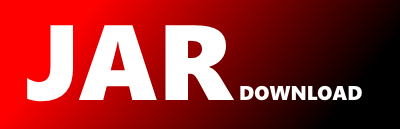
jodd.db.oom.config.AutomagicDbOomConfigurator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jodd-db Show documentation
Show all versions of jodd-db Show documentation
Jodd DB is efficient and thin database facade; DbOom is convenient database object mapper.
// Copyright (c) 2003-2012, Jodd Team (jodd.org). All Rights Reserved.
package jodd.db.oom.config;
import jodd.db.oom.DbOomManager;
import jodd.io.findfile.ClassFinder;
import jodd.db.oom.DbOomException;
import jodd.db.oom.meta.DbTable;
import jodd.util.ClassLoaderUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.InputStream;
/**
* Auto-magically reads classpath for domain objects annotated
*/
public class AutomagicDbOomConfigurator extends ClassFinder {
private static final Logger log = LoggerFactory.getLogger(AutomagicDbOomConfigurator.class);
protected final byte[] dbTableAnnotationBytes;
protected final boolean registerAsEntities;
public AutomagicDbOomConfigurator(boolean registerAsEntities) {
dbTableAnnotationBytes = getTypeSignatureBytes(DbTable.class);
this.registerAsEntities = registerAsEntities;
}
public AutomagicDbOomConfigurator() {
this(true);
}
protected DbOomManager dbOomManager;
protected long elapsed;
/**
* Return elapsed number of milliseconds for configuration.
*/
public long getElapsed() {
return elapsed;
}
/**
* Configures {@link DbOomManager} with specified class path.
* @see AutomagicDbOomConfigurator#configure(jodd.db.oom.DbOomManager)
*/
public void configure(DbOomManager dbOomManager, File[] classpath) {
this.dbOomManager = dbOomManager;
elapsed = System.currentTimeMillis();
try {
scanPaths(classpath);
} catch (Exception ex) {
throw new DbOomException("Unable to scan classpath.", ex);
}
elapsed = System.currentTimeMillis() - elapsed;
log.info("DbOomManager configured in " + elapsed + " ms. Total entities: " + dbOomManager.getTotalNames());
}
/**
* Configures {@link DbOomManager} with default class path.
* @see AutomagicDbOomConfigurator#configure(jodd.db.oom.DbOomManager, java.io.File[])
*/
public void configure(DbOomManager dbOomManager) {
configure(dbOomManager, ClassLoaderUtil.getDefaultClasspath());
}
/**
* Scans all classes and registers only those annotated with {@link DbTable}.
* Because of performance purposes, classes are not dynamically loaded; instead, their
* file content is examined.
*/
@Override
protected void onEntry(EntryData entryData) {
String entryName = entryData.getName();
InputStream inputStream = entryData.openInputStream();
if (isTypeSignatureInUse(inputStream, dbTableAnnotationBytes) == false) {
return;
}
Class> beanClass;
try {
beanClass = loadClass(entryName);
} catch (ClassNotFoundException cnfex) {
throw new DbOomException("Unable to load class: " + entryName, cnfex);
}
DbTable dbTable = beanClass.getAnnotation(DbTable.class);
if (dbTable == null) {
return;
}
if (registerAsEntities == true) {
dbOomManager.registerEntity(beanClass);
} else {
dbOomManager.registerType(beanClass);
}
}
/**
* Loads class from classname using default classloader.
*/
protected Class loadClass(String className) throws ClassNotFoundException {
return ClassLoaderUtil.loadClass(className);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy