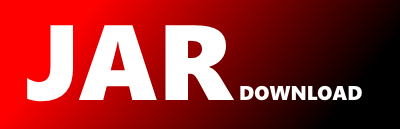
org.joda.beans.impl.reflection.ReflectiveMetaBean Maven / Gradle / Ivy
/*
* Copyright 2001-2014 Stephen Colebourne
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joda.beans.impl.reflection;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.NoSuchElementException;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.PropertyMap;
import org.joda.beans.impl.BasicBeanBuilder;
import org.joda.beans.impl.BasicPropertyMap;
/**
* A standard meta-bean implementation.
*
* This is the standard implementation of a meta-bean.
* It requires that the bean implements {@code Bean} and has a no-arguments constructor.
*
* @author Stephen Colebourne
*/
public final class ReflectiveMetaBean implements MetaBean {
/** The bean type. */
private final Class extends Bean> beanType;
/** The meta-property instances of the bean. */
private final Map> metaPropertyMap;
/**
* Factory to create a meta-bean avoiding duplicate generics.
*
* @param the type of the bean
* @param beanClass the bean class, not null
* @return the meta-bean, not null
*/
public static ReflectiveMetaBean of(Class beanClass) {
return new ReflectiveMetaBean(beanClass);
}
/**
* Constructor.
*
* @param beanType the bean type, not null
*/
@SuppressWarnings("unchecked")
private ReflectiveMetaBean(Class extends Bean> beanType) {
if (beanType == null) {
throw new NullPointerException("Bean class must not be null");
}
this.beanType = beanType;
Map> map = new HashMap>();
Field[] fields = beanType.getDeclaredFields();
for (Field field : fields) {
if (MetaProperty.class.isAssignableFrom(field.getType()) && Modifier.isStatic(field.getModifiers())) {
field.setAccessible(true);
MetaProperty