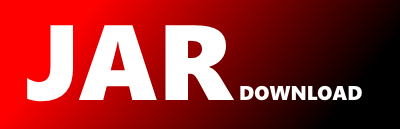
java.com.jogamp.opencl.llb.CLDeviceBinding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jocl-android Show documentation
Show all versions of jocl-android Show documentation
Java™ Binding for the OpenCL® API (Android runtime)
The newest version!
/* !---- DO NOT EDIT: This file autogenerated by com/jogamp/gluegen/JavaEmitter.java on Sat Oct 10 04:56:45 CEST 2015 ----! */
package com.jogamp.opencl.llb;
import com.jogamp.gluegen.runtime.*;
import com.jogamp.common.os.*;
import com.jogamp.common.nio.*;
import java.nio.*;
/**
* Java bindings to OpenCL devices.
* @author Michael Bien, GlueGen, et al.
*/
public interface CLDeviceBinding {
/** CType: int */
public static final int CL_DEVICE_IMAGE2D_MAX_HEIGHT = 0x1012;
/** CType: int */
public static final int CL_DEVICE_TYPE_GPU = ( 0x1 << 0x2 );
/** CType: int */
public static final int CL_DEVICE_IMAGE3D_MAX_HEIGHT = 0x1014;
/** CType: int */
public static final int CL_FP_INF_NAN = ( 0x1 << 0x1 );
/** CType: int */
public static final int CL_DEVICE_IMAGE3D_MAX_DEPTH = 0x1015;
/** CType: int */
public static final int CL_DEVICE_REFERENCE_COUNT_EXT = 0x4057;
/** CType: int */
public static final int CL_DEVICE_PREFERRED_VECTOR_WIDTH_DOUBLE = 0x100b;
/** CType: int */
public static final int CL_DEVICE_TYPE = 0x1000;
/** CType: int */
public static final int CL_DEVICE_PROFILING_TIMER_RESOLUTION = 0x1025;
/** CType: int */
public static final int CL_DEVICE_COMPUTE_CAPABILITY_MINOR_NV = 0x4001;
/** CType: int */
public static final int CL_DEVICE_MAX_COMPUTE_UNITS = 0x1002;
/** CType: int */
public static final int CL_DEVICE_KERNEL_EXEC_TIMEOUT_NV = 0x4005;
/** CType: int */
public static final int CL_DEVICE_PREFERRED_VECTOR_WIDTH_FLOAT = 0x100a;
/** CType: int */
public static final int CL_DEVICE_PREFERRED_VECTOR_WIDTH_LONG = 0x1009;
/** CType: int */
public static final int CL_DEVICE_GPU_OVERLAP_NV = 0x4004;
/** CType: int */
public static final int CL_DEVICE_PARTITION_TYPES_EXT = 0x4055;
/** CType: int */
public static final int CL_DEVICE_NATIVE_VECTOR_WIDTH_SHORT = 0x1037;
/** CType: int */
public static final int CL_DEVICE_LOCAL_MEM_TYPE = 0x1022;
/** CType: int */
public static final int CL_DEVICE_NATIVE_VECTOR_WIDTH_LONG = 0x1039;
/** CType: int */
public static final int CL_DEVICE_PARTITION_BY_NAMES_EXT = 0x4052;
/** CType: int */
public static final int CL_DEVICE_NATIVE_VECTOR_WIDTH_DOUBLE = 0x103b;
/** CType: int */
public static final int CL_DEVICE_VENDOR = 0x102c;
/** CType: int */
public static final int CL_DEVICE_MAX_WRITE_IMAGE_ARGS = 0x100f;
/** CType: int */
public static final int CL_LOCAL = 0x1;
/** CType: int */
public static final int CL_DEVICE_EXECUTION_CAPABILITIES = 0x1029;
/** CType: int */
public static final int CL_FP_DENORM = ( 0x1 << 0x0 );
/** CType: int */
public static final int CL_DEVICE_GLOBAL_MEM_CACHE_TYPE = 0x101c;
/** CType: int */
public static final int CL_DEVICE_MAX_SAMPLERS = 0x1018;
/** CType: int */
public static final int CL_DEVICE_HALF_FP_CONFIG = 0x1033;
/** CType: int */
public static final int CL_DEVICE_TYPE_ACCELERATOR = ( 0x1 << 0x3 );
/** CType: int */
public static final int CL_DEVICE_COMPILER_AVAILABLE = 0x1028;
/** CType: int */
public static final int CL_DEVICE_IMAGE2D_MAX_WIDTH = 0x1011;
/** CType: int */
public static final int CL_DEVICE_PLATFORM = 0x1031;
/** CType: int */
public static final int CL_DEVICE_MAX_MEM_ALLOC_SIZE = 0x1010;
/** CType: int */
public static final int CL_DEVICE_PARTITION_EQUALLY_EXT = 0x4050;
/** CType: int */
public static final int CL_DEVICE_NATIVE_VECTOR_WIDTH_FLOAT = 0x103a;
/** CType: int */
public static final int CL_DEVICE_PREFERRED_VECTOR_WIDTH_CHAR = 0x1006;
/** CType: int */
public static final int CL_DEVICE_MAX_CLOCK_FREQUENCY = 0x100c;
/** CType: int */
public static final int CL_FP_FMA = ( 0x1 << 0x5 );
/** CType: int */
public static final int CL_DEVICE_PARTITION_STYLE_EXT = 0x4058;
/** CType: int */
public static final int CL_DEVICE_NOT_AVAILABLE = -2;
/** CType: int */
public static final int CL_DEVICE_MAX_PARAMETER_SIZE = 0x1017;
/** CType: int */
public static final int CL_DEVICE_EXTENSIONS = 0x1030;
/** CType: int */
public static final int CL_DEVICE_PARTITION_BY_COUNTS_EXT = 0x4051;
/** CType: int */
public static final int CL_READ_ONLY_CACHE = 0x1;
/** CType: int */
public static final int CL_DEVICE_MAX_WORK_ITEM_SIZES = 0x1005;
/** CType: int */
public static final int CL_DEVICE_MAX_WORK_ITEM_DIMENSIONS = 0x1003;
/** CType: int */
public static final int CL_DEVICE_OPENCL_C_VERSION = 0x103d;
/** CType: int */
public static final int CL_DEVICE_NATIVE_VECTOR_WIDTH_CHAR = 0x1036;
/** CType: int */
public static final int CL_DEVICE_MAX_ATOMIC_COUNTERS_EXT = 0x4032;
/** CType: int */
public static final int CL_DEVICE_PARTITION_BY_AFFINITY_DOMAIN_EXT = 0x4053;
/** CType: int */
public static final int CL_FP_ROUND_TO_INF = ( 0x1 << 0x4 );
/** CType: int */
public static final int CL_DEVICE_MIN_DATA_TYPE_ALIGN_SIZE = 0x101a;
/** CType: int */
public static final int CL_DEVICE_MAX_CONSTANT_ARGS = 0x1021;
/** CType: int */
public static final int CL_DEVICE_GLOBAL_MEM_SIZE = 0x101f;
/** CType: int */
public static final int CL_DEVICE_ADDRESS_BITS = 0x100d;
/** CType: int */
public static final int CL_DEVICE_GLOBAL_MEM_CACHE_SIZE = 0x101e;
/** CType: int */
public static final int CL_FP_ROUND_TO_ZERO = ( 0x1 << 0x3 );
/** CType: int */
public static final int CL_DEVICE_WARP_SIZE_NV = 0x4003;
/** CType: int */
public static final int CL_DEVICE_IMAGE3D_MAX_WIDTH = 0x1013;
/** CType: int */
public static final int CL_DEVICE_NATIVE_VECTOR_WIDTH_INT = 0x1038;
/** CType: int */
public static final int CL_DEVICE_AVAILABLE = 0x1027;
/** CType: int */
public static final int CL_DEVICE_IMAGE_SUPPORT = 0x1016;
/** CType: int */
public static final int CL_DEVICE_PREFERRED_VECTOR_WIDTH_HALF = 0x1034;
/** CType: int */
public static final int CL_DEVICE_REGISTERS_PER_BLOCK_NV = 0x4002;
/** CType: int */
public static final int CL_DEVICE_PARENT_DEVICE_EXT = 0x4054;
/** CType: int */
public static final int CL_DEVICE_TYPE_DEFAULT = ( 0x1 << 0x0 );
/** CType: int */
public static final int CL_DEVICE_GLOBAL_MEM_CACHELINE_SIZE = 0x101d;
/** CType: int */
public static final int CL_READ_WRITE_CACHE = 0x2;
/** CType: int */
public static final int CL_DEVICE_LOCAL_MEM_SIZE = 0x1023;
/** CType: int */
public static final int CL_DEVICE_PROFILE = 0x102e;
/** CType: int */
public static final int CL_DEVICE_HOST_UNIFIED_MEMORY = 0x1035;
/** CType: int */
public static final int CL_FP_SOFT_FLOAT = ( 0x1 << 0x6 );
/** CType: int */
public static final int CL_DEVICE_COMPUTE_CAPABILITY_MAJOR_NV = 0x4000;
/** CType: long */
public static final long CL_DEVICE_TYPE_ALL = 0xffffffffL;
/** CType: int */
public static final int CL_DEVICE_DOUBLE_FP_CONFIG = 0x1032;
/** CType: int */
public static final int CL_DEVICE_NAME = 0x102b;
/** CType: int */
public static final int CL_DEVICE_TYPE_CPU = ( 0x1 << 0x1 );
/** CType: int */
public static final int CL_DEVICE_VERSION = 0x102f;
/** CType: int */
public static final int CL_DEVICE_SINGLE_FP_CONFIG = 0x101b;
/** CType: int */
public static final int CL_DEVICE_MEM_BASE_ADDR_ALIGN = 0x1019;
/** CType: int */
public static final int CL_DEVICE_PROFILING_TIMER_OFFSET_AMD = 0x4036;
/** CType: int */
public static final int CL_DEVICE_PREFERRED_VECTOR_WIDTH_SHORT = 0x1007;
/** CType: int */
public static final int CL_DEVICE_MAX_READ_IMAGE_ARGS = 0x100e;
/** CType: int */
public static final int CL_DEVICE_PARTITION_FAILED_EXT = -1057;
/** CType: int */
public static final int CL_DEVICE_NOT_FOUND = -1;
/** CType: int */
public static final int CL_DEVICE_AFFINITY_DOMAINS_EXT = 0x4056;
/** CType: int */
public static final int CL_DEVICE_PREFERRED_VECTOR_WIDTH_INT = 0x1008;
/** CType: int */
public static final int CL_DEVICE_QUEUE_PROPERTIES = 0x102a;
/** CType: int */
public static final int CL_DEVICE_MAX_CONSTANT_BUFFER_SIZE = 0x1020;
/** CType: int */
public static final int CL_DEVICE_MAX_WORK_GROUP_SIZE = 0x1004;
/** CType: int */
public static final int CL_GLOBAL = 0x2;
/** CType: int */
public static final int CL_DEVICE_VENDOR_ID = 0x1001;
/** CType: int */
public static final int CL_FP_ROUND_TO_NEAREST = ( 0x1 << 0x2 );
/** CType: int */
public static final int CL_DEVICE_ERROR_CORRECTION_SUPPORT = 0x1024;
/** CType: int */
public static final int CL_DEVICE_ENDIAN_LITTLE = 0x1026;
/** CType: int */
public static final int CL_DEVICE_NATIVE_VECTOR_WIDTH_HALF = 0x103c;
/** CType: int */
public static final int CL_DEVICE_INTEGRATED_MEMORY_NV = 0x4006;
/** Interface to C language function:
cl_int {@native clGetDeviceInfo}(cl_device_id device, cl_device_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetDeviceInfo(long device, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret);
/** Interface to C language function:
cl_int {@native clReleaseDeviceEXT}(cl_device_id)
*/
public int clReleaseDeviceEXT(long arg0);
/** Interface to C language function:
cl_int {@native clRetainDeviceEXT}(cl_device_id)
*/
public int clRetainDeviceEXT(long arg0);
/** Interface to C language function:
cl_int {@native clCreateSubDevicesEXT}(cl_device_id, const cl_device_partition_property_ext * , cl_uint, cl_device_id * , cl_uint * )
@param arg1 a direct or array-backed {@link java.nio.LongBuffer}
@param arg3 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct or array-backed {@link java.nio.IntBuffer} */
public int clCreateSubDevicesEXT(long arg0, LongBuffer arg1, int arg2, PointerBuffer arg3, IntBuffer arg4);
/** Interface to C language function:
cl_int {@native clCreateSubDevicesEXT}(cl_device_id, const cl_device_partition_property_ext * , cl_uint, cl_device_id * , cl_uint * )
@param arg3 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer} */
public int clCreateSubDevicesEXT(long arg0, long[] arg1, int arg1_offset, int arg2, PointerBuffer arg3, int[] arg4, int arg4_offset);
} // end of class CLDeviceBinding
© 2015 - 2025 Weber Informatics LLC | Privacy Policy