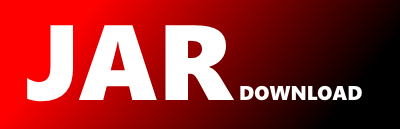
org.jnario.util.JnarioSwitch Maven / Gradle / Ivy
/**
*
*
*
* $Id$
*/
package org.jnario.util;
import java.util.List;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtend.core.xtend.XtendAnnotationTarget;
import org.eclipse.xtend.core.xtend.XtendClass;
import org.eclipse.xtend.core.xtend.XtendFunction;
import org.eclipse.xtend.core.xtend.XtendField;
import org.eclipse.xtend.core.xtend.XtendMember;
import org.eclipse.xtend.core.xtend.XtendTypeDeclaration;
import org.eclipse.xtext.xbase.XAbstractFeatureCall;
import org.eclipse.xtext.xbase.XBinaryOperation;
import org.eclipse.xtext.xbase.XExpression;
import org.eclipse.xtext.xbase.XTypeLiteral;
import org.jnario.*;
import org.jnario.Assertion;
import org.jnario.ExampleColumn;
import org.jnario.ExampleRow;
import org.jnario.ExampleTable;
import org.jnario.Executable;
import org.jnario.JnarioPackage;
import org.jnario.MockLiteral;
import org.jnario.Should;
import org.jnario.ShouldThrow;
import org.jnario.Specification;
/**
*
* The Switch for the model's inheritance hierarchy.
* It supports the call {@link #doSwitch(EObject) doSwitch(object)}
* to invoke the caseXXX
method for each class of the model,
* starting with the actual class of the object
* and proceeding up the inheritance hierarchy
* until a non-null result is returned,
* which is the result of the switch.
*
* @see org.jnario.JnarioPackage
* @generated
*/
public class JnarioSwitch {
/**
* The cached model package
*
*
* @generated
*/
protected static JnarioPackage modelPackage;
/**
* Creates an instance of the switch.
*
*
* @generated
*/
public JnarioSwitch() {
if (modelPackage == null) {
modelPackage = JnarioPackage.eINSTANCE;
}
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
public T doSwitch(EObject theEObject) {
return doSwitch(theEObject.eClass(), theEObject);
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
protected T doSwitch(EClass theEClass, EObject theEObject) {
if (theEClass.eContainer() == modelPackage) {
return doSwitch(theEClass.getClassifierID(), theEObject);
}
else {
List eSuperTypes = theEClass.getESuperTypes();
return
eSuperTypes.isEmpty() ?
defaultCase(theEObject) :
doSwitch(eSuperTypes.get(0), theEObject);
}
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
protected T doSwitch(int classifierID, EObject theEObject) {
switch (classifierID) {
case JnarioPackage.EXAMPLE_TABLE: {
ExampleTable exampleTable = (ExampleTable)theEObject;
T result = caseExampleTable(exampleTable);
if (result == null) result = caseXtendMember(exampleTable);
if (result == null) result = caseXExpression(exampleTable);
if (result == null) result = caseXtendAnnotationTarget(exampleTable);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.EXAMPLE_ROW: {
ExampleRow exampleRow = (ExampleRow)theEObject;
T result = caseExampleRow(exampleRow);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.ASSERTION: {
Assertion assertion = (Assertion)theEObject;
T result = caseAssertion(assertion);
if (result == null) result = caseXExpression(assertion);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.EXAMPLE_COLUMN: {
ExampleColumn exampleColumn = (ExampleColumn)theEObject;
T result = caseExampleColumn(exampleColumn);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.SHOULD: {
Should should = (Should)theEObject;
T result = caseShould(should);
if (result == null) result = caseXBinaryOperation(should);
if (result == null) result = caseXAbstractFeatureCall(should);
if (result == null) result = caseXExpression(should);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.SHOULD_THROW: {
ShouldThrow shouldThrow = (ShouldThrow)theEObject;
T result = caseShouldThrow(shouldThrow);
if (result == null) result = caseXExpression(shouldThrow);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.SPECIFICATION: {
Specification specification = (Specification)theEObject;
T result = caseSpecification(specification);
if (result == null) result = caseXtendClass(specification);
if (result == null) result = caseExecutable(specification);
if (result == null) result = caseXtendTypeDeclaration(specification);
if (result == null) result = caseXtendMember(specification);
if (result == null) result = caseXtendAnnotationTarget(specification);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.EXECUTABLE: {
Executable executable = (Executable)theEObject;
T result = caseExecutable(executable);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.MOCK_LITERAL: {
MockLiteral mockLiteral = (MockLiteral)theEObject;
T result = caseMockLiteral(mockLiteral);
if (result == null) result = caseXTypeLiteral(mockLiteral);
if (result == null) result = caseXExpression(mockLiteral);
if (result == null) result = defaultCase(theEObject);
return result;
}
case JnarioPackage.EXAMPLE_CELL: {
ExampleCell exampleCell = (ExampleCell)theEObject;
T result = caseExampleCell(exampleCell);
if (result == null) result = caseXtendFunction(exampleCell);
if (result == null) result = caseXtendMember(exampleCell);
if (result == null) result = caseXtendAnnotationTarget(exampleCell);
if (result == null) result = defaultCase(theEObject);
return result;
}
default: return defaultCase(theEObject);
}
}
/**
* Returns the result of interpreting the object as an instance of 'Example Table'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Example Table'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseExampleTable(ExampleTable object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Example Row'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Example Row'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseExampleRow(ExampleRow object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Assertion'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Assertion'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssertion(Assertion object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Example Column'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Example Column'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseExampleColumn(ExampleColumn object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Should'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Should'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseShould(Should object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Should Throw'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Should Throw'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseShouldThrow(ShouldThrow object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Specification'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Specification'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseSpecification(Specification object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Executable'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Executable'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseExecutable(Executable object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Mock Literal'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Mock Literal'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseMockLiteral(MockLiteral object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Example Cell'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Example Cell'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseExampleCell(ExampleCell object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Annotation Target'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Annotation Target'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXtendAnnotationTarget(XtendAnnotationTarget object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Member'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Member'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXtendMember(XtendMember object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'XExpression'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'XExpression'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXExpression(XExpression object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'XAbstract Feature Call'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'XAbstract Feature Call'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXAbstractFeatureCall(XAbstractFeatureCall object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'XBinary Operation'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'XBinary Operation'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXBinaryOperation(XBinaryOperation object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Type Declaration'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Type Declaration'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXtendTypeDeclaration(XtendTypeDeclaration object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Class'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Class'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXtendClass(XtendClass object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'XType Literal'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'XType Literal'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXTypeLiteral(XTypeLiteral object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Function'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Function'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseXtendFunction(XtendFunction object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EObject'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch, but this is the last case anyway.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'EObject'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject)
* @generated
*/
public T defaultCase(EObject object) {
return null;
}
} //JnarioSwitch
© 2015 - 2025 Weber Informatics LLC | Privacy Policy