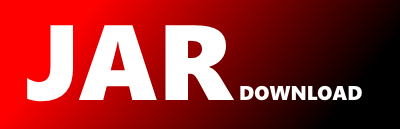
org.jnario.util.JnarioAdapterFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/**
*
*
*
* $Id$
*/
package org.jnario.util;
import org.eclipse.emf.common.notify.Adapter;
import org.eclipse.emf.common.notify.Notifier;
import org.eclipse.emf.common.notify.impl.AdapterFactoryImpl;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtend.core.xtend.XtendAnnotationTarget;
import org.eclipse.xtend.core.xtend.XtendClass;
import org.eclipse.xtend.core.xtend.XtendFunction;
import org.eclipse.xtend.core.xtend.XtendField;
import org.eclipse.xtend.core.xtend.XtendMember;
import org.eclipse.xtend.core.xtend.XtendTypeDeclaration;
import org.eclipse.xtext.xbase.XAbstractFeatureCall;
import org.eclipse.xtext.xbase.XBinaryOperation;
import org.eclipse.xtext.xbase.XExpression;
import org.eclipse.xtext.xbase.XTypeLiteral;
import org.jnario.*;
import org.jnario.Assertion;
import org.jnario.ExampleColumn;
import org.jnario.ExampleRow;
import org.jnario.ExampleTable;
import org.jnario.Executable;
import org.jnario.JnarioPackage;
import org.jnario.MockLiteral;
import org.jnario.Should;
import org.jnario.ShouldThrow;
import org.jnario.Specification;
/**
*
* The Adapter Factory for the model.
* It provides an adapter createXXX
method for each class of the model.
*
* @see org.jnario.JnarioPackage
* @generated
*/
public class JnarioAdapterFactory extends AdapterFactoryImpl {
/**
* The cached model package.
*
*
* @generated
*/
protected static JnarioPackage modelPackage;
/**
* Creates an instance of the adapter factory.
*
*
* @generated
*/
public JnarioAdapterFactory() {
if (modelPackage == null) {
modelPackage = JnarioPackage.eINSTANCE;
}
}
/**
* Returns whether this factory is applicable for the type of the object.
*
* This implementation returns true
if the object is either the model's package or is an instance object of the model.
*
* @return whether this factory is applicable for the type of the object.
* @generated
*/
@Override
public boolean isFactoryForType(Object object) {
if (object == modelPackage) {
return true;
}
if (object instanceof EObject) {
return ((EObject)object).eClass().getEPackage() == modelPackage;
}
return false;
}
/**
* The switch that delegates to the createXXX
methods.
*
*
* @generated
*/
protected JnarioSwitch modelSwitch =
new JnarioSwitch() {
@Override
public Adapter caseExampleTable(ExampleTable object) {
return createExampleTableAdapter();
}
@Override
public Adapter caseExampleRow(ExampleRow object) {
return createExampleRowAdapter();
}
@Override
public Adapter caseAssertion(Assertion object) {
return createAssertionAdapter();
}
@Override
public Adapter caseExampleColumn(ExampleColumn object) {
return createExampleColumnAdapter();
}
@Override
public Adapter caseShould(Should object) {
return createShouldAdapter();
}
@Override
public Adapter caseShouldThrow(ShouldThrow object) {
return createShouldThrowAdapter();
}
@Override
public Adapter caseSpecification(Specification object) {
return createSpecificationAdapter();
}
@Override
public Adapter caseExecutable(Executable object) {
return createExecutableAdapter();
}
@Override
public Adapter caseMockLiteral(MockLiteral object) {
return createMockLiteralAdapter();
}
@Override
public Adapter caseExampleCell(ExampleCell object) {
return createExampleCellAdapter();
}
@Override
public Adapter caseXtendAnnotationTarget(XtendAnnotationTarget object) {
return createXtendAnnotationTargetAdapter();
}
@Override
public Adapter caseXtendMember(XtendMember object) {
return createXtendMemberAdapter();
}
@Override
public Adapter caseXExpression(XExpression object) {
return createXExpressionAdapter();
}
@Override
public Adapter caseXAbstractFeatureCall(XAbstractFeatureCall object) {
return createXAbstractFeatureCallAdapter();
}
@Override
public Adapter caseXBinaryOperation(XBinaryOperation object) {
return createXBinaryOperationAdapter();
}
@Override
public Adapter caseXtendTypeDeclaration(XtendTypeDeclaration object) {
return createXtendTypeDeclarationAdapter();
}
@Override
public Adapter caseXtendClass(XtendClass object) {
return createXtendClassAdapter();
}
@Override
public Adapter caseXTypeLiteral(XTypeLiteral object) {
return createXTypeLiteralAdapter();
}
@Override
public Adapter caseXtendFunction(XtendFunction object) {
return createXtendFunctionAdapter();
}
@Override
public Adapter defaultCase(EObject object) {
return createEObjectAdapter();
}
};
/**
* Creates an adapter for the target
.
*
*
* @param target the object to adapt.
* @return the adapter for the target
.
* @generated
*/
@Override
public Adapter createAdapter(Notifier target) {
return modelSwitch.doSwitch((EObject)target);
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.ExampleTable Example Table}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.ExampleTable
* @generated
*/
public Adapter createExampleTableAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.ExampleRow Example Row}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.ExampleRow
* @generated
*/
public Adapter createExampleRowAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.Assertion Assertion}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.Assertion
* @generated
*/
public Adapter createAssertionAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.ExampleColumn Example Column}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.ExampleColumn
* @generated
*/
public Adapter createExampleColumnAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.Should Should}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.Should
* @generated
*/
public Adapter createShouldAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.ShouldThrow Should Throw}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.ShouldThrow
* @generated
*/
public Adapter createShouldThrowAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.Specification Specification}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.Specification
* @generated
*/
public Adapter createSpecificationAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.Executable Executable}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.Executable
* @generated
*/
public Adapter createExecutableAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.MockLiteral Mock Literal}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.MockLiteral
* @generated
*/
public Adapter createMockLiteralAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.jnario.ExampleCell Example Cell}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.jnario.ExampleCell
* @generated
*/
public Adapter createExampleCellAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtend.core.xtend.XtendAnnotationTarget Annotation Target}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtend.core.xtend.XtendAnnotationTarget
* @generated
*/
public Adapter createXtendAnnotationTargetAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtend.core.xtend.XtendMember Member}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtend.core.xtend.XtendMember
* @generated
*/
public Adapter createXtendMemberAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.xbase.XExpression XExpression}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.xbase.XExpression
* @generated
*/
public Adapter createXExpressionAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall XAbstract Feature Call}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall
* @generated
*/
public Adapter createXAbstractFeatureCallAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.xbase.XBinaryOperation XBinary Operation}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.xbase.XBinaryOperation
* @generated
*/
public Adapter createXBinaryOperationAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtend.core.xtend.XtendTypeDeclaration Type Declaration}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtend.core.xtend.XtendTypeDeclaration
* @generated
*/
public Adapter createXtendTypeDeclarationAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtend.core.xtend.XtendClass Class}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtend.core.xtend.XtendClass
* @generated
*/
public Adapter createXtendClassAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.xbase.XTypeLiteral XType Literal}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.xbase.XTypeLiteral
* @generated
*/
public Adapter createXTypeLiteralAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtend.core.xtend.XtendFunction Function}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtend.core.xtend.XtendFunction
* @generated
*/
public Adapter createXtendFunctionAdapter() {
return null;
}
/**
* Creates a new adapter for the default case.
*
* This default implementation returns null.
*
* @return the new adapter.
* @generated
*/
public Adapter createEObjectAdapter() {
return null;
}
} //JnarioAdapterFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy