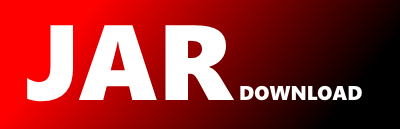
org.joinedworkz.ux.angular.AngularCartridge.xtend Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ux-angular Show documentation
Show all versions of ux-angular Show documentation
DSL based modeling framework - facilities angular
The newest version!
package org.joinedworkz.ux.angular
import java.util.Properties
import java.util.Set
import javax.inject.Inject
import javax.inject.Singleton
import org.joinedworkz.common.helper.CmnModelHelper
import org.joinedworkz.common.helper.OpenApiHelper
import org.joinedworkz.core.facility.AbstractCartridge
import org.joinedworkz.core.facility.Outlet
import org.joinedworkz.core.facility.Outlets
import org.joinedworkz.core.model.CmnComplexType
import org.joinedworkz.core.model.CmnComponent
import org.joinedworkz.core.model.CmnModel
import org.joinedworkz.core.model.CmnObject
import org.joinedworkz.core.model.CmnResource
import org.joinedworkz.core.model.CmnType
import org.joinedworkz.core.model.data.CmnReferenceData
import org.joinedworkz.core.model.ux.CmnPage
import org.joinedworkz.ux.angular.context.PageGeneratorContext
import org.joinedworkz.ux.angular.context.ServiceGeneratorContext
import org.joinedworkz.ux.angular.context.TypeScriptGeneratorContext
import org.joinedworkz.ux.angular.generator.ModelGenerator
import org.joinedworkz.ux.angular.generator.PageGenerator
import org.joinedworkz.ux.angular.generator.RoutesGenerator
import org.joinedworkz.ux.angular.generator.ServiceGenerator
import org.joinedworkz.ux.angular.generator.StoreGenerator
import org.slf4j.Logger
import org.slf4j.LoggerFactory
@Singleton
class AngularCartridge extends AbstractCartridge {
final static Logger log = LoggerFactory.getLogger(AngularCartridge);
@Inject
protected extension CmnModelHelper cmnModelHelper
@Inject
protected RoutesGenerator routesGenerator;
@Inject
protected StoreGenerator storeGenerator
@Inject
protected PageGenerator pageGenerator;
@Inject
protected ModelGenerator modelGenerator;
@Inject
protected ServiceGenerator serviceGenerator;
@Inject
extension OpenApiHelper
override apply(CmnObject cmnObj, Outlets outlets) {
apply(cmnObj, outlets, null)
}
override void apply(CmnObject obj, Outlets outlets, Properties properties) {
log.info("Apply cartridge: '{}'", class.canonicalName)
val generatedJavaSourceSlot = outlets.get("generatedAngularSource")
if (obj instanceof CmnModel) {
log.info("Invoke generator '{}' on model '{}'", pageGenerator.class.canonicalName, obj.namespace)
for (modelElement : obj.modelElements) {
modelElement.generateModelElement(generatedJavaSourceSlot, properties)
}
}
}
def void generateModelElement(CmnObject obj, Outlet outlet, Properties properties) {
if (obj instanceof CmnComponent) {
if (!obj.hasStereoType("ux")) {
return
}
if (!obj.usedData.empty) {
var usedResources = newLinkedHashSet
for (usedDataItem : obj.usedData) {
if (usedDataItem instanceof CmnReferenceData) {
if (usedDataItem.resourcePath !== null) {
val usedResource = usedDataItem.resourcePath.get(0).resource
usedResources.add(usedResource)
val component = usedDataItem.referencedComponent
/* base package (default is namespace of component) */
var basePackage = component.getString('basePackage')
if (basePackage === null) {
basePackage = component.model.namespace
}
val usedResourcesWithServices = newLinkedHashSet
usedResource.collectResourcesWithServices(usedResourcesWithServices)
for (resourceWithService : usedResourcesWithServices) {
val ctx = createServiceGeneratorContext(basePackage, usedResource, resourceWithService, outlet, properties)
ctx.mergedOperationInfos = createMergedResourceOperationInfos(component, resourceWithService, ctx)
serviceGenerator.generate(obj, resourceWithService, ctx)
}
}
}
}
var usedTypes = usedResources.flatMap[collectUsedTypes].toSet
for (usedType : usedTypes.filter(CmnComplexType)) {
modelGenerator.generate(obj, usedType, createTypeScriptGeneratorContext(outlet, properties))
}
}
if (!obj.routes.empty) {
routesGenerator.generateRoutes(obj, obj.routes, createTypeScriptGeneratorContext(outlet, properties))
}
// if (obj.propertyDefinitions !== null) {
// storeGenerator.generateStore(obj, obj.propertyDefinitions, createGeneratorContext(outlet))
// }
if (obj.features !== null) {
val pages = obj.features.filter(CmnPage)
for (page : pages) {
pageGenerator.generatePage(obj, page, createPageGeneratorContext(outlet, properties))
}
}
}
}
def void collectResourcesWithServices(CmnResource resource, Set collectedResources) {
if (resource.name !== null && resource.representation !== null) {
collectedResources.add(resource)
}
if (resource.subResources !== null) {
for (subResource : resource.subResources) {
collectResourcesWithServices(subResource, collectedResources)
}
}
}
def Set collectUsedTypes(CmnResource resource) {
val usedTypes = newLinkedHashSet
collectUsedTypes(usedTypes, resource)
return usedTypes
}
def void collectUsedTypes(Set usedTypes, CmnResource resource) {
if (resource.representation !== null) {
collectUsedTypes(usedTypes, resource.representation)
}
if (resource.operations !== null) {
for (operation : resource.operations) {
val consumes = operation.getPropertyValue("consumes")
if (consumes instanceof CmnComplexType) {
collectUsedTypes(usedTypes, consumes)
}
if (operation.responses !== null) {
for (response : operation.responses) {
if (response.content !== null) {
val responseType = response.content.type
if (responseType !== null) {
collectUsedTypes(usedTypes, responseType)
}
}
}
}
}
}
if (resource.subResources !== null) {
for (subResource : resource.subResources) {
collectUsedTypes(usedTypes, subResource)
}
}
}
def void collectUsedTypes(Set usedTypes, CmnType type) {
if (!usedTypes.contains(type)) {
usedTypes.add(type)
if (type instanceof CmnComplexType) {
for (field : type.fields) {
collectUsedTypes(usedTypes, field.type)
}
}
}
}
def PageGeneratorContext createPageGeneratorContext(Outlet outlet, Properties properties) {
return new PageGeneratorContext(outlet, properties)
}
def TypeScriptGeneratorContext createTypeScriptGeneratorContext(Outlet outlet, Properties properties) {
return new TypeScriptGeneratorContext(outlet, properties)
}
def ServiceGeneratorContext createServiceGeneratorContext(String basePackage, CmnResource rootResource, CmnResource mainResource, Outlet outlet, Properties properties) {
return new ServiceGeneratorContext(basePackage, rootResource, mainResource, outlet, properties)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy