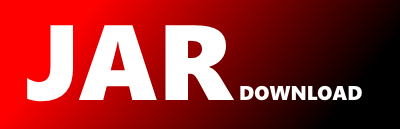
org.jnosql.diana.couchbase.key.CouchbaseBucketManagerFactory Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Otávio Santana and others
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Apache License v2.0 is available at http://www.opensource.org/licenses/apache2.0.php.
*
* You may elect to redistribute this code under either of these licenses.
*
* Contributors:
*
* Otavio Santana
*/
package org.jnosql.diana.couchbase.key;
import org.jnosql.diana.api.key.BucketManagerFactory;
import java.util.List;
import java.util.Map;
import java.util.Queue;
import java.util.Set;
/**
* The couchbase implementation of {@link BucketManagerFactory}. That has support to
* {@link BucketManagerFactory#getBucketManager(String)} and also the structure {@link Map}, {@link Set},
* {@link Queue}, {@link List}. Each structure has this specific implementation.
* {@link CouchbaseList}
* {@link CouchbaseSet}
* {@link CouchbaseQueue}
* {@link CouchbaseMap}
* The default implementation creates the particular structure with the bucket name as the key.
*/
public interface CouchbaseBucketManagerFactory extends BucketManagerFactory {
/**
* Creates a {@link Queue} from bucket name
*
* @param bucketName a bucket name
* @param clazz the value class
* @param key key to the queue
* @param the value type
* @return a {@link Queue} instance
* @throws UnsupportedOperationException when the database does not have to it
* @throws NullPointerException when either bucketName or class are null
*/
Queue getQueue(String bucketName, String key, Class clazz);
/**
* Creates a {@link Set} from bucket name
*
* @param bucketName a bucket name
* @param clazz the valeu class
* @param key key to the set
* @param the value type
* @return a {@link Set} instance
* @throws UnsupportedOperationException when the database does not have to it
* @throws NullPointerException when either bucketName or class are null
*/
Set getSet(String bucketName, String key, Class clazz);
/**
* Creates a {@link List} from bucket name
*
* @param bucketName a bucket name
* @param clazz the valeu class
* @param key key to the List
* @param the value type
* @return a {@link List} instance
* @throws UnsupportedOperationException when the database does not have to it
* @throws NullPointerException when either bucketName or class are null
*/
List getList(String bucketName, String key, Class clazz);
/**
* Creates a {@link Map} from bucket name
*
* @param bucketName the bucket name
* @param key key to the Map
* @param keyValue the key class
* @param valueValue the value class
* @param the key type
* @param the value type
* @return a {@link Map} instance
* @throws UnsupportedOperationException when the database does not have to it
* @throws NullPointerException when either bucketName or class are null
*/
Map getMap(String bucketName, String key, Class keyValue, Class valueValue);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy