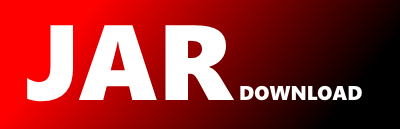
jogamp.openal.ALImpl Maven / Gradle / Ivy
/* !---- DO NOT EDIT: This file autogenerated by com/jogamp/gluegen/procaddress/ProcAddressEmitter.java on Thu Jan 30 12:09:32 CET 2014 ----! */
package jogamp.openal;
import com.jogamp.openal.*;
import jogamp.openal.*;
import java.security.AccessController;
import java.security.PrivilegedAction;
import com.jogamp.gluegen.runtime.*;
import com.jogamp.common.os.*;
import com.jogamp.common.nio.*;
import java.nio.*;
public class ALImpl implements AL{
/** Entry point (through function pointer) to C language function:
void alBuffer3f(ALuint buffer, ALenum param, ALfloat value1, ALfloat value2, ALfloat value3);
*/
public void alBuffer3f(int buffer, int param, float value1, float value2, float value3) {
final long __addr_ = alProcAddressTable._addressof_alBuffer3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBuffer3f"));
}
dispatch_alBuffer3f1(buffer, param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBuffer3f(ALuint buffer, ALenum param, ALfloat value1, ALfloat value2, ALfloat value3);
*/
private native void dispatch_alBuffer3f1(int buffer, int param, float value1, float value2, float value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBuffer3i(ALuint buffer, ALenum param, ALint value1, ALint value2, ALint value3);
*/
public void alBuffer3i(int buffer, int param, int value1, int value2, int value3) {
final long __addr_ = alProcAddressTable._addressof_alBuffer3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBuffer3i"));
}
dispatch_alBuffer3i1(buffer, param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBuffer3i(ALuint buffer, ALenum param, ALint value1, ALint value2, ALint value3);
*/
private native void dispatch_alBuffer3i1(int buffer, int param, int value1, int value2, int value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferData(ALuint buffer, ALenum format, const ALvoid * data, ALsizei size, ALsizei freq);
@param data a direct or array-backed {@link java.nio.Buffer} */
public void alBufferData(int buffer, int format, Buffer data, int size, int freq) {
final boolean data_is_direct = Buffers.isDirect(data);
final long __addr_ = alProcAddressTable._addressof_alBufferData;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferData"));
}
dispatch_alBufferData1(buffer, format, data_is_direct ? data : Buffers.getArray(data), data_is_direct ? Buffers.getDirectBufferByteOffset(data) : Buffers.getIndirectBufferByteOffset(data), data_is_direct, size, freq, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferData(ALuint buffer, ALenum format, const ALvoid * data, ALsizei size, ALsizei freq);
@param data a direct or array-backed {@link java.nio.Buffer} */
private native void dispatch_alBufferData1(int buffer, int format, Object data, int data_byte_offset, boolean data_is_direct, int size, int freq, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferf(ALuint buffer, ALenum param, ALfloat value);
*/
public void alBufferf(int buffer, int param, float value) {
final long __addr_ = alProcAddressTable._addressof_alBufferf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferf"));
}
dispatch_alBufferf1(buffer, param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferf(ALuint buffer, ALenum param, ALfloat value);
*/
private native void dispatch_alBufferf1(int buffer, int param, float value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferfv(ALuint buffer, ALenum param, const ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
public void alBufferfv(int buffer, int param, FloatBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alBufferfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferfv"));
}
dispatch_alBufferfv1(buffer, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferfv(ALuint buffer, ALenum param, const ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alBufferfv1(int buffer, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferfv(ALuint buffer, ALenum param, const ALfloat * values);
*/
public void alBufferfv(int buffer, int param, float[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alBufferfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferfv"));
}
dispatch_alBufferfv1(buffer, param, values, Buffers.SIZEOF_FLOAT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferi(ALuint buffer, ALenum param, ALint value);
*/
public void alBufferi(int buffer, int param, int value) {
final long __addr_ = alProcAddressTable._addressof_alBufferi;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferi"));
}
dispatch_alBufferi1(buffer, param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferi(ALuint buffer, ALenum param, ALint value);
*/
private native void dispatch_alBufferi1(int buffer, int param, int value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferiv(ALuint buffer, ALenum param, const ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
public void alBufferiv(int buffer, int param, IntBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alBufferiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferiv"));
}
dispatch_alBufferiv1(buffer, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferiv(ALuint buffer, ALenum param, const ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alBufferiv1(int buffer, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferiv(ALuint buffer, ALenum param, const ALint * values);
*/
public void alBufferiv(int buffer, int param, int[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alBufferiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferiv"));
}
dispatch_alBufferiv1(buffer, param, values, Buffers.SIZEOF_INT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDeleteBuffers(ALsizei n, const ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
public void alDeleteBuffers(int n, IntBuffer buffers) {
final boolean buffers_is_direct = Buffers.isDirect(buffers);
final long __addr_ = alProcAddressTable._addressof_alDeleteBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteBuffers"));
}
dispatch_alDeleteBuffers1(n, buffers_is_direct ? buffers : Buffers.getArray(buffers), buffers_is_direct ? Buffers.getDirectBufferByteOffset(buffers) : Buffers.getIndirectBufferByteOffset(buffers), buffers_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDeleteBuffers(ALsizei n, const ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alDeleteBuffers1(int n, Object buffers, int buffers_byte_offset, boolean buffers_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alDeleteBuffers(ALsizei n, const ALuint * buffers);
*/
public void alDeleteBuffers(int n, int[] buffers, int buffers_offset) {
if(buffers != null && buffers.length <= buffers_offset)
throw new ALException("array offset argument \"buffers_offset\" (" + buffers_offset + ") equals or exceeds array length (" + buffers.length + ")");
final long __addr_ = alProcAddressTable._addressof_alDeleteBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteBuffers"));
}
dispatch_alDeleteBuffers1(n, buffers, Buffers.SIZEOF_INT * buffers_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDeleteSources(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
public void alDeleteSources(int n, IntBuffer sources) {
final boolean sources_is_direct = Buffers.isDirect(sources);
final long __addr_ = alProcAddressTable._addressof_alDeleteSources;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteSources"));
}
dispatch_alDeleteSources1(n, sources_is_direct ? sources : Buffers.getArray(sources), sources_is_direct ? Buffers.getDirectBufferByteOffset(sources) : Buffers.getIndirectBufferByteOffset(sources), sources_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDeleteSources(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alDeleteSources1(int n, Object sources, int sources_byte_offset, boolean sources_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alDeleteSources(ALsizei n, const ALuint * sources);
*/
public void alDeleteSources(int n, int[] sources, int sources_offset) {
if(sources != null && sources.length <= sources_offset)
throw new ALException("array offset argument \"sources_offset\" (" + sources_offset + ") equals or exceeds array length (" + sources.length + ")");
final long __addr_ = alProcAddressTable._addressof_alDeleteSources;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteSources"));
}
dispatch_alDeleteSources1(n, sources, Buffers.SIZEOF_INT * sources_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDisable(ALenum capability);
*/
public void alDisable(int capability) {
final long __addr_ = alProcAddressTable._addressof_alDisable;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDisable"));
}
dispatch_alDisable1(capability, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDisable(ALenum capability);
*/
private native void dispatch_alDisable1(int capability, long procAddress);
/** Entry point (through function pointer) to C language function:
void alDistanceModel(ALenum distanceModel);
*/
public void alDistanceModel(int distanceModel) {
final long __addr_ = alProcAddressTable._addressof_alDistanceModel;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDistanceModel"));
}
dispatch_alDistanceModel1(distanceModel, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDistanceModel(ALenum distanceModel);
*/
private native void dispatch_alDistanceModel1(int distanceModel, long procAddress);
/** Entry point (through function pointer) to C language function:
void alDopplerFactor(ALfloat value);
*/
public void alDopplerFactor(float value) {
final long __addr_ = alProcAddressTable._addressof_alDopplerFactor;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDopplerFactor"));
}
dispatch_alDopplerFactor1(value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDopplerFactor(ALfloat value);
*/
private native void dispatch_alDopplerFactor1(float value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alDopplerVelocity(ALfloat value);
*/
public void alDopplerVelocity(float value) {
final long __addr_ = alProcAddressTable._addressof_alDopplerVelocity;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDopplerVelocity"));
}
dispatch_alDopplerVelocity1(value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alDopplerVelocity(ALfloat value);
*/
private native void dispatch_alDopplerVelocity1(float value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alEnable(ALenum capability);
*/
public void alEnable(int capability) {
final long __addr_ = alProcAddressTable._addressof_alEnable;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alEnable"));
}
dispatch_alEnable1(capability, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alEnable(ALenum capability);
*/
private native void dispatch_alEnable1(int capability, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGenBuffers(ALsizei n, ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
public void alGenBuffers(int n, IntBuffer buffers) {
final boolean buffers_is_direct = Buffers.isDirect(buffers);
final long __addr_ = alProcAddressTable._addressof_alGenBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenBuffers"));
}
dispatch_alGenBuffers1(n, buffers_is_direct ? buffers : Buffers.getArray(buffers), buffers_is_direct ? Buffers.getDirectBufferByteOffset(buffers) : Buffers.getIndirectBufferByteOffset(buffers), buffers_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGenBuffers(ALsizei n, ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGenBuffers1(int n, Object buffers, int buffers_byte_offset, boolean buffers_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGenBuffers(ALsizei n, ALuint * buffers);
*/
public void alGenBuffers(int n, int[] buffers, int buffers_offset) {
if(buffers != null && buffers.length <= buffers_offset)
throw new ALException("array offset argument \"buffers_offset\" (" + buffers_offset + ") equals or exceeds array length (" + buffers.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGenBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenBuffers"));
}
dispatch_alGenBuffers1(n, buffers, Buffers.SIZEOF_INT * buffers_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGenSources(ALsizei n, ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
public void alGenSources(int n, IntBuffer sources) {
final boolean sources_is_direct = Buffers.isDirect(sources);
final long __addr_ = alProcAddressTable._addressof_alGenSources;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenSources"));
}
dispatch_alGenSources1(n, sources_is_direct ? sources : Buffers.getArray(sources), sources_is_direct ? Buffers.getDirectBufferByteOffset(sources) : Buffers.getIndirectBufferByteOffset(sources), sources_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGenSources(ALsizei n, ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGenSources1(int n, Object sources, int sources_byte_offset, boolean sources_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGenSources(ALsizei n, ALuint * sources);
*/
public void alGenSources(int n, int[] sources, int sources_offset) {
if(sources != null && sources.length <= sources_offset)
throw new ALException("array offset argument \"sources_offset\" (" + sources_offset + ") equals or exceeds array length (" + sources.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGenSources;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenSources"));
}
dispatch_alGenSources1(n, sources, Buffers.SIZEOF_INT * sources_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alGetBoolean(ALenum param);
*/
public boolean alGetBoolean(int param) {
final long __addr_ = alProcAddressTable._addressof_alGetBoolean;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBoolean"));
}
return dispatch_alGetBoolean1(param, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alGetBoolean(ALenum param);
*/
private native boolean dispatch_alGetBoolean1(int param, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBooleanv(ALenum param, ALboolean * values);
@param values a direct or array-backed {@link java.nio.ByteBuffer} */
public void alGetBooleanv(int param, ByteBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetBooleanv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBooleanv"));
}
dispatch_alGetBooleanv1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBooleanv(ALenum param, ALboolean * values);
@param values a direct or array-backed {@link java.nio.ByteBuffer} */
private native void dispatch_alGetBooleanv1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBooleanv(ALenum param, ALboolean * values);
*/
public void alGetBooleanv(int param, byte[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetBooleanv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBooleanv"));
}
dispatch_alGetBooleanv1(param, values, values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBuffer3f(ALuint buffer, ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
@param value1 a direct or array-backed {@link java.nio.FloatBuffer}
@param value2 a direct or array-backed {@link java.nio.FloatBuffer}
@param value3 a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetBuffer3f(int buffer, int param, FloatBuffer value1, FloatBuffer value2, FloatBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alProcAddressTable._addressof_alGetBuffer3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBuffer3f"));
}
dispatch_alGetBuffer3f1(buffer, param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBuffer3f(ALuint buffer, ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
@param value1 a direct or array-backed {@link java.nio.FloatBuffer}
@param value2 a direct or array-backed {@link java.nio.FloatBuffer}
@param value3 a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetBuffer3f1(int buffer, int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBuffer3f(ALuint buffer, ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
*/
public void alGetBuffer3f(int buffer, int param, float[] value1, int value1_offset, float[] value2, int value2_offset, float[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetBuffer3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBuffer3f"));
}
dispatch_alGetBuffer3f1(buffer, param, value1, Buffers.SIZEOF_FLOAT * value1_offset, false, value2, Buffers.SIZEOF_FLOAT * value2_offset, false, value3, Buffers.SIZEOF_FLOAT * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBuffer3i(ALuint buffer, ALenum param, ALint * value1, ALint * value2, ALint * value3);
@param value1 a direct or array-backed {@link java.nio.IntBuffer}
@param value2 a direct or array-backed {@link java.nio.IntBuffer}
@param value3 a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetBuffer3i(int buffer, int param, IntBuffer value1, IntBuffer value2, IntBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alProcAddressTable._addressof_alGetBuffer3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBuffer3i"));
}
dispatch_alGetBuffer3i1(buffer, param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBuffer3i(ALuint buffer, ALenum param, ALint * value1, ALint * value2, ALint * value3);
@param value1 a direct or array-backed {@link java.nio.IntBuffer}
@param value2 a direct or array-backed {@link java.nio.IntBuffer}
@param value3 a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetBuffer3i1(int buffer, int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBuffer3i(ALuint buffer, ALenum param, ALint * value1, ALint * value2, ALint * value3);
*/
public void alGetBuffer3i(int buffer, int param, int[] value1, int value1_offset, int[] value2, int value2_offset, int[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetBuffer3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBuffer3i"));
}
dispatch_alGetBuffer3i1(buffer, param, value1, Buffers.SIZEOF_INT * value1_offset, false, value2, Buffers.SIZEOF_INT * value2_offset, false, value3, Buffers.SIZEOF_INT * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferf(ALuint buffer, ALenum param, ALfloat * value);
@param value a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetBufferf(int buffer, int param, FloatBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alProcAddressTable._addressof_alGetBufferf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferf"));
}
dispatch_alGetBufferf1(buffer, param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferf(ALuint buffer, ALenum param, ALfloat * value);
@param value a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetBufferf1(int buffer, int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBufferf(ALuint buffer, ALenum param, ALfloat * value);
*/
public void alGetBufferf(int buffer, int param, float[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetBufferf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferf"));
}
dispatch_alGetBufferf1(buffer, param, value, Buffers.SIZEOF_FLOAT * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferfv(ALuint buffer, ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetBufferfv(int buffer, int param, FloatBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetBufferfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferfv"));
}
dispatch_alGetBufferfv1(buffer, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferfv(ALuint buffer, ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetBufferfv1(int buffer, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBufferfv(ALuint buffer, ALenum param, ALfloat * values);
*/
public void alGetBufferfv(int buffer, int param, float[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetBufferfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferfv"));
}
dispatch_alGetBufferfv1(buffer, param, values, Buffers.SIZEOF_FLOAT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferi(ALuint buffer, ALenum param, ALint * value);
@param value a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetBufferi(int buffer, int param, IntBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alProcAddressTable._addressof_alGetBufferi;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferi"));
}
dispatch_alGetBufferi1(buffer, param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferi(ALuint buffer, ALenum param, ALint * value);
@param value a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetBufferi1(int buffer, int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBufferi(ALuint buffer, ALenum param, ALint * value);
*/
public void alGetBufferi(int buffer, int param, int[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetBufferi;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferi"));
}
dispatch_alGetBufferi1(buffer, param, value, Buffers.SIZEOF_INT * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferiv(ALuint buffer, ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetBufferiv(int buffer, int param, IntBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetBufferiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferiv"));
}
dispatch_alGetBufferiv1(buffer, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferiv(ALuint buffer, ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetBufferiv1(int buffer, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetBufferiv(ALuint buffer, ALenum param, ALint * values);
*/
public void alGetBufferiv(int buffer, int param, int[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetBufferiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferiv"));
}
dispatch_alGetBufferiv1(buffer, param, values, Buffers.SIZEOF_INT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALdouble alGetDouble(ALenum param);
*/
public double alGetDouble(int param) {
final long __addr_ = alProcAddressTable._addressof_alGetDouble;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetDouble"));
}
return dispatch_alGetDouble1(param, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALdouble alGetDouble(ALenum param);
*/
private native double dispatch_alGetDouble1(int param, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetDoublev(ALenum param, ALdouble * values);
@param values a direct or array-backed {@link java.nio.DoubleBuffer} */
public void alGetDoublev(int param, DoubleBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetDoublev;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetDoublev"));
}
dispatch_alGetDoublev1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetDoublev(ALenum param, ALdouble * values);
@param values a direct or array-backed {@link java.nio.DoubleBuffer} */
private native void dispatch_alGetDoublev1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetDoublev(ALenum param, ALdouble * values);
*/
public void alGetDoublev(int param, double[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetDoublev;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetDoublev"));
}
dispatch_alGetDoublev1(param, values, Buffers.SIZEOF_DOUBLE * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALenum alGetEnumValue(const ALchar * ename);
*/
public int alGetEnumValue(String ename) {
final long __addr_ = alProcAddressTable._addressof_alGetEnumValue;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEnumValue"));
}
return dispatch_alGetEnumValue1(ename, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALenum alGetEnumValue(const ALchar * ename);
*/
private native int dispatch_alGetEnumValue1(String ename, long procAddress);
/** Entry point (through function pointer) to C language function:
ALenum alGetError(void);
*/
public int alGetError() {
final long __addr_ = alProcAddressTable._addressof_alGetError;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetError"));
}
return dispatch_alGetError1(__addr_);
}
/** Entry point (through function pointer) to C language function:
ALenum alGetError(void);
*/
private native int dispatch_alGetError1(long procAddress);
/** Entry point (through function pointer) to C language function:
ALfloat alGetFloat(ALenum param);
*/
public float alGetFloat(int param) {
final long __addr_ = alProcAddressTable._addressof_alGetFloat;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFloat"));
}
return dispatch_alGetFloat1(param, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALfloat alGetFloat(ALenum param);
*/
private native float dispatch_alGetFloat1(int param, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetFloatv(ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetFloatv(int param, FloatBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetFloatv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFloatv"));
}
dispatch_alGetFloatv1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetFloatv(ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetFloatv1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetFloatv(ALenum param, ALfloat * values);
*/
public void alGetFloatv(int param, float[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetFloatv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFloatv"));
}
dispatch_alGetFloatv1(param, values, Buffers.SIZEOF_FLOAT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALint alGetInteger(ALenum param);
*/
public int alGetInteger(int param) {
final long __addr_ = alProcAddressTable._addressof_alGetInteger;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetInteger"));
}
return dispatch_alGetInteger1(param, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALint alGetInteger(ALenum param);
*/
private native int dispatch_alGetInteger1(int param, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetIntegerv(ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetIntegerv(int param, IntBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetIntegerv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetIntegerv"));
}
dispatch_alGetIntegerv1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetIntegerv(ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetIntegerv1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetIntegerv(ALenum param, ALint * values);
*/
public void alGetIntegerv(int param, int[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetIntegerv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetIntegerv"));
}
dispatch_alGetIntegerv1(param, values, Buffers.SIZEOF_INT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListener3f(ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
@param value1 a direct or array-backed {@link java.nio.FloatBuffer}
@param value2 a direct or array-backed {@link java.nio.FloatBuffer}
@param value3 a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetListener3f(int param, FloatBuffer value1, FloatBuffer value2, FloatBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alProcAddressTable._addressof_alGetListener3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListener3f"));
}
dispatch_alGetListener3f1(param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListener3f(ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
@param value1 a direct or array-backed {@link java.nio.FloatBuffer}
@param value2 a direct or array-backed {@link java.nio.FloatBuffer}
@param value3 a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetListener3f1(int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetListener3f(ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
*/
public void alGetListener3f(int param, float[] value1, int value1_offset, float[] value2, int value2_offset, float[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetListener3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListener3f"));
}
dispatch_alGetListener3f1(param, value1, Buffers.SIZEOF_FLOAT * value1_offset, false, value2, Buffers.SIZEOF_FLOAT * value2_offset, false, value3, Buffers.SIZEOF_FLOAT * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListener3i(ALenum param, ALint * value1, ALint * value2, ALint * value3);
@param value1 a direct or array-backed {@link java.nio.IntBuffer}
@param value2 a direct or array-backed {@link java.nio.IntBuffer}
@param value3 a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetListener3i(int param, IntBuffer value1, IntBuffer value2, IntBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alProcAddressTable._addressof_alGetListener3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListener3i"));
}
dispatch_alGetListener3i1(param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListener3i(ALenum param, ALint * value1, ALint * value2, ALint * value3);
@param value1 a direct or array-backed {@link java.nio.IntBuffer}
@param value2 a direct or array-backed {@link java.nio.IntBuffer}
@param value3 a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetListener3i1(int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetListener3i(ALenum param, ALint * value1, ALint * value2, ALint * value3);
*/
public void alGetListener3i(int param, int[] value1, int value1_offset, int[] value2, int value2_offset, int[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetListener3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListener3i"));
}
dispatch_alGetListener3i1(param, value1, Buffers.SIZEOF_INT * value1_offset, false, value2, Buffers.SIZEOF_INT * value2_offset, false, value3, Buffers.SIZEOF_INT * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListenerf(ALenum param, ALfloat * value);
@param value a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetListenerf(int param, FloatBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alProcAddressTable._addressof_alGetListenerf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListenerf"));
}
dispatch_alGetListenerf1(param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListenerf(ALenum param, ALfloat * value);
@param value a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetListenerf1(int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetListenerf(ALenum param, ALfloat * value);
*/
public void alGetListenerf(int param, float[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetListenerf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListenerf"));
}
dispatch_alGetListenerf1(param, value, Buffers.SIZEOF_FLOAT * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListenerfv(ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetListenerfv(int param, FloatBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetListenerfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListenerfv"));
}
dispatch_alGetListenerfv1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListenerfv(ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetListenerfv1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetListenerfv(ALenum param, ALfloat * values);
*/
public void alGetListenerfv(int param, float[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetListenerfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListenerfv"));
}
dispatch_alGetListenerfv1(param, values, Buffers.SIZEOF_FLOAT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListeneri(ALenum param, ALint * value);
@param value a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetListeneri(int param, IntBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alProcAddressTable._addressof_alGetListeneri;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListeneri"));
}
dispatch_alGetListeneri1(param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListeneri(ALenum param, ALint * value);
@param value a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetListeneri1(int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetListeneri(ALenum param, ALint * value);
*/
public void alGetListeneri(int param, int[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetListeneri;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListeneri"));
}
dispatch_alGetListeneri1(param, value, Buffers.SIZEOF_INT * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListeneriv(ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetListeneriv(int param, IntBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetListeneriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListeneriv"));
}
dispatch_alGetListeneriv1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetListeneriv(ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetListeneriv1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetListeneriv(ALenum param, ALint * values);
*/
public void alGetListeneriv(int param, int[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetListeneriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetListeneriv"));
}
dispatch_alGetListeneriv1(param, values, Buffers.SIZEOF_INT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALproc alGetProcAddress(const ALchar * fname);
*/
long alGetProcAddress(String fname) {
final long __addr_ = alProcAddressTable._addressof_alGetProcAddress;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetProcAddress"));
}
return dispatch_alGetProcAddress1(fname, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALproc alGetProcAddress(const ALchar * fname);
*/
private native long dispatch_alGetProcAddress1(String fname, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSource3f(ALuint source, ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
@param value1 a direct or array-backed {@link java.nio.FloatBuffer}
@param value2 a direct or array-backed {@link java.nio.FloatBuffer}
@param value3 a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetSource3f(int source, int param, FloatBuffer value1, FloatBuffer value2, FloatBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alProcAddressTable._addressof_alGetSource3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3f"));
}
dispatch_alGetSource3f1(source, param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSource3f(ALuint source, ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
@param value1 a direct or array-backed {@link java.nio.FloatBuffer}
@param value2 a direct or array-backed {@link java.nio.FloatBuffer}
@param value3 a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetSource3f1(int source, int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSource3f(ALuint source, ALenum param, ALfloat * value1, ALfloat * value2, ALfloat * value3);
*/
public void alGetSource3f(int source, int param, float[] value1, int value1_offset, float[] value2, int value2_offset, float[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetSource3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3f"));
}
dispatch_alGetSource3f1(source, param, value1, Buffers.SIZEOF_FLOAT * value1_offset, false, value2, Buffers.SIZEOF_FLOAT * value2_offset, false, value3, Buffers.SIZEOF_FLOAT * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSource3i(ALuint source, ALenum param, ALint * value1, ALint * value2, ALint * value3);
@param value1 a direct or array-backed {@link java.nio.IntBuffer}
@param value2 a direct or array-backed {@link java.nio.IntBuffer}
@param value3 a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetSource3i(int source, int param, IntBuffer value1, IntBuffer value2, IntBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alProcAddressTable._addressof_alGetSource3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3i"));
}
dispatch_alGetSource3i1(source, param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSource3i(ALuint source, ALenum param, ALint * value1, ALint * value2, ALint * value3);
@param value1 a direct or array-backed {@link java.nio.IntBuffer}
@param value2 a direct or array-backed {@link java.nio.IntBuffer}
@param value3 a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetSource3i1(int source, int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSource3i(ALuint source, ALenum param, ALint * value1, ALint * value2, ALint * value3);
*/
public void alGetSource3i(int source, int param, int[] value1, int value1_offset, int[] value2, int value2_offset, int[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetSource3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3i"));
}
dispatch_alGetSource3i1(source, param, value1, Buffers.SIZEOF_INT * value1_offset, false, value2, Buffers.SIZEOF_INT * value2_offset, false, value3, Buffers.SIZEOF_INT * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcef(ALuint source, ALenum param, ALfloat * value);
@param value a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetSourcef(int source, int param, FloatBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alProcAddressTable._addressof_alGetSourcef;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcef"));
}
dispatch_alGetSourcef1(source, param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcef(ALuint source, ALenum param, ALfloat * value);
@param value a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetSourcef1(int source, int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourcef(ALuint source, ALenum param, ALfloat * value);
*/
public void alGetSourcef(int source, int param, float[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetSourcef;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcef"));
}
dispatch_alGetSourcef1(source, param, value, Buffers.SIZEOF_FLOAT * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcefv(ALuint source, ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetSourcefv(int source, int param, FloatBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetSourcefv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcefv"));
}
dispatch_alGetSourcefv1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcefv(ALuint source, ALenum param, ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetSourcefv1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourcefv(ALuint source, ALenum param, ALfloat * values);
*/
public void alGetSourcefv(int source, int param, float[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetSourcefv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcefv"));
}
dispatch_alGetSourcefv1(source, param, values, Buffers.SIZEOF_FLOAT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcei(ALuint source, ALenum param, ALint * value);
@param value a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetSourcei(int source, int param, IntBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alProcAddressTable._addressof_alGetSourcei;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcei"));
}
dispatch_alGetSourcei1(source, param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcei(ALuint source, ALenum param, ALint * value);
@param value a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetSourcei1(int source, int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourcei(ALuint source, ALenum param, ALint * value);
*/
public void alGetSourcei(int source, int param, int[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetSourcei;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcei"));
}
dispatch_alGetSourcei1(source, param, value, Buffers.SIZEOF_INT * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourceiv(ALuint source, ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetSourceiv(int source, int param, IntBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alGetSourceiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourceiv"));
}
dispatch_alGetSourceiv1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourceiv(ALuint source, ALenum param, ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetSourceiv1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourceiv(ALuint source, ALenum param, ALint * values);
*/
public void alGetSourceiv(int source, int param, int[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alGetSourceiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourceiv"));
}
dispatch_alGetSourceiv1(source, param, values, Buffers.SIZEOF_INT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
const ALchar * alGetString(ALenum param);
*/
public String alGetString(int param) {
final long __addr_ = alProcAddressTable._addressof_alGetString;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetString"));
}
return dispatch_alGetString1(param, __addr_);
}
/** Entry point (through function pointer) to C language function:
const ALchar * alGetString(ALenum param);
*/
private native String dispatch_alGetString1(int param, long procAddress);
/** Entry point (through function pointer) to C language function:
ALboolean alIsBuffer(ALuint buffer);
*/
public boolean alIsBuffer(int buffer) {
final long __addr_ = alProcAddressTable._addressof_alIsBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsBuffer"));
}
return dispatch_alIsBuffer1(buffer, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsBuffer(ALuint buffer);
*/
private native boolean dispatch_alIsBuffer1(int buffer, long procAddress);
/** Entry point (through function pointer) to C language function:
ALboolean alIsEnabled(ALenum capability);
*/
public boolean alIsEnabled(int capability) {
final long __addr_ = alProcAddressTable._addressof_alIsEnabled;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsEnabled"));
}
return dispatch_alIsEnabled1(capability, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsEnabled(ALenum capability);
*/
private native boolean dispatch_alIsEnabled1(int capability, long procAddress);
/** Entry point (through function pointer) to C language function:
ALboolean alIsExtensionPresent(const ALchar * extname);
*/
public boolean alIsExtensionPresent(String extname) {
final long __addr_ = alProcAddressTable._addressof_alIsExtensionPresent;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsExtensionPresent"));
}
return dispatch_alIsExtensionPresent1(extname, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsExtensionPresent(const ALchar * extname);
*/
private native boolean dispatch_alIsExtensionPresent1(String extname, long procAddress);
/** Entry point (through function pointer) to C language function:
ALboolean alIsSource(ALuint source);
*/
public boolean alIsSource(int source) {
final long __addr_ = alProcAddressTable._addressof_alIsSource;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsSource"));
}
return dispatch_alIsSource1(source, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsSource(ALuint source);
*/
private native boolean dispatch_alIsSource1(int source, long procAddress);
/** Entry point (through function pointer) to C language function:
void alListener3f(ALenum param, ALfloat value1, ALfloat value2, ALfloat value3);
*/
public void alListener3f(int param, float value1, float value2, float value3) {
final long __addr_ = alProcAddressTable._addressof_alListener3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListener3f"));
}
dispatch_alListener3f1(param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alListener3f(ALenum param, ALfloat value1, ALfloat value2, ALfloat value3);
*/
private native void dispatch_alListener3f1(int param, float value1, float value2, float value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alListener3i(ALenum param, ALint value1, ALint value2, ALint value3);
*/
public void alListener3i(int param, int value1, int value2, int value3) {
final long __addr_ = alProcAddressTable._addressof_alListener3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListener3i"));
}
dispatch_alListener3i1(param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alListener3i(ALenum param, ALint value1, ALint value2, ALint value3);
*/
private native void dispatch_alListener3i1(int param, int value1, int value2, int value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alListenerf(ALenum param, ALfloat value);
*/
public void alListenerf(int param, float value) {
final long __addr_ = alProcAddressTable._addressof_alListenerf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListenerf"));
}
dispatch_alListenerf1(param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alListenerf(ALenum param, ALfloat value);
*/
private native void dispatch_alListenerf1(int param, float value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alListenerfv(ALenum param, const ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
public void alListenerfv(int param, FloatBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alListenerfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListenerfv"));
}
dispatch_alListenerfv1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alListenerfv(ALenum param, const ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alListenerfv1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alListenerfv(ALenum param, const ALfloat * values);
*/
public void alListenerfv(int param, float[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alListenerfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListenerfv"));
}
dispatch_alListenerfv1(param, values, Buffers.SIZEOF_FLOAT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alListeneri(ALenum param, ALint value);
*/
public void alListeneri(int param, int value) {
final long __addr_ = alProcAddressTable._addressof_alListeneri;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListeneri"));
}
dispatch_alListeneri1(param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alListeneri(ALenum param, ALint value);
*/
private native void dispatch_alListeneri1(int param, int value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alListeneriv(ALenum param, const ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
public void alListeneriv(int param, IntBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alListeneriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListeneriv"));
}
dispatch_alListeneriv1(param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alListeneriv(ALenum param, const ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alListeneriv1(int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alListeneriv(ALenum param, const ALint * values);
*/
public void alListeneriv(int param, int[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alListeneriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alListeneriv"));
}
dispatch_alListeneriv1(param, values, Buffers.SIZEOF_INT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSource3f(ALuint source, ALenum param, ALfloat value1, ALfloat value2, ALfloat value3);
*/
public void alSource3f(int source, int param, float value1, float value2, float value3) {
final long __addr_ = alProcAddressTable._addressof_alSource3f;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSource3f"));
}
dispatch_alSource3f1(source, param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSource3f(ALuint source, ALenum param, ALfloat value1, ALfloat value2, ALfloat value3);
*/
private native void dispatch_alSource3f1(int source, int param, float value1, float value2, float value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSource3i(ALuint source, ALenum param, ALint value1, ALint value2, ALint value3);
*/
public void alSource3i(int source, int param, int value1, int value2, int value3) {
final long __addr_ = alProcAddressTable._addressof_alSource3i;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSource3i"));
}
dispatch_alSource3i1(source, param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSource3i(ALuint source, ALenum param, ALint value1, ALint value2, ALint value3);
*/
private native void dispatch_alSource3i1(int source, int param, int value1, int value2, int value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcePause(ALuint source);
*/
public void alSourcePause(int source) {
final long __addr_ = alProcAddressTable._addressof_alSourcePause;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcePause"));
}
dispatch_alSourcePause1(source, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcePause(ALuint source);
*/
private native void dispatch_alSourcePause1(int source, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcePausev(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
public void alSourcePausev(int n, IntBuffer sources) {
final boolean sources_is_direct = Buffers.isDirect(sources);
final long __addr_ = alProcAddressTable._addressof_alSourcePausev;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcePausev"));
}
dispatch_alSourcePausev1(n, sources_is_direct ? sources : Buffers.getArray(sources), sources_is_direct ? Buffers.getDirectBufferByteOffset(sources) : Buffers.getIndirectBufferByteOffset(sources), sources_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcePausev(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alSourcePausev1(int n, Object sources, int sources_byte_offset, boolean sources_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcePausev(ALsizei n, const ALuint * sources);
*/
public void alSourcePausev(int n, int[] sources, int sources_offset) {
if(sources != null && sources.length <= sources_offset)
throw new ALException("array offset argument \"sources_offset\" (" + sources_offset + ") equals or exceeds array length (" + sources.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourcePausev;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcePausev"));
}
dispatch_alSourcePausev1(n, sources, Buffers.SIZEOF_INT * sources_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcePlay(ALuint source);
*/
public void alSourcePlay(int source) {
final long __addr_ = alProcAddressTable._addressof_alSourcePlay;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcePlay"));
}
dispatch_alSourcePlay1(source, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcePlay(ALuint source);
*/
private native void dispatch_alSourcePlay1(int source, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcePlayv(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
public void alSourcePlayv(int n, IntBuffer sources) {
final boolean sources_is_direct = Buffers.isDirect(sources);
final long __addr_ = alProcAddressTable._addressof_alSourcePlayv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcePlayv"));
}
dispatch_alSourcePlayv1(n, sources_is_direct ? sources : Buffers.getArray(sources), sources_is_direct ? Buffers.getDirectBufferByteOffset(sources) : Buffers.getIndirectBufferByteOffset(sources), sources_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcePlayv(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alSourcePlayv1(int n, Object sources, int sources_byte_offset, boolean sources_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcePlayv(ALsizei n, const ALuint * sources);
*/
public void alSourcePlayv(int n, int[] sources, int sources_offset) {
if(sources != null && sources.length <= sources_offset)
throw new ALException("array offset argument \"sources_offset\" (" + sources_offset + ") equals or exceeds array length (" + sources.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourcePlayv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcePlayv"));
}
dispatch_alSourcePlayv1(n, sources, Buffers.SIZEOF_INT * sources_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceQueueBuffers(ALuint source, ALsizei nb, const ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
public void alSourceQueueBuffers(int source, int nb, IntBuffer buffers) {
final boolean buffers_is_direct = Buffers.isDirect(buffers);
final long __addr_ = alProcAddressTable._addressof_alSourceQueueBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceQueueBuffers"));
}
dispatch_alSourceQueueBuffers1(source, nb, buffers_is_direct ? buffers : Buffers.getArray(buffers), buffers_is_direct ? Buffers.getDirectBufferByteOffset(buffers) : Buffers.getIndirectBufferByteOffset(buffers), buffers_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceQueueBuffers(ALuint source, ALsizei nb, const ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alSourceQueueBuffers1(int source, int nb, Object buffers, int buffers_byte_offset, boolean buffers_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceQueueBuffers(ALuint source, ALsizei nb, const ALuint * buffers);
*/
public void alSourceQueueBuffers(int source, int nb, int[] buffers, int buffers_offset) {
if(buffers != null && buffers.length <= buffers_offset)
throw new ALException("array offset argument \"buffers_offset\" (" + buffers_offset + ") equals or exceeds array length (" + buffers.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourceQueueBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceQueueBuffers"));
}
dispatch_alSourceQueueBuffers1(source, nb, buffers, Buffers.SIZEOF_INT * buffers_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceRewind(ALuint source);
*/
public void alSourceRewind(int source) {
final long __addr_ = alProcAddressTable._addressof_alSourceRewind;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceRewind"));
}
dispatch_alSourceRewind1(source, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceRewind(ALuint source);
*/
private native void dispatch_alSourceRewind1(int source, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceRewindv(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
public void alSourceRewindv(int n, IntBuffer sources) {
final boolean sources_is_direct = Buffers.isDirect(sources);
final long __addr_ = alProcAddressTable._addressof_alSourceRewindv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceRewindv"));
}
dispatch_alSourceRewindv1(n, sources_is_direct ? sources : Buffers.getArray(sources), sources_is_direct ? Buffers.getDirectBufferByteOffset(sources) : Buffers.getIndirectBufferByteOffset(sources), sources_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceRewindv(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alSourceRewindv1(int n, Object sources, int sources_byte_offset, boolean sources_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceRewindv(ALsizei n, const ALuint * sources);
*/
public void alSourceRewindv(int n, int[] sources, int sources_offset) {
if(sources != null && sources.length <= sources_offset)
throw new ALException("array offset argument \"sources_offset\" (" + sources_offset + ") equals or exceeds array length (" + sources.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourceRewindv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceRewindv"));
}
dispatch_alSourceRewindv1(n, sources, Buffers.SIZEOF_INT * sources_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceStop(ALuint source);
*/
public void alSourceStop(int source) {
final long __addr_ = alProcAddressTable._addressof_alSourceStop;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceStop"));
}
dispatch_alSourceStop1(source, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceStop(ALuint source);
*/
private native void dispatch_alSourceStop1(int source, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceStopv(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
public void alSourceStopv(int n, IntBuffer sources) {
final boolean sources_is_direct = Buffers.isDirect(sources);
final long __addr_ = alProcAddressTable._addressof_alSourceStopv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceStopv"));
}
dispatch_alSourceStopv1(n, sources_is_direct ? sources : Buffers.getArray(sources), sources_is_direct ? Buffers.getDirectBufferByteOffset(sources) : Buffers.getIndirectBufferByteOffset(sources), sources_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceStopv(ALsizei n, const ALuint * sources);
@param sources a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alSourceStopv1(int n, Object sources, int sources_byte_offset, boolean sources_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceStopv(ALsizei n, const ALuint * sources);
*/
public void alSourceStopv(int n, int[] sources, int sources_offset) {
if(sources != null && sources.length <= sources_offset)
throw new ALException("array offset argument \"sources_offset\" (" + sources_offset + ") equals or exceeds array length (" + sources.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourceStopv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceStopv"));
}
dispatch_alSourceStopv1(n, sources, Buffers.SIZEOF_INT * sources_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceUnqueueBuffers(ALuint source, ALsizei nb, ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
public void alSourceUnqueueBuffers(int source, int nb, IntBuffer buffers) {
final boolean buffers_is_direct = Buffers.isDirect(buffers);
final long __addr_ = alProcAddressTable._addressof_alSourceUnqueueBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceUnqueueBuffers"));
}
dispatch_alSourceUnqueueBuffers1(source, nb, buffers_is_direct ? buffers : Buffers.getArray(buffers), buffers_is_direct ? Buffers.getDirectBufferByteOffset(buffers) : Buffers.getIndirectBufferByteOffset(buffers), buffers_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceUnqueueBuffers(ALuint source, ALsizei nb, ALuint * buffers);
@param buffers a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alSourceUnqueueBuffers1(int source, int nb, Object buffers, int buffers_byte_offset, boolean buffers_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceUnqueueBuffers(ALuint source, ALsizei nb, ALuint * buffers);
*/
public void alSourceUnqueueBuffers(int source, int nb, int[] buffers, int buffers_offset) {
if(buffers != null && buffers.length <= buffers_offset)
throw new ALException("array offset argument \"buffers_offset\" (" + buffers_offset + ") equals or exceeds array length (" + buffers.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourceUnqueueBuffers;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceUnqueueBuffers"));
}
dispatch_alSourceUnqueueBuffers1(source, nb, buffers, Buffers.SIZEOF_INT * buffers_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcef(ALuint source, ALenum param, ALfloat value);
*/
public void alSourcef(int source, int param, float value) {
final long __addr_ = alProcAddressTable._addressof_alSourcef;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcef"));
}
dispatch_alSourcef1(source, param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcef(ALuint source, ALenum param, ALfloat value);
*/
private native void dispatch_alSourcef1(int source, int param, float value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcefv(ALuint source, ALenum param, const ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
public void alSourcefv(int source, int param, FloatBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alSourcefv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcefv"));
}
dispatch_alSourcefv1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcefv(ALuint source, ALenum param, const ALfloat * values);
@param values a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alSourcefv1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcefv(ALuint source, ALenum param, const ALfloat * values);
*/
public void alSourcefv(int source, int param, float[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourcefv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcefv"));
}
dispatch_alSourcefv1(source, param, values, Buffers.SIZEOF_FLOAT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcei(ALuint source, ALenum param, ALint value);
*/
public void alSourcei(int source, int param, int value) {
final long __addr_ = alProcAddressTable._addressof_alSourcei;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcei"));
}
dispatch_alSourcei1(source, param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcei(ALuint source, ALenum param, ALint value);
*/
private native void dispatch_alSourcei1(int source, int param, int value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceiv(ALuint source, ALenum param, const ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
public void alSourceiv(int source, int param, IntBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alProcAddressTable._addressof_alSourceiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceiv"));
}
dispatch_alSourceiv1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourceiv(ALuint source, ALenum param, const ALint * values);
@param values a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alSourceiv1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourceiv(ALuint source, ALenum param, const ALint * values);
*/
public void alSourceiv(int source, int param, int[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alProcAddressTable._addressof_alSourceiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourceiv"));
}
dispatch_alSourceiv1(source, param, values, Buffers.SIZEOF_INT * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSpeedOfSound(ALfloat value);
*/
public void alSpeedOfSound(float value) {
final long __addr_ = alProcAddressTable._addressof_alSpeedOfSound;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSpeedOfSound"));
}
dispatch_alSpeedOfSound1(value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSpeedOfSound(ALfloat value);
*/
private native void dispatch_alSpeedOfSound1(float value, long procAddress);
// --- Begin CustomJavaCode .cfg declarations
static final DynamicLibraryBundle alDynamicLookupHelper;
private static final ALProcAddressTable alProcAddressTable;
static {
alProcAddressTable = new ALProcAddressTable();
if(null==alProcAddressTable) {
throw new RuntimeException("Couldn't instantiate ALProcAddressTable");
}
alDynamicLookupHelper = AccessController.doPrivileged(new PrivilegedAction() {
public DynamicLibraryBundle run() {
final DynamicLibraryBundle bundle = new DynamicLibraryBundle(new ALDynamicLibraryBundleInfo());
if(null==bundle) {
throw new RuntimeException("Null ALDynamicLookupHelper");
}
if(!bundle.isToolLibLoaded()) {
throw new RuntimeException("Couln't load native AL library");
}
if(!bundle.isLibComplete()) {
throw new RuntimeException("Couln't load native AL/JNI glue library");
}
alProcAddressTable.reset(bundle);
return bundle;
} } );
}
public static ALProcAddressTable getALProcAddressTable() { return alProcAddressTable; }
static long alGetProcAddress(long alGetProcAddressHandle, java.lang.String procname)
{
if (alGetProcAddressHandle == 0) {
throw new RuntimeException("Passed null pointer for method \"alGetProcAddress\"");
}
return dispatch_alGetProcAddressStatic(procname, alGetProcAddressHandle);
}
static native long dispatch_alGetProcAddressStatic(String fname, long procAddress);
// ---- End CustomJavaCode .cfg declarations
} // end of class ALImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy