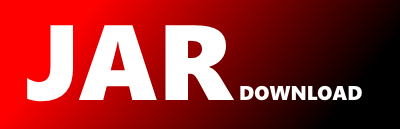
jodd.madvoc.WebApp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jodd-madvoc Show documentation
Show all versions of jodd-madvoc Show documentation
Jodd Madvoc is elegant web MVC framework that uses CoC and annotations in a pragmatic way to simplify web application development.
// Copyright (c) 2003-present, Jodd Team (http://jodd.org)
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions are met:
//
// 1. Redistributions of source code must retain the above copyright notice,
// this list of conditions and the following disclaimer.
//
// 2. Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
// AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
// ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
// LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
// CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
// SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
// INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
// CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
// ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
package jodd.madvoc;
import jodd.log.Logger;
import jodd.log.LoggerFactory;
import jodd.madvoc.component.ActionConfigManager;
import jodd.madvoc.component.ActionMethodParamNameResolver;
import jodd.madvoc.component.ActionMethodParser;
import jodd.madvoc.component.ActionPathRewriter;
import jodd.madvoc.component.ActionsManager;
import jodd.madvoc.component.AsyncActionExecutor;
import jodd.madvoc.component.ContextInjectorComponent;
import jodd.madvoc.component.FileUploader;
import jodd.madvoc.component.FiltersManager;
import jodd.madvoc.component.InterceptorsManager;
import jodd.madvoc.component.MadvocComponentLifecycle;
import jodd.madvoc.component.MadvocComponentLifecycle.Init;
import jodd.madvoc.component.MadvocComponentLifecycle.Ready;
import jodd.madvoc.component.MadvocComponentLifecycle.Start;
import jodd.madvoc.component.MadvocContainer;
import jodd.madvoc.component.MadvocController;
import jodd.madvoc.component.MadvocEncoding;
import jodd.madvoc.component.ResultMapper;
import jodd.madvoc.component.ResultsManager;
import jodd.madvoc.component.RootPackages;
import jodd.madvoc.component.ScopeDataInspector;
import jodd.madvoc.component.ScopeResolver;
import jodd.madvoc.component.ServletContextProvider;
import jodd.madvoc.meta.Action;
import jodd.madvoc.meta.RestAction;
import jodd.props.Props;
import jodd.util.ClassConsumer;
import jodd.util.function.Consumers;
import javax.servlet.ServletContext;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Consumer;
/**
* Web application contains all configurations and holds all managers and controllers of one web application.
* Custom implementations may override this class to enhance several different functionality.
*/
@SuppressWarnings({"UnusedDeclaration"})
public class WebApp {
private static Logger log;
private static final String WEBAPP_ATTR = WebApp.class.getName();
public static WebApp createWebApp() {
return new WebApp();
}
/**
* Returns WebApp
instance from servlet context.
* May return null
indicating WebApp
* is not yet initialized.
*/
public static WebApp get(final ServletContext servletContext) {
return (WebApp) servletContext.getAttribute(WEBAPP_ATTR);
}
// ---------------------------------------------------------------- builder
protected ServletContext servletContext;
private List propsList = new ArrayList<>();
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy