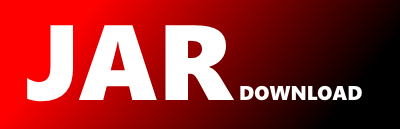
org.jolokia.service.serializer.json.MapExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jolokia-service-serializer Show documentation
Show all versions of jolokia-service-serializer Show documentation
Jolokia :: Service :: JSON Serializer
The newest version!
package org.jolokia.service.serializer.json;
import java.lang.reflect.InvocationTargetException;
import java.util.Deque;
import java.util.LinkedList;
import java.util.Map;
import javax.management.AttributeNotFoundException;
import org.jolokia.server.core.service.serializer.ValueFaultHandler;
import org.jolokia.service.serializer.object.StringToObjectConverter;
import org.jolokia.json.JSONObject;
/*
* Copyright 2009-2013 Roland Huss
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* Extractor for Maps (which turns {@link Map} into {@link JSONObject})
*
* @author roland
* @since Apr 19, 2009
*/
public class MapExtractor implements Extractor {
private static final int MAX_STRING_LENGTH = 400;
/** {@inheritDoc} */
public Class> getType() {
return Map.class;
}
/**
* Convert a Map to JSON (if jsonify
is true
). If a path is used, the
* path is interpreted as a key into the map. The key in the path is a string and is compared agains
* all keys in the map against their string representation.
*
* @param pConverter the global converter in order to be able do dispatch for
* serializing inner data types
* @param pValue the value to convert which must be a {@link Map}
* @param pPathParts extra argument stack which on top must be a key into the map
* @param jsonify whether to convert to a JSON object/list or whether the plain object
* should be returned. The later is required for writing an inner value
* @return the extracted object
* @throws AttributeNotFoundException
*/
public Object extractObject(ObjectToJsonConverter pConverter, Object pValue,
Deque pPathParts,boolean jsonify) throws AttributeNotFoundException {
// the map is not necessarily using string keys
// see https://github.com/jolokia/jolokia/issues/732
Map, ?> map = (Map, ?>) pValue;
int length = pConverter.getCollectionLength(map.size());
String pathParth = pPathParts.isEmpty() ? null : pPathParts.pop();
if (pathParth != null) {
return extractMapValueWithPath(pConverter, map, pPathParts, jsonify, map, pathParth);
} else {
return jsonify ? extractMapValues(pConverter, pPathParts, jsonify, map, length) : map;
}
}
private JSONObject extractMapValues(ObjectToJsonConverter pConverter, Deque pPathParts, boolean jsonify, Map, ?> pMap, int pLength) throws AttributeNotFoundException {
JSONObject ret = new JSONObject();
int i = 0;
for(Map.Entry, ?> entry : pMap.entrySet()) {
Deque paths = new LinkedList<>(pPathParts);
try {
ret.put(entry.getKey().toString(),
pConverter.extractObject(entry.getValue(), paths, jsonify));
if (++i > pLength) {
break;
}
} catch (ValueFaultHandler.AttributeFilteredException exp) {
// Filtered out ...
}
}
if (ret.isEmpty() && pLength > 0) {
// Not a single value passed the filter
throw new ValueFaultHandler.AttributeFilteredException();
}
return ret;
}
private Object extractMapValueWithPath(ObjectToJsonConverter pConverter, Object pValue, Deque pPathParts, boolean jsonify, Map, ?> pMap, String pPathParth) throws AttributeNotFoundException {
for (Map.Entry, ?> entry : pMap.entrySet()) {
// We dont access the map via a lookup since the key
// are potentially object but we have to deal with string
// representations
if(pPathParth.equals(entry.getKey().toString())) {
return pConverter.extractObject(entry.getValue(), pPathParts, jsonify);
}
}
ValueFaultHandler faultHandler = pConverter.getValueFaultHandler();
return faultHandler.handleException(
new AttributeNotFoundException("Map key '" + pPathParth +
"' is unknown for map " + trimString(pValue.toString())));
}
/**
* Set the value within a map, where the attribute is taken as key into the map.
*
* @param pConverter the global converter in order to be able do dispatch for
* serializing inner data types
* @param pMap map on which to set the value
* @param pKey key in the map where to put the value
* @param pValue the new value to set
* @return the old value or null
if a new map entry was created/
* @throws IllegalAccessException
* @throws InvocationTargetException
*/
public Object setObjectValue(StringToObjectConverter pConverter, Object pMap, String pKey, Object pValue)
throws IllegalAccessException, InvocationTargetException {
@SuppressWarnings("unchecked")
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy