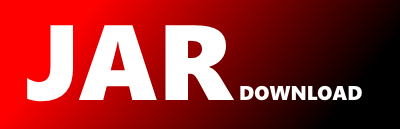
org.jomc.logging.it.LoggerTest Maven / Gradle / Ivy
Show all versions of jomc-logging-it Show documentation
// SECTION-START[License Header]
//
/*
* Java Object Management and Configuration
* Copyright (C) Christian Schulte, 2005-206
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* o Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* o Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* THIS SOFTWARE IS PROVIDED "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY
* AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL
* THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* $JOMC: LoggerTest.java 4808 2013-09-25 17:50:53Z schulte $
*
*/
//
// SECTION-END
package org.jomc.logging.it;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.logging.LogManager;
import org.junit.Test;
import org.junit.runner.JUnitCore;
import static org.junit.Assert.fail;
// SECTION-START[Documentation]
//
/**
* Object management and configuration logging system test suite.
*
*
* - Identifier:
- org.jomc.logging.it.LoggerTest
* - Name:
- JOMC Logging ⁑ Test Suite
* - Abstract:
- No
* - Final:
- No
* - Stateless:
- Yes
*
*
* @author Christian Schulte 1.0
* @version 1.3
*/
//
// SECTION-END
// SECTION-START[Annotations]
//
@javax.annotation.Generated( value = "org.jomc.tools.SourceFileProcessor 1.5", comments = "See http://www.jomc.org/jomc/1.5/jomc-tools-1.5" )
//
// SECTION-END
public class LoggerTest
{
// SECTION-START[Constructors]
//
/** Creates a new {@code LoggerTest} instance. */
@javax.annotation.Generated( value = "org.jomc.tools.SourceFileProcessor 1.5", comments = "See http://www.jomc.org/jomc/1.5/jomc-tools-1.5" )
public LoggerTest()
{
// SECTION-START[Default Constructor]
super();
// SECTION-END
}
//
// SECTION-END
// SECTION-START[LoggerTest]
/**
* Tests the {@link org.jomc.logging.Logger#isInfoEnabled() isXxxEnabled()} methods to not throw any exceptions.
*
* @throws Exception if testing fails.
*/
@Test public void testIsEnabled() throws Exception
{
if ( this.getLogger() != null )
{
this.getLogger().isConfigEnabled();
this.getLogger().isDebugEnabled();
this.getLogger().isErrorEnabled();
this.getLogger().isFatalEnabled();
this.getLogger().isInfoEnabled();
this.getLogger().isTraceEnabled();
this.getLogger().isWarnEnabled();
}
else
{
fail( this.getTestImplementationNotFoundMessage( this.getLocale() ) );
}
}
/**
* Tests the various logger methods to not throw any exceptions.
*
* @throws Exception if testing fails.
*/
@Test public void testLog() throws Exception
{
if ( this.getLogger() != null )
{
this.getLogger().config( "TEST" );
this.getLogger().config( new Exception() );
this.getLogger().config( "TEST", new Exception() );
this.getLogger().debug( "TEST" );
this.getLogger().debug( new Exception() );
this.getLogger().debug( "TEST", new Exception() );
this.getLogger().error( "TEST" );
this.getLogger().error( new Exception() );
this.getLogger().error( "TEST", new Exception() );
this.getLogger().fatal( "TEST" );
this.getLogger().fatal( new Exception() );
this.getLogger().fatal( "TEST", new Exception() );
this.getLogger().info( "TEST" );
this.getLogger().info( new Exception() );
this.getLogger().info( "TEST", new Exception() );
this.getLogger().trace( "TEST" );
this.getLogger().trace( new Exception() );
this.getLogger().trace( "TEST", new Exception() );
this.getLogger().warn( "TEST" );
this.getLogger().warn( new Exception() );
this.getLogger().warn( "TEST", new Exception() );
}
else
{
fail( this.getTestImplementationNotFoundMessage( this.getLocale() ) );
}
}
/**
* Test runner entry point.
* This method sets up the JDK's {@code LogManager} with properties found at class path location
* {@code "/logging.properties"} and executes {@link JUnitCore#main} passing the given arguments with this classes
* name prepended.
*
* @param args Command line arguments.
*/
public static void main( final String... args )
{
try
{
final URL loggingProperties = LoggerTest.class.getResource( "/logging.properties" );
if ( loggingProperties != null )
{
final InputStream in = loggingProperties.openStream();
LogManager.getLogManager().readConfiguration( in );
in.close();
}
final List l = new ArrayList( Arrays.asList( args ) );
l.add( 0, LoggerTest.class.getName() );
JUnitCore.main( l.toArray( new String[ l.size() ] ) );
}
catch ( final IOException e )
{
e.printStackTrace();
System.exit( 1 );
}
}
// SECTION-END
// SECTION-START[Dependencies]
//
/**
* Gets the {@code } dependency.
*
* This method returns the {@code } object of the {@code } specification at specification level 1.1.
* That specification does not apply to any scope. A new object is returned whenever requested and bound to this instance.
*
*
* - Final:
- No
*
* @return The {@code } dependency.
* @throws org.jomc.ObjectManagementException if getting the dependency instance fails.
*/
@SuppressWarnings("unused")
@javax.annotation.Generated( value = "org.jomc.tools.SourceFileProcessor 1.5", comments = "See http://www.jomc.org/jomc/1.5/jomc-tools-1.5" )
private java.util.Locale getLocale()
{
final java.util.Locale _d = (java.util.Locale) org.jomc.ObjectManagerFactory.getObjectManager( this.getClass().getClassLoader() ).getDependency( this, "Locale" );
assert _d != null : "'Locale' dependency not found.";
return _d;
}
/**
* Gets the {@code } dependency.
*
* This method returns any available object of the {@code } specification at specification level 1.1.
* That specification does not apply to any scope. A new object is returned whenever requested and bound to this instance.
*
* Properties:
*
*
* Name
* Type
* Documentation
*
*
* {@code }
* {@code java.lang.String}
*
*
*
*
*
* - Final:
- No
*
* @return Implementation tests are performed with.
* {@code null} if no object is available.
* @throws org.jomc.ObjectManagementException if getting the dependency instance fails.
*/
@SuppressWarnings("unused")
@javax.annotation.Generated( value = "org.jomc.tools.SourceFileProcessor 1.5", comments = "See http://www.jomc.org/jomc/1.5/jomc-tools-1.5" )
private org.jomc.logging.Logger getLogger()
{
return (org.jomc.logging.Logger) org.jomc.ObjectManagerFactory.getObjectManager( this.getClass().getClassLoader() ).getDependency( this, "Logger" );
}
//
// SECTION-END
// SECTION-START[Properties]
// SECTION-END
// SECTION-START[Messages]
//
/**
* Gets the text of the {@code } message.
*
* - Languages:
* - English (default)
* - Deutsch
* - Final:
- Yes
*
* @param locale The locale of the message to return.
* @return The text of the {@code } message for {@code locale}.
* @throws org.jomc.ObjectManagementException if getting the message instance fails.
*/
@SuppressWarnings("unused")
@javax.annotation.Generated( value = "org.jomc.tools.SourceFileProcessor 1.5", comments = "See http://www.jomc.org/jomc/1.5/jomc-tools-1.5" )
private String getTestImplementationNotFoundMessage( final java.util.Locale locale )
{
final String _m = org.jomc.ObjectManagerFactory.getObjectManager( this.getClass().getClassLoader() ).getMessage( this, "Test Implementation Not Found Message", locale );
assert _m != null : "'Test Implementation Not Found Message' message not found.";
return _m;
}
//
// SECTION-END
}