbaseGrammarAccess.XMultiplicativeExpressionElements getXMultiplicativeExpressionAccess() {
return gaXtend.getXMultiplicativeExpressionAccess();
}
public ParserRule getXMultiplicativeExpressionRule() {
return getXMultiplicativeExpressionAccess().getRule();
}
//OpMulti:
// "*" | "**" | "/" | "%";
public XbaseGrammarAccess.OpMultiElements getOpMultiAccess() {
return gaXtend.getOpMultiAccess();
}
public ParserRule getOpMultiRule() {
return getOpMultiAccess().getRule();
}
//XUnaryOperation returns XExpression:
// {XUnaryOperation} feature=[types::JvmIdentifiableElement|OpUnary] operand=XUnaryOperation | XCastedExpression;
public XbaseGrammarAccess.XUnaryOperationElements getXUnaryOperationAccess() {
return gaXtend.getXUnaryOperationAccess();
}
public ParserRule getXUnaryOperationRule() {
return getXUnaryOperationAccess().getRule();
}
//OpUnary:
// "!" | "-" | "+";
public XbaseGrammarAccess.OpUnaryElements getOpUnaryAccess() {
return gaXtend.getOpUnaryAccess();
}
public ParserRule getOpUnaryRule() {
return getOpUnaryAccess().getRule();
}
//XCastedExpression returns XExpression:
// XPostfixOperation (=> ({XCastedExpression.target=current} "as") type=JvmTypeReference)*;
public XbaseGrammarAccess.XCastedExpressionElements getXCastedExpressionAccess() {
return gaXtend.getXCastedExpressionAccess();
}
public ParserRule getXCastedExpressionRule() {
return getXCastedExpressionAccess().getRule();
}
//XPostfixOperation returns XExpression:
// XMemberFeatureCall => ({XPostfixOperation.operand=current} feature=[types::JvmIdentifiableElement|OpPostfix])?;
public XbaseGrammarAccess.XPostfixOperationElements getXPostfixOperationAccess() {
return gaXtend.getXPostfixOperationAccess();
}
public ParserRule getXPostfixOperationRule() {
return getXPostfixOperationAccess().getRule();
}
//OpPostfix:
// "++" | "--";
public XbaseGrammarAccess.OpPostfixElements getOpPostfixAccess() {
return gaXtend.getOpPostfixAccess();
}
public ParserRule getOpPostfixRule() {
return getOpPostfixAccess().getRule();
}
//XMemberFeatureCall returns XExpression:
// XPrimaryExpression (=> ({XAssignment.assignable=current} ("." | explicitStatic?="::")
// feature=[types::JvmIdentifiableElement|FeatureCallID] OpSingleAssign) value=XAssignment | =>
// ({XMemberFeatureCall.memberCallTarget=current} ("." | nullSafe?="?." | explicitStatic?="::")) ("<"
// typeArguments+=JvmArgumentTypeReference ("," typeArguments+=JvmArgumentTypeReference)* ">")?
// feature=[types::JvmIdentifiableElement|IdOrSuper] (=> explicitOperationCall?="(" (memberCallArguments+=XShortClosure |
// memberCallArguments+=XExpression ("," memberCallArguments+=XExpression)*)? ")")? memberCallArguments+=XClosure?)*;
public XbaseGrammarAccess.XMemberFeatureCallElements getXMemberFeatureCallAccess() {
return gaXtend.getXMemberFeatureCallAccess();
}
public ParserRule getXMemberFeatureCallRule() {
return getXMemberFeatureCallAccess().getRule();
}
//XLiteral returns XExpression:
// XCollectionLiteral | XClosure | XBooleanLiteral | XNumberLiteral | XNullLiteral | XStringLiteral | XTypeLiteral;
public XbaseGrammarAccess.XLiteralElements getXLiteralAccess() {
return gaXtend.getXLiteralAccess();
}
public ParserRule getXLiteralRule() {
return getXLiteralAccess().getRule();
}
//XCollectionLiteral:
// XSetLiteral | XListLiteral;
public XbaseGrammarAccess.XCollectionLiteralElements getXCollectionLiteralAccess() {
return gaXtend.getXCollectionLiteralAccess();
}
public ParserRule getXCollectionLiteralRule() {
return getXCollectionLiteralAccess().getRule();
}
//XSetLiteral:
// {XSetLiteral} "#" "{" (elements+=XExpression ("," elements+=XExpression)*)? "}";
public XbaseGrammarAccess.XSetLiteralElements getXSetLiteralAccess() {
return gaXtend.getXSetLiteralAccess();
}
public ParserRule getXSetLiteralRule() {
return getXSetLiteralAccess().getRule();
}
//XListLiteral:
// {XListLiteral} "#" "[" (elements+=XExpression ("," elements+=XExpression)*)? "]";
public XbaseGrammarAccess.XListLiteralElements getXListLiteralAccess() {
return gaXtend.getXListLiteralAccess();
}
public ParserRule getXListLiteralRule() {
return getXListLiteralAccess().getRule();
}
//XClosure returns XExpression:
// => ({XClosure} "[") => ((declaredFormalParameters+=JvmFormalParameter (","
// declaredFormalParameters+=JvmFormalParameter)*)? explicitSyntax?="|")? expression=XExpressionInClosure "]";
public XbaseGrammarAccess.XClosureElements getXClosureAccess() {
return gaXtend.getXClosureAccess();
}
public ParserRule getXClosureRule() {
return getXClosureAccess().getRule();
}
//XExpressionInClosure returns XExpression:
// {XBlockExpression} (expressions+=XExpressionOrVarDeclaration ";"?)*;
public XbaseGrammarAccess.XExpressionInClosureElements getXExpressionInClosureAccess() {
return gaXtend.getXExpressionInClosureAccess();
}
public ParserRule getXExpressionInClosureRule() {
return getXExpressionInClosureAccess().getRule();
}
//XShortClosure returns XExpression:
// => ({XClosure} (declaredFormalParameters+=JvmFormalParameter ("," declaredFormalParameters+=JvmFormalParameter)*)?
// explicitSyntax?="|") expression=XExpression;
public XbaseGrammarAccess.XShortClosureElements getXShortClosureAccess() {
return gaXtend.getXShortClosureAccess();
}
public ParserRule getXShortClosureRule() {
return getXShortClosureAccess().getRule();
}
//XParenthesizedExpression returns XExpression:
// "(" XExpression ")";
public XbaseGrammarAccess.XParenthesizedExpressionElements getXParenthesizedExpressionAccess() {
return gaXtend.getXParenthesizedExpressionAccess();
}
public ParserRule getXParenthesizedExpressionRule() {
return getXParenthesizedExpressionAccess().getRule();
}
//XIfExpression returns XExpression:
// {XIfExpression} "if" "(" if=XExpression ")" then=XExpression ("else" else=XExpression)?;
public XbaseGrammarAccess.XIfExpressionElements getXIfExpressionAccess() {
return gaXtend.getXIfExpressionAccess();
}
public ParserRule getXIfExpressionRule() {
return getXIfExpressionAccess().getRule();
}
//XCasePart:
// {XCasePart} typeGuard=JvmTypeReference? ("case" case=XExpression)? (":" then=XExpression | ",");
public XbaseGrammarAccess.XCasePartElements getXCasePartAccess() {
return gaXtend.getXCasePartAccess();
}
public ParserRule getXCasePartRule() {
return getXCasePartAccess().getRule();
}
//XForLoopExpression returns XExpression:
// => ({XForLoopExpression} "for" "(" declaredParam=JvmFormalParameter ":") forExpression=XExpression ")"
// eachExpression=XExpression;
public XbaseGrammarAccess.XForLoopExpressionElements getXForLoopExpressionAccess() {
return gaXtend.getXForLoopExpressionAccess();
}
public ParserRule getXForLoopExpressionRule() {
return getXForLoopExpressionAccess().getRule();
}
//XBasicForLoopExpression returns XExpression:
// {XBasicForLoopExpression} "for" "(" (initExpressions+=XExpressionOrVarDeclaration (","
// initExpressions+=XExpressionOrVarDeclaration)*)? ";" expression=XExpression? ";" (updateExpressions+=XExpression (","
// updateExpressions+=XExpression)*)? ")" eachExpression=XExpression;
public XbaseGrammarAccess.XBasicForLoopExpressionElements getXBasicForLoopExpressionAccess() {
return gaXtend.getXBasicForLoopExpressionAccess();
}
public ParserRule getXBasicForLoopExpressionRule() {
return getXBasicForLoopExpressionAccess().getRule();
}
//XWhileExpression returns XExpression:
// {XWhileExpression} "while" "(" predicate=XExpression ")" body=XExpression;
public XbaseGrammarAccess.XWhileExpressionElements getXWhileExpressionAccess() {
return gaXtend.getXWhileExpressionAccess();
}
public ParserRule getXWhileExpressionRule() {
return getXWhileExpressionAccess().getRule();
}
//XDoWhileExpression returns XExpression:
// {XDoWhileExpression} "do" body=XExpression "while" "(" predicate=XExpression ")";
public XbaseGrammarAccess.XDoWhileExpressionElements getXDoWhileExpressionAccess() {
return gaXtend.getXDoWhileExpressionAccess();
}
public ParserRule getXDoWhileExpressionRule() {
return getXDoWhileExpressionAccess().getRule();
}
//XBlockExpression returns XExpression:
// {XBlockExpression} "{" (expressions+=XExpressionOrVarDeclaration ";"?)* "}";
public XbaseGrammarAccess.XBlockExpressionElements getXBlockExpressionAccess() {
return gaXtend.getXBlockExpressionAccess();
}
public ParserRule getXBlockExpressionRule() {
return getXBlockExpressionAccess().getRule();
}
//XExpressionOrVarDeclaration returns XExpression:
// XVariableDeclaration | XExpression;
public XbaseGrammarAccess.XExpressionOrVarDeclarationElements getXExpressionOrVarDeclarationAccess() {
return gaXtend.getXExpressionOrVarDeclarationAccess();
}
public ParserRule getXExpressionOrVarDeclarationRule() {
return getXExpressionOrVarDeclarationAccess().getRule();
}
//XFeatureCall returns XExpression:
// {XFeatureCall} ("<" typeArguments+=JvmArgumentTypeReference ("," typeArguments+=JvmArgumentTypeReference)* ">")?
// feature=[types::JvmIdentifiableElement|IdOrSuper] (=> explicitOperationCall?="(" (featureCallArguments+=XShortClosure
// | featureCallArguments+=XExpression ("," featureCallArguments+=XExpression)*)? ")")? featureCallArguments+=XClosure?;
public XbaseGrammarAccess.XFeatureCallElements getXFeatureCallAccess() {
return gaXtend.getXFeatureCallAccess();
}
public ParserRule getXFeatureCallRule() {
return getXFeatureCallAccess().getRule();
}
//IdOrSuper:
// FeatureCallID | "super";
public XbaseGrammarAccess.IdOrSuperElements getIdOrSuperAccess() {
return gaXtend.getIdOrSuperAccess();
}
public ParserRule getIdOrSuperRule() {
return getIdOrSuperAccess().getRule();
}
//XBooleanLiteral returns XExpression:
// {XBooleanLiteral} ("false" | isTrue?="true");
public XbaseGrammarAccess.XBooleanLiteralElements getXBooleanLiteralAccess() {
return gaXtend.getXBooleanLiteralAccess();
}
public ParserRule getXBooleanLiteralRule() {
return getXBooleanLiteralAccess().getRule();
}
//XNullLiteral returns XExpression:
// {XNullLiteral} "null";
public XbaseGrammarAccess.XNullLiteralElements getXNullLiteralAccess() {
return gaXtend.getXNullLiteralAccess();
}
public ParserRule getXNullLiteralRule() {
return getXNullLiteralAccess().getRule();
}
//XNumberLiteral returns XExpression:
// {XNumberLiteral} value=Number;
public XbaseGrammarAccess.XNumberLiteralElements getXNumberLiteralAccess() {
return gaXtend.getXNumberLiteralAccess();
}
public ParserRule getXNumberLiteralRule() {
return getXNumberLiteralAccess().getRule();
}
//XTypeLiteral returns XExpression:
// {XTypeLiteral} "typeof" "(" type=[types::JvmType|QualifiedName] arrayDimensions+=ArrayBrackets* ")";
public XbaseGrammarAccess.XTypeLiteralElements getXTypeLiteralAccess() {
return gaXtend.getXTypeLiteralAccess();
}
public ParserRule getXTypeLiteralRule() {
return getXTypeLiteralAccess().getRule();
}
//XThrowExpression returns XExpression:
// {XThrowExpression} "throw" expression=XExpression;
public XbaseGrammarAccess.XThrowExpressionElements getXThrowExpressionAccess() {
return gaXtend.getXThrowExpressionAccess();
}
public ParserRule getXThrowExpressionRule() {
return getXThrowExpressionAccess().getRule();
}
//XReturnExpression returns XExpression:
// {XReturnExpression} "return" -> expression=XExpression?;
public XbaseGrammarAccess.XReturnExpressionElements getXReturnExpressionAccess() {
return gaXtend.getXReturnExpressionAccess();
}
public ParserRule getXReturnExpressionRule() {
return getXReturnExpressionAccess().getRule();
}
//XTryCatchFinallyExpression returns XExpression:
// {XTryCatchFinallyExpression} "try" expression=XExpression (catchClauses+=XCatchClause+ ("finally"
// finallyExpression=XExpression)? | "finally" finallyExpression=XExpression);
public XbaseGrammarAccess.XTryCatchFinallyExpressionElements getXTryCatchFinallyExpressionAccess() {
return gaXtend.getXTryCatchFinallyExpressionAccess();
}
public ParserRule getXTryCatchFinallyExpressionRule() {
return getXTryCatchFinallyExpressionAccess().getRule();
}
//XSynchronizedExpression returns XExpression:
// => ({XSynchronizedExpression} "synchronized" "(") param=XExpression ")" expression=XExpression;
public XbaseGrammarAccess.XSynchronizedExpressionElements getXSynchronizedExpressionAccess() {
return gaXtend.getXSynchronizedExpressionAccess();
}
public ParserRule getXSynchronizedExpressionRule() {
return getXSynchronizedExpressionAccess().getRule();
}
//XCatchClause:
// "catch" "(" declaredParam=FullJvmFormalParameter ")" expression=XExpression;
public XbaseGrammarAccess.XCatchClauseElements getXCatchClauseAccess() {
return gaXtend.getXCatchClauseAccess();
}
public ParserRule getXCatchClauseRule() {
return getXCatchClauseAccess().getRule();
}
//QualifiedName:
// ValidID ("." ValidID)*;
public XbaseGrammarAccess.QualifiedNameElements getQualifiedNameAccess() {
return gaXtend.getQualifiedNameAccess();
}
public ParserRule getQualifiedNameRule() {
return getQualifiedNameAccess().getRule();
}
//Number hidden():
// HEX | (INT | DECIMAL) ("." (INT | DECIMAL))?;
public XbaseGrammarAccess.NumberElements getNumberAccess() {
return gaXtend.getNumberAccess();
}
public ParserRule getNumberRule() {
return getNumberAccess().getRule();
}
/// **
// * Dummy rule, for "better" downwards compatibility, since GrammarAccess generates non-static inner classes,
// * which makes downstream grammars break on classloading, when a rule is removed.
// * / StaticQualifier:
// (ValidID "::")+;
public XbaseGrammarAccess.StaticQualifierElements getStaticQualifierAccess() {
return gaXtend.getStaticQualifierAccess();
}
public ParserRule getStaticQualifierRule() {
return getStaticQualifierAccess().getRule();
}
//terminal HEX:
// ("0x" | "0X") ("0".."9" | "a".."f" | "A".."F" | "_")+ ("#" (("b" | "B") ("i" | "I") | ("l" | "L")))?;
public TerminalRule getHEXRule() {
return gaXtend.getHEXRule();
}
//terminal INT returns ecore::EInt:
// "0".."9" ("0".."9" | "_")*;
public TerminalRule getINTRule() {
return gaXtend.getINTRule();
}
//terminal DECIMAL:
// INT (("e" | "E") ("+" | "-")? INT)? (("b" | "B") ("i" | "I" | "d" | "D") | ("l" | "L" | "d" | "D" | "f" | "F"))?;
public TerminalRule getDECIMALRule() {
return gaXtend.getDECIMALRule();
}
//JvmTypeReference:
// JvmParameterizedTypeReference => ({JvmGenericArrayTypeReference.componentType=current} ArrayBrackets)* |
// XFunctionTypeRef;
public XtypeGrammarAccess.JvmTypeReferenceElements getJvmTypeReferenceAccess() {
return gaXtend.getJvmTypeReferenceAccess();
}
public ParserRule getJvmTypeReferenceRule() {
return getJvmTypeReferenceAccess().getRule();
}
//ArrayBrackets:
// "[" "]";
public XtypeGrammarAccess.ArrayBracketsElements getArrayBracketsAccess() {
return gaXtend.getArrayBracketsAccess();
}
public ParserRule getArrayBracketsRule() {
return getArrayBracketsAccess().getRule();
}
//XFunctionTypeRef:
// ("(" (paramTypes+=JvmTypeReference ("," paramTypes+=JvmTypeReference)*)? ")")? "=>" returnType=JvmTypeReference;
public XtypeGrammarAccess.XFunctionTypeRefElements getXFunctionTypeRefAccess() {
return gaXtend.getXFunctionTypeRefAccess();
}
public ParserRule getXFunctionTypeRefRule() {
return getXFunctionTypeRefAccess().getRule();
}
//JvmParameterizedTypeReference:
// type=[JvmType|QualifiedName] ("<" arguments+=JvmArgumentTypeReference ("," arguments+=JvmArgumentTypeReference)*
// ">")?;
public XtypeGrammarAccess.JvmParameterizedTypeReferenceElements getJvmParameterizedTypeReferenceAccess() {
return gaXtend.getJvmParameterizedTypeReferenceAccess();
}
public ParserRule getJvmParameterizedTypeReferenceRule() {
return getJvmParameterizedTypeReferenceAccess().getRule();
}
//JvmArgumentTypeReference returns JvmTypeReference:
// JvmTypeReference | JvmWildcardTypeReference;
public XtypeGrammarAccess.JvmArgumentTypeReferenceElements getJvmArgumentTypeReferenceAccess() {
return gaXtend.getJvmArgumentTypeReferenceAccess();
}
public ParserRule getJvmArgumentTypeReferenceRule() {
return getJvmArgumentTypeReferenceAccess().getRule();
}
//JvmWildcardTypeReference:
// {JvmWildcardTypeReference} "?" (constraints+=JvmUpperBound | constraints+=JvmLowerBound)?;
public XtypeGrammarAccess.JvmWildcardTypeReferenceElements getJvmWildcardTypeReferenceAccess() {
return gaXtend.getJvmWildcardTypeReferenceAccess();
}
public ParserRule getJvmWildcardTypeReferenceRule() {
return getJvmWildcardTypeReferenceAccess().getRule();
}
//JvmUpperBound:
// "extends" typeReference=JvmTypeReference;
public XtypeGrammarAccess.JvmUpperBoundElements getJvmUpperBoundAccess() {
return gaXtend.getJvmUpperBoundAccess();
}
public ParserRule getJvmUpperBoundRule() {
return getJvmUpperBoundAccess().getRule();
}
//JvmUpperBoundAnded returns JvmUpperBound:
// "&" typeReference=JvmTypeReference;
public XtypeGrammarAccess.JvmUpperBoundAndedElements getJvmUpperBoundAndedAccess() {
return gaXtend.getJvmUpperBoundAndedAccess();
}
public ParserRule getJvmUpperBoundAndedRule() {
return getJvmUpperBoundAndedAccess().getRule();
}
//JvmLowerBound:
// "super" typeReference=JvmTypeReference;
public XtypeGrammarAccess.JvmLowerBoundElements getJvmLowerBoundAccess() {
return gaXtend.getJvmLowerBoundAccess();
}
public ParserRule getJvmLowerBoundRule() {
return getJvmLowerBoundAccess().getRule();
}
//JvmTypeParameter:
// name=ValidID (constraints+=JvmUpperBound constraints+=JvmUpperBoundAnded*)?;
public XtypeGrammarAccess.JvmTypeParameterElements getJvmTypeParameterAccess() {
return gaXtend.getJvmTypeParameterAccess();
}
public ParserRule getJvmTypeParameterRule() {
return getJvmTypeParameterAccess().getRule();
}
//QualifiedNameWithWildcard:
// QualifiedName "." "*";
public XtypeGrammarAccess.QualifiedNameWithWildcardElements getQualifiedNameWithWildcardAccess() {
return gaXtend.getQualifiedNameWithWildcardAccess();
}
public ParserRule getQualifiedNameWithWildcardRule() {
return getQualifiedNameWithWildcardAccess().getRule();
}
//XImportSection:
// importDeclarations+=XImportDeclaration+;
public XtypeGrammarAccess.XImportSectionElements getXImportSectionAccess() {
return gaXtend.getXImportSectionAccess();
}
public ParserRule getXImportSectionRule() {
return getXImportSectionAccess().getRule();
}
//XImportDeclaration:
// "import" (static?="static" extension?="extension"? importedType=[JvmDeclaredType|QualifiedNameInStaticImport]
// (wildcard?="*" | memberName=ValidID) | importedType=[JvmDeclaredType|QualifiedName] |
// importedNamespace=QualifiedNameWithWildcard) ";"?;
public XtypeGrammarAccess.XImportDeclarationElements getXImportDeclarationAccess() {
return gaXtend.getXImportDeclarationAccess();
}
public ParserRule getXImportDeclarationRule() {
return getXImportDeclarationAccess().getRule();
}
//QualifiedNameInStaticImport:
// (ValidID ".")+;
public XtypeGrammarAccess.QualifiedNameInStaticImportElements getQualifiedNameInStaticImportAccess() {
return gaXtend.getQualifiedNameInStaticImportAccess();
}
public ParserRule getQualifiedNameInStaticImportRule() {
return getQualifiedNameInStaticImportAccess().getRule();
}
//terminal STRING:
// "\"" ("\\" ("b" | "t" | "n" | "f" | "r" | "u" | "\"" | "\'" | "\\") | !("\\" | "\""))* "\"" | "\'" ("\\" ("b" | "t" |
// "n" | "f" | "r" | "u" | "\"" | "\'" | "\\") | !("\\" | "\'"))* "\'";
public TerminalRule getSTRINGRule() {
return gaXtend.getSTRINGRule();
}
//terminal SL_COMMENT:
// "//" !("\n" | "\r")* ("\r"? "\n")?;
public TerminalRule getSL_COMMENTRule() {
return gaXtend.getSL_COMMENTRule();
}
//terminal WS:
// (" " | "\t" | "\r" | "\n")+;
public TerminalRule getWSRule() {
return gaXtend.getWSRule();
}
//terminal ANY_OTHER:
// .;
public TerminalRule getANY_OTHERRule() {
return gaXtend.getANY_OTHERRule();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy