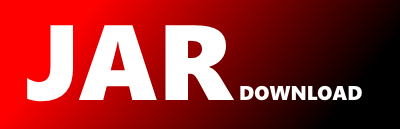
org.joinfaces.autoconfigure.javaxfaces.JsfBeansAutoConfiguration Maven / Gradle / Ivy
/*
* Copyright 2016-2022 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joinfaces.autoconfigure.javaxfaces;
import java.util.Map;
import jakarta.faces.annotation.ApplicationMap;
import jakarta.faces.application.ResourceHandler;
import jakarta.faces.component.UIViewRoot;
import jakarta.faces.context.ExternalContext;
import jakarta.faces.context.FacesContext;
import jakarta.faces.context.Flash;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.config.ConfigurableBeanFactory;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnWebApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Scope;
import org.springframework.context.annotation.ScopedProxyMode;
/**
* JSF 2.3-like Bean Definitions.
*
* These bean definitions are taken from the mojarra {@link com.sun.faces.cdi.CdiProducer}s.
*
* @author Lars Grefer
* @see http://arjan-tijms.omnifaces.org/p/jsf-23.html#1316
*/
@Configuration
@ConditionalOnWebApplication(type = ConditionalOnWebApplication.Type.SERVLET)
@ConditionalOnClass(FacesContext.class)
public class JsfBeansAutoConfiguration {
@Bean("application")
@ConditionalOnMissingBean(name = "application")
@Scope(ConfigurableBeanFactory.SCOPE_PROTOTYPE)
public Object application() {
return FacesContext.getCurrentInstance().getExternalContext().getContext();
}
@Bean("applicationScope")
@ConditionalOnMissingBean(name = "applicationScope")
@Scope(value = ConfigurableBeanFactory.SCOPE_PROTOTYPE, proxyMode = ScopedProxyMode.INTERFACES)
public Map applicationMap() {
return FacesContext.getCurrentInstance().getExternalContext().getApplicationMap();
}
@Bean("cookie")
@ConditionalOnMissingBean(name = "cookie")
@Scope(value = ConfigurableBeanFactory.SCOPE_PROTOTYPE, proxyMode = ScopedProxyMode.INTERFACES)
public Map requestCookieMap() {
return FacesContext.getCurrentInstance().getExternalContext().getRequestCookieMap();
}
/**
* Spring bean definition for the JSF {@link FacesContext}.
*
* @return The current {@link FacesContext#getCurrentInstance() FacesContext}.
* @see com.sun.faces.cdi.FacesContextProducer
*/
@Bean("facesContext")
@Scope(value = ConfigurableBeanFactory.SCOPE_PROTOTYPE, proxyMode = ScopedProxyMode.TARGET_CLASS)
@ConditionalOnMissingBean
public FacesContext facesContext() {
return FacesContext.getCurrentInstance();
}
@Bean("flash")
@Scope(value = ConfigurableBeanFactory.SCOPE_PROTOTYPE, proxyMode = ScopedProxyMode.TARGET_CLASS)
@ConditionalOnMissingBean
public Flash flash() {
return FacesContext.getCurrentInstance().getExternalContext().getFlash();
}
@Bean("flowScope")
@ConditionalOnMissingBean(name = "flowScope")
@Scope(value = ConfigurableBeanFactory.SCOPE_PROTOTYPE, proxyMode = ScopedProxyMode.INTERFACES)
public Map