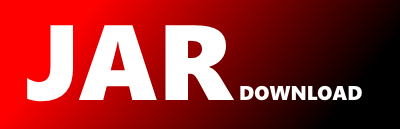
org.jnosql.artemis.column.ColumnTemplateAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artemis-column Show documentation
Show all versions of artemis-column Show documentation
Eclipse JNoSQL Mapping, Artemis API, to column NoSQL databases
/*
* Copyright (c) 2017 Otávio Santana and others
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Apache License v2.0 is available at http://www.opensource.org/licenses/apache2.0.php.
*
* You may elect to redistribute this code under either of these licenses.
*
* Contributors:
*
* Otavio Santana
*/
package org.jnosql.artemis.column;
import org.jnosql.artemis.PreparedStatementAsync;
import org.jnosql.diana.api.NonUniqueResultException;
import org.jnosql.diana.api.column.ColumnDeleteQuery;
import org.jnosql.diana.api.column.ColumnQuery;
import java.time.Duration;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.StreamSupport;
import static java.util.Objects.requireNonNull;
/**
* This interface that represents the common operation between an entity
* and {@link org.jnosql.diana.api.column.ColumnEntity} to do async operations
*
* @see org.jnosql.diana.api.column.ColumnFamilyManagerAsync
*/
public interface ColumnTemplateAsync {
/**
* Inserts an entity asynchronously
*
* @param entity entity to be saved
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when entity is null
*/
void insert(T entity);
/**
* Inserts an entity asynchronously with time to live
*
* @param entity entity to be saved
* @param ttl the time to live
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when either entity or ttl are null
*/
void insert(T entity, Duration ttl);
/**
* Inserts entities asynchronously, by default it's just run for each saving using
* {@link ColumnTemplate#insert(Object)},
* each NoSQL vendor might replace to a more appropriate one.
*
* @param entities entities to be saved
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when entities is null
*/
default void insert(Iterable entities) {
Objects.requireNonNull(entities, "entities is required");
StreamSupport.stream(entities.spliterator(), false).forEach(this::insert);
}
/**
* Inserts entities asynchronously with time to live, by default it's just run for each saving using
* {@link ColumnTemplate#insert(Object, Duration)},
* each NoSQL vendor might replace to a more appropriate one.
*
* @param entities entities to be saved
* @param ttl time to live
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when either entities or ttl are null
*/
default void insert(Iterable entities, Duration ttl) {
Objects.requireNonNull(entities, "entities is required");
Objects.requireNonNull(ttl, "ttl is required");
StreamSupport.stream(entities.spliterator(), false).forEach(d -> insert(d, ttl));
}
/**
* Inserts an entity asynchronously
*
* @param entity entity to be saved
* @param callback the callback, when the process is finished will call this instance returning
* the saved entity within parameters
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when either entity or callback are null
*/
void insert(T entity, Consumer callback);
/**
* Inserts an entity asynchronously with time to live
*
* @param entity entity to be saved
* @param ttl time to live
* @param callback the callback, when the process is finished will call this instance returning
* the saved entity within parameters
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when either entity or ttl or callback are null
*/
void insert(T entity, Duration ttl, Consumer callback);
/**
* Inserts an entity asynchronously
*
* @param entity entity to be updated
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when entity is null
*/
void update(T entity);
/**
* Inserts entities asynchronously, by default it's just run for each saving using
* {@link ColumnTemplate#update(Object)},
* each NoSQL vendor might replace to a more appropriate one.
*
* @param entities entities to be saved
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when entities is null
*/
default void update(Iterable entities) {
Objects.requireNonNull(entities, "entities is required");
StreamSupport.stream(entities.spliterator(), false).forEach(this::update);
}
/**
* Inserts an entity asynchronously
*
* @param entity entity to be updated
* @param callback the callback, when the process is finished will call this instance returning
* the updated entity within parametersa
* @param the instance type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when either entity or callback are null
*/
void update(T entity, Consumer callback);
/**
* Deletes an entity asynchronously
*
* @param query query to delete an entity
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when query are null
*/
void delete(ColumnDeleteQuery query);
/**
* Deletes an entity asynchronously
*
* @param query query to delete an entity
* @param callback the callback, when the process is finished will call this instance returning
* the null within parameters
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to delete asynchronous
* @throws NullPointerException when either query or callback are null
*/
void delete(ColumnDeleteQuery query, Consumer callback);
/**
* Finds entities from query asynchronously
*
* @param query query to select entities
* @param the instance type
* @param callback the callback, when the process is finished will call this instance returning
* the result of query within parameters
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when either query or callback are null
*/
void select(ColumnQuery query, Consumer> callback);
/**
* Executes a query then bring the result as a {@link List}
*
* @param callback the callback, when the process is finished will call this instance returning
* @param query the query
* @param the entity type
* @throws NullPointerException when the query is null
*/
void query(String query, Consumer> callback);
/**
* Executes a query then bring the result as a unique result
*
* @param callback the callback, when the process is finished will call this instance returning
* @param query the query
* @param the entity type
* @throws NullPointerException when the query is null
* @throws NonUniqueResultException if returns more than one result
*/
void singleResult(String query, Consumer> callback);
/**
* Creates a {@link PreparedStatementAsync} from the query
*
* @param query the query
* @return a {@link PreparedStatementAsync} instance
* @throws NullPointerException when the query is null
*/
PreparedStatementAsync prepare(String query);
/**
* Finds by Id.
*
* @param entityClass the entity class
* @param id the id value
* @param the entity class type
* @param the id type
* @param callback the callback observer
* @throws NullPointerException when either the entityClass or id are null
* @throws org.jnosql.artemis.IdNotFoundException when the entityClass does not have the Id annotation
*/
void find(Class entityClass, ID id, Consumer> callback);
/**
* Deletes by Id.
*
* @param entityClass the entity class
* @param id the id value
* @param the entity class type
* @param the id type
* @param callback the callback
* @throws NullPointerException when either the entityClass or id are null
* @throws org.jnosql.artemis.IdNotFoundException when the entityClass does not have the Id annotation
*/
void delete(Class entityClass, ID id, Consumer callback);
/**
* Deletes by Id.
*
* @param entityClass the entity class
* @param id the id value
* @param the entity class type
* @param the id type
* @throws NullPointerException when either the entityClass or id are null
* @throws org.jnosql.artemis.IdNotFoundException when the entityClass does not have the Id annotation
*/
void delete(Class entityClass, ID id);
/**
* Returns the number of elements from column family
*
* @param columnFamily the column family
* @param callback the callback with the response
* @throws NullPointerException when there is null parameter
* @throws UnsupportedOperationException when the database dot not have support
*/
void count(String columnFamily, Consumer callback);
/**
* Returns the number of elements from column family
*
* @param entityClass the entity class
* @param callback the callback with the response
* @throws NullPointerException when there is null parameter
* @throws UnsupportedOperationException when the database dot not have support
*/
void count(Class entityClass, Consumer callback);
/**
* Execute a query to consume an unique result
*
* @param query the query
* @param callback the callback
* @param the type
* @throws org.jnosql.diana.api.ExecuteAsyncQueryException when there is a async error
* @throws UnsupportedOperationException when the database does not have support to insert asynchronous
* @throws NullPointerException when either query or callback are null
* @throws NonUniqueResultException when it returns more than one result
*/
default void singleResult(ColumnQuery query, Consumer> callback) {
requireNonNull(callback, "callback is required");
Consumer> singleCallBack = entities -> {
if (entities.isEmpty()) {
callback.accept(Optional.empty());
} else if (entities.size() == 1) {
callback.accept(Optional.of(entities.get(0)));
} else {
throw new NonUniqueResultException("The query returns more than one entity, query: " + query);
}
};
select(query, singleCallBack);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy