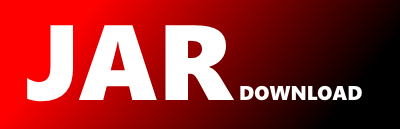
org.jnosql.diana.cassandra.column.CassandraConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cassandra-driver Show documentation
Show all versions of cassandra-driver Show documentation
The Eclipse JNoSQL communication layer, Diana, to Cassandra
/*
* Copyright (c) 2017 Otávio Santana and others
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Apache License v2.0 is available at http://www.opensource.org/licenses/apache2.0.php.
*
* You may elect to redistribute this code under either of these licenses.
*
* Contributors:
*
* Otavio Santana
*/
package org.jnosql.diana.cassandra.column;
import com.datastax.driver.core.Cluster;
import org.jnosql.diana.api.Settings;
import org.jnosql.diana.api.column.UnaryColumnConfiguration;
import org.jnosql.diana.driver.ConfigurationReader;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.ExecutorService;
import static java.util.Objects.requireNonNull;
/**
* The Cassandra implementation to {@link UnaryColumnConfiguration} that returns
* {@link CassandraColumnFamilyManagerFactory}
* This configuration reads "diana-cassandra.properties" files and has the following configuration:
* cassandra-host-: The Cassandra host as prefix, you can set how much you want just setting the number order,
* eg: cassandra-host-1 = host, cassandra-host-2 = host2
* cassandra-query-: The Cassandra query to run when an instance is started, you can set how much you want just
* setting the order number, eg: cassandra-query-1=cql, cassandra-query-2=cql2...
* cassandra-threads-number: The number of executor to run on Async process, if it isn't defined that will use the number of processor
* cassandra-ssl: Define ssl, the default value is false
* cassandra-metrics: enable metrics, the default value is true
* cassandra-jmx: enable JMX, the default value is true
*/
public class CassandraConfiguration implements UnaryColumnConfiguration {
private static final String CASSANDRA_FILE_CONFIGURATION = "diana-cassandra.properties";
public CassandraColumnFamilyManagerFactory getManagerFactory(Map configurations) {
requireNonNull(configurations);
CassandraProperties properties = CassandraProperties.of(configurations);
ExecutorService executorService = properties.createExecutorService();
return new CassandraColumnFamilyManagerFactory(properties.createCluster(), properties.getQueries(), executorService);
}
public CassandraColumnFamilyManagerFactory getEntityManagerFactory(Cluster cluster) {
requireNonNull(cluster, "Cluster is required");
Map configuration = ConfigurationReader.from(CASSANDRA_FILE_CONFIGURATION);
CassandraProperties properties = CassandraProperties.of(configuration);
ExecutorService executorService = properties.createExecutorService();
return new CassandraColumnFamilyManagerFactory(cluster, properties.getQueries(), executorService);
}
@Override
public CassandraColumnFamilyManagerFactory get() {
Map configuration = ConfigurationReader.from(CASSANDRA_FILE_CONFIGURATION);
return getManagerFactory(configuration);
}
@Override
public CassandraColumnFamilyManagerFactory get(Settings settings) throws NullPointerException {
requireNonNull(settings, "settings is required");
Map configurations = new HashMap<>();
settings.forEach((key, value) -> configurations.put(key, value.toString()));
return getManagerFactory(configurations);
}
@Override
public CassandraColumnFamilyManagerFactory getAsync() {
Map configuration = ConfigurationReader.from(CASSANDRA_FILE_CONFIGURATION);
return getManagerFactory(configuration);
}
@Override
public CassandraColumnFamilyManagerFactory getAsync(Settings settings) throws NullPointerException {
return get(settings);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy