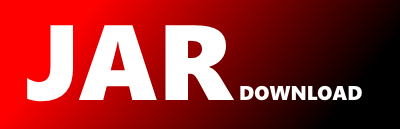
java.com.jogamp.opencl.llb.gl.CLGL Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jocl-android Show documentation
Show all versions of jocl-android Show documentation
Java™ Binding for the OpenCL® API (Android runtime)
The newest version!
/* !---- DO NOT EDIT: This file autogenerated by com/jogamp/gluegen/JavaEmitter.java on Sat Oct 10 04:57:51 CEST 2015 ----! */
package com.jogamp.opencl.llb.gl;
import com.jogamp.opencl.llb.CL;
import com.jogamp.gluegen.runtime.*;
import com.jogamp.common.os.*;
import com.jogamp.common.nio.*;
import java.nio.*;
/**
* Java bindings to OpenCL, the Open Computing Language.
* OpenGL - OpenCL interoperability.
* @author Michael Bien, GlueGen, et al.
*/
public interface CLGL extends CL{
/** CType: int */
public static final int CL_DEVICES_FOR_GL_CONTEXT_KHR = 0x2007;
/** CType: int */
public static final int CL_COMMAND_ACQUIRE_GL_OBJECTS = 0x11ff;
/** CType: int */
public static final int CL_GL_OBJECT_RENDERBUFFER = 0x2003;
/** CType: int */
public static final int CL_GL_MIPMAP_LEVEL = 0x2005;
/** CType: int */
public static final int CL_GL_TEXTURE_TARGET = 0x2004;
/** CType: int */
public static final int CL_COMMAND_GL_FENCE_SYNC_OBJECT_KHR = 0x200d;
/** CType: int */
public static final int CL_CURRENT_DEVICE_FOR_GL_CONTEXT_KHR = 0x2006;
/** CType: int */
public static final int CL_GL_OBJECT_BUFFER = 0x2000;
/** CType: int */
public static final int CL_GL_CONTEXT_KHR = 0x2008;
/** CType: int */
public static final int CL_INVALID_GL_SHAREGROUP_REFERENCE_KHR = -1000;
/** CType: int */
public static final int CL_INVALID_GL_OBJECT = -60;
/** CType: int */
public static final int CL_COMMAND_RELEASE_GL_OBJECTS = 0x1200;
/** CType: int */
public static final int CL_GL_OBJECT_TEXTURE2D = 0x2001;
/** CType: int */
public static final int CL_GL_OBJECT_TEXTURE3D = 0x2002;
/** Interface to C language function:
cl_mem {@native clCreateFromGLBuffer}(cl_context context, cl_mem_flags flags, cl_GLuint bufobj, int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLBuffer(long context, long flags, int bufobj, IntBuffer errcode_ret);
/** Interface to C language function:
cl_mem {@native clCreateFromGLBuffer}(cl_context context, cl_mem_flags flags, cl_GLuint bufobj, int * errcode_ret)
*/
public long clCreateFromGLBuffer(long context, long flags, int bufobj, int[] errcode_ret, int errcode_ret_offset);
/** Interface to C language function:
cl_mem {@native clCreateFromGLTexture2D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLTexture2D(long context, long flags, int target, int miplevel, int texture, IntBuffer errcode_ret);
/** Interface to C language function:
cl_mem {@native clCreateFromGLTexture2D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
*/
public long clCreateFromGLTexture2D(long context, long flags, int target, int miplevel, int texture, int[] errcode_ret, int errcode_ret_offset);
/** Interface to C language function:
cl_mem {@native clCreateFromGLTexture3D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLTexture3D(long context, long flags, int target, int miplevel, int texture, IntBuffer errcode_ret);
/** Interface to C language function:
cl_mem {@native clCreateFromGLTexture3D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
*/
public long clCreateFromGLTexture3D(long context, long flags, int target, int miplevel, int texture, int[] errcode_ret, int errcode_ret_offset);
/** Interface to C language function:
cl_mem {@native clCreateFromGLRenderbuffer}(cl_context context, cl_mem_flags flags, cl_GLuint renderbuffer, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLRenderbuffer(long context, long flags, int renderbuffer, IntBuffer errcode_ret);
/** Interface to C language function:
cl_mem {@native clCreateFromGLRenderbuffer}(cl_context context, cl_mem_flags flags, cl_GLuint renderbuffer, cl_int * errcode_ret)
*/
public long clCreateFromGLRenderbuffer(long context, long flags, int renderbuffer, int[] errcode_ret, int errcode_ret_offset);
/** Interface to C language function:
cl_int {@native clGetGLObjectInfo}(cl_mem memobj, cl_gl_object_type * gl_object_type, cl_GLuint * gl_object_name)
@param gl_object_type a direct or array-backed {@link java.nio.IntBuffer}
@param gl_object_name a direct or array-backed {@link java.nio.IntBuffer} */
public int clGetGLObjectInfo(long memobj, IntBuffer gl_object_type, IntBuffer gl_object_name);
/** Interface to C language function:
cl_int {@native clGetGLObjectInfo}(cl_mem memobj, cl_gl_object_type * gl_object_type, cl_GLuint * gl_object_name)
*/
public int clGetGLObjectInfo(long memobj, int[] gl_object_type, int gl_object_type_offset, int[] gl_object_name, int gl_object_name_offset);
/** Interface to C language function:
cl_int {@native clGetGLTextureInfo}(cl_mem memobj, cl_gl_texture_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct or array-backed {@link java.nio.Buffer}
@param param_value_size_ret a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetGLTextureInfo(long memobj, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret);
/** Interface to C language function:
cl_int {@native clEnqueueAcquireGLObjects}(cl_command_queue command_queue, cl_uint num_objects, const cl_mem * mem_objects, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mem_objects a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueAcquireGLObjects(long command_queue, int num_objects, PointerBuffer mem_objects, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event);
/** Interface to C language function:
cl_int {@native clEnqueueReleaseGLObjects}(cl_command_queue command_queue, cl_uint num_objects, const cl_mem * mem_objects, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mem_objects a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueReleaseGLObjects(long command_queue, int num_objects, PointerBuffer mem_objects, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event);
/** Interface to C language function:
cl_int {@native clGetGLContextInfoKHR}(const cl_context_properties * properties, cl_gl_context_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param properties a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetGLContextInfoKHR(PointerBuffer properties, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret);
/** Interface to C language function:
cl_event {@native clCreateEventFromGLsyncKHR}(cl_context, cl_GLsync, cl_int * )
@param arg2 a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateEventFromGLsyncKHR(long arg0, long arg1, IntBuffer arg2);
/** Interface to C language function:
cl_event {@native clCreateEventFromGLsyncKHR}(cl_context, cl_GLsync, cl_int * )
*/
public long clCreateEventFromGLsyncKHR(long arg0, long arg1, int[] arg2, int arg2_offset);
// --- Begin CustomJavaCode .cfg declarations
/** To be used on Mac OSX instead of {@link #CL_CGL_SHAREGROUP_KHR}}. FIXME: For all Mac OSX versions? */
public static final int CL_CONTEXT_PROPERTY_USE_CGL_SHAREGROUP_APPLE = 0x10000000;
// ---- End CustomJavaCode .cfg declarations
} // end of class CLGL
© 2015 - 2025 Weber Informatics LLC | Privacy Policy