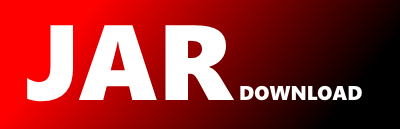
java.com.jogamp.opencl.llb.impl.CLAbstractImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jocl-android Show documentation
Show all versions of jocl-android Show documentation
Java™ Binding for the OpenCL® API (Android runtime)
The newest version!
/* !---- DO NOT EDIT: This file autogenerated by com/jogamp/gluegen/procaddress/ProcAddressEmitter.java on Sat Oct 10 04:58:01 CEST 2015 ----! */
package com.jogamp.opencl.llb.impl;
import com.jogamp.opencl.llb.gl.CLGL;
import java.security.AccessController;
import java.security.PrivilegedAction;
import com.jogamp.gluegen.runtime.*;
import com.jogamp.common.os.*;
import com.jogamp.common.nio.*;
import java.nio.*;
/**
* Java bindings to OpenCL, the Open Computing Language (generated).
* @author Michael Bien, GlueGen, et al.
*/
public abstract class CLAbstractImpl implements CLGL{
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetPlatformIDs}(cl_uint num_entries, cl_platform_id * platforms, cl_uint * num_platforms)
@param platforms a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param num_platforms a direct only {@link java.nio.IntBuffer} */
public int clGetPlatformIDs(int num_entries, PointerBuffer platforms, IntBuffer num_platforms) {
if (!Buffers.isDirect(platforms))
throw new IllegalArgumentException("Argument \"platforms\" is not a direct buffer");
if (!Buffers.isDirect(num_platforms))
throw new IllegalArgumentException("Argument \"num_platforms\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetPlatformIDs;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetPlatformIDs"));
}
return dispatch_clGetPlatformIDs0(num_entries, platforms != null ? platforms.getBuffer() : null, Buffers.getDirectBufferByteOffset(platforms), num_platforms, Buffers.getDirectBufferByteOffset(num_platforms), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetPlatformIDs}(cl_uint num_entries, cl_platform_id * platforms, cl_uint * num_platforms)
@param platforms a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param num_platforms a direct only {@link java.nio.IntBuffer} */
private native int dispatch_clGetPlatformIDs0(int num_entries, Object platforms, int platforms_byte_offset, Object num_platforms, int num_platforms_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetPlatformInfo}(cl_platform_id platform, cl_platform_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetPlatformInfo(long platform, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetPlatformInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetPlatformInfo"));
}
return dispatch_clGetPlatformInfo0(platform, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetPlatformInfo}(cl_platform_id platform, cl_platform_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetPlatformInfo0(long platform, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetDeviceIDs}(cl_platform_id platform, cl_device_type device_type, cl_uint num_entries, cl_device_id * devices, cl_uint * num_devices)
@param devices a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param num_devices a direct only {@link java.nio.IntBuffer} */
public int clGetDeviceIDs(long platform, long device_type, int num_entries, PointerBuffer devices, IntBuffer num_devices) {
if (!Buffers.isDirect(devices))
throw new IllegalArgumentException("Argument \"devices\" is not a direct buffer");
if (!Buffers.isDirect(num_devices))
throw new IllegalArgumentException("Argument \"num_devices\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetDeviceIDs;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetDeviceIDs"));
}
return dispatch_clGetDeviceIDs0(platform, device_type, num_entries, devices != null ? devices.getBuffer() : null, Buffers.getDirectBufferByteOffset(devices), num_devices, Buffers.getDirectBufferByteOffset(num_devices), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetDeviceIDs}(cl_platform_id platform, cl_device_type device_type, cl_uint num_entries, cl_device_id * devices, cl_uint * num_devices)
@param devices a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param num_devices a direct only {@link java.nio.IntBuffer} */
private native int dispatch_clGetDeviceIDs0(long platform, long device_type, int num_entries, Object devices, int devices_byte_offset, Object num_devices, int num_devices_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetDeviceInfo}(cl_device_id device, cl_device_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetDeviceInfo(long device, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetDeviceInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetDeviceInfo"));
}
return dispatch_clGetDeviceInfo0(device, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetDeviceInfo}(cl_device_id device, cl_device_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetDeviceInfo0(long device, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainContext}(cl_context context)
*/
public int clRetainContext(long context) {
final long __addr_ = addressTable._addressof_clRetainContext;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainContext"));
}
return dispatch_clRetainContext1(context, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainContext}(cl_context context)
*/
private native int dispatch_clRetainContext1(long context, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetContextInfo}(cl_context context, cl_context_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetContextInfo(long context, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetContextInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetContextInfo"));
}
return dispatch_clGetContextInfo0(context, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetContextInfo}(cl_context context, cl_context_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetContextInfo0(long context, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_command_queue {@native clCreateCommandQueue}(cl_context context, cl_device_id device, cl_command_queue_properties properties, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateCommandQueue(long context, long device, long properties, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateCommandQueue;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateCommandQueue"));
}
return dispatch_clCreateCommandQueue1(context, device, properties, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_command_queue {@native clCreateCommandQueue}(cl_context context, cl_device_id device, cl_command_queue_properties properties, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateCommandQueue1(long context, long device, long properties, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_command_queue {@native clCreateCommandQueue}(cl_context context, cl_device_id device, cl_command_queue_properties properties, cl_int * errcode_ret)
*/
public long clCreateCommandQueue(long context, long device, long properties, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateCommandQueue;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateCommandQueue"));
}
return dispatch_clCreateCommandQueue1(context, device, properties, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainCommandQueue}(cl_command_queue command_queue)
*/
public int clRetainCommandQueue(long command_queue) {
final long __addr_ = addressTable._addressof_clRetainCommandQueue;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainCommandQueue"));
}
return dispatch_clRetainCommandQueue1(command_queue, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainCommandQueue}(cl_command_queue command_queue)
*/
private native int dispatch_clRetainCommandQueue1(long command_queue, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseCommandQueue}(cl_command_queue command_queue)
*/
public int clReleaseCommandQueue(long command_queue) {
final long __addr_ = addressTable._addressof_clReleaseCommandQueue;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clReleaseCommandQueue"));
}
return dispatch_clReleaseCommandQueue1(command_queue, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseCommandQueue}(cl_command_queue command_queue)
*/
private native int dispatch_clReleaseCommandQueue1(long command_queue, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetCommandQueueInfo}(cl_command_queue command_queue, cl_command_queue_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetCommandQueueInfo(long command_queue, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetCommandQueueInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetCommandQueueInfo"));
}
return dispatch_clGetCommandQueueInfo0(command_queue, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetCommandQueueInfo}(cl_command_queue command_queue, cl_command_queue_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetCommandQueueInfo0(long command_queue, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateBuffer}(cl_context context, cl_mem_flags flags, size_t size, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateBuffer(long context, long flags, long size, Buffer host_ptr, IntBuffer errcode_ret) {
final boolean host_ptr_is_direct = Buffers.isDirect(host_ptr);
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateBuffer"));
}
return dispatch_clCreateBuffer1(context, flags, size, host_ptr_is_direct ? host_ptr : Buffers.getArray(host_ptr), host_ptr_is_direct ? Buffers.getDirectBufferByteOffset(host_ptr) : Buffers.getIndirectBufferByteOffset(host_ptr), host_ptr_is_direct, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateBuffer}(cl_context context, cl_mem_flags flags, size_t size, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateBuffer1(long context, long flags, long size, Object host_ptr, int host_ptr_byte_offset, boolean host_ptr_is_direct, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateBuffer}(cl_context context, cl_mem_flags flags, size_t size, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer} */
public long clCreateBuffer(long context, long flags, long size, Buffer host_ptr, int[] errcode_ret, int errcode_ret_offset) {
final boolean host_ptr_is_direct = Buffers.isDirect(host_ptr);
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateBuffer"));
}
return dispatch_clCreateBuffer1(context, flags, size, host_ptr_is_direct ? host_ptr : Buffers.getArray(host_ptr), host_ptr_is_direct ? Buffers.getDirectBufferByteOffset(host_ptr) : Buffers.getIndirectBufferByteOffset(host_ptr), host_ptr_is_direct, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateSubBuffer}(cl_mem buffer, cl_mem_flags flags, cl_buffer_create_type buffer_create_type, const void * buffer_create_info, cl_int * errcode_ret)
@param buffer_create_info a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateSubBuffer(long buffer, long flags, int buffer_create_type, Buffer buffer_create_info, IntBuffer errcode_ret) {
final boolean buffer_create_info_is_direct = Buffers.isDirect(buffer_create_info);
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateSubBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateSubBuffer"));
}
return dispatch_clCreateSubBuffer1(buffer, flags, buffer_create_type, buffer_create_info_is_direct ? buffer_create_info : Buffers.getArray(buffer_create_info), buffer_create_info_is_direct ? Buffers.getDirectBufferByteOffset(buffer_create_info) : Buffers.getIndirectBufferByteOffset(buffer_create_info), buffer_create_info_is_direct, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateSubBuffer}(cl_mem buffer, cl_mem_flags flags, cl_buffer_create_type buffer_create_type, const void * buffer_create_info, cl_int * errcode_ret)
@param buffer_create_info a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateSubBuffer1(long buffer, long flags, int buffer_create_type, Object buffer_create_info, int buffer_create_info_byte_offset, boolean buffer_create_info_is_direct, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateSubBuffer}(cl_mem buffer, cl_mem_flags flags, cl_buffer_create_type buffer_create_type, const void * buffer_create_info, cl_int * errcode_ret)
@param buffer_create_info a direct or array-backed {@link java.nio.Buffer} */
public long clCreateSubBuffer(long buffer, long flags, int buffer_create_type, Buffer buffer_create_info, int[] errcode_ret, int errcode_ret_offset) {
final boolean buffer_create_info_is_direct = Buffers.isDirect(buffer_create_info);
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateSubBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateSubBuffer"));
}
return dispatch_clCreateSubBuffer1(buffer, flags, buffer_create_type, buffer_create_info_is_direct ? buffer_create_info : Buffers.getArray(buffer_create_info), buffer_create_info_is_direct ? Buffers.getDirectBufferByteOffset(buffer_create_info) : Buffers.getIndirectBufferByteOffset(buffer_create_info), buffer_create_info_is_direct, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateImage2D}(cl_context context, cl_mem_flags flags, const cl_image_format * image_format, size_t image_width, size_t image_height, size_t image_row_pitch, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateImage2D(long context, long flags, CLImageFormatImpl image_format, long image_width, long image_height, long image_row_pitch, Buffer host_ptr, IntBuffer errcode_ret) {
final boolean host_ptr_is_direct = Buffers.isDirect(host_ptr);
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateImage2D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateImage2D"));
}
return dispatch_clCreateImage2D1(context, flags, ((image_format == null) ? null : image_format.getBuffer()), image_width, image_height, image_row_pitch, host_ptr_is_direct ? host_ptr : Buffers.getArray(host_ptr), host_ptr_is_direct ? Buffers.getDirectBufferByteOffset(host_ptr) : Buffers.getIndirectBufferByteOffset(host_ptr), host_ptr_is_direct, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateImage2D}(cl_context context, cl_mem_flags flags, const cl_image_format * image_format, size_t image_width, size_t image_height, size_t image_row_pitch, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateImage2D1(long context, long flags, ByteBuffer image_format, long image_width, long image_height, long image_row_pitch, Object host_ptr, int host_ptr_byte_offset, boolean host_ptr_is_direct, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateImage2D}(cl_context context, cl_mem_flags flags, const cl_image_format * image_format, size_t image_width, size_t image_height, size_t image_row_pitch, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer} */
public long clCreateImage2D(long context, long flags, CLImageFormatImpl image_format, long image_width, long image_height, long image_row_pitch, Buffer host_ptr, int[] errcode_ret, int errcode_ret_offset) {
final boolean host_ptr_is_direct = Buffers.isDirect(host_ptr);
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateImage2D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateImage2D"));
}
return dispatch_clCreateImage2D1(context, flags, ((image_format == null) ? null : image_format.getBuffer()), image_width, image_height, image_row_pitch, host_ptr_is_direct ? host_ptr : Buffers.getArray(host_ptr), host_ptr_is_direct ? Buffers.getDirectBufferByteOffset(host_ptr) : Buffers.getIndirectBufferByteOffset(host_ptr), host_ptr_is_direct, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateImage3D}(cl_context context, cl_mem_flags flags, const cl_image_format * image_format, size_t image_width, size_t image_height, size_t image_depth, size_t image_row_pitch, size_t image_slice_pitch, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateImage3D(long context, long flags, CLImageFormatImpl image_format, long image_width, long image_height, long image_depth, long image_row_pitch, long image_slice_pitch, Buffer host_ptr, IntBuffer errcode_ret) {
final boolean host_ptr_is_direct = Buffers.isDirect(host_ptr);
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateImage3D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateImage3D"));
}
return dispatch_clCreateImage3D1(context, flags, ((image_format == null) ? null : image_format.getBuffer()), image_width, image_height, image_depth, image_row_pitch, image_slice_pitch, host_ptr_is_direct ? host_ptr : Buffers.getArray(host_ptr), host_ptr_is_direct ? Buffers.getDirectBufferByteOffset(host_ptr) : Buffers.getIndirectBufferByteOffset(host_ptr), host_ptr_is_direct, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateImage3D}(cl_context context, cl_mem_flags flags, const cl_image_format * image_format, size_t image_width, size_t image_height, size_t image_depth, size_t image_row_pitch, size_t image_slice_pitch, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer}
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateImage3D1(long context, long flags, ByteBuffer image_format, long image_width, long image_height, long image_depth, long image_row_pitch, long image_slice_pitch, Object host_ptr, int host_ptr_byte_offset, boolean host_ptr_is_direct, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateImage3D}(cl_context context, cl_mem_flags flags, const cl_image_format * image_format, size_t image_width, size_t image_height, size_t image_depth, size_t image_row_pitch, size_t image_slice_pitch, void * host_ptr, cl_int * errcode_ret)
@param host_ptr a direct or array-backed {@link java.nio.Buffer} */
public long clCreateImage3D(long context, long flags, CLImageFormatImpl image_format, long image_width, long image_height, long image_depth, long image_row_pitch, long image_slice_pitch, Buffer host_ptr, int[] errcode_ret, int errcode_ret_offset) {
final boolean host_ptr_is_direct = Buffers.isDirect(host_ptr);
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateImage3D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateImage3D"));
}
return dispatch_clCreateImage3D1(context, flags, ((image_format == null) ? null : image_format.getBuffer()), image_width, image_height, image_depth, image_row_pitch, image_slice_pitch, host_ptr_is_direct ? host_ptr : Buffers.getArray(host_ptr), host_ptr_is_direct ? Buffers.getDirectBufferByteOffset(host_ptr) : Buffers.getIndirectBufferByteOffset(host_ptr), host_ptr_is_direct, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainMemObject}(cl_mem memobj)
*/
public int clRetainMemObject(long memobj) {
final long __addr_ = addressTable._addressof_clRetainMemObject;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainMemObject"));
}
return dispatch_clRetainMemObject1(memobj, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainMemObject}(cl_mem memobj)
*/
private native int dispatch_clRetainMemObject1(long memobj, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseMemObject}(cl_mem memobj)
*/
public int clReleaseMemObject(long memobj) {
final long __addr_ = addressTable._addressof_clReleaseMemObject;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clReleaseMemObject"));
}
return dispatch_clReleaseMemObject1(memobj, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseMemObject}(cl_mem memobj)
*/
private native int dispatch_clReleaseMemObject1(long memobj, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetSupportedImageFormats}(cl_context context, cl_mem_flags flags, cl_mem_object_type image_type, cl_uint num_entries, cl_image_format * image_formats, cl_uint * num_image_formats)
@param num_image_formats a direct or array-backed {@link java.nio.IntBuffer} */
public int clGetSupportedImageFormats(long context, long flags, int image_type, int num_entries, CLImageFormatImpl image_formats, IntBuffer num_image_formats) {
final boolean num_image_formats_is_direct = Buffers.isDirect(num_image_formats);
final long __addr_ = addressTable._addressof_clGetSupportedImageFormats;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetSupportedImageFormats"));
}
return dispatch_clGetSupportedImageFormats1(context, flags, image_type, num_entries, ((image_formats == null) ? null : image_formats.getBuffer()), num_image_formats_is_direct ? num_image_formats : Buffers.getArray(num_image_formats), num_image_formats_is_direct ? Buffers.getDirectBufferByteOffset(num_image_formats) : Buffers.getIndirectBufferByteOffset(num_image_formats), num_image_formats_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetSupportedImageFormats}(cl_context context, cl_mem_flags flags, cl_mem_object_type image_type, cl_uint num_entries, cl_image_format * image_formats, cl_uint * num_image_formats)
@param num_image_formats a direct or array-backed {@link java.nio.IntBuffer} */
private native int dispatch_clGetSupportedImageFormats1(long context, long flags, int image_type, int num_entries, ByteBuffer image_formats, Object num_image_formats, int num_image_formats_byte_offset, boolean num_image_formats_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetSupportedImageFormats}(cl_context context, cl_mem_flags flags, cl_mem_object_type image_type, cl_uint num_entries, cl_image_format * image_formats, cl_uint * num_image_formats)
*/
public int clGetSupportedImageFormats(long context, long flags, int image_type, int num_entries, CLImageFormatImpl image_formats, int[] num_image_formats, int num_image_formats_offset) {
if(num_image_formats != null && num_image_formats.length <= num_image_formats_offset)
throw new IllegalArgumentException("array offset argument \"num_image_formats_offset\" (" + num_image_formats_offset + ") equals or exceeds array length (" + num_image_formats.length + ")");
final long __addr_ = addressTable._addressof_clGetSupportedImageFormats;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetSupportedImageFormats"));
}
return dispatch_clGetSupportedImageFormats1(context, flags, image_type, num_entries, ((image_formats == null) ? null : image_formats.getBuffer()), num_image_formats, Buffers.SIZEOF_INT * num_image_formats_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetMemObjectInfo}(cl_mem memobj, cl_mem_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetMemObjectInfo(long memobj, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetMemObjectInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetMemObjectInfo"));
}
return dispatch_clGetMemObjectInfo0(memobj, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetMemObjectInfo}(cl_mem memobj, cl_mem_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetMemObjectInfo0(long memobj, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetImageInfo}(cl_mem image, cl_image_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetImageInfo(long image, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetImageInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetImageInfo"));
}
return dispatch_clGetImageInfo0(image, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetImageInfo}(cl_mem image, cl_image_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetImageInfo0(long image, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_sampler {@native clCreateSampler}(cl_context context, cl_bool normalized_coords, cl_addressing_mode addressing_mode, cl_filter_mode filter_mode, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateSampler(long context, int normalized_coords, int addressing_mode, int filter_mode, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateSampler;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateSampler"));
}
return dispatch_clCreateSampler1(context, normalized_coords, addressing_mode, filter_mode, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_sampler {@native clCreateSampler}(cl_context context, cl_bool normalized_coords, cl_addressing_mode addressing_mode, cl_filter_mode filter_mode, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateSampler1(long context, int normalized_coords, int addressing_mode, int filter_mode, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_sampler {@native clCreateSampler}(cl_context context, cl_bool normalized_coords, cl_addressing_mode addressing_mode, cl_filter_mode filter_mode, cl_int * errcode_ret)
*/
public long clCreateSampler(long context, int normalized_coords, int addressing_mode, int filter_mode, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateSampler;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateSampler"));
}
return dispatch_clCreateSampler1(context, normalized_coords, addressing_mode, filter_mode, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainSampler}(cl_sampler sampler)
*/
public int clRetainSampler(long sampler) {
final long __addr_ = addressTable._addressof_clRetainSampler;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainSampler"));
}
return dispatch_clRetainSampler1(sampler, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainSampler}(cl_sampler sampler)
*/
private native int dispatch_clRetainSampler1(long sampler, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseSampler}(cl_sampler sampler)
*/
public int clReleaseSampler(long sampler) {
final long __addr_ = addressTable._addressof_clReleaseSampler;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clReleaseSampler"));
}
return dispatch_clReleaseSampler1(sampler, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseSampler}(cl_sampler sampler)
*/
private native int dispatch_clReleaseSampler1(long sampler, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetSamplerInfo}(cl_sampler sampler, cl_sampler_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetSamplerInfo(long sampler, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetSamplerInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetSamplerInfo"));
}
return dispatch_clGetSamplerInfo0(sampler, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetSamplerInfo}(cl_sampler sampler, cl_sampler_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetSamplerInfo0(long sampler, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_program {@native clCreateProgramWithSource}(cl_context context, cl_uint count, const char * * strings, const size_t * lengths, cl_int * errcode_ret)
@param lengths a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param errcode_ret a direct only {@link java.nio.IntBuffer} */
public long clCreateProgramWithSource(long context, int count, String[] strings, PointerBuffer lengths, IntBuffer errcode_ret) {
if (!Buffers.isDirect(lengths))
throw new IllegalArgumentException("Argument \"lengths\" is not a direct buffer");
if (!Buffers.isDirect(errcode_ret))
throw new IllegalArgumentException("Argument \"errcode_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clCreateProgramWithSource;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateProgramWithSource"));
}
return dispatch_clCreateProgramWithSource0(context, count, strings, lengths != null ? lengths.getBuffer() : null, Buffers.getDirectBufferByteOffset(lengths), errcode_ret, Buffers.getDirectBufferByteOffset(errcode_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_program {@native clCreateProgramWithSource}(cl_context context, cl_uint count, const char * * strings, const size_t * lengths, cl_int * errcode_ret)
@param lengths a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param errcode_ret a direct only {@link java.nio.IntBuffer} */
private native long dispatch_clCreateProgramWithSource0(long context, int count, String[] strings, Object lengths, int lengths_byte_offset, Object errcode_ret, int errcode_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_program {@native clCreateProgramWithBinary}(cl_context context, cl_uint num_devices, const cl_device_id * device_list, const size_t * lengths, unsigned const char * * binaries, cl_int * binary_status, cl_int * errcode_ret)
@param device_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param lengths a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param binaries a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param binary_status a direct only {@link java.nio.IntBuffer}
@param errcode_ret a direct only {@link java.nio.IntBuffer} */
public long clCreateProgramWithBinary(long context, int num_devices, PointerBuffer device_list, PointerBuffer lengths, PointerBuffer binaries, IntBuffer binary_status, IntBuffer errcode_ret) {
if (!Buffers.isDirect(device_list))
throw new IllegalArgumentException("Argument \"device_list\" is not a direct buffer");
if (!Buffers.isDirect(lengths))
throw new IllegalArgumentException("Argument \"lengths\" is not a direct buffer");
if (!Buffers.isDirect(binaries))
throw new IllegalArgumentException("Argument \"binaries\" is not a direct buffer");
if (!Buffers.isDirect(binary_status))
throw new IllegalArgumentException("Argument \"binary_status\" is not a direct buffer");
if (!Buffers.isDirect(errcode_ret))
throw new IllegalArgumentException("Argument \"errcode_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clCreateProgramWithBinary;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateProgramWithBinary"));
}
return dispatch_clCreateProgramWithBinary0(context, num_devices, device_list != null ? device_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(device_list), lengths != null ? lengths.getBuffer() : null, Buffers.getDirectBufferByteOffset(lengths), binaries != null ? binaries.getBuffer() : null, Buffers.getDirectBufferByteOffset(binaries), binary_status, Buffers.getDirectBufferByteOffset(binary_status), errcode_ret, Buffers.getDirectBufferByteOffset(errcode_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_program {@native clCreateProgramWithBinary}(cl_context context, cl_uint num_devices, const cl_device_id * device_list, const size_t * lengths, unsigned const char * * binaries, cl_int * binary_status, cl_int * errcode_ret)
@param device_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param lengths a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param binaries a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param binary_status a direct only {@link java.nio.IntBuffer}
@param errcode_ret a direct only {@link java.nio.IntBuffer} */
private native long dispatch_clCreateProgramWithBinary0(long context, int num_devices, Object device_list, int device_list_byte_offset, Object lengths, int lengths_byte_offset, Object binaries, int binaries_byte_offset, Object binary_status, int binary_status_byte_offset, Object errcode_ret, int errcode_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainProgram}(cl_program program)
*/
public int clRetainProgram(long program) {
final long __addr_ = addressTable._addressof_clRetainProgram;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainProgram"));
}
return dispatch_clRetainProgram1(program, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainProgram}(cl_program program)
*/
private native int dispatch_clRetainProgram1(long program, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseProgram}(cl_program program)
*/
public int clReleaseProgram(long program) {
final long __addr_ = addressTable._addressof_clReleaseProgram;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clReleaseProgram"));
}
return dispatch_clReleaseProgram1(program, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseProgram}(cl_program program)
*/
private native int dispatch_clReleaseProgram1(long program, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clUnloadCompiler}(void)
*/
public int clUnloadCompiler() {
final long __addr_ = addressTable._addressof_clUnloadCompiler;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clUnloadCompiler"));
}
return dispatch_clUnloadCompiler1(__addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clUnloadCompiler}(void)
*/
private native int dispatch_clUnloadCompiler1(long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetProgramInfo}(cl_program program, cl_program_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetProgramInfo(long program, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetProgramInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetProgramInfo"));
}
return dispatch_clGetProgramInfo0(program, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetProgramInfo}(cl_program program, cl_program_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetProgramInfo0(long program, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetProgramBuildInfo}(cl_program program, cl_device_id device, cl_program_build_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetProgramBuildInfo(long program, long device, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetProgramBuildInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetProgramBuildInfo"));
}
return dispatch_clGetProgramBuildInfo0(program, device, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetProgramBuildInfo}(cl_program program, cl_device_id device, cl_program_build_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetProgramBuildInfo0(long program, long device, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_kernel {@native clCreateKernel}(cl_program program, const char * kernel_name, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateKernel(long program, String kernel_name, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateKernel;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateKernel"));
}
return dispatch_clCreateKernel1(program, kernel_name, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_kernel {@native clCreateKernel}(cl_program program, const char * kernel_name, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateKernel1(long program, String kernel_name, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_kernel {@native clCreateKernel}(cl_program program, const char * kernel_name, cl_int * errcode_ret)
*/
public long clCreateKernel(long program, String kernel_name, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateKernel;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateKernel"));
}
return dispatch_clCreateKernel1(program, kernel_name, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clCreateKernelsInProgram}(cl_program program, cl_uint num_kernels, cl_kernel * kernels, cl_uint * num_kernels_ret)
@param kernels a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param num_kernels_ret a direct only {@link java.nio.IntBuffer} */
public int clCreateKernelsInProgram(long program, int num_kernels, PointerBuffer kernels, IntBuffer num_kernels_ret) {
if (!Buffers.isDirect(kernels))
throw new IllegalArgumentException("Argument \"kernels\" is not a direct buffer");
if (!Buffers.isDirect(num_kernels_ret))
throw new IllegalArgumentException("Argument \"num_kernels_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clCreateKernelsInProgram;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateKernelsInProgram"));
}
return dispatch_clCreateKernelsInProgram0(program, num_kernels, kernels != null ? kernels.getBuffer() : null, Buffers.getDirectBufferByteOffset(kernels), num_kernels_ret, Buffers.getDirectBufferByteOffset(num_kernels_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clCreateKernelsInProgram}(cl_program program, cl_uint num_kernels, cl_kernel * kernels, cl_uint * num_kernels_ret)
@param kernels a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param num_kernels_ret a direct only {@link java.nio.IntBuffer} */
private native int dispatch_clCreateKernelsInProgram0(long program, int num_kernels, Object kernels, int kernels_byte_offset, Object num_kernels_ret, int num_kernels_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainKernel}(cl_kernel kernel)
*/
public int clRetainKernel(long kernel) {
final long __addr_ = addressTable._addressof_clRetainKernel;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainKernel"));
}
return dispatch_clRetainKernel1(kernel, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainKernel}(cl_kernel kernel)
*/
private native int dispatch_clRetainKernel1(long kernel, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseKernel}(cl_kernel kernel)
*/
public int clReleaseKernel(long kernel) {
final long __addr_ = addressTable._addressof_clReleaseKernel;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clReleaseKernel"));
}
return dispatch_clReleaseKernel1(kernel, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseKernel}(cl_kernel kernel)
*/
private native int dispatch_clReleaseKernel1(long kernel, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clSetKernelArg}(cl_kernel kernel, cl_uint arg_index, size_t arg_size, const void * arg_value)
@param arg_value a direct only {@link java.nio.Buffer} */
public int clSetKernelArg(long kernel, int arg_index, long arg_size, Buffer arg_value) {
if (!Buffers.isDirect(arg_value))
throw new IllegalArgumentException("Argument \"arg_value\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clSetKernelArg;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clSetKernelArg"));
}
return dispatch_clSetKernelArg0(kernel, arg_index, arg_size, arg_value, Buffers.getDirectBufferByteOffset(arg_value), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clSetKernelArg}(cl_kernel kernel, cl_uint arg_index, size_t arg_size, const void * arg_value)
@param arg_value a direct only {@link java.nio.Buffer} */
private native int dispatch_clSetKernelArg0(long kernel, int arg_index, long arg_size, Object arg_value, int arg_value_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetKernelInfo}(cl_kernel kernel, cl_kernel_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetKernelInfo(long kernel, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetKernelInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetKernelInfo"));
}
return dispatch_clGetKernelInfo0(kernel, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetKernelInfo}(cl_kernel kernel, cl_kernel_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetKernelInfo0(long kernel, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetKernelWorkGroupInfo}(cl_kernel kernel, cl_device_id device, cl_kernel_work_group_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetKernelWorkGroupInfo(long kernel, long device, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetKernelWorkGroupInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetKernelWorkGroupInfo"));
}
return dispatch_clGetKernelWorkGroupInfo0(kernel, device, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetKernelWorkGroupInfo}(cl_kernel kernel, cl_device_id device, cl_kernel_work_group_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetKernelWorkGroupInfo0(long kernel, long device, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clWaitForEvents}(cl_uint num_events, const cl_event * event_list)
@param event_list a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clWaitForEvents(int num_events, PointerBuffer event_list) {
if (!Buffers.isDirect(event_list))
throw new IllegalArgumentException("Argument \"event_list\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clWaitForEvents;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clWaitForEvents"));
}
return dispatch_clWaitForEvents0(num_events, event_list != null ? event_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_list), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clWaitForEvents}(cl_uint num_events, const cl_event * event_list)
@param event_list a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clWaitForEvents0(int num_events, Object event_list, int event_list_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetEventInfo}(cl_event event, cl_event_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetEventInfo(long event, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetEventInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetEventInfo"));
}
return dispatch_clGetEventInfo0(event, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetEventInfo}(cl_event event, cl_event_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetEventInfo0(long event, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_event {@native clCreateUserEvent}(cl_context context, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateUserEvent(long context, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateUserEvent;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateUserEvent"));
}
return dispatch_clCreateUserEvent1(context, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_event {@native clCreateUserEvent}(cl_context context, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateUserEvent1(long context, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_event {@native clCreateUserEvent}(cl_context context, cl_int * errcode_ret)
*/
public long clCreateUserEvent(long context, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateUserEvent;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateUserEvent"));
}
return dispatch_clCreateUserEvent1(context, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainEvent}(cl_event event)
*/
public int clRetainEvent(long event) {
final long __addr_ = addressTable._addressof_clRetainEvent;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainEvent"));
}
return dispatch_clRetainEvent1(event, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainEvent}(cl_event event)
*/
private native int dispatch_clRetainEvent1(long event, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseEvent}(cl_event event)
*/
public int clReleaseEvent(long event) {
final long __addr_ = addressTable._addressof_clReleaseEvent;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clReleaseEvent"));
}
return dispatch_clReleaseEvent1(event, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseEvent}(cl_event event)
*/
private native int dispatch_clReleaseEvent1(long event, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clSetUserEventStatus}(cl_event event, cl_int execution_status)
*/
public int clSetUserEventStatus(long event, int execution_status) {
final long __addr_ = addressTable._addressof_clSetUserEventStatus;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clSetUserEventStatus"));
}
return dispatch_clSetUserEventStatus1(event, execution_status, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clSetUserEventStatus}(cl_event event, cl_int execution_status)
*/
private native int dispatch_clSetUserEventStatus1(long event, int execution_status, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetEventProfilingInfo}(cl_event event, cl_profiling_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetEventProfilingInfo(long event, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetEventProfilingInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetEventProfilingInfo"));
}
return dispatch_clGetEventProfilingInfo0(event, param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetEventProfilingInfo}(cl_event event, cl_profiling_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetEventProfilingInfo0(long event, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clFlush}(cl_command_queue command_queue)
*/
public int clFlush(long command_queue) {
final long __addr_ = addressTable._addressof_clFlush;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clFlush"));
}
return dispatch_clFlush1(command_queue, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clFlush}(cl_command_queue command_queue)
*/
private native int dispatch_clFlush1(long command_queue, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clFinish}(cl_command_queue command_queue)
*/
public int clFinish(long command_queue) {
final long __addr_ = addressTable._addressof_clFinish;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clFinish"));
}
return dispatch_clFinish1(command_queue, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clFinish}(cl_command_queue command_queue)
*/
private native int dispatch_clFinish1(long command_queue, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReadBuffer}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_read, size_t offset, size_t cb, void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueReadBuffer(long command_queue, long buffer, int blocking_read, long offset, long cb, Buffer ptr, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(ptr))
throw new IllegalArgumentException("Argument \"ptr\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueReadBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueReadBuffer"));
}
return dispatch_clEnqueueReadBuffer0(command_queue, buffer, blocking_read, offset, cb, ptr, Buffers.getDirectBufferByteOffset(ptr), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReadBuffer}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_read, size_t offset, size_t cb, void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueReadBuffer0(long command_queue, long buffer, int blocking_read, long offset, long cb, Object ptr, int ptr_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReadBufferRect}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_read, const size_t * buffer_origin, const size_t * host_origin, const size_t * region, size_t buffer_row_pitch, size_t buffer_slice_pitch, size_t host_row_pitch, size_t host_slice_pitch, void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param buffer_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param host_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param region a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueReadBufferRect(long command_queue, long buffer, int blocking_read, PointerBuffer buffer_origin, PointerBuffer host_origin, PointerBuffer region, long buffer_row_pitch, long buffer_slice_pitch, long host_row_pitch, long host_slice_pitch, Buffer ptr, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(buffer_origin))
throw new IllegalArgumentException("Argument \"buffer_origin\" is not a direct buffer");
if (!Buffers.isDirect(host_origin))
throw new IllegalArgumentException("Argument \"host_origin\" is not a direct buffer");
if (!Buffers.isDirect(region))
throw new IllegalArgumentException("Argument \"region\" is not a direct buffer");
if (!Buffers.isDirect(ptr))
throw new IllegalArgumentException("Argument \"ptr\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueReadBufferRect;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueReadBufferRect"));
}
return dispatch_clEnqueueReadBufferRect0(command_queue, buffer, blocking_read, buffer_origin != null ? buffer_origin.getBuffer() : null, Buffers.getDirectBufferByteOffset(buffer_origin), host_origin != null ? host_origin.getBuffer() : null, Buffers.getDirectBufferByteOffset(host_origin), region != null ? region.getBuffer() : null, Buffers.getDirectBufferByteOffset(region), buffer_row_pitch, buffer_slice_pitch, host_row_pitch, host_slice_pitch, ptr, Buffers.getDirectBufferByteOffset(ptr), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReadBufferRect}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_read, const size_t * buffer_origin, const size_t * host_origin, const size_t * region, size_t buffer_row_pitch, size_t buffer_slice_pitch, size_t host_row_pitch, size_t host_slice_pitch, void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param buffer_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param host_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param region a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueReadBufferRect0(long command_queue, long buffer, int blocking_read, Object buffer_origin, int buffer_origin_byte_offset, Object host_origin, int host_origin_byte_offset, Object region, int region_byte_offset, long buffer_row_pitch, long buffer_slice_pitch, long host_row_pitch, long host_slice_pitch, Object ptr, int ptr_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWriteBuffer}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_write, size_t offset, size_t cb, const void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueWriteBuffer(long command_queue, long buffer, int blocking_write, long offset, long cb, Buffer ptr, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(ptr))
throw new IllegalArgumentException("Argument \"ptr\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueWriteBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueWriteBuffer"));
}
return dispatch_clEnqueueWriteBuffer0(command_queue, buffer, blocking_write, offset, cb, ptr, Buffers.getDirectBufferByteOffset(ptr), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWriteBuffer}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_write, size_t offset, size_t cb, const void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueWriteBuffer0(long command_queue, long buffer, int blocking_write, long offset, long cb, Object ptr, int ptr_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWriteBufferRect}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_write, const size_t * buffer_origin, const size_t * host_origin, const size_t * region, size_t buffer_row_pitch, size_t buffer_slice_pitch, size_t host_row_pitch, size_t host_slice_pitch, const void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param buffer_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param host_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param region a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueWriteBufferRect(long command_queue, long buffer, int blocking_write, PointerBuffer buffer_origin, PointerBuffer host_origin, PointerBuffer region, long buffer_row_pitch, long buffer_slice_pitch, long host_row_pitch, long host_slice_pitch, Buffer ptr, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(buffer_origin))
throw new IllegalArgumentException("Argument \"buffer_origin\" is not a direct buffer");
if (!Buffers.isDirect(host_origin))
throw new IllegalArgumentException("Argument \"host_origin\" is not a direct buffer");
if (!Buffers.isDirect(region))
throw new IllegalArgumentException("Argument \"region\" is not a direct buffer");
if (!Buffers.isDirect(ptr))
throw new IllegalArgumentException("Argument \"ptr\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueWriteBufferRect;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueWriteBufferRect"));
}
return dispatch_clEnqueueWriteBufferRect0(command_queue, buffer, blocking_write, buffer_origin != null ? buffer_origin.getBuffer() : null, Buffers.getDirectBufferByteOffset(buffer_origin), host_origin != null ? host_origin.getBuffer() : null, Buffers.getDirectBufferByteOffset(host_origin), region != null ? region.getBuffer() : null, Buffers.getDirectBufferByteOffset(region), buffer_row_pitch, buffer_slice_pitch, host_row_pitch, host_slice_pitch, ptr, Buffers.getDirectBufferByteOffset(ptr), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWriteBufferRect}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_write, const size_t * buffer_origin, const size_t * host_origin, const size_t * region, size_t buffer_row_pitch, size_t buffer_slice_pitch, size_t host_row_pitch, size_t host_slice_pitch, const void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param buffer_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param host_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param region a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueWriteBufferRect0(long command_queue, long buffer, int blocking_write, Object buffer_origin, int buffer_origin_byte_offset, Object host_origin, int host_origin_byte_offset, Object region, int region_byte_offset, long buffer_row_pitch, long buffer_slice_pitch, long host_row_pitch, long host_slice_pitch, Object ptr, int ptr_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyBuffer}(cl_command_queue command_queue, cl_mem src_buffer, cl_mem dst_buffer, size_t src_offset, size_t dst_offset, size_t cb, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueCopyBuffer(long command_queue, long src_buffer, long dst_buffer, long src_offset, long dst_offset, long cb, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueCopyBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueCopyBuffer"));
}
return dispatch_clEnqueueCopyBuffer0(command_queue, src_buffer, dst_buffer, src_offset, dst_offset, cb, num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyBuffer}(cl_command_queue command_queue, cl_mem src_buffer, cl_mem dst_buffer, size_t src_offset, size_t dst_offset, size_t cb, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueCopyBuffer0(long command_queue, long src_buffer, long dst_buffer, long src_offset, long dst_offset, long cb, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyBufferRect}(cl_command_queue command_queue, cl_mem src_buffer, cl_mem dst_buffer, const size_t * src_origin, const size_t * dst_origin, const size_t * region, size_t src_row_pitch, size_t src_slice_pitch, size_t dst_row_pitch, size_t dst_slice_pitch, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param src_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param dst_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param region a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueCopyBufferRect(long command_queue, long src_buffer, long dst_buffer, PointerBuffer src_origin, PointerBuffer dst_origin, PointerBuffer region, long src_row_pitch, long src_slice_pitch, long dst_row_pitch, long dst_slice_pitch, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(src_origin))
throw new IllegalArgumentException("Argument \"src_origin\" is not a direct buffer");
if (!Buffers.isDirect(dst_origin))
throw new IllegalArgumentException("Argument \"dst_origin\" is not a direct buffer");
if (!Buffers.isDirect(region))
throw new IllegalArgumentException("Argument \"region\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueCopyBufferRect;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueCopyBufferRect"));
}
return dispatch_clEnqueueCopyBufferRect0(command_queue, src_buffer, dst_buffer, src_origin != null ? src_origin.getBuffer() : null, Buffers.getDirectBufferByteOffset(src_origin), dst_origin != null ? dst_origin.getBuffer() : null, Buffers.getDirectBufferByteOffset(dst_origin), region != null ? region.getBuffer() : null, Buffers.getDirectBufferByteOffset(region), src_row_pitch, src_slice_pitch, dst_row_pitch, dst_slice_pitch, num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyBufferRect}(cl_command_queue command_queue, cl_mem src_buffer, cl_mem dst_buffer, const size_t * src_origin, const size_t * dst_origin, const size_t * region, size_t src_row_pitch, size_t src_slice_pitch, size_t dst_row_pitch, size_t dst_slice_pitch, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param src_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param dst_origin a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param region a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueCopyBufferRect0(long command_queue, long src_buffer, long dst_buffer, Object src_origin, int src_origin_byte_offset, Object dst_origin, int dst_origin_byte_offset, Object region, int region_byte_offset, long src_row_pitch, long src_slice_pitch, long dst_row_pitch, long dst_slice_pitch, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReadImage}(cl_command_queue command_queue, cl_mem image, cl_bool blocking_read, const size_t * , const size_t * , size_t row_pitch, size_t slice_pitch, void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueReadImage(long command_queue, long image, int blocking_read, PointerBuffer arg3, PointerBuffer arg4, long row_pitch, long slice_pitch, Buffer ptr, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(arg3))
throw new IllegalArgumentException("Argument \"arg3\" is not a direct buffer");
if (!Buffers.isDirect(arg4))
throw new IllegalArgumentException("Argument \"arg4\" is not a direct buffer");
if (!Buffers.isDirect(ptr))
throw new IllegalArgumentException("Argument \"ptr\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueReadImage;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueReadImage"));
}
return dispatch_clEnqueueReadImage0(command_queue, image, blocking_read, arg3 != null ? arg3.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg3), arg4 != null ? arg4.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg4), row_pitch, slice_pitch, ptr, Buffers.getDirectBufferByteOffset(ptr), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReadImage}(cl_command_queue command_queue, cl_mem image, cl_bool blocking_read, const size_t * , const size_t * , size_t row_pitch, size_t slice_pitch, void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueReadImage0(long command_queue, long image, int blocking_read, Object arg3, int arg3_byte_offset, Object arg4, int arg4_byte_offset, long row_pitch, long slice_pitch, Object ptr, int ptr_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWriteImage}(cl_command_queue command_queue, cl_mem image, cl_bool blocking_write, const size_t * , const size_t * , size_t input_row_pitch, size_t input_slice_pitch, const void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueWriteImage(long command_queue, long image, int blocking_write, PointerBuffer arg3, PointerBuffer arg4, long input_row_pitch, long input_slice_pitch, Buffer ptr, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(arg3))
throw new IllegalArgumentException("Argument \"arg3\" is not a direct buffer");
if (!Buffers.isDirect(arg4))
throw new IllegalArgumentException("Argument \"arg4\" is not a direct buffer");
if (!Buffers.isDirect(ptr))
throw new IllegalArgumentException("Argument \"ptr\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueWriteImage;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueWriteImage"));
}
return dispatch_clEnqueueWriteImage0(command_queue, image, blocking_write, arg3 != null ? arg3.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg3), arg4 != null ? arg4.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg4), input_row_pitch, input_slice_pitch, ptr, Buffers.getDirectBufferByteOffset(ptr), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWriteImage}(cl_command_queue command_queue, cl_mem image, cl_bool blocking_write, const size_t * , const size_t * , size_t input_row_pitch, size_t input_slice_pitch, const void * ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueWriteImage0(long command_queue, long image, int blocking_write, Object arg3, int arg3_byte_offset, Object arg4, int arg4_byte_offset, long input_row_pitch, long input_slice_pitch, Object ptr, int ptr_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyImage}(cl_command_queue command_queue, cl_mem src_image, cl_mem dst_image, const size_t * , const size_t * , const size_t * , cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg5 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueCopyImage(long command_queue, long src_image, long dst_image, PointerBuffer arg3, PointerBuffer arg4, PointerBuffer arg5, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(arg3))
throw new IllegalArgumentException("Argument \"arg3\" is not a direct buffer");
if (!Buffers.isDirect(arg4))
throw new IllegalArgumentException("Argument \"arg4\" is not a direct buffer");
if (!Buffers.isDirect(arg5))
throw new IllegalArgumentException("Argument \"arg5\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueCopyImage;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueCopyImage"));
}
return dispatch_clEnqueueCopyImage0(command_queue, src_image, dst_image, arg3 != null ? arg3.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg3), arg4 != null ? arg4.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg4), arg5 != null ? arg5.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg5), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyImage}(cl_command_queue command_queue, cl_mem src_image, cl_mem dst_image, const size_t * , const size_t * , const size_t * , cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg5 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueCopyImage0(long command_queue, long src_image, long dst_image, Object arg3, int arg3_byte_offset, Object arg4, int arg4_byte_offset, Object arg5, int arg5_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyImageToBuffer}(cl_command_queue command_queue, cl_mem src_image, cl_mem dst_buffer, const size_t * , const size_t * , size_t dst_offset, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueCopyImageToBuffer(long command_queue, long src_image, long dst_buffer, PointerBuffer arg3, PointerBuffer arg4, long dst_offset, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(arg3))
throw new IllegalArgumentException("Argument \"arg3\" is not a direct buffer");
if (!Buffers.isDirect(arg4))
throw new IllegalArgumentException("Argument \"arg4\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueCopyImageToBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueCopyImageToBuffer"));
}
return dispatch_clEnqueueCopyImageToBuffer0(command_queue, src_image, dst_buffer, arg3 != null ? arg3.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg3), arg4 != null ? arg4.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg4), dst_offset, num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyImageToBuffer}(cl_command_queue command_queue, cl_mem src_image, cl_mem dst_buffer, const size_t * , const size_t * , size_t dst_offset, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg3 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueCopyImageToBuffer0(long command_queue, long src_image, long dst_buffer, Object arg3, int arg3_byte_offset, Object arg4, int arg4_byte_offset, long dst_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyBufferToImage}(cl_command_queue command_queue, cl_mem src_buffer, cl_mem dst_image, size_t src_offset, const size_t * , const size_t * , cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg5 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueCopyBufferToImage(long command_queue, long src_buffer, long dst_image, long src_offset, PointerBuffer arg4, PointerBuffer arg5, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(arg4))
throw new IllegalArgumentException("Argument \"arg4\" is not a direct buffer");
if (!Buffers.isDirect(arg5))
throw new IllegalArgumentException("Argument \"arg5\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueCopyBufferToImage;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueCopyBufferToImage"));
}
return dispatch_clEnqueueCopyBufferToImage0(command_queue, src_buffer, dst_image, src_offset, arg4 != null ? arg4.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg4), arg5 != null ? arg5.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg5), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueCopyBufferToImage}(cl_command_queue command_queue, cl_mem src_buffer, cl_mem dst_image, size_t src_offset, const size_t * , const size_t * , cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param arg4 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg5 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueCopyBufferToImage0(long command_queue, long src_buffer, long dst_image, long src_offset, Object arg4, int arg4_byte_offset, Object arg5, int arg5_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
void * {@native clEnqueueMapBuffer}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_map, cl_map_flags map_flags, size_t offset, size_t cb, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event, cl_int * errcode_ret)
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param errcode_ret a direct only {@link java.nio.IntBuffer} */
public ByteBuffer clEnqueueMapBuffer(long command_queue, long buffer, int blocking_map, long map_flags, long offset, long cb, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event, IntBuffer errcode_ret) {
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
if (!Buffers.isDirect(errcode_ret))
throw new IllegalArgumentException("Argument \"errcode_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueMapBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueMapBuffer"));
}
final ByteBuffer _res;
_res = dispatch_clEnqueueMapBuffer0(command_queue, buffer, blocking_map, map_flags, offset, cb, num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), errcode_ret, Buffers.getDirectBufferByteOffset(errcode_ret), __addr_);
if (_res == null) return null;
Buffers.nativeOrder(_res);
return _res;
}
/** Entry point (through function pointer) to C language function:
void * {@native clEnqueueMapBuffer}(cl_command_queue command_queue, cl_mem buffer, cl_bool blocking_map, cl_map_flags map_flags, size_t offset, size_t cb, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event, cl_int * errcode_ret)
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param errcode_ret a direct only {@link java.nio.IntBuffer} */
private native ByteBuffer dispatch_clEnqueueMapBuffer0(long command_queue, long buffer, int blocking_map, long map_flags, long offset, long cb, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, Object errcode_ret, int errcode_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueUnmapMemObject}(cl_command_queue command_queue, cl_mem memobj, void * mapped_ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mapped_ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueUnmapMemObject(long command_queue, long memobj, Buffer mapped_ptr, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(mapped_ptr))
throw new IllegalArgumentException("Argument \"mapped_ptr\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueUnmapMemObject;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueUnmapMemObject"));
}
return dispatch_clEnqueueUnmapMemObject0(command_queue, memobj, mapped_ptr, Buffers.getDirectBufferByteOffset(mapped_ptr), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueUnmapMemObject}(cl_command_queue command_queue, cl_mem memobj, void * mapped_ptr, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mapped_ptr a direct only {@link java.nio.Buffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueUnmapMemObject0(long command_queue, long memobj, Object mapped_ptr, int mapped_ptr_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueNDRangeKernel}(cl_command_queue command_queue, cl_kernel kernel, cl_uint work_dim, const size_t * global_work_offset, const size_t * global_work_size, const size_t * local_work_size, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param global_work_offset a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param global_work_size a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param local_work_size a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueNDRangeKernel(long command_queue, long kernel, int work_dim, PointerBuffer global_work_offset, PointerBuffer global_work_size, PointerBuffer local_work_size, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(global_work_offset))
throw new IllegalArgumentException("Argument \"global_work_offset\" is not a direct buffer");
if (!Buffers.isDirect(global_work_size))
throw new IllegalArgumentException("Argument \"global_work_size\" is not a direct buffer");
if (!Buffers.isDirect(local_work_size))
throw new IllegalArgumentException("Argument \"local_work_size\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueNDRangeKernel;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueNDRangeKernel"));
}
return dispatch_clEnqueueNDRangeKernel0(command_queue, kernel, work_dim, global_work_offset != null ? global_work_offset.getBuffer() : null, Buffers.getDirectBufferByteOffset(global_work_offset), global_work_size != null ? global_work_size.getBuffer() : null, Buffers.getDirectBufferByteOffset(global_work_size), local_work_size != null ? local_work_size.getBuffer() : null, Buffers.getDirectBufferByteOffset(local_work_size), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueNDRangeKernel}(cl_command_queue command_queue, cl_kernel kernel, cl_uint work_dim, const size_t * global_work_offset, const size_t * global_work_size, const size_t * local_work_size, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param global_work_offset a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param global_work_size a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param local_work_size a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueNDRangeKernel0(long command_queue, long kernel, int work_dim, Object global_work_offset, int global_work_offset_byte_offset, Object global_work_size, int global_work_size_byte_offset, Object local_work_size, int local_work_size_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueTask}(cl_command_queue command_queue, cl_kernel kernel, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueTask(long command_queue, long kernel, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueTask;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueTask"));
}
return dispatch_clEnqueueTask0(command_queue, kernel, num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueTask}(cl_command_queue command_queue, cl_kernel kernel, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueTask0(long command_queue, long kernel, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueMarker}(cl_command_queue command_queue, cl_event * event)
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueMarker(long command_queue, PointerBuffer event) {
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueMarker;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueMarker"));
}
return dispatch_clEnqueueMarker0(command_queue, event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueMarker}(cl_command_queue command_queue, cl_event * event)
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueMarker0(long command_queue, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWaitForEvents}(cl_command_queue command_queue, cl_uint num_events, const cl_event * event_list)
@param event_list a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueWaitForEvents(long command_queue, int num_events, PointerBuffer event_list) {
if (!Buffers.isDirect(event_list))
throw new IllegalArgumentException("Argument \"event_list\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueWaitForEvents;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueWaitForEvents"));
}
return dispatch_clEnqueueWaitForEvents0(command_queue, num_events, event_list != null ? event_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_list), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueWaitForEvents}(cl_command_queue command_queue, cl_uint num_events, const cl_event * event_list)
@param event_list a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueWaitForEvents0(long command_queue, int num_events, Object event_list, int event_list_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueBarrier}(cl_command_queue command_queue)
*/
public int clEnqueueBarrier(long command_queue) {
final long __addr_ = addressTable._addressof_clEnqueueBarrier;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueBarrier"));
}
return dispatch_clEnqueueBarrier0(command_queue, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueBarrier}(cl_command_queue command_queue)
*/
private native int dispatch_clEnqueueBarrier0(long command_queue, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clIcdGetPlatformIDsKHR}(cl_uint, cl_platform_id * , cl_uint * )
@param arg1 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg2 a direct only {@link java.nio.IntBuffer} */
public int clIcdGetPlatformIDsKHR(int arg0, PointerBuffer arg1, IntBuffer arg2) {
if (!Buffers.isDirect(arg1))
throw new IllegalArgumentException("Argument \"arg1\" is not a direct buffer");
if (!Buffers.isDirect(arg2))
throw new IllegalArgumentException("Argument \"arg2\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clIcdGetPlatformIDsKHR;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clIcdGetPlatformIDsKHR"));
}
return dispatch_clIcdGetPlatformIDsKHR0(arg0, arg1 != null ? arg1.getBuffer() : null, Buffers.getDirectBufferByteOffset(arg1), arg2, Buffers.getDirectBufferByteOffset(arg2), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clIcdGetPlatformIDsKHR}(cl_uint, cl_platform_id * , cl_uint * )
@param arg1 a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param arg2 a direct only {@link java.nio.IntBuffer} */
private native int dispatch_clIcdGetPlatformIDsKHR0(int arg0, Object arg1, int arg1_byte_offset, Object arg2, int arg2_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseDeviceEXT}(cl_device_id)
*/
public int clReleaseDeviceEXT(long arg0) {
final long __addr_ = addressTable._addressof_clReleaseDeviceEXT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clReleaseDeviceEXT"));
}
return dispatch_clReleaseDeviceEXT1(arg0, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clReleaseDeviceEXT}(cl_device_id)
*/
private native int dispatch_clReleaseDeviceEXT1(long arg0, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainDeviceEXT}(cl_device_id)
*/
public int clRetainDeviceEXT(long arg0) {
final long __addr_ = addressTable._addressof_clRetainDeviceEXT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clRetainDeviceEXT"));
}
return dispatch_clRetainDeviceEXT1(arg0, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clRetainDeviceEXT}(cl_device_id)
*/
private native int dispatch_clRetainDeviceEXT1(long arg0, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clCreateSubDevicesEXT}(cl_device_id, const cl_device_partition_property_ext * , cl_uint, cl_device_id * , cl_uint * )
@param arg1 a direct or array-backed {@link java.nio.LongBuffer}
@param arg3 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct or array-backed {@link java.nio.IntBuffer} */
public int clCreateSubDevicesEXT(long arg0, LongBuffer arg1, int arg2, PointerBuffer arg3, IntBuffer arg4) {
final boolean arg1_is_direct = Buffers.isDirect(arg1);
final boolean arg3_is_direct = Buffers.isDirect(arg3);
final boolean arg4_is_direct = Buffers.isDirect(arg4);
final long __addr_ = addressTable._addressof_clCreateSubDevicesEXT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateSubDevicesEXT"));
}
return dispatch_clCreateSubDevicesEXT1(arg0, arg1_is_direct ? arg1 : Buffers.getArray(arg1), arg1_is_direct ? Buffers.getDirectBufferByteOffset(arg1) : Buffers.getIndirectBufferByteOffset(arg1), arg1_is_direct, arg2, arg3_is_direct ? ( arg3 != null ? arg3.getBuffer() : null ) : Buffers.getArray(arg3), arg3_is_direct ? Buffers.getDirectBufferByteOffset(arg3) : Buffers.getIndirectBufferByteOffset(arg3), arg3_is_direct, arg4_is_direct ? arg4 : Buffers.getArray(arg4), arg4_is_direct ? Buffers.getDirectBufferByteOffset(arg4) : Buffers.getIndirectBufferByteOffset(arg4), arg4_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clCreateSubDevicesEXT}(cl_device_id, const cl_device_partition_property_ext * , cl_uint, cl_device_id * , cl_uint * )
@param arg1 a direct or array-backed {@link java.nio.LongBuffer}
@param arg3 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer}
@param arg4 a direct or array-backed {@link java.nio.IntBuffer} */
private native int dispatch_clCreateSubDevicesEXT1(long arg0, Object arg1, int arg1_byte_offset, boolean arg1_is_direct, int arg2, Object arg3, int arg3_byte_offset, boolean arg3_is_direct, Object arg4, int arg4_byte_offset, boolean arg4_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clCreateSubDevicesEXT}(cl_device_id, const cl_device_partition_property_ext * , cl_uint, cl_device_id * , cl_uint * )
@param arg3 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer} */
public int clCreateSubDevicesEXT(long arg0, long[] arg1, int arg1_offset, int arg2, PointerBuffer arg3, int[] arg4, int arg4_offset) {
if(arg1 != null && arg1.length <= arg1_offset)
throw new IllegalArgumentException("array offset argument \"arg1_offset\" (" + arg1_offset + ") equals or exceeds array length (" + arg1.length + ")");
final boolean arg3_is_direct = Buffers.isDirect(arg3);
if(arg4 != null && arg4.length <= arg4_offset)
throw new IllegalArgumentException("array offset argument \"arg4_offset\" (" + arg4_offset + ") equals or exceeds array length (" + arg4.length + ")");
final long __addr_ = addressTable._addressof_clCreateSubDevicesEXT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateSubDevicesEXT"));
}
return dispatch_clCreateSubDevicesEXT1(arg0, arg1, Buffers.SIZEOF_LONG * arg1_offset, false, arg2, arg3_is_direct ? ( arg3 != null ? arg3.getBuffer() : null ) : Buffers.getArray(arg3), arg3_is_direct ? Buffers.getDirectBufferByteOffset(arg3) : Buffers.getIndirectBufferByteOffset(arg3), arg3_is_direct, arg4, Buffers.SIZEOF_INT * arg4_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueMigrateMemObjectEXT}(cl_command_queue, cl_uint, const cl_mem * , cl_mem_migration_flags_ext, cl_uint, const cl_event * , cl_event * )
@param arg2 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer}
@param arg5 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer}
@param arg6 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueMigrateMemObjectEXT(long arg0, int arg1, PointerBuffer arg2, long arg3, int arg4, PointerBuffer arg5, PointerBuffer arg6) {
final boolean arg2_is_direct = Buffers.isDirect(arg2);
final boolean arg5_is_direct = Buffers.isDirect(arg5);
final boolean arg6_is_direct = Buffers.isDirect(arg6);
final long __addr_ = addressTable._addressof_clEnqueueMigrateMemObjectEXT;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueMigrateMemObjectEXT"));
}
return dispatch_clEnqueueMigrateMemObjectEXT1(arg0, arg1, arg2_is_direct ? ( arg2 != null ? arg2.getBuffer() : null ) : Buffers.getArray(arg2), arg2_is_direct ? Buffers.getDirectBufferByteOffset(arg2) : Buffers.getIndirectBufferByteOffset(arg2), arg2_is_direct, arg3, arg4, arg5_is_direct ? ( arg5 != null ? arg5.getBuffer() : null ) : Buffers.getArray(arg5), arg5_is_direct ? Buffers.getDirectBufferByteOffset(arg5) : Buffers.getIndirectBufferByteOffset(arg5), arg5_is_direct, arg6_is_direct ? ( arg6 != null ? arg6.getBuffer() : null ) : Buffers.getArray(arg6), arg6_is_direct ? Buffers.getDirectBufferByteOffset(arg6) : Buffers.getIndirectBufferByteOffset(arg6), arg6_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueMigrateMemObjectEXT}(cl_command_queue, cl_uint, const cl_mem * , cl_mem_migration_flags_ext, cl_uint, const cl_event * , cl_event * )
@param arg2 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer}
@param arg5 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer}
@param arg6 a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueMigrateMemObjectEXT1(long arg0, int arg1, Object arg2, int arg2_byte_offset, boolean arg2_is_direct, long arg3, int arg4, Object arg5, int arg5_byte_offset, boolean arg5_is_direct, Object arg6, int arg6_byte_offset, boolean arg6_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLBuffer}(cl_context context, cl_mem_flags flags, cl_GLuint bufobj, int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLBuffer(long context, long flags, int bufobj, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateFromGLBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLBuffer"));
}
return dispatch_clCreateFromGLBuffer1(context, flags, bufobj, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLBuffer}(cl_context context, cl_mem_flags flags, cl_GLuint bufobj, int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateFromGLBuffer1(long context, long flags, int bufobj, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLBuffer}(cl_context context, cl_mem_flags flags, cl_GLuint bufobj, int * errcode_ret)
*/
public long clCreateFromGLBuffer(long context, long flags, int bufobj, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateFromGLBuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLBuffer"));
}
return dispatch_clCreateFromGLBuffer1(context, flags, bufobj, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLTexture2D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLTexture2D(long context, long flags, int target, int miplevel, int texture, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateFromGLTexture2D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLTexture2D"));
}
return dispatch_clCreateFromGLTexture2D1(context, flags, target, miplevel, texture, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLTexture2D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateFromGLTexture2D1(long context, long flags, int target, int miplevel, int texture, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLTexture2D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
*/
public long clCreateFromGLTexture2D(long context, long flags, int target, int miplevel, int texture, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateFromGLTexture2D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLTexture2D"));
}
return dispatch_clCreateFromGLTexture2D1(context, flags, target, miplevel, texture, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLTexture3D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLTexture3D(long context, long flags, int target, int miplevel, int texture, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateFromGLTexture3D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLTexture3D"));
}
return dispatch_clCreateFromGLTexture3D1(context, flags, target, miplevel, texture, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLTexture3D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateFromGLTexture3D1(long context, long flags, int target, int miplevel, int texture, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLTexture3D}(cl_context context, cl_mem_flags flags, cl_GLenum target, cl_GLint miplevel, cl_GLuint texture, cl_int * errcode_ret)
*/
public long clCreateFromGLTexture3D(long context, long flags, int target, int miplevel, int texture, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateFromGLTexture3D;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLTexture3D"));
}
return dispatch_clCreateFromGLTexture3D1(context, flags, target, miplevel, texture, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLRenderbuffer}(cl_context context, cl_mem_flags flags, cl_GLuint renderbuffer, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateFromGLRenderbuffer(long context, long flags, int renderbuffer, IntBuffer errcode_ret) {
final boolean errcode_ret_is_direct = Buffers.isDirect(errcode_ret);
final long __addr_ = addressTable._addressof_clCreateFromGLRenderbuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLRenderbuffer"));
}
return dispatch_clCreateFromGLRenderbuffer1(context, flags, renderbuffer, errcode_ret_is_direct ? errcode_ret : Buffers.getArray(errcode_ret), errcode_ret_is_direct ? Buffers.getDirectBufferByteOffset(errcode_ret) : Buffers.getIndirectBufferByteOffset(errcode_ret), errcode_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLRenderbuffer}(cl_context context, cl_mem_flags flags, cl_GLuint renderbuffer, cl_int * errcode_ret)
@param errcode_ret a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateFromGLRenderbuffer1(long context, long flags, int renderbuffer, Object errcode_ret, int errcode_ret_byte_offset, boolean errcode_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_mem {@native clCreateFromGLRenderbuffer}(cl_context context, cl_mem_flags flags, cl_GLuint renderbuffer, cl_int * errcode_ret)
*/
public long clCreateFromGLRenderbuffer(long context, long flags, int renderbuffer, int[] errcode_ret, int errcode_ret_offset) {
if(errcode_ret != null && errcode_ret.length <= errcode_ret_offset)
throw new IllegalArgumentException("array offset argument \"errcode_ret_offset\" (" + errcode_ret_offset + ") equals or exceeds array length (" + errcode_ret.length + ")");
final long __addr_ = addressTable._addressof_clCreateFromGLRenderbuffer;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateFromGLRenderbuffer"));
}
return dispatch_clCreateFromGLRenderbuffer1(context, flags, renderbuffer, errcode_ret, Buffers.SIZEOF_INT * errcode_ret_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetGLObjectInfo}(cl_mem memobj, cl_gl_object_type * gl_object_type, cl_GLuint * gl_object_name)
@param gl_object_type a direct or array-backed {@link java.nio.IntBuffer}
@param gl_object_name a direct or array-backed {@link java.nio.IntBuffer} */
public int clGetGLObjectInfo(long memobj, IntBuffer gl_object_type, IntBuffer gl_object_name) {
final boolean gl_object_type_is_direct = Buffers.isDirect(gl_object_type);
final boolean gl_object_name_is_direct = Buffers.isDirect(gl_object_name);
final long __addr_ = addressTable._addressof_clGetGLObjectInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetGLObjectInfo"));
}
return dispatch_clGetGLObjectInfo1(memobj, gl_object_type_is_direct ? gl_object_type : Buffers.getArray(gl_object_type), gl_object_type_is_direct ? Buffers.getDirectBufferByteOffset(gl_object_type) : Buffers.getIndirectBufferByteOffset(gl_object_type), gl_object_type_is_direct, gl_object_name_is_direct ? gl_object_name : Buffers.getArray(gl_object_name), gl_object_name_is_direct ? Buffers.getDirectBufferByteOffset(gl_object_name) : Buffers.getIndirectBufferByteOffset(gl_object_name), gl_object_name_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetGLObjectInfo}(cl_mem memobj, cl_gl_object_type * gl_object_type, cl_GLuint * gl_object_name)
@param gl_object_type a direct or array-backed {@link java.nio.IntBuffer}
@param gl_object_name a direct or array-backed {@link java.nio.IntBuffer} */
private native int dispatch_clGetGLObjectInfo1(long memobj, Object gl_object_type, int gl_object_type_byte_offset, boolean gl_object_type_is_direct, Object gl_object_name, int gl_object_name_byte_offset, boolean gl_object_name_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetGLObjectInfo}(cl_mem memobj, cl_gl_object_type * gl_object_type, cl_GLuint * gl_object_name)
*/
public int clGetGLObjectInfo(long memobj, int[] gl_object_type, int gl_object_type_offset, int[] gl_object_name, int gl_object_name_offset) {
if(gl_object_type != null && gl_object_type.length <= gl_object_type_offset)
throw new IllegalArgumentException("array offset argument \"gl_object_type_offset\" (" + gl_object_type_offset + ") equals or exceeds array length (" + gl_object_type.length + ")");
if(gl_object_name != null && gl_object_name.length <= gl_object_name_offset)
throw new IllegalArgumentException("array offset argument \"gl_object_name_offset\" (" + gl_object_name_offset + ") equals or exceeds array length (" + gl_object_name.length + ")");
final long __addr_ = addressTable._addressof_clGetGLObjectInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetGLObjectInfo"));
}
return dispatch_clGetGLObjectInfo1(memobj, gl_object_type, Buffers.SIZEOF_INT * gl_object_type_offset, false, gl_object_name, Buffers.SIZEOF_INT * gl_object_name_offset, false, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetGLTextureInfo}(cl_mem memobj, cl_gl_texture_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct or array-backed {@link java.nio.Buffer}
@param param_value_size_ret a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetGLTextureInfo(long memobj, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
final boolean param_value_is_direct = Buffers.isDirect(param_value);
final boolean param_value_size_ret_is_direct = Buffers.isDirect(param_value_size_ret);
final long __addr_ = addressTable._addressof_clGetGLTextureInfo;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetGLTextureInfo"));
}
return dispatch_clGetGLTextureInfo1(memobj, param_name, param_value_size, param_value_is_direct ? param_value : Buffers.getArray(param_value), param_value_is_direct ? Buffers.getDirectBufferByteOffset(param_value) : Buffers.getIndirectBufferByteOffset(param_value), param_value_is_direct, param_value_size_ret_is_direct ? ( param_value_size_ret != null ? param_value_size_ret.getBuffer() : null ) : Buffers.getArray(param_value_size_ret), param_value_size_ret_is_direct ? Buffers.getDirectBufferByteOffset(param_value_size_ret) : Buffers.getIndirectBufferByteOffset(param_value_size_ret), param_value_size_ret_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetGLTextureInfo}(cl_mem memobj, cl_gl_texture_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param param_value a direct or array-backed {@link java.nio.Buffer}
@param param_value_size_ret a direct or array-backed {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetGLTextureInfo1(long memobj, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, boolean param_value_is_direct, Object param_value_size_ret, int param_value_size_ret_byte_offset, boolean param_value_size_ret_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueAcquireGLObjects}(cl_command_queue command_queue, cl_uint num_objects, const cl_mem * mem_objects, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mem_objects a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueAcquireGLObjects(long command_queue, int num_objects, PointerBuffer mem_objects, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(mem_objects))
throw new IllegalArgumentException("Argument \"mem_objects\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueAcquireGLObjects;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueAcquireGLObjects"));
}
return dispatch_clEnqueueAcquireGLObjects0(command_queue, num_objects, mem_objects != null ? mem_objects.getBuffer() : null, Buffers.getDirectBufferByteOffset(mem_objects), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueAcquireGLObjects}(cl_command_queue command_queue, cl_uint num_objects, const cl_mem * mem_objects, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mem_objects a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueAcquireGLObjects0(long command_queue, int num_objects, Object mem_objects, int mem_objects_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReleaseGLObjects}(cl_command_queue command_queue, cl_uint num_objects, const cl_mem * mem_objects, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mem_objects a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clEnqueueReleaseGLObjects(long command_queue, int num_objects, PointerBuffer mem_objects, int num_events_in_wait_list, PointerBuffer event_wait_list, PointerBuffer event) {
if (!Buffers.isDirect(mem_objects))
throw new IllegalArgumentException("Argument \"mem_objects\" is not a direct buffer");
if (!Buffers.isDirect(event_wait_list))
throw new IllegalArgumentException("Argument \"event_wait_list\" is not a direct buffer");
if (!Buffers.isDirect(event))
throw new IllegalArgumentException("Argument \"event\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clEnqueueReleaseGLObjects;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clEnqueueReleaseGLObjects"));
}
return dispatch_clEnqueueReleaseGLObjects0(command_queue, num_objects, mem_objects != null ? mem_objects.getBuffer() : null, Buffers.getDirectBufferByteOffset(mem_objects), num_events_in_wait_list, event_wait_list != null ? event_wait_list.getBuffer() : null, Buffers.getDirectBufferByteOffset(event_wait_list), event != null ? event.getBuffer() : null, Buffers.getDirectBufferByteOffset(event), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clEnqueueReleaseGLObjects}(cl_command_queue command_queue, cl_uint num_objects, const cl_mem * mem_objects, cl_uint num_events_in_wait_list, const cl_event * event_wait_list, cl_event * event)
@param mem_objects a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event_wait_list a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param event a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clEnqueueReleaseGLObjects0(long command_queue, int num_objects, Object mem_objects, int mem_objects_byte_offset, int num_events_in_wait_list, Object event_wait_list, int event_wait_list_byte_offset, Object event, int event_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetGLContextInfoKHR}(const cl_context_properties * properties, cl_gl_context_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param properties a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
public int clGetGLContextInfoKHR(PointerBuffer properties, int param_name, long param_value_size, Buffer param_value, PointerBuffer param_value_size_ret) {
if (!Buffers.isDirect(properties))
throw new IllegalArgumentException("Argument \"properties\" is not a direct buffer");
if (!Buffers.isDirect(param_value))
throw new IllegalArgumentException("Argument \"param_value\" is not a direct buffer");
if (!Buffers.isDirect(param_value_size_ret))
throw new IllegalArgumentException("Argument \"param_value_size_ret\" is not a direct buffer");
final long __addr_ = addressTable._addressof_clGetGLContextInfoKHR;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clGetGLContextInfoKHR"));
}
return dispatch_clGetGLContextInfoKHR0(properties != null ? properties.getBuffer() : null, Buffers.getDirectBufferByteOffset(properties), param_name, param_value_size, param_value, Buffers.getDirectBufferByteOffset(param_value), param_value_size_ret != null ? param_value_size_ret.getBuffer() : null, Buffers.getDirectBufferByteOffset(param_value_size_ret), __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_int {@native clGetGLContextInfoKHR}(const cl_context_properties * properties, cl_gl_context_info param_name, size_t param_value_size, void * param_value, size_t * param_value_size_ret)
@param properties a direct only {@link com.jogamp.common.nio.PointerBuffer}
@param param_value a direct only {@link java.nio.Buffer}
@param param_value_size_ret a direct only {@link com.jogamp.common.nio.PointerBuffer} */
private native int dispatch_clGetGLContextInfoKHR0(Object properties, int properties_byte_offset, int param_name, long param_value_size, Object param_value, int param_value_byte_offset, Object param_value_size_ret, int param_value_size_ret_byte_offset, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_event {@native clCreateEventFromGLsyncKHR}(cl_context, cl_GLsync, cl_int * )
@param arg2 a direct or array-backed {@link java.nio.IntBuffer} */
public long clCreateEventFromGLsyncKHR(long arg0, long arg1, IntBuffer arg2) {
final boolean arg2_is_direct = Buffers.isDirect(arg2);
final long __addr_ = addressTable._addressof_clCreateEventFromGLsyncKHR;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateEventFromGLsyncKHR"));
}
return dispatch_clCreateEventFromGLsyncKHR1(arg0, arg1, arg2_is_direct ? arg2 : Buffers.getArray(arg2), arg2_is_direct ? Buffers.getDirectBufferByteOffset(arg2) : Buffers.getIndirectBufferByteOffset(arg2), arg2_is_direct, __addr_);
}
/** Entry point (through function pointer) to C language function:
cl_event {@native clCreateEventFromGLsyncKHR}(cl_context, cl_GLsync, cl_int * )
@param arg2 a direct or array-backed {@link java.nio.IntBuffer} */
private native long dispatch_clCreateEventFromGLsyncKHR1(long arg0, long arg1, Object arg2, int arg2_byte_offset, boolean arg2_is_direct, long procAddress);
/** Entry point (through function pointer) to C language function:
cl_event {@native clCreateEventFromGLsyncKHR}(cl_context, cl_GLsync, cl_int * )
*/
public long clCreateEventFromGLsyncKHR(long arg0, long arg1, int[] arg2, int arg2_offset) {
if(arg2 != null && arg2.length <= arg2_offset)
throw new IllegalArgumentException("array offset argument \"arg2_offset\" (" + arg2_offset + ") equals or exceeds array length (" + arg2.length + ")");
final long __addr_ = addressTable._addressof_clCreateEventFromGLsyncKHR;
if (__addr_ == 0) {
throw new UnsupportedOperationException(String.format("Method \"%s\" not available", "clCreateEventFromGLsyncKHR"));
}
return dispatch_clCreateEventFromGLsyncKHR1(arg0, arg1, arg2, Buffers.SIZEOF_INT * arg2_offset, false, __addr_);
}
// --- Begin CustomJavaCode .cfg declarations
/** If null, OpenCL is not available on this machine. */
static final DynamicLibraryBundle dynamicLookupHelper;
protected static final CLProcAddressTable addressTable;
static {
addressTable = new CLProcAddressTable();
dynamicLookupHelper = AccessController.doPrivileged(new PrivilegedAction() {
public DynamicLibraryBundle run() {
final DynamicLibraryBundle bundle = new DynamicLibraryBundle(new CLDynamicLibraryBundleInfo());
if(!bundle.isToolLibLoaded()) {
// couldn't load native CL library
// TODO: log this?
return null;
}
if(!bundle.isLibComplete()) {
System.err.println("Couln't load native CL/JNI glue library");
return null;
}
addressTable.reset(bundle);
/** Not required nor forced
if( !initializeImpl() ) {
System.err.println("Native initialization failure of CL/JNI glue library");
return null;
} */
return bundle;
} } );
}
/**
* Accessor.
* @returns true if OpenCL is available on this machine.
*/
public static boolean isAvailable() { return dynamicLookupHelper != null; }
public static CLProcAddressTable getCLProcAddressTable() { return addressTable; }
static long clGetExtensionFunctionAddress(long clGetExtensionFunctionAddressHandle, java.lang.String procname)
{
if (clGetExtensionFunctionAddressHandle == 0) {
throw new RuntimeException("Passed null pointer for method \"clGetExtensionFunctionAddress\"");
}
return dispatch_clGetExtensionFunctionAddressStatic(procname, clGetExtensionFunctionAddressHandle);
}
public CLAbstractImpl() {
}
/** Entry point (through function pointer) to C language function:
void* clGetExtensionFunctionAddress(const char * fname);
*/
long clGetExtensionFunctionAddress(String fname) {
final long __addr_ = addressTable._addressof_clGetExtensionFunctionAddress;
if (__addr_ == 0) {
throw new UnsupportedOperationException("Method \"clGetExtensionFunctionAddress\" not available");
}
return dispatch_clGetExtensionFunctionAddressStatic(fname, __addr_);
}
/** Entry point (through function pointer) to C language function:
void* clGetExtensionFunctionAddress(const char * fname);
*/
private static native long dispatch_clGetExtensionFunctionAddressStatic(String fname, long procAddress);
// ---- End CustomJavaCode .cfg declarations
} // end of class CLAbstractImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy