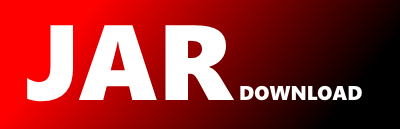
org.jobrunr.utils.mapper.jsonb.adapters.JobMetadataAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jobrunr Show documentation
Show all versions of jobrunr Show documentation
An easy way to perform background processing on the JVM. Backed by persistent storage. Open and free for commercial use.
package org.jobrunr.utils.mapper.jsonb.adapters;
import org.jobrunr.utils.mapper.jsonb.JobRunrJsonb;
import javax.json.*;
import javax.json.bind.adapter.JsonbAdapter;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import static javax.json.JsonValue.ValueType.*;
import static org.jobrunr.utils.mapper.jsonb.NullSafeJsonBuilder.nullSafeJsonObjectBuilder;
import static org.jobrunr.utils.reflection.ReflectionUtils.toClass;
public class JobMetadataAdapter implements JsonbAdapter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy