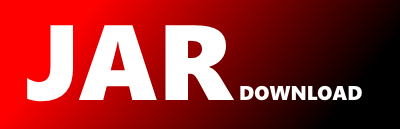
jogamp.openal.ALExtAbstractImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of joal Show documentation
Show all versions of joal Show documentation
Java™ Binding for the OpenAL® API
/* !---- DO NOT EDIT: This file autogenerated by com/jogamp/gluegen/procaddress/ProcAddressEmitter.java on Mon Sep 08 06:16:53 CEST 2014 ----! */
package jogamp.openal;
import java.io.UnsupportedEncodingException;
import java.util.*;
import com.jogamp.openal.*;
import jogamp.openal.*;
import java.security.AccessController;
import java.security.PrivilegedAction;
import com.jogamp.gluegen.runtime.*;
import com.jogamp.common.os.*;
import com.jogamp.common.nio.*;
import java.nio.*;
public abstract class ALExtAbstractImpl implements ALExt{
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotf(ALuint effectslot, ALenum param, ALfloat flValue)
*/
public void alAuxiliaryEffectSlotf(int effectslot, int param, float flValue) {
final long __addr_ = alExtProcAddressTable._addressof_alAuxiliaryEffectSlotf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alAuxiliaryEffectSlotf"));
}
dispatch_alAuxiliaryEffectSlotf1(effectslot, param, flValue, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotf(ALuint effectslot, ALenum param, ALfloat flValue)
*/
private native void dispatch_alAuxiliaryEffectSlotf1(int effectslot, int param, float flValue, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotfv(ALuint effectslot, ALenum param, const ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
public void alAuxiliaryEffectSlotfv(int effectslot, int param, FloatBuffer pflValues) {
final boolean pflValues_is_direct = Buffers.isDirect(pflValues);
final long __addr_ = alExtProcAddressTable._addressof_alAuxiliaryEffectSlotfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alAuxiliaryEffectSlotfv"));
}
dispatch_alAuxiliaryEffectSlotfv1(effectslot, param, pflValues_is_direct ? pflValues : Buffers.getArray(pflValues), pflValues_is_direct ? Buffers.getDirectBufferByteOffset(pflValues) : Buffers.getIndirectBufferByteOffset(pflValues), pflValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotfv(ALuint effectslot, ALenum param, const ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alAuxiliaryEffectSlotfv1(int effectslot, int param, Object pflValues, int pflValues_byte_offset, boolean pflValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotfv(ALuint effectslot, ALenum param, const ALfloat * pflValues)
*/
public void alAuxiliaryEffectSlotfv(int effectslot, int param, float[] pflValues, int pflValues_offset) {
if(pflValues != null && pflValues.length <= pflValues_offset)
throw new ALException("array offset argument \"pflValues_offset\" (" + pflValues_offset + ") equals or exceeds array length (" + pflValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alAuxiliaryEffectSlotfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alAuxiliaryEffectSlotfv"));
}
dispatch_alAuxiliaryEffectSlotfv1(effectslot, param, pflValues, Buffers.SIZEOF_FLOAT * pflValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSloti(ALuint effectslot, ALenum param, ALint iValue)
*/
public void alAuxiliaryEffectSloti(int effectslot, int param, int iValue) {
final long __addr_ = alExtProcAddressTable._addressof_alAuxiliaryEffectSloti;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alAuxiliaryEffectSloti"));
}
dispatch_alAuxiliaryEffectSloti1(effectslot, param, iValue, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSloti(ALuint effectslot, ALenum param, ALint iValue)
*/
private native void dispatch_alAuxiliaryEffectSloti1(int effectslot, int param, int iValue, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotiv(ALuint effectslot, ALenum param, const ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
public void alAuxiliaryEffectSlotiv(int effectslot, int param, IntBuffer piValues) {
final boolean piValues_is_direct = Buffers.isDirect(piValues);
final long __addr_ = alExtProcAddressTable._addressof_alAuxiliaryEffectSlotiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alAuxiliaryEffectSlotiv"));
}
dispatch_alAuxiliaryEffectSlotiv1(effectslot, param, piValues_is_direct ? piValues : Buffers.getArray(piValues), piValues_is_direct ? Buffers.getDirectBufferByteOffset(piValues) : Buffers.getIndirectBufferByteOffset(piValues), piValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotiv(ALuint effectslot, ALenum param, const ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alAuxiliaryEffectSlotiv1(int effectslot, int param, Object piValues, int piValues_byte_offset, boolean piValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alAuxiliaryEffectSlotiv(ALuint effectslot, ALenum param, const ALint * piValues)
*/
public void alAuxiliaryEffectSlotiv(int effectslot, int param, int[] piValues, int piValues_offset) {
if(piValues != null && piValues.length <= piValues_offset)
throw new ALException("array offset argument \"piValues_offset\" (" + piValues_offset + ") equals or exceeds array length (" + piValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alAuxiliaryEffectSlotiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alAuxiliaryEffectSlotiv"));
}
dispatch_alAuxiliaryEffectSlotiv1(effectslot, param, piValues, Buffers.SIZEOF_INT * piValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alBufferDataStatic(const ALint buffer, ALenum format, ALvoid * data, ALsizei len, ALsizei freq)
@param data a direct or array-backed {@link java.nio.Buffer} */
public void alBufferDataStatic(int buffer, int format, Buffer data, int len, int freq) {
final boolean data_is_direct = Buffers.isDirect(data);
final long __addr_ = alExtProcAddressTable._addressof_alBufferDataStatic;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferDataStatic"));
}
dispatch_alBufferDataStatic1(buffer, format, data_is_direct ? data : Buffers.getArray(data), data_is_direct ? Buffers.getDirectBufferByteOffset(data) : Buffers.getIndirectBufferByteOffset(data), data_is_direct, len, freq, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alBufferDataStatic(const ALint buffer, ALenum format, ALvoid * data, ALsizei len, ALsizei freq)
@param data a direct or array-backed {@link java.nio.Buffer} */
private native void dispatch_alBufferDataStatic1(int buffer, int format, Object data, int data_byte_offset, boolean data_is_direct, int len, int freq, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferSamplesSOFT(ALuint buffer, ALuint samplerate, ALenum internalformat, ALsizei samples, ALenum channels, ALenum type, const ALvoid * data)
@param data a direct or array-backed {@link java.nio.Buffer} */
public void alBufferSamplesSOFT(int buffer, int samplerate, int internalformat, int samples, int channels, int type, Buffer data) {
final boolean data_is_direct = Buffers.isDirect(data);
final long __addr_ = alExtProcAddressTable._addressof_alBufferSamplesSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferSamplesSOFT"));
}
dispatch_alBufferSamplesSOFT1(buffer, samplerate, internalformat, samples, channels, type, data_is_direct ? data : Buffers.getArray(data), data_is_direct ? Buffers.getDirectBufferByteOffset(data) : Buffers.getIndirectBufferByteOffset(data), data_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferSamplesSOFT(ALuint buffer, ALuint samplerate, ALenum internalformat, ALsizei samples, ALenum channels, ALenum type, const ALvoid * data)
@param data a direct or array-backed {@link java.nio.Buffer} */
private native void dispatch_alBufferSamplesSOFT1(int buffer, int samplerate, int internalformat, int samples, int channels, int type, Object data, int data_byte_offset, boolean data_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alBufferSubDataSOFT(ALuint buffer, ALenum format, const ALvoid * data, ALsizei offset, ALsizei length)
@param data a direct or array-backed {@link java.nio.Buffer} */
public void alBufferSubDataSOFT(int buffer, int format, Buffer data, int offset, int length) {
final boolean data_is_direct = Buffers.isDirect(data);
final long __addr_ = alExtProcAddressTable._addressof_alBufferSubDataSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferSubDataSOFT"));
}
dispatch_alBufferSubDataSOFT1(buffer, format, data_is_direct ? data : Buffers.getArray(data), data_is_direct ? Buffers.getDirectBufferByteOffset(data) : Buffers.getIndirectBufferByteOffset(data), data_is_direct, offset, length, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alBufferSubDataSOFT(ALuint buffer, ALenum format, const ALvoid * data, ALsizei offset, ALsizei length)
@param data a direct or array-backed {@link java.nio.Buffer} */
private native void dispatch_alBufferSubDataSOFT1(int buffer, int format, Object data, int data_byte_offset, boolean data_is_direct, int offset, int length, long procAddress);
/** Entry point (through function pointer) to C language function:
void alBufferSubSamplesSOFT(ALuint buffer, ALsizei offset, ALsizei samples, ALenum channels, ALenum type, const ALvoid * data)
@param data a direct or array-backed {@link java.nio.Buffer} */
public void alBufferSubSamplesSOFT(int buffer, int offset, int samples, int channels, int type, Buffer data) {
final boolean data_is_direct = Buffers.isDirect(data);
final long __addr_ = alExtProcAddressTable._addressof_alBufferSubSamplesSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alBufferSubSamplesSOFT"));
}
dispatch_alBufferSubSamplesSOFT1(buffer, offset, samples, channels, type, data_is_direct ? data : Buffers.getArray(data), data_is_direct ? Buffers.getDirectBufferByteOffset(data) : Buffers.getIndirectBufferByteOffset(data), data_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alBufferSubSamplesSOFT(ALuint buffer, ALsizei offset, ALsizei samples, ALenum channels, ALenum type, const ALvoid * data)
@param data a direct or array-backed {@link java.nio.Buffer} */
private native void dispatch_alBufferSubSamplesSOFT1(int buffer, int offset, int samples, int channels, int type, Object data, int data_byte_offset, boolean data_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteAuxiliaryEffectSlots(ALsizei n, const ALuint * effectslots)
@param effectslots a direct or array-backed {@link java.nio.IntBuffer} */
public void alDeleteAuxiliaryEffectSlots(int n, IntBuffer effectslots) {
final boolean effectslots_is_direct = Buffers.isDirect(effectslots);
final long __addr_ = alExtProcAddressTable._addressof_alDeleteAuxiliaryEffectSlots;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteAuxiliaryEffectSlots"));
}
dispatch_alDeleteAuxiliaryEffectSlots1(n, effectslots_is_direct ? effectslots : Buffers.getArray(effectslots), effectslots_is_direct ? Buffers.getDirectBufferByteOffset(effectslots) : Buffers.getIndirectBufferByteOffset(effectslots), effectslots_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteAuxiliaryEffectSlots(ALsizei n, const ALuint * effectslots)
@param effectslots a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alDeleteAuxiliaryEffectSlots1(int n, Object effectslots, int effectslots_byte_offset, boolean effectslots_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteAuxiliaryEffectSlots(ALsizei n, const ALuint * effectslots)
*/
public void alDeleteAuxiliaryEffectSlots(int n, int[] effectslots, int effectslots_offset) {
if(effectslots != null && effectslots.length <= effectslots_offset)
throw new ALException("array offset argument \"effectslots_offset\" (" + effectslots_offset + ") equals or exceeds array length (" + effectslots.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alDeleteAuxiliaryEffectSlots;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteAuxiliaryEffectSlots"));
}
dispatch_alDeleteAuxiliaryEffectSlots1(n, effectslots, Buffers.SIZEOF_INT * effectslots_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteEffects(ALsizei n, const ALuint * effects)
@param effects a direct or array-backed {@link java.nio.IntBuffer} */
public void alDeleteEffects(int n, IntBuffer effects) {
final boolean effects_is_direct = Buffers.isDirect(effects);
final long __addr_ = alExtProcAddressTable._addressof_alDeleteEffects;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteEffects"));
}
dispatch_alDeleteEffects1(n, effects_is_direct ? effects : Buffers.getArray(effects), effects_is_direct ? Buffers.getDirectBufferByteOffset(effects) : Buffers.getIndirectBufferByteOffset(effects), effects_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteEffects(ALsizei n, const ALuint * effects)
@param effects a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alDeleteEffects1(int n, Object effects, int effects_byte_offset, boolean effects_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteEffects(ALsizei n, const ALuint * effects)
*/
public void alDeleteEffects(int n, int[] effects, int effects_offset) {
if(effects != null && effects.length <= effects_offset)
throw new ALException("array offset argument \"effects_offset\" (" + effects_offset + ") equals or exceeds array length (" + effects.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alDeleteEffects;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteEffects"));
}
dispatch_alDeleteEffects1(n, effects, Buffers.SIZEOF_INT * effects_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteFilters(ALsizei n, const ALuint * filters)
@param filters a direct or array-backed {@link java.nio.IntBuffer} */
public void alDeleteFilters(int n, IntBuffer filters) {
final boolean filters_is_direct = Buffers.isDirect(filters);
final long __addr_ = alExtProcAddressTable._addressof_alDeleteFilters;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteFilters"));
}
dispatch_alDeleteFilters1(n, filters_is_direct ? filters : Buffers.getArray(filters), filters_is_direct ? Buffers.getDirectBufferByteOffset(filters) : Buffers.getIndirectBufferByteOffset(filters), filters_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteFilters(ALsizei n, const ALuint * filters)
@param filters a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alDeleteFilters1(int n, Object filters, int filters_byte_offset, boolean filters_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alDeleteFilters(ALsizei n, const ALuint * filters)
*/
public void alDeleteFilters(int n, int[] filters, int filters_offset) {
if(filters != null && filters.length <= filters_offset)
throw new ALException("array offset argument \"filters_offset\" (" + filters_offset + ") equals or exceeds array length (" + filters.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alDeleteFilters;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alDeleteFilters"));
}
dispatch_alDeleteFilters1(n, filters, Buffers.SIZEOF_INT * filters_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alEffectf(ALuint effect, ALenum param, ALfloat flValue)
*/
public void alEffectf(int effect, int param, float flValue) {
final long __addr_ = alExtProcAddressTable._addressof_alEffectf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alEffectf"));
}
dispatch_alEffectf1(effect, param, flValue, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alEffectf(ALuint effect, ALenum param, ALfloat flValue)
*/
private native void dispatch_alEffectf1(int effect, int param, float flValue, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alEffectfv(ALuint effect, ALenum param, const ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
public void alEffectfv(int effect, int param, FloatBuffer pflValues) {
final boolean pflValues_is_direct = Buffers.isDirect(pflValues);
final long __addr_ = alExtProcAddressTable._addressof_alEffectfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alEffectfv"));
}
dispatch_alEffectfv1(effect, param, pflValues_is_direct ? pflValues : Buffers.getArray(pflValues), pflValues_is_direct ? Buffers.getDirectBufferByteOffset(pflValues) : Buffers.getIndirectBufferByteOffset(pflValues), pflValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alEffectfv(ALuint effect, ALenum param, const ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alEffectfv1(int effect, int param, Object pflValues, int pflValues_byte_offset, boolean pflValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alEffectfv(ALuint effect, ALenum param, const ALfloat * pflValues)
*/
public void alEffectfv(int effect, int param, float[] pflValues, int pflValues_offset) {
if(pflValues != null && pflValues.length <= pflValues_offset)
throw new ALException("array offset argument \"pflValues_offset\" (" + pflValues_offset + ") equals or exceeds array length (" + pflValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alEffectfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alEffectfv"));
}
dispatch_alEffectfv1(effect, param, pflValues, Buffers.SIZEOF_FLOAT * pflValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alEffecti(ALuint effect, ALenum param, ALint iValue)
*/
public void alEffecti(int effect, int param, int iValue) {
final long __addr_ = alExtProcAddressTable._addressof_alEffecti;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alEffecti"));
}
dispatch_alEffecti1(effect, param, iValue, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alEffecti(ALuint effect, ALenum param, ALint iValue)
*/
private native void dispatch_alEffecti1(int effect, int param, int iValue, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alEffectiv(ALuint effect, ALenum param, const ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
public void alEffectiv(int effect, int param, IntBuffer piValues) {
final boolean piValues_is_direct = Buffers.isDirect(piValues);
final long __addr_ = alExtProcAddressTable._addressof_alEffectiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alEffectiv"));
}
dispatch_alEffectiv1(effect, param, piValues_is_direct ? piValues : Buffers.getArray(piValues), piValues_is_direct ? Buffers.getDirectBufferByteOffset(piValues) : Buffers.getIndirectBufferByteOffset(piValues), piValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alEffectiv(ALuint effect, ALenum param, const ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alEffectiv1(int effect, int param, Object piValues, int piValues_byte_offset, boolean piValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alEffectiv(ALuint effect, ALenum param, const ALint * piValues)
*/
public void alEffectiv(int effect, int param, int[] piValues, int piValues_offset) {
if(piValues != null && piValues.length <= piValues_offset)
throw new ALException("array offset argument \"piValues_offset\" (" + piValues_offset + ") equals or exceeds array length (" + piValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alEffectiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alEffectiv"));
}
dispatch_alEffectiv1(effect, param, piValues, Buffers.SIZEOF_INT * piValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alFilterf(ALuint filter, ALenum param, ALfloat flValue)
*/
public void alFilterf(int filter, int param, float flValue) {
final long __addr_ = alExtProcAddressTable._addressof_alFilterf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alFilterf"));
}
dispatch_alFilterf1(filter, param, flValue, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alFilterf(ALuint filter, ALenum param, ALfloat flValue)
*/
private native void dispatch_alFilterf1(int filter, int param, float flValue, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alFilterfv(ALuint filter, ALenum param, const ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
public void alFilterfv(int filter, int param, FloatBuffer pflValues) {
final boolean pflValues_is_direct = Buffers.isDirect(pflValues);
final long __addr_ = alExtProcAddressTable._addressof_alFilterfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alFilterfv"));
}
dispatch_alFilterfv1(filter, param, pflValues_is_direct ? pflValues : Buffers.getArray(pflValues), pflValues_is_direct ? Buffers.getDirectBufferByteOffset(pflValues) : Buffers.getIndirectBufferByteOffset(pflValues), pflValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alFilterfv(ALuint filter, ALenum param, const ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alFilterfv1(int filter, int param, Object pflValues, int pflValues_byte_offset, boolean pflValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alFilterfv(ALuint filter, ALenum param, const ALfloat * pflValues)
*/
public void alFilterfv(int filter, int param, float[] pflValues, int pflValues_offset) {
if(pflValues != null && pflValues.length <= pflValues_offset)
throw new ALException("array offset argument \"pflValues_offset\" (" + pflValues_offset + ") equals or exceeds array length (" + pflValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alFilterfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alFilterfv"));
}
dispatch_alFilterfv1(filter, param, pflValues, Buffers.SIZEOF_FLOAT * pflValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alFilteri(ALuint filter, ALenum param, ALint iValue)
*/
public void alFilteri(int filter, int param, int iValue) {
final long __addr_ = alExtProcAddressTable._addressof_alFilteri;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alFilteri"));
}
dispatch_alFilteri1(filter, param, iValue, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alFilteri(ALuint filter, ALenum param, ALint iValue)
*/
private native void dispatch_alFilteri1(int filter, int param, int iValue, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alFilteriv(ALuint filter, ALenum param, const ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
public void alFilteriv(int filter, int param, IntBuffer piValues) {
final boolean piValues_is_direct = Buffers.isDirect(piValues);
final long __addr_ = alExtProcAddressTable._addressof_alFilteriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alFilteriv"));
}
dispatch_alFilteriv1(filter, param, piValues_is_direct ? piValues : Buffers.getArray(piValues), piValues_is_direct ? Buffers.getDirectBufferByteOffset(piValues) : Buffers.getIndirectBufferByteOffset(piValues), piValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alFilteriv(ALuint filter, ALenum param, const ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alFilteriv1(int filter, int param, Object piValues, int piValues_byte_offset, boolean piValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alFilteriv(ALuint filter, ALenum param, const ALint * piValues)
*/
public void alFilteriv(int filter, int param, int[] piValues, int piValues_offset) {
if(piValues != null && piValues.length <= piValues_offset)
throw new ALException("array offset argument \"piValues_offset\" (" + piValues_offset + ") equals or exceeds array length (" + piValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alFilteriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alFilteriv"));
}
dispatch_alFilteriv1(filter, param, piValues, Buffers.SIZEOF_INT * piValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGenAuxiliaryEffectSlots(ALsizei n, ALuint * effectslots)
@param effectslots a direct or array-backed {@link java.nio.IntBuffer} */
public void alGenAuxiliaryEffectSlots(int n, IntBuffer effectslots) {
final boolean effectslots_is_direct = Buffers.isDirect(effectslots);
final long __addr_ = alExtProcAddressTable._addressof_alGenAuxiliaryEffectSlots;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenAuxiliaryEffectSlots"));
}
dispatch_alGenAuxiliaryEffectSlots1(n, effectslots_is_direct ? effectslots : Buffers.getArray(effectslots), effectslots_is_direct ? Buffers.getDirectBufferByteOffset(effectslots) : Buffers.getIndirectBufferByteOffset(effectslots), effectslots_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGenAuxiliaryEffectSlots(ALsizei n, ALuint * effectslots)
@param effectslots a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGenAuxiliaryEffectSlots1(int n, Object effectslots, int effectslots_byte_offset, boolean effectslots_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGenAuxiliaryEffectSlots(ALsizei n, ALuint * effectslots)
*/
public void alGenAuxiliaryEffectSlots(int n, int[] effectslots, int effectslots_offset) {
if(effectslots != null && effectslots.length <= effectslots_offset)
throw new ALException("array offset argument \"effectslots_offset\" (" + effectslots_offset + ") equals or exceeds array length (" + effectslots.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGenAuxiliaryEffectSlots;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenAuxiliaryEffectSlots"));
}
dispatch_alGenAuxiliaryEffectSlots1(n, effectslots, Buffers.SIZEOF_INT * effectslots_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGenEffects(ALsizei n, ALuint * effects)
@param effects a direct or array-backed {@link java.nio.IntBuffer} */
public void alGenEffects(int n, IntBuffer effects) {
final boolean effects_is_direct = Buffers.isDirect(effects);
final long __addr_ = alExtProcAddressTable._addressof_alGenEffects;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenEffects"));
}
dispatch_alGenEffects1(n, effects_is_direct ? effects : Buffers.getArray(effects), effects_is_direct ? Buffers.getDirectBufferByteOffset(effects) : Buffers.getIndirectBufferByteOffset(effects), effects_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGenEffects(ALsizei n, ALuint * effects)
@param effects a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGenEffects1(int n, Object effects, int effects_byte_offset, boolean effects_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGenEffects(ALsizei n, ALuint * effects)
*/
public void alGenEffects(int n, int[] effects, int effects_offset) {
if(effects != null && effects.length <= effects_offset)
throw new ALException("array offset argument \"effects_offset\" (" + effects_offset + ") equals or exceeds array length (" + effects.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGenEffects;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenEffects"));
}
dispatch_alGenEffects1(n, effects, Buffers.SIZEOF_INT * effects_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGenFilters(ALsizei n, ALuint * filters)
@param filters a direct or array-backed {@link java.nio.IntBuffer} */
public void alGenFilters(int n, IntBuffer filters) {
final boolean filters_is_direct = Buffers.isDirect(filters);
final long __addr_ = alExtProcAddressTable._addressof_alGenFilters;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenFilters"));
}
dispatch_alGenFilters1(n, filters_is_direct ? filters : Buffers.getArray(filters), filters_is_direct ? Buffers.getDirectBufferByteOffset(filters) : Buffers.getIndirectBufferByteOffset(filters), filters_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGenFilters(ALsizei n, ALuint * filters)
@param filters a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGenFilters1(int n, Object filters, int filters_byte_offset, boolean filters_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGenFilters(ALsizei n, ALuint * filters)
*/
public void alGenFilters(int n, int[] filters, int filters_offset) {
if(filters != null && filters.length <= filters_offset)
throw new ALException("array offset argument \"filters_offset\" (" + filters_offset + ") equals or exceeds array length (" + filters.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGenFilters;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGenFilters"));
}
dispatch_alGenFilters1(n, filters, Buffers.SIZEOF_INT * filters_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotf(ALuint effectslot, ALenum param, ALfloat * pflValue)
@param pflValue a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetAuxiliaryEffectSlotf(int effectslot, int param, FloatBuffer pflValue) {
final boolean pflValue_is_direct = Buffers.isDirect(pflValue);
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSlotf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSlotf"));
}
dispatch_alGetAuxiliaryEffectSlotf1(effectslot, param, pflValue_is_direct ? pflValue : Buffers.getArray(pflValue), pflValue_is_direct ? Buffers.getDirectBufferByteOffset(pflValue) : Buffers.getIndirectBufferByteOffset(pflValue), pflValue_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotf(ALuint effectslot, ALenum param, ALfloat * pflValue)
@param pflValue a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetAuxiliaryEffectSlotf1(int effectslot, int param, Object pflValue, int pflValue_byte_offset, boolean pflValue_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotf(ALuint effectslot, ALenum param, ALfloat * pflValue)
*/
public void alGetAuxiliaryEffectSlotf(int effectslot, int param, float[] pflValue, int pflValue_offset) {
if(pflValue != null && pflValue.length <= pflValue_offset)
throw new ALException("array offset argument \"pflValue_offset\" (" + pflValue_offset + ") equals or exceeds array length (" + pflValue.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSlotf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSlotf"));
}
dispatch_alGetAuxiliaryEffectSlotf1(effectslot, param, pflValue, Buffers.SIZEOF_FLOAT * pflValue_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotfv(ALuint effectslot, ALenum param, ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetAuxiliaryEffectSlotfv(int effectslot, int param, FloatBuffer pflValues) {
final boolean pflValues_is_direct = Buffers.isDirect(pflValues);
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSlotfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSlotfv"));
}
dispatch_alGetAuxiliaryEffectSlotfv1(effectslot, param, pflValues_is_direct ? pflValues : Buffers.getArray(pflValues), pflValues_is_direct ? Buffers.getDirectBufferByteOffset(pflValues) : Buffers.getIndirectBufferByteOffset(pflValues), pflValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotfv(ALuint effectslot, ALenum param, ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetAuxiliaryEffectSlotfv1(int effectslot, int param, Object pflValues, int pflValues_byte_offset, boolean pflValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotfv(ALuint effectslot, ALenum param, ALfloat * pflValues)
*/
public void alGetAuxiliaryEffectSlotfv(int effectslot, int param, float[] pflValues, int pflValues_offset) {
if(pflValues != null && pflValues.length <= pflValues_offset)
throw new ALException("array offset argument \"pflValues_offset\" (" + pflValues_offset + ") equals or exceeds array length (" + pflValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSlotfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSlotfv"));
}
dispatch_alGetAuxiliaryEffectSlotfv1(effectslot, param, pflValues, Buffers.SIZEOF_FLOAT * pflValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSloti(ALuint effectslot, ALenum param, ALint * piValue)
@param piValue a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetAuxiliaryEffectSloti(int effectslot, int param, IntBuffer piValue) {
final boolean piValue_is_direct = Buffers.isDirect(piValue);
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSloti;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSloti"));
}
dispatch_alGetAuxiliaryEffectSloti1(effectslot, param, piValue_is_direct ? piValue : Buffers.getArray(piValue), piValue_is_direct ? Buffers.getDirectBufferByteOffset(piValue) : Buffers.getIndirectBufferByteOffset(piValue), piValue_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSloti(ALuint effectslot, ALenum param, ALint * piValue)
@param piValue a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetAuxiliaryEffectSloti1(int effectslot, int param, Object piValue, int piValue_byte_offset, boolean piValue_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSloti(ALuint effectslot, ALenum param, ALint * piValue)
*/
public void alGetAuxiliaryEffectSloti(int effectslot, int param, int[] piValue, int piValue_offset) {
if(piValue != null && piValue.length <= piValue_offset)
throw new ALException("array offset argument \"piValue_offset\" (" + piValue_offset + ") equals or exceeds array length (" + piValue.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSloti;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSloti"));
}
dispatch_alGetAuxiliaryEffectSloti1(effectslot, param, piValue, Buffers.SIZEOF_INT * piValue_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotiv(ALuint effectslot, ALenum param, ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetAuxiliaryEffectSlotiv(int effectslot, int param, IntBuffer piValues) {
final boolean piValues_is_direct = Buffers.isDirect(piValues);
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSlotiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSlotiv"));
}
dispatch_alGetAuxiliaryEffectSlotiv1(effectslot, param, piValues_is_direct ? piValues : Buffers.getArray(piValues), piValues_is_direct ? Buffers.getDirectBufferByteOffset(piValues) : Buffers.getIndirectBufferByteOffset(piValues), piValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotiv(ALuint effectslot, ALenum param, ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetAuxiliaryEffectSlotiv1(int effectslot, int param, Object piValues, int piValues_byte_offset, boolean piValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetAuxiliaryEffectSlotiv(ALuint effectslot, ALenum param, ALint * piValues)
*/
public void alGetAuxiliaryEffectSlotiv(int effectslot, int param, int[] piValues, int piValues_offset) {
if(piValues != null && piValues.length <= piValues_offset)
throw new ALException("array offset argument \"piValues_offset\" (" + piValues_offset + ") equals or exceeds array length (" + piValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetAuxiliaryEffectSlotiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetAuxiliaryEffectSlotiv"));
}
dispatch_alGetAuxiliaryEffectSlotiv1(effectslot, param, piValues, Buffers.SIZEOF_INT * piValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferSamplesSOFT(ALuint buffer, ALsizei offset, ALsizei samples, ALenum channels, ALenum type, ALvoid * data)
@param data a direct or array-backed {@link java.nio.Buffer} */
public void alGetBufferSamplesSOFT(int buffer, int offset, int samples, int channels, int type, Buffer data) {
final boolean data_is_direct = Buffers.isDirect(data);
final long __addr_ = alExtProcAddressTable._addressof_alGetBufferSamplesSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetBufferSamplesSOFT"));
}
dispatch_alGetBufferSamplesSOFT1(buffer, offset, samples, channels, type, data_is_direct ? data : Buffers.getArray(data), data_is_direct ? Buffers.getDirectBufferByteOffset(data) : Buffers.getIndirectBufferByteOffset(data), data_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetBufferSamplesSOFT(ALuint buffer, ALsizei offset, ALsizei samples, ALenum channels, ALenum type, ALvoid * data)
@param data a direct or array-backed {@link java.nio.Buffer} */
private native void dispatch_alGetBufferSamplesSOFT1(int buffer, int offset, int samples, int channels, int type, Object data, int data_byte_offset, boolean data_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectf(ALuint effect, ALenum param, ALfloat * pflValue)
@param pflValue a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetEffectf(int effect, int param, FloatBuffer pflValue) {
final boolean pflValue_is_direct = Buffers.isDirect(pflValue);
final long __addr_ = alExtProcAddressTable._addressof_alGetEffectf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffectf"));
}
dispatch_alGetEffectf1(effect, param, pflValue_is_direct ? pflValue : Buffers.getArray(pflValue), pflValue_is_direct ? Buffers.getDirectBufferByteOffset(pflValue) : Buffers.getIndirectBufferByteOffset(pflValue), pflValue_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectf(ALuint effect, ALenum param, ALfloat * pflValue)
@param pflValue a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetEffectf1(int effect, int param, Object pflValue, int pflValue_byte_offset, boolean pflValue_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectf(ALuint effect, ALenum param, ALfloat * pflValue)
*/
public void alGetEffectf(int effect, int param, float[] pflValue, int pflValue_offset) {
if(pflValue != null && pflValue.length <= pflValue_offset)
throw new ALException("array offset argument \"pflValue_offset\" (" + pflValue_offset + ") equals or exceeds array length (" + pflValue.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetEffectf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffectf"));
}
dispatch_alGetEffectf1(effect, param, pflValue, Buffers.SIZEOF_FLOAT * pflValue_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectfv(ALuint effect, ALenum param, ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetEffectfv(int effect, int param, FloatBuffer pflValues) {
final boolean pflValues_is_direct = Buffers.isDirect(pflValues);
final long __addr_ = alExtProcAddressTable._addressof_alGetEffectfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffectfv"));
}
dispatch_alGetEffectfv1(effect, param, pflValues_is_direct ? pflValues : Buffers.getArray(pflValues), pflValues_is_direct ? Buffers.getDirectBufferByteOffset(pflValues) : Buffers.getIndirectBufferByteOffset(pflValues), pflValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectfv(ALuint effect, ALenum param, ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetEffectfv1(int effect, int param, Object pflValues, int pflValues_byte_offset, boolean pflValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectfv(ALuint effect, ALenum param, ALfloat * pflValues)
*/
public void alGetEffectfv(int effect, int param, float[] pflValues, int pflValues_offset) {
if(pflValues != null && pflValues.length <= pflValues_offset)
throw new ALException("array offset argument \"pflValues_offset\" (" + pflValues_offset + ") equals or exceeds array length (" + pflValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetEffectfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffectfv"));
}
dispatch_alGetEffectfv1(effect, param, pflValues, Buffers.SIZEOF_FLOAT * pflValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffecti(ALuint effect, ALenum param, ALint * piValue)
@param piValue a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetEffecti(int effect, int param, IntBuffer piValue) {
final boolean piValue_is_direct = Buffers.isDirect(piValue);
final long __addr_ = alExtProcAddressTable._addressof_alGetEffecti;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffecti"));
}
dispatch_alGetEffecti1(effect, param, piValue_is_direct ? piValue : Buffers.getArray(piValue), piValue_is_direct ? Buffers.getDirectBufferByteOffset(piValue) : Buffers.getIndirectBufferByteOffset(piValue), piValue_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffecti(ALuint effect, ALenum param, ALint * piValue)
@param piValue a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetEffecti1(int effect, int param, Object piValue, int piValue_byte_offset, boolean piValue_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffecti(ALuint effect, ALenum param, ALint * piValue)
*/
public void alGetEffecti(int effect, int param, int[] piValue, int piValue_offset) {
if(piValue != null && piValue.length <= piValue_offset)
throw new ALException("array offset argument \"piValue_offset\" (" + piValue_offset + ") equals or exceeds array length (" + piValue.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetEffecti;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffecti"));
}
dispatch_alGetEffecti1(effect, param, piValue, Buffers.SIZEOF_INT * piValue_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectiv(ALuint effect, ALenum param, ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetEffectiv(int effect, int param, IntBuffer piValues) {
final boolean piValues_is_direct = Buffers.isDirect(piValues);
final long __addr_ = alExtProcAddressTable._addressof_alGetEffectiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffectiv"));
}
dispatch_alGetEffectiv1(effect, param, piValues_is_direct ? piValues : Buffers.getArray(piValues), piValues_is_direct ? Buffers.getDirectBufferByteOffset(piValues) : Buffers.getIndirectBufferByteOffset(piValues), piValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectiv(ALuint effect, ALenum param, ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetEffectiv1(int effect, int param, Object piValues, int piValues_byte_offset, boolean piValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetEffectiv(ALuint effect, ALenum param, ALint * piValues)
*/
public void alGetEffectiv(int effect, int param, int[] piValues, int piValues_offset) {
if(piValues != null && piValues.length <= piValues_offset)
throw new ALException("array offset argument \"piValues_offset\" (" + piValues_offset + ") equals or exceeds array length (" + piValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetEffectiv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetEffectiv"));
}
dispatch_alGetEffectiv1(effect, param, piValues, Buffers.SIZEOF_INT * piValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilterf(ALuint filter, ALenum param, ALfloat * pflValue)
@param pflValue a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetFilterf(int filter, int param, FloatBuffer pflValue) {
final boolean pflValue_is_direct = Buffers.isDirect(pflValue);
final long __addr_ = alExtProcAddressTable._addressof_alGetFilterf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilterf"));
}
dispatch_alGetFilterf1(filter, param, pflValue_is_direct ? pflValue : Buffers.getArray(pflValue), pflValue_is_direct ? Buffers.getDirectBufferByteOffset(pflValue) : Buffers.getIndirectBufferByteOffset(pflValue), pflValue_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilterf(ALuint filter, ALenum param, ALfloat * pflValue)
@param pflValue a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetFilterf1(int filter, int param, Object pflValue, int pflValue_byte_offset, boolean pflValue_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilterf(ALuint filter, ALenum param, ALfloat * pflValue)
*/
public void alGetFilterf(int filter, int param, float[] pflValue, int pflValue_offset) {
if(pflValue != null && pflValue.length <= pflValue_offset)
throw new ALException("array offset argument \"pflValue_offset\" (" + pflValue_offset + ") equals or exceeds array length (" + pflValue.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetFilterf;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilterf"));
}
dispatch_alGetFilterf1(filter, param, pflValue, Buffers.SIZEOF_FLOAT * pflValue_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilterfv(ALuint filter, ALenum param, ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
public void alGetFilterfv(int filter, int param, FloatBuffer pflValues) {
final boolean pflValues_is_direct = Buffers.isDirect(pflValues);
final long __addr_ = alExtProcAddressTable._addressof_alGetFilterfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilterfv"));
}
dispatch_alGetFilterfv1(filter, param, pflValues_is_direct ? pflValues : Buffers.getArray(pflValues), pflValues_is_direct ? Buffers.getDirectBufferByteOffset(pflValues) : Buffers.getIndirectBufferByteOffset(pflValues), pflValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilterfv(ALuint filter, ALenum param, ALfloat * pflValues)
@param pflValues a direct or array-backed {@link java.nio.FloatBuffer} */
private native void dispatch_alGetFilterfv1(int filter, int param, Object pflValues, int pflValues_byte_offset, boolean pflValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilterfv(ALuint filter, ALenum param, ALfloat * pflValues)
*/
public void alGetFilterfv(int filter, int param, float[] pflValues, int pflValues_offset) {
if(pflValues != null && pflValues.length <= pflValues_offset)
throw new ALException("array offset argument \"pflValues_offset\" (" + pflValues_offset + ") equals or exceeds array length (" + pflValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetFilterfv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilterfv"));
}
dispatch_alGetFilterfv1(filter, param, pflValues, Buffers.SIZEOF_FLOAT * pflValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilteri(ALuint filter, ALenum param, ALint * piValue)
@param piValue a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetFilteri(int filter, int param, IntBuffer piValue) {
final boolean piValue_is_direct = Buffers.isDirect(piValue);
final long __addr_ = alExtProcAddressTable._addressof_alGetFilteri;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilteri"));
}
dispatch_alGetFilteri1(filter, param, piValue_is_direct ? piValue : Buffers.getArray(piValue), piValue_is_direct ? Buffers.getDirectBufferByteOffset(piValue) : Buffers.getIndirectBufferByteOffset(piValue), piValue_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilteri(ALuint filter, ALenum param, ALint * piValue)
@param piValue a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetFilteri1(int filter, int param, Object piValue, int piValue_byte_offset, boolean piValue_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilteri(ALuint filter, ALenum param, ALint * piValue)
*/
public void alGetFilteri(int filter, int param, int[] piValue, int piValue_offset) {
if(piValue != null && piValue.length <= piValue_offset)
throw new ALException("array offset argument \"piValue_offset\" (" + piValue_offset + ") equals or exceeds array length (" + piValue.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetFilteri;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilteri"));
}
dispatch_alGetFilteri1(filter, param, piValue, Buffers.SIZEOF_INT * piValue_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilteriv(ALuint filter, ALenum param, ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
public void alGetFilteriv(int filter, int param, IntBuffer piValues) {
final boolean piValues_is_direct = Buffers.isDirect(piValues);
final long __addr_ = alExtProcAddressTable._addressof_alGetFilteriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilteriv"));
}
dispatch_alGetFilteriv1(filter, param, piValues_is_direct ? piValues : Buffers.getArray(piValues), piValues_is_direct ? Buffers.getDirectBufferByteOffset(piValues) : Buffers.getIndirectBufferByteOffset(piValues), piValues_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilteriv(ALuint filter, ALenum param, ALint * piValues)
@param piValues a direct or array-backed {@link java.nio.IntBuffer} */
private native void dispatch_alGetFilteriv1(int filter, int param, Object piValues, int piValues_byte_offset, boolean piValues_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
ALvoid alGetFilteriv(ALuint filter, ALenum param, ALint * piValues)
*/
public void alGetFilteriv(int filter, int param, int[] piValues, int piValues_offset) {
if(piValues != null && piValues.length <= piValues_offset)
throw new ALException("array offset argument \"piValues_offset\" (" + piValues_offset + ") equals or exceeds array length (" + piValues.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetFilteriv;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetFilteriv"));
}
dispatch_alGetFilteriv1(filter, param, piValues, Buffers.SIZEOF_INT * piValues_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALCproc alGetProcAddress(const ALchar * fname)
*/
long alGetProcAddress(String fname) {
final long __addr_ = alExtProcAddressTable._addressof_alGetProcAddress;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetProcAddress"));
}
return dispatch_alGetProcAddress1(fname, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALCproc alGetProcAddress(const ALchar * fname)
*/
private native long dispatch_alGetProcAddress1(String fname, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSource3dSOFT(ALuint source, ALenum param, ALdouble * value1, ALdouble * value2, ALdouble * value3)
@param value1 a direct or array-backed {@link java.nio.DoubleBuffer}
@param value2 a direct or array-backed {@link java.nio.DoubleBuffer}
@param value3 a direct or array-backed {@link java.nio.DoubleBuffer} */
public void alGetSource3dSOFT(int source, int param, DoubleBuffer value1, DoubleBuffer value2, DoubleBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alExtProcAddressTable._addressof_alGetSource3dSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3dSOFT"));
}
dispatch_alGetSource3dSOFT1(source, param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSource3dSOFT(ALuint source, ALenum param, ALdouble * value1, ALdouble * value2, ALdouble * value3)
@param value1 a direct or array-backed {@link java.nio.DoubleBuffer}
@param value2 a direct or array-backed {@link java.nio.DoubleBuffer}
@param value3 a direct or array-backed {@link java.nio.DoubleBuffer} */
private native void dispatch_alGetSource3dSOFT1(int source, int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSource3dSOFT(ALuint source, ALenum param, ALdouble * value1, ALdouble * value2, ALdouble * value3)
*/
public void alGetSource3dSOFT(int source, int param, double[] value1, int value1_offset, double[] value2, int value2_offset, double[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetSource3dSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3dSOFT"));
}
dispatch_alGetSource3dSOFT1(source, param, value1, Buffers.SIZEOF_DOUBLE * value1_offset, false, value2, Buffers.SIZEOF_DOUBLE * value2_offset, false, value3, Buffers.SIZEOF_DOUBLE * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSource3i64SOFT(ALuint source, ALenum param, ALint64SOFT * value1, ALint64SOFT * value2, ALint64SOFT * value3)
@param value1 a direct or array-backed {@link java.nio.LongBuffer}
@param value2 a direct or array-backed {@link java.nio.LongBuffer}
@param value3 a direct or array-backed {@link java.nio.LongBuffer} */
public void alGetSource3i64SOFT(int source, int param, LongBuffer value1, LongBuffer value2, LongBuffer value3) {
final boolean value1_is_direct = Buffers.isDirect(value1);
final boolean value2_is_direct = Buffers.isDirect(value2);
final boolean value3_is_direct = Buffers.isDirect(value3);
final long __addr_ = alExtProcAddressTable._addressof_alGetSource3i64SOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3i64SOFT"));
}
dispatch_alGetSource3i64SOFT1(source, param, value1_is_direct ? value1 : Buffers.getArray(value1), value1_is_direct ? Buffers.getDirectBufferByteOffset(value1) : Buffers.getIndirectBufferByteOffset(value1), value1_is_direct, value2_is_direct ? value2 : Buffers.getArray(value2), value2_is_direct ? Buffers.getDirectBufferByteOffset(value2) : Buffers.getIndirectBufferByteOffset(value2), value2_is_direct, value3_is_direct ? value3 : Buffers.getArray(value3), value3_is_direct ? Buffers.getDirectBufferByteOffset(value3) : Buffers.getIndirectBufferByteOffset(value3), value3_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSource3i64SOFT(ALuint source, ALenum param, ALint64SOFT * value1, ALint64SOFT * value2, ALint64SOFT * value3)
@param value1 a direct or array-backed {@link java.nio.LongBuffer}
@param value2 a direct or array-backed {@link java.nio.LongBuffer}
@param value3 a direct or array-backed {@link java.nio.LongBuffer} */
private native void dispatch_alGetSource3i64SOFT1(int source, int param, Object value1, int value1_byte_offset, boolean value1_is_direct, Object value2, int value2_byte_offset, boolean value2_is_direct, Object value3, int value3_byte_offset, boolean value3_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSource3i64SOFT(ALuint source, ALenum param, ALint64SOFT * value1, ALint64SOFT * value2, ALint64SOFT * value3)
*/
public void alGetSource3i64SOFT(int source, int param, long[] value1, int value1_offset, long[] value2, int value2_offset, long[] value3, int value3_offset) {
if(value1 != null && value1.length <= value1_offset)
throw new ALException("array offset argument \"value1_offset\" (" + value1_offset + ") equals or exceeds array length (" + value1.length + ")");
if(value2 != null && value2.length <= value2_offset)
throw new ALException("array offset argument \"value2_offset\" (" + value2_offset + ") equals or exceeds array length (" + value2.length + ")");
if(value3 != null && value3.length <= value3_offset)
throw new ALException("array offset argument \"value3_offset\" (" + value3_offset + ") equals or exceeds array length (" + value3.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetSource3i64SOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSource3i64SOFT"));
}
dispatch_alGetSource3i64SOFT1(source, param, value1, Buffers.SIZEOF_LONG * value1_offset, false, value2, Buffers.SIZEOF_LONG * value2_offset, false, value3, Buffers.SIZEOF_LONG * value3_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcedSOFT(ALuint source, ALenum param, ALdouble * value)
@param value a direct or array-backed {@link java.nio.DoubleBuffer} */
public void alGetSourcedSOFT(int source, int param, DoubleBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcedSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcedSOFT"));
}
dispatch_alGetSourcedSOFT1(source, param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcedSOFT(ALuint source, ALenum param, ALdouble * value)
@param value a direct or array-backed {@link java.nio.DoubleBuffer} */
private native void dispatch_alGetSourcedSOFT1(int source, int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourcedSOFT(ALuint source, ALenum param, ALdouble * value)
*/
public void alGetSourcedSOFT(int source, int param, double[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcedSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcedSOFT"));
}
dispatch_alGetSourcedSOFT1(source, param, value, Buffers.SIZEOF_DOUBLE * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcedvSOFT(ALuint source, ALenum param, ALdouble * values)
@param values a direct or array-backed {@link java.nio.DoubleBuffer} */
public void alGetSourcedvSOFT(int source, int param, DoubleBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcedvSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcedvSOFT"));
}
dispatch_alGetSourcedvSOFT1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcedvSOFT(ALuint source, ALenum param, ALdouble * values)
@param values a direct or array-backed {@link java.nio.DoubleBuffer} */
private native void dispatch_alGetSourcedvSOFT1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourcedvSOFT(ALuint source, ALenum param, ALdouble * values)
*/
public void alGetSourcedvSOFT(int source, int param, double[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcedvSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcedvSOFT"));
}
dispatch_alGetSourcedvSOFT1(source, param, values, Buffers.SIZEOF_DOUBLE * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcei64SOFT(ALuint source, ALenum param, ALint64SOFT * value)
@param value a direct or array-backed {@link java.nio.LongBuffer} */
public void alGetSourcei64SOFT(int source, int param, LongBuffer value) {
final boolean value_is_direct = Buffers.isDirect(value);
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcei64SOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcei64SOFT"));
}
dispatch_alGetSourcei64SOFT1(source, param, value_is_direct ? value : Buffers.getArray(value), value_is_direct ? Buffers.getDirectBufferByteOffset(value) : Buffers.getIndirectBufferByteOffset(value), value_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcei64SOFT(ALuint source, ALenum param, ALint64SOFT * value)
@param value a direct or array-backed {@link java.nio.LongBuffer} */
private native void dispatch_alGetSourcei64SOFT1(int source, int param, Object value, int value_byte_offset, boolean value_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourcei64SOFT(ALuint source, ALenum param, ALint64SOFT * value)
*/
public void alGetSourcei64SOFT(int source, int param, long[] value, int value_offset) {
if(value != null && value.length <= value_offset)
throw new ALException("array offset argument \"value_offset\" (" + value_offset + ") equals or exceeds array length (" + value.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcei64SOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcei64SOFT"));
}
dispatch_alGetSourcei64SOFT1(source, param, value, Buffers.SIZEOF_LONG * value_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcei64vSOFT(ALuint source, ALenum param, ALint64SOFT * values)
@param values a direct or array-backed {@link java.nio.LongBuffer} */
public void alGetSourcei64vSOFT(int source, int param, LongBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcei64vSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcei64vSOFT"));
}
dispatch_alGetSourcei64vSOFT1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alGetSourcei64vSOFT(ALuint source, ALenum param, ALint64SOFT * values)
@param values a direct or array-backed {@link java.nio.LongBuffer} */
private native void dispatch_alGetSourcei64vSOFT1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alGetSourcei64vSOFT(ALuint source, ALenum param, ALint64SOFT * values)
*/
public void alGetSourcei64vSOFT(int source, int param, long[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alGetSourcei64vSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alGetSourcei64vSOFT"));
}
dispatch_alGetSourcei64vSOFT1(source, param, values, Buffers.SIZEOF_LONG * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsAuxiliaryEffectSlot(ALuint effectslot)
*/
public boolean alIsAuxiliaryEffectSlot(int effectslot) {
final long __addr_ = alExtProcAddressTable._addressof_alIsAuxiliaryEffectSlot;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsAuxiliaryEffectSlot"));
}
return dispatch_alIsAuxiliaryEffectSlot1(effectslot, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsAuxiliaryEffectSlot(ALuint effectslot)
*/
private native boolean dispatch_alIsAuxiliaryEffectSlot1(int effectslot, long procAddress);
/** Entry point (through function pointer) to C language function:
ALboolean alIsBufferFormatSupportedSOFT(ALenum format)
*/
public boolean alIsBufferFormatSupportedSOFT(int format) {
final long __addr_ = alExtProcAddressTable._addressof_alIsBufferFormatSupportedSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsBufferFormatSupportedSOFT"));
}
return dispatch_alIsBufferFormatSupportedSOFT1(format, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsBufferFormatSupportedSOFT(ALenum format)
*/
private native boolean dispatch_alIsBufferFormatSupportedSOFT1(int format, long procAddress);
/** Entry point (through function pointer) to C language function:
ALboolean alIsEffect(ALuint effect)
*/
public boolean alIsEffect(int effect) {
final long __addr_ = alExtProcAddressTable._addressof_alIsEffect;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsEffect"));
}
return dispatch_alIsEffect1(effect, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsEffect(ALuint effect)
*/
private native boolean dispatch_alIsEffect1(int effect, long procAddress);
/** Entry point (through function pointer) to C language function:
ALboolean alIsFilter(ALuint filter)
*/
public boolean alIsFilter(int filter) {
final long __addr_ = alExtProcAddressTable._addressof_alIsFilter;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alIsFilter"));
}
return dispatch_alIsFilter1(filter, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALboolean alIsFilter(ALuint filter)
*/
private native boolean dispatch_alIsFilter1(int filter, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSource3dSOFT(ALuint source, ALenum param, ALdouble value1, ALdouble value2, ALdouble value3)
*/
public void alSource3dSOFT(int source, int param, double value1, double value2, double value3) {
final long __addr_ = alExtProcAddressTable._addressof_alSource3dSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSource3dSOFT"));
}
dispatch_alSource3dSOFT1(source, param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSource3dSOFT(ALuint source, ALenum param, ALdouble value1, ALdouble value2, ALdouble value3)
*/
private native void dispatch_alSource3dSOFT1(int source, int param, double value1, double value2, double value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSource3i64SOFT(ALuint source, ALenum param, ALint64SOFT value1, ALint64SOFT value2, ALint64SOFT value3)
*/
public void alSource3i64SOFT(int source, int param, long value1, long value2, long value3) {
final long __addr_ = alExtProcAddressTable._addressof_alSource3i64SOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSource3i64SOFT"));
}
dispatch_alSource3i64SOFT1(source, param, value1, value2, value3, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSource3i64SOFT(ALuint source, ALenum param, ALint64SOFT value1, ALint64SOFT value2, ALint64SOFT value3)
*/
private native void dispatch_alSource3i64SOFT1(int source, int param, long value1, long value2, long value3, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcedSOFT(ALuint source, ALenum param, ALdouble value)
*/
public void alSourcedSOFT(int source, int param, double value) {
final long __addr_ = alExtProcAddressTable._addressof_alSourcedSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcedSOFT"));
}
dispatch_alSourcedSOFT1(source, param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcedSOFT(ALuint source, ALenum param, ALdouble value)
*/
private native void dispatch_alSourcedSOFT1(int source, int param, double value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcedvSOFT(ALuint source, ALenum param, const ALdouble * values)
@param values a direct or array-backed {@link java.nio.DoubleBuffer} */
public void alSourcedvSOFT(int source, int param, DoubleBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alExtProcAddressTable._addressof_alSourcedvSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcedvSOFT"));
}
dispatch_alSourcedvSOFT1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcedvSOFT(ALuint source, ALenum param, const ALdouble * values)
@param values a direct or array-backed {@link java.nio.DoubleBuffer} */
private native void dispatch_alSourcedvSOFT1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcedvSOFT(ALuint source, ALenum param, const ALdouble * values)
*/
public void alSourcedvSOFT(int source, int param, double[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alSourcedvSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcedvSOFT"));
}
dispatch_alSourcedvSOFT1(source, param, values, Buffers.SIZEOF_DOUBLE * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcei64SOFT(ALuint source, ALenum param, ALint64SOFT value)
*/
public void alSourcei64SOFT(int source, int param, long value) {
final long __addr_ = alExtProcAddressTable._addressof_alSourcei64SOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcei64SOFT"));
}
dispatch_alSourcei64SOFT1(source, param, value, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcei64SOFT(ALuint source, ALenum param, ALint64SOFT value)
*/
private native void dispatch_alSourcei64SOFT1(int source, int param, long value, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcei64vSOFT(ALuint source, ALenum param, const ALint64SOFT * values)
@param values a direct or array-backed {@link java.nio.LongBuffer} */
public void alSourcei64vSOFT(int source, int param, LongBuffer values) {
final boolean values_is_direct = Buffers.isDirect(values);
final long __addr_ = alExtProcAddressTable._addressof_alSourcei64vSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcei64vSOFT"));
}
dispatch_alSourcei64vSOFT1(source, param, values_is_direct ? values : Buffers.getArray(values), values_is_direct ? Buffers.getDirectBufferByteOffset(values) : Buffers.getIndirectBufferByteOffset(values), values_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alSourcei64vSOFT(ALuint source, ALenum param, const ALint64SOFT * values)
@param values a direct or array-backed {@link java.nio.LongBuffer} */
private native void dispatch_alSourcei64vSOFT1(int source, int param, Object values, int values_byte_offset, boolean values_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
void alSourcei64vSOFT(ALuint source, ALenum param, const ALint64SOFT * values)
*/
public void alSourcei64vSOFT(int source, int param, long[] values, int values_offset) {
if(values != null && values.length <= values_offset)
throw new ALException("array offset argument \"values_offset\" (" + values_offset + ") equals or exceeds array length (" + values.length + ")");
final long __addr_ = alExtProcAddressTable._addressof_alSourcei64vSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alSourcei64vSOFT"));
}
dispatch_alSourcei64vSOFT1(source, param, values, Buffers.SIZEOF_LONG * values_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALCproc alcGetProcAddress(ALCdevice * device, const ALCchar * funcname)
*/
long alcGetProcAddress(ALCdevice device, String funcname) {
final long __addr_ = alExtProcAddressTable._addressof_alcGetProcAddress;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alcGetProcAddress"));
}
return dispatch_alcGetProcAddress1(((device == null) ? null : device.getBuffer()), funcname, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALCproc alcGetProcAddress(ALCdevice * device, const ALCchar * funcname)
*/
private native long dispatch_alcGetProcAddress1(ByteBuffer device, String funcname, long procAddress);
/** Entry point (through function pointer) to C language function:
ALCcontext * alcGetThreadContext(void)
*/
public ALCcontext alcGetThreadContext() {
final long __addr_ = alExtProcAddressTable._addressof_alcGetThreadContext;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alcGetThreadContext"));
}
final ByteBuffer _res;
_res = dispatch_alcGetThreadContext1(__addr_);
if (_res == null) return null;
return ALCcontext.create(Buffers.nativeOrder(_res));
}
/** Entry point (through function pointer) to C language function:
ALCcontext * alcGetThreadContext(void)
*/
private native ByteBuffer dispatch_alcGetThreadContext1(long procAddress);
/** Entry point (through function pointer) to C language function:
ALCboolean alcIsRenderFormatSupportedSOFT(ALCdevice * device, ALCsizei freq, ALCenum channels, ALCenum type)
*/
public boolean alcIsRenderFormatSupportedSOFT(ALCdevice device, int freq, int channels, int type) {
final long __addr_ = alExtProcAddressTable._addressof_alcIsRenderFormatSupportedSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alcIsRenderFormatSupportedSOFT"));
}
return dispatch_alcIsRenderFormatSupportedSOFT1(((device == null) ? null : device.getBuffer()), freq, channels, type, __addr_);
}
/** Entry point (through function pointer) to C language function:
ALCboolean alcIsRenderFormatSupportedSOFT(ALCdevice * device, ALCsizei freq, ALCenum channels, ALCenum type)
*/
private native boolean dispatch_alcIsRenderFormatSupportedSOFT1(ByteBuffer device, int freq, int channels, int type, long procAddress);
/** Entry point (through function pointer) to C language function:
ALCdevice * alcLoopbackOpenDeviceSOFT(const ALCchar * deviceName)
*/
public ALCdevice alcLoopbackOpenDeviceSOFT(String deviceName) {
final long __addr_ = alExtProcAddressTable._addressof_alcLoopbackOpenDeviceSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alcLoopbackOpenDeviceSOFT"));
}
final ByteBuffer _res;
_res = dispatch_alcLoopbackOpenDeviceSOFT1(deviceName, __addr_);
if (_res == null) return null;
return ALCdevice.create(Buffers.nativeOrder(_res));
}
/** Entry point (through function pointer) to C language function:
ALCdevice * alcLoopbackOpenDeviceSOFT(const ALCchar * deviceName)
*/
private native ByteBuffer dispatch_alcLoopbackOpenDeviceSOFT1(String deviceName, long procAddress);
/** Entry point (through function pointer) to C language function:
void alcRenderSamplesSOFT(ALCdevice * device, ALCvoid * buffer, ALCsizei samples)
@param buffer a direct or array-backed {@link java.nio.Buffer} */
public void alcRenderSamplesSOFT(ALCdevice device, Buffer buffer, int samples) {
final boolean buffer_is_direct = Buffers.isDirect(buffer);
final long __addr_ = alExtProcAddressTable._addressof_alcRenderSamplesSOFT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alcRenderSamplesSOFT"));
}
dispatch_alcRenderSamplesSOFT1(((device == null) ? null : device.getBuffer()), buffer_is_direct ? buffer : Buffers.getArray(buffer), buffer_is_direct ? Buffers.getDirectBufferByteOffset(buffer) : Buffers.getIndirectBufferByteOffset(buffer), buffer_is_direct, samples, __addr_);
}
/** Entry point (through function pointer) to C language function:
void alcRenderSamplesSOFT(ALCdevice * device, ALCvoid * buffer, ALCsizei samples)
@param buffer a direct or array-backed {@link java.nio.Buffer} */
private native void dispatch_alcRenderSamplesSOFT1(ByteBuffer device, Object buffer, int buffer_byte_offset, boolean buffer_is_direct, int samples, long procAddress);
/** Entry point (through function pointer) to C language function:
ALCboolean alcSetThreadContext(ALCcontext * context)
*/
public boolean alcSetThreadContext(ALCcontext context) {
final long __addr_ = alExtProcAddressTable._addressof_alcSetThreadContext;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "alcSetThreadContext"));
}
return dispatch_alcSetThreadContext1(((context == null) ? null : context.getBuffer()), __addr_);
}
/** Entry point (through function pointer) to C language function:
ALCboolean alcSetThreadContext(ALCcontext * context)
*/
private native boolean dispatch_alcSetThreadContext1(ByteBuffer context, long procAddress);
// --- Begin CustomJavaCode .cfg declarations
private static final ALExtProcAddressTable alExtProcAddressTable;
static {
alExtProcAddressTable = AccessController.doPrivileged(new PrivilegedAction() {
public ALExtProcAddressTable run() {
final ALExtProcAddressTable alExtProcAddressTable = new ALExtProcAddressTable();
if(null==alExtProcAddressTable) {
throw new RuntimeException("Couldn't instantiate ALExtProcAddressTable");
}
alExtProcAddressTable.reset(ALImpl.alDynamicLookupHelper);
/** Not required nor forced
if( !initializeImpl() ) {
throw new RuntimeException("Initialization failure");
} */
return alExtProcAddressTable;
} } );
}
public static ALExtProcAddressTable getALExtProcAddressTable() { return alExtProcAddressTable; }
// ---- End CustomJavaCode .cfg declarations
} // end of class ALExtAbstractImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy