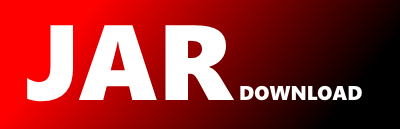
org.jomc.mojo.AbstractJomcMojo Maven / Gradle / Ivy
Show all versions of maven-jomc-plugin Show documentation
/*
* Copyright (C) Christian Schulte, 2005-206
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* o Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* o Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* THIS SOFTWARE IS PROVIDED "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY
* AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL
* THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* $JOMC: AbstractJomcMojo.java 5005 2014-11-17 03:00:17Z schulte $
*
*/
package org.jomc.mojo;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.io.StringWriter;
import java.net.MalformedURLException;
import java.net.SocketTimeoutException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.net.URLClassLoader;
import java.net.URLConnection;
import java.util.Collection;
import java.util.Date;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.logging.Level;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.transform.ErrorListener;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerConfigurationException;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.stream.StreamSource;
import org.apache.commons.lang.StringEscapeUtils;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.ArtifactUtils;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugin.descriptor.MojoDescriptor;
import org.apache.maven.project.MavenProject;
import org.jomc.model.Module;
import org.jomc.model.Modules;
import org.jomc.model.modlet.DefaultModelProcessor;
import org.jomc.model.modlet.DefaultModelProvider;
import org.jomc.model.modlet.DefaultModelValidator;
import org.jomc.model.modlet.ModelHelper;
import org.jomc.modlet.DefaultModelContext;
import org.jomc.modlet.DefaultModletProvider;
import org.jomc.modlet.Model;
import org.jomc.modlet.ModelContext;
import org.jomc.modlet.ModelContextFactory;
import org.jomc.modlet.ModelException;
import org.jomc.modlet.ModelValidationReport;
import org.jomc.modlet.Modlet;
import org.jomc.modlet.Modlets;
import org.jomc.tools.ClassFileProcessor;
import org.jomc.tools.JomcTool;
import org.jomc.tools.ResourceFileProcessor;
import org.jomc.tools.SourceFileProcessor;
import org.jomc.tools.modlet.ToolsModelProcessor;
import org.jomc.tools.modlet.ToolsModelProvider;
/**
* Base class for executing {@code JomcTool}s.
*
* @author Christian Schulte
* @version $JOMC: AbstractJomcMojo.java 5005 2014-11-17 03:00:17Z schulte $
*/
public abstract class AbstractJomcMojo extends AbstractMojo
{
/**
* The encoding to use for reading and writing files.
*
* @parameter default-value="${project.build.sourceEncoding}" expression="${jomc.sourceEncoding}"
*/
private String sourceEncoding;
/**
* The encoding to use for reading templates.
* Deprecated: As of JOMC 1.3, please use the 'defaultTemplateEncoding' parameter. This
* parameter will be removed in version 2.0.
*
* @parameter expression="${jomc.templateEncoding}"
*/
@Deprecated
private String templateEncoding;
/**
* The encoding to use for reading templates.
*
* @parameter expression="${jomc.defaultTemplateEncoding}"
*
* @since 1.3
*/
private String defaultTemplateEncoding;
/**
* Location to search for templates in addition to searching the class path of the plugin.
* First an attempt is made to parse the location value to an URL. On successful parsing, that URL is used.
* Otherwise the location value is interpreted as a directory name relative to the base directory of the project.
* If that directory exists, that directory is used. If nothing is found at the given location, a warning message is
* logged.
*
* @parameter expression="${jomc.templateLocation}"
* @since 1.2
*/
private String templateLocation;
/**
* The template profile to use when accessing templates.
*
* @parameter expression="${jomc.templateProfile}"
*/
private String templateProfile;
/**
* The default template profile to use when accessing templates.
*
* @parameter expression="${jomc.defaultTemplateProfile}"
*/
private String defaultTemplateProfile;
/**
* The location to search for providers.
*
* @parameter expression="${jomc.providerLocation}"
*/
private String providerLocation;
/**
* The location to search for platform providers.
*
* @parameter expression="${jomc.platformProviderLocation}"
*/
private String platformProviderLocation;
/**
* The identifier of the model to process.
*
* @parameter default-value="http://jomc.org/model" expression="${jomc.model}"
*/
private String model;
/**
* The name of the {@code ModelContextFactory} implementation class backing the task.
*
* @parameter expression="${jomc.modelContextFactoryClassName}"
* @since 1.2
*/
private String modelContextFactoryClassName;
/**
* The location to search for modlets.
*
* @parameter expression="${jomc.modletLocation}"
*/
private String modletLocation;
/**
* The {@code http://jomc.org/modlet} namespace schema system id.
*
* @parameter expression="${jomc.modletSchemaSystemId}"
* @since 1.2
*/
private String modletSchemaSystemId;
/**
* The location to search for modules.
*
* @parameter expression="${jomc.moduleLocation}"
*/
private String moduleLocation;
/**
* The location to search for transformers.
*
* @parameter expression="${jomc.transformerLocation}"
*/
private String transformerLocation;
/**
* The indentation string ('\t' for tab).
*
* @parameter expression="${jomc.indentation}"
*/
private String indentation;
/**
* The line separator ('\r\n' for DOS, '\r' for Mac, '\n' for Unix).
*
* @parameter expression="${jomc.lineSeparator}"
*/
private String lineSeparator;
/**
* The locale.
*
* <locale>
* <language>Lowercase two-letter ISO-639 code.</language>
* <country>Uppercase two-letter ISO-3166 code.</country>
* <variant>Vendor and browser specific code.</variant>
* </locale>
*
*
* @parameter
* @since 1.2
* @see Locale
*/
private LocaleType locale;
/**
* Controls verbosity of the plugin.
*
* @parameter expression="${jomc.verbose}" default-value="false"
*/
private boolean verbose;
/**
* Controls processing of source code files.
*
* @parameter expression="${jomc.sourceProcessing}" default-value="true"
*/
private boolean sourceProcessingEnabled;
/**
* Controls processing of resource files.
*
* @parameter expression="${jomc.resourceProcessing}" default-value="true"
*/
private boolean resourceProcessingEnabled;
/**
* Controls processing of class files.
*
* @parameter expression="${jomc.classProcessing}" default-value="true"
*/
private boolean classProcessingEnabled;
/**
* Controls processing of models.
*
* @parameter expression="${jomc.modelProcessing}" default-value="true"
*/
private boolean modelProcessingEnabled;
/**
* Controls model object class path resolution.
*
* @parameter expression="${jomc.modelObjectClasspathResolution}" default-value="true"
*/
private boolean modelObjectClasspathResolutionEnabled;
/**
* Name of the module to process.
*
* @parameter default-value="${project.name}" expression="${jomc.moduleName}"
*/
private String moduleName;
/**
* Name of the test module to process.
*
* @parameter default-value="${project.name} Tests" expression="${jomc.testModuleName}"
*/
private String testModuleName;
/**
* Directory holding the compiled class files of the project.
* Deprecated: As of JOMC 1.1, please use the 'outputDirectory' parameter. This parameter will
* be removed in version 2.0.
*
* @parameter
*/
@Deprecated
private String classesDirectory;
/**
* Directory holding the compiled test class files of the project.
* Deprecated: As of JOMC 1.1, please use the 'testOutputDirectory' parameter. This parameter
* will be removed in version 2.0.
*
* @parameter
*/
@Deprecated
private String testClassesDirectory;
/**
* Output directory of the project.
*
* @parameter default-value="${project.build.outputDirectory}" expression="${jomc.outputDirectory}"
* @since 1.1
*/
private String outputDirectory;
/**
* Test output directory of the project.
*
* @parameter default-value="${project.build.testOutputDirectory}" expression="${jomc.testOutputDirectory}"
* @since 1.1
*/
private String testOutputDirectory;
/**
* Directory holding the source files of the project.
*
* @parameter default-value="${project.build.sourceDirectory}" expression="${jomc.sourceDirectory}"
* @since 1.1
*/
private String sourceDirectory;
/**
* Directory holding the test source files of the project.
*
* @parameter default-value="${project.build.testSourceDirectory}" expression="${jomc.testSourceDirectory}"
* @since 1.1
*/
private String testSourceDirectory;
/**
* Directory holding the session related files of the project.
*
* @parameter default-value="${project.build.directory}/jomc-sessions" expression="${jomc.sessionDirectory}"
* @since 1.1
*/
private String sessionDirectory;
/**
* Directory holding the reports of the project.
*
* @parameter default-value="${project.reporting.outputDirectory}" expression="${jomc.reportOutputDirectory}"
* @since 1.1
*/
private String reportOutputDirectory;
/**
* Velocity runtime properties.
*
* <velocityProperties>
* <velocityProperty>
* <key>The name of the property.</key>
* <value>The value of the property.</value>
* <type>The name of the class of the properties object.</type>
* </velocityProperty>
* </velocityProperties>
*
*
* @parameter
* @since 1.2
*/
private List velocityProperties;
/**
* Velocity runtime property resources.
*
* <velocityPropertyResources>
* <velocityPropertyResource>
* <location>The location of the properties resource.</location>
* <optional>Flag indicating the properties resource is optional.</optional>
* <format>The format of the properties resource.</format>
* <connectTimeout>Timeout value, in milliseconds.</connectTimeout>
* <readTimeout>Timeout value, in milliseconds.</readTimeout>
* </velocityPropertyResource>
* </velocityPropertyResources>
*
* The location value is used to first search the class path of the plugin and the project's main or test class
* path. If a class path resource is found, that resource is used. If no class path resource is found, an attempt is
* made to parse the location value to an URL. On successful parsing, that URL is used. Otherwise the location value
* is interpreted as a file name relative to the base directory of the project. If that file exists, that file is
* used. If nothing is found at the given location, depending on the optional flag, a warning message is logged or a
* build failure is produced.
* The optional flag is used to flag the resource optional. When an optional resource is not found, a warning
* message is logged instead of producing a build failure.
Default value is: false
* The format value is used to specify the format of the properties resource. Supported values are {@code plain}
* and {@code xml}.
Default value is: plain
* The connectTimeout value is used to specify the timeout, in milliseconds, to be used when opening
* communications links to the resource. A timeout of zero is interpreted as an infinite timeout.
* Default value is: 60000
* The readTimeout value is used to specify the timeout, in milliseconds, to be used when reading the resource.
* A timeout of zero is interpreted as an infinite timeout.
* Default value is: 60000
*
* @parameter
* @since 1.2
*/
private List velocityPropertyResources;
/**
* Template parameters.
*
* <templateParameters>
* <templateParameter>
* <key>The name of the parameter.</key>
* <value>The value of the parameter.</value>
* <type>The name of the class of the parameter's object.</type>
* </templateParameter>
* </templateParameters>
*
*
* @parameter
* @since 1.2
*/
private List templateParameters;
/**
* Template parameter resources.
*
* <templateParameterResources>
* <templateParameterResource>
* <location>The location of the properties resource.</location>
* <optional>Flag indicating the properties resource is optional.</optional>
* <format>The format of the properties resource.</format>
* <connectTimeout>Timeout value, in milliseconds.</connectTimeout>
* <readTimeout>Timeout value, in milliseconds.</readTimeout>
* </templateParameterResource>
* </templateParameterResources>
*
* The location value is used to first search the class path of the plugin and the project's main or test class
* path. If a class path resource is found, that resource is used. If no class path resource is found, an attempt is
* made to parse the location value to an URL. On successful parsing, that URL is used. Otherwise the location value
* is interpreted as a file name relative to the base directory of the project. If that file exists, that file is
* used. If nothing is found at the given location, depending on the optional flag, a warning message is logged or a
* build failure is produced.
* The optional flag is used to flag the resource optional. When an optional resource is not found, a warning
* message is logged instead of producing a build failure.
Default value is: false
* The format value is used to specify the format of the properties resource. Supported values are {@code plain}
* and {@code xml}.
Default value is: plain
* The connectTimeout value is used to specify the timeout, in milliseconds, to be used when opening
* communications links to the resource. A timeout of zero is interpreted as an infinite timeout.
* Default value is: 60000
* The readTimeout value is used to specify the timeout, in milliseconds, to be used when reading the resource.
* A timeout of zero is interpreted as an infinite timeout.
* Default value is: 60000
*
* @parameter
* @since 1.2
*/
private List templateParameterResources;
/**
* Global transformation parameters.
*
* <transformationParameters>
* <transformationParameter>
* <key>The name of the parameter.</key>
* <value>The value of the parameter.</value>
* <type>The name of the class of the parameter's object.</type>
* </transformationParameter>
* </transformationParameters>
*
*
* @parameter
* @since 1.2
*/
private List transformationParameters;
/**
* Global transformation output properties.
*
* <transformationOutputProperties>
* <transformationOutputProperty>
* <key>The name of the property.</key>
* <value>The value of the property.</value>
* <type>The name of the class of the properties object.</type>
* </transformationOutputProperty>
* </transformationOutputProperties>
*
*
* @parameter
* @since 1.2
*/
private List transformationOutputProperties;
/**
* Global transformation parameter resources.
*
* <transformationParameterResources>
* <transformationParameterResource>
* <location>The location of the properties resource.</location>
* <optional>Flag indicating the properties resource is optional.</optional>
* <format>The format of the properties resource.</format>
* <connectTimeout>Timeout value, in milliseconds.</connectTimeout>
* <readTimeout>Timeout value, in milliseconds.</readTimeout>
* </transformationParameterResource>
* </transformationParameterResources>
*
* The location value is used to first search the class path of the plugin and the project's main or test class
* path. If a class path resource is found, that resource is used. If no class path resource is found, an attempt is
* made to parse the location value to an URL. On successful parsing, that URL is used. Otherwise the location value
* is interpreted as a file name relative to the base directory of the project. If that file exists, that file is
* used. If nothing is found at the given location, depending on the optional flag, a warning message is logged or a
* build failure is produced.
* The optional flag is used to flag the resource optional. When an optional resource is not found, a warning
* message is logged instead of producing a build failure.
Default value is: false
* The format value is used to specify the format of the properties resource. Supported values are {@code plain}
* and {@code xml}.
Default value is: plain
* The connectTimeout value is used to specify the timeout, in milliseconds, to be used when opening
* communications links to the resource. A timeout of zero is interpreted as an infinite timeout.
* Default value is: 60000
* The readTimeout value is used to specify the timeout, in milliseconds, to be used when reading the resource.
* A timeout of zero is interpreted as an infinite timeout.
* Default value is: 60000
*
* @parameter
* @since 1.2
*/
private List transformationParameterResources;
/**
* Class name of the {@code ClassFileProcessor} backing the goal.
*
* @parameter default-value="org.jomc.tools.ClassFileProcessor" expression="${jomc.classFileProcessorClassName}"
* @since 1.2
*/
private String classFileProcessorClassName;
/**
* Class name of the {@code ResourceFileProcessor} backing the goal.
*
* @parameter default-value="org.jomc.tools.ResourceFileProcessor"
* expression="${jomc.resourceFileProcessorClassName}"
* @since 1.2
*/
private String resourceFileProcessorClassName;
/**
* Class name of the {@code SourceFileProcessor} backing the goal.
*
* @parameter default-value="org.jomc.tools.SourceFileProcessor" expression="${jomc.sourceFileProcessorClassName}"
* @since 1.2
*/
private String sourceFileProcessorClassName;
/**
* {@code ModelContext} attributes.
*
* <modelContextAttributes>
* <modelContextAttribute>
* <key>The name of the attribute.</key>
* <value>The value of the attribute.</value>
* <type>The name of the class of the attributes's object.</type>
* </modelContextAttribute>
* </modelContextAttributes>
*
*
* @parameter
* @since 1.2
*/
private List modelContextAttributes;
/**
* Flag controlling JAXP schema validation of model resources.
*
* @parameter default-value="true" expression="${jomc.modelResourceValidationEnabled}"
*
* @since 1.2
*/
private boolean modelResourceValidationEnabled;
/**
* Flag controlling JAXP schema validation of modlet resources.
*
* @parameter default-value="true" expression="${jomc.modletResourceValidationEnabled}"
*
* @since 1.2
*/
private boolean modletResourceValidationEnabled;
/**
* Flag controlling Java validation.
*
* @parameter default-value="true" expression="${jomc.javaValidationEnabled}"
*
* @since 1.4
*/
private boolean javaValidationEnabled;
/**
* Names of modlets to exclude.
*
* @parameter expression="${jomc.modletExcludes}"
*
* @since 1.6
*/
private List modletExcludes;
/**
* Names of modlets to include.
*
* @parameter expression="${jomc.modletIncludes}"
*
* @since 1.6
*/
private List modletIncludes;
/**
* The Maven project of the instance.
*
* @parameter expression="${project}"
* @required
* @readonly
*/
private MavenProject mavenProject;
/**
* List of plugin artifacts.
*
* @parameter expression="${plugin.artifacts}"
* @required
* @readonly
*/
private List pluginArtifacts;
/**
* The Maven session of the instance.
*
* @parameter expression="${session}"
* @required
* @readonly
* @since 1.1
*/
private MavenSession mavenSession;
/** Creates a new {@code AbstractJomcMojo} instance. */
public AbstractJomcMojo()
{
super();
}
/**
* {@inheritDoc}
* @see #assertValidParameters()
* @see #isExecutionPermitted()
* @see #executeTool()
*/
@SuppressWarnings( "deprecation" )
public void execute() throws MojoExecutionException, MojoFailureException
{
this.assertValidParameters();
try
{
this.logSeparator();
if ( this.isLoggable( Level.INFO ) )
{
this.log( Level.INFO, Messages.getMessage( "title" ), null );
}
if ( this.isExecutionPermitted() )
{
this.executeTool();
}
else if ( this.isLoggable( Level.INFO ) )
{
this.log( Level.INFO, Messages.getMessage( "executionSuppressed", this.getExecutionStrategy() ), null );
}
}
catch ( final Exception e )
{
throw new MojoExecutionException( Messages.getMessage( e ), e );
}
finally
{
JomcTool.setDefaultTemplateProfile( null );
this.logSeparator();
}
}
/**
* Validates the parameters of the goal.
*
* @throws MojoFailureException if illegal parameter values are detected.
*
* @see #assertValidResources(java.util.Collection)
* @since 1.2
*/
protected void assertValidParameters() throws MojoFailureException
{
this.assertValidResources( this.templateParameterResources );
this.assertValidResources( this.transformationParameterResources );
this.assertValidResources( this.velocityPropertyResources );
}
/**
* Validates a given resource collection.
*
* @param resources The resource collection to validate or {@code null}.
*
* @throws MojoFailureException if a location property of a given resource holds a {@code null} value or a given
* {@code PropertiesResourceType} holds an illegal format.
*
* @see #assertValidParameters()
* @see PropertiesResourceType#isFormatSupported(java.lang.String)
* @since 1.2
*/
protected final void assertValidResources( final Collection extends ResourceType> resources )
throws MojoFailureException
{
if ( resources != null )
{
for ( final ResourceType r : resources )
{
if ( r.getLocation() == null )
{
throw new MojoFailureException( Messages.getMessage( "mandatoryParameter", "location" ) );
}
if ( r instanceof PropertiesResourceType )
{
final PropertiesResourceType p = (PropertiesResourceType) r;
if ( !PropertiesResourceType.isFormatSupported( p.getFormat() ) )
{
throw new MojoFailureException( Messages.getMessage(
"illegalPropertiesFormat", p.getFormat(),
StringUtils.join( PropertiesResourceType.getSupportedFormats(), ',' ) ) );
}
}
}
}
}
/**
* Executes this tool.
*
* @throws Exception if execution of this tool fails.
*/
protected abstract void executeTool() throws Exception;
/**
* Gets the goal of the instance.
*
* @return The goal of the instance.
*
* @throws MojoExecutionException if getting the goal of the instance fails.
* @since 1.1
*/
protected abstract String getGoal() throws MojoExecutionException;
/**
* Gets the execution strategy of the instance.
*
* @return The execution strategy of the instance.
*
* @throws MojoExecutionException if getting the execution strategy of the instance fails.
* @since 1.1
*/
protected abstract String getExecutionStrategy() throws MojoExecutionException;
/**
* Gets a flag indicating the current execution is permitted.
*
* @return {@code true}, if the current execution is permitted; {@code false}, if the current execution is
* suppressed.
*
* @throws MojoExecutionException if getting the flag fails.
*
* @since 1.1
* @see #getGoal()
* @see #getExecutionStrategy()
*/
protected boolean isExecutionPermitted() throws MojoExecutionException
{
try
{
boolean permitted = true;
if ( MojoDescriptor.SINGLE_PASS_EXEC_STRATEGY.equals( this.getExecutionStrategy() ) )
{
final File flagFile =
new File( this.getSessionDirectory(),
ArtifactUtils.versionlessKey( this.getMavenProject().getArtifact() ).hashCode()
+ "-" + this.getGoal()
+ "-" + this.getMavenSession().getStartTime().getTime() + ".flg" );
if ( !this.getSessionDirectory().exists() && !this.getSessionDirectory().mkdirs() )
{
throw new MojoExecutionException( Messages.getMessage(
"failedCreatingDirectory", this.getSessionDirectory().getAbsolutePath() ) );
}
permitted = flagFile.createNewFile();
}
return permitted;
}
catch ( final IOException e )
{
throw new MojoExecutionException( Messages.getMessage( e ), e );
}
}
/**
* Gets the Maven project of the instance.
*
* @return The Maven project of the instance.
*
* @throws MojoExecutionException if getting the Maven project of the instance fails.
*/
protected MavenProject getMavenProject() throws MojoExecutionException
{
return this.mavenProject;
}
/**
* Gets the Maven session of the instance.
*
* @return The Maven session of the instance.
*
* @throws MojoExecutionException if getting the Maven session of the instance fails.
*
* @since 1.1
*/
protected MavenSession getMavenSession() throws MojoExecutionException
{
return this.mavenSession;
}
/**
* Gets an absolute {@code File} instance for a given name.
* This method constructs a new {@code File} instance using the given name. If the resulting file is not
* absolute, the value of the {@code basedir} property of the current Maven project is prepended.
*
* @param name The name to get an absolute {@code File} instance for.
*
* @return An absolute {@code File} instance constructed from {@code name}.
*
* @throws MojoExecutionException if getting an absolute {@code File} instance for {@code name} fails.
* @throws NullPointerException if {@code name} is {@code null}.
*
* @since 1.1
*/
protected File getAbsoluteFile( final String name ) throws MojoExecutionException
{
if ( name == null )
{
throw new NullPointerException( "name" );
}
File file = new File( name );
if ( !file.isAbsolute() )
{
file = new File( this.getMavenProject().getBasedir(), name );
}
return file;
}
/**
* Gets the directory holding the compiled class files of the project.
*
* @return The directory holding the compiled class files of the project.
*
* @throws MojoExecutionException if getting the directory fails.
*
* @since 1.1
*/
protected File getOutputDirectory() throws MojoExecutionException
{
if ( this.classesDirectory != null )
{
if ( this.isLoggable( Level.WARNING ) )
{
this.log( Level.WARNING, Messages.getMessage(
"deprecationWarning", "classesDirectory", "outputDirectory" ), null );
}
if ( !this.classesDirectory.equals( this.outputDirectory ) )
{
if ( this.isLoggable( Level.WARNING ) )
{
this.log( Level.WARNING, Messages.getMessage( "ignoringParameter", "outputDirectory" ), null );
}
this.outputDirectory = this.classesDirectory;
}
this.classesDirectory = null;
}
final File dir = this.getAbsoluteFile( this.outputDirectory );
if ( !dir.exists() && !dir.mkdirs() )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingDirectory", dir.getAbsolutePath() ) );
}
return dir;
}
/**
* Gets the directory holding the compiled test class files of the project.
*
* @return The directory holding the compiled test class files of the project.
*
* @throws MojoExecutionException if getting the directory fails.
*
* @since 1.1
*/
protected File getTestOutputDirectory() throws MojoExecutionException
{
if ( this.testClassesDirectory != null )
{
if ( this.isLoggable( Level.WARNING ) )
{
this.log( Level.WARNING, Messages.getMessage(
"deprecationWarning", "testClassesDirectory", "testOutputDirectory" ), null );
}
if ( !this.testClassesDirectory.equals( this.testOutputDirectory ) )
{
if ( this.isLoggable( Level.WARNING ) )
{
this.log( Level.WARNING, Messages.getMessage( "ignoringParameter", "testOutputDirectory" ), null );
}
this.testOutputDirectory = this.testClassesDirectory;
}
this.testClassesDirectory = null;
}
final File dir = this.getAbsoluteFile( this.testOutputDirectory );
if ( !dir.exists() && !dir.mkdirs() )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingDirectory", dir.getAbsolutePath() ) );
}
return dir;
}
/**
* Gets the directory holding the source files of the project.
*
* @return The directory holding the source files of the project.
*
* @throws MojoExecutionException if getting the directory fails.
*
* @since 1.1
*/
protected File getSourceDirectory() throws MojoExecutionException
{
return this.getAbsoluteFile( this.sourceDirectory );
}
/**
* Gets the directory holding the test source files of the project.
*
* @return The directory holding the test source files of the project.
*
* @throws MojoExecutionException if getting the directory fails.
*
* @since 1.1
*/
protected File getTestSourceDirectory() throws MojoExecutionException
{
return this.getAbsoluteFile( this.testSourceDirectory );
}
/**
* Gets the directory holding the session related files of the project.
*
* @return The directory holding the session related files of the project.
*
* @throws MojoExecutionException if getting the directory fails.
*
* @since 1.1
*/
protected File getSessionDirectory() throws MojoExecutionException
{
return this.getAbsoluteFile( this.sessionDirectory );
}
/**
* Gets the directory holding the reports of the project.
*
* @return The directory holding the reports of the project.
*
* @throws MojoExecutionException if getting the directory fails.
*
* @since 1.1
*/
protected File getReportOutputDirectory() throws MojoExecutionException
{
return this.getAbsoluteFile( this.reportOutputDirectory );
}
/**
* Gets the project's runtime class loader of the instance.
*
* @return The project's runtime class loader of the instance.
*
* @throws MojoExecutionException if getting the class loader fails.
*/
protected ClassLoader getMainClassLoader() throws MojoExecutionException
{
try
{
final Set mainClasspathElements = this.getMainClasspathElements();
final Set uris = new HashSet( mainClasspathElements.size() );
for ( final String element : mainClasspathElements )
{
final URI uri = new File( element ).toURI();
if ( !uris.contains( uri ) )
{
uris.add( uri );
}
}
if ( this.isLoggable( Level.FINEST ) )
{
this.log( Level.FINEST, Messages.getMessage( "mainClasspathInfo" ), null );
}
int i = 0;
final URL[] urls = new URL[ uris.size() ];
for ( final URI uri : uris )
{
urls[i++] = uri.toURL();
if ( this.isLoggable( Level.FINEST ) )
{
this.log( Level.FINEST, "\t" + urls[i - 1].toExternalForm(), null );
}
}
return new URLClassLoader( urls, Thread.currentThread().getContextClassLoader() );
}
catch ( final IOException e )
{
throw new MojoExecutionException( Messages.getMessage( e ), e );
}
}
/**
* Gets the project's test class loader of the instance.
*
* @return The project's test class loader of the instance.
*
* @throws MojoExecutionException if getting the class loader fails.
*/
protected ClassLoader getTestClassLoader() throws MojoExecutionException
{
try
{
final Set testClasspathElements = this.getTestClasspathElements();
final Set uris = new HashSet( testClasspathElements.size() );
for ( final String element : testClasspathElements )
{
final URI uri = new File( element ).toURI();
if ( !uris.contains( uri ) )
{
uris.add( uri );
}
}
if ( this.isLoggable( Level.FINEST ) )
{
this.log( Level.FINEST, Messages.getMessage( "testClasspathInfo" ), null );
}
int i = 0;
final URL[] urls = new URL[ uris.size() ];
for ( final URI uri : uris )
{
urls[i++] = uri.toURL();
if ( this.isLoggable( Level.FINEST ) )
{
this.log( Level.FINEST, "\t" + urls[i - 1].toExternalForm(), null );
}
}
return new URLClassLoader( urls, Thread.currentThread().getContextClassLoader() );
}
catch ( final IOException e )
{
throw new MojoExecutionException( Messages.getMessage( e ), e );
}
}
/**
* Gets the project's runtime class path elements.
*
* @return A set of class path element strings.
*
* @throws MojoExecutionException if getting the class path elements fails.
*/
protected Set getMainClasspathElements() throws MojoExecutionException
{
final List> runtimeArtifacts = this.getMavenProject().getRuntimeArtifacts();
final List> compileArtifacts = this.getMavenProject().getCompileArtifacts();
final Set elements = new HashSet( runtimeArtifacts.size() + compileArtifacts.size() + 1 );
elements.add( this.getOutputDirectory().getAbsolutePath() );
for ( final Iterator> it = runtimeArtifacts.iterator(); it.hasNext(); )
{
final Artifact a = (Artifact) it.next();
final Artifact pluginArtifact = this.getPluginArtifact( a );
if ( a.getFile() == null )
{
if ( this.isLoggable( Level.WARNING ) )
{
this.log( Level.WARNING, Messages.getMessage( "ignoringArtifact", a.toString() ), null );
}
continue;
}
if ( pluginArtifact != null )
{
if ( this.isLoggable( Level.FINER ) )
{
this.log( Level.FINER, Messages.getMessage(
"ignoringPluginArtifact", a.toString(), pluginArtifact.toString() ), null );
}
continue;
}
final String element = a.getFile().getAbsolutePath();
elements.add( element );
}
for ( final Iterator> it = compileArtifacts.iterator(); it.hasNext(); )
{
final Artifact a = (Artifact) it.next();
final Artifact pluginArtifact = this.getPluginArtifact( a );
if ( a.getFile() == null )
{
if ( this.isLoggable( Level.WARNING ) )
{
this.log( Level.WARNING, Messages.getMessage( "ignoringArtifact", a.toString() ), null );
}
continue;
}
if ( pluginArtifact != null )
{
if ( this.isLoggable( Level.FINER ) )
{
this.log( Level.FINER, Messages.getMessage(
"ignoringPluginArtifact", a.toString(), pluginArtifact.toString() ), null );
}
continue;
}
final String element = a.getFile().getAbsolutePath();
elements.add( element );
}
return elements;
}
/**
* Gets the project's test class path elements.
*
* @return A set of class path element strings.
*
* @throws MojoExecutionException if getting the class path elements fails.
*/
protected Set getTestClasspathElements() throws MojoExecutionException
{
final List> testArtifacts = this.getMavenProject().getTestArtifacts();
final Set elements = new HashSet( testArtifacts.size() + 2 );
elements.add( this.getOutputDirectory().getAbsolutePath() );
elements.add( this.getTestOutputDirectory().getAbsolutePath() );
for ( final Iterator> it = testArtifacts.iterator(); it.hasNext(); )
{
final Artifact a = (Artifact) it.next();
final Artifact pluginArtifact = this.getPluginArtifact( a );
if ( a.getFile() == null )
{
if ( this.isLoggable( Level.WARNING ) )
{
this.log( Level.WARNING, Messages.getMessage( "ignoringArtifact", a.toString() ), null );
}
continue;
}
if ( pluginArtifact != null )
{
if ( this.isLoggable( Level.FINER ) )
{
this.log( Level.FINER, Messages.getMessage(
"ignoringPluginArtifact", a.toString(), pluginArtifact.toString() ), null );
}
continue;
}
final String element = a.getFile().getAbsolutePath();
elements.add( element );
}
return elements;
}
/**
* Gets a flag indicating verbose output is enabled.
*
* @return {@code true}, if verbose output is enabled; {@code false}, if information messages are suppressed.
*
* @throws MojoExecutionException if getting the flag fails.
*
* @since 1.1
*/
protected final boolean isVerbose() throws MojoExecutionException
{
return this.verbose;
}
/**
* Sets the flag indicating verbose output is enabled.
*
* @param value {@code true}, to enable verbose output; {@code false}, to suppress information messages.
*
* @throws MojoExecutionException if setting the flag fails.
*
* @since 1.1
*/
protected final void setVerbose( final boolean value ) throws MojoExecutionException
{
this.verbose = value;
}
/**
* Gets a flag indicating the processing of sources is enabled.
*
* @return {@code true}, if processing of sources is enabled; {@code false}, else.
*
* @throws MojoExecutionException if getting the flag fails.
*/
protected final boolean isSourceProcessingEnabled() throws MojoExecutionException
{
return this.sourceProcessingEnabled;
}
/**
* Sets the flag indicating the processing of sources is enabled.
*
* @param value {@code true}, to enable processing of sources; {@code false}, to disable processing of sources.
*
* @throws MojoExecutionException if setting the flag fails.
*
* @since 1.1
*/
protected final void setSourceProcessingEnabled( final boolean value ) throws MojoExecutionException
{
this.sourceProcessingEnabled = value;
}
/**
* Gets a flag indicating the processing of resources is enabled.
*
* @return {@code true}, if processing of resources is enabled; {@code false}, else.
*
* @throws MojoExecutionException if getting the flag fails.
*/
protected final boolean isResourceProcessingEnabled() throws MojoExecutionException
{
return this.resourceProcessingEnabled;
}
/**
* Sets the flag indicating the processing of resources is enabled.
*
* @param value {@code true}, to enable processing of resources; {@code false}, to disable processing of resources.
*
* @throws MojoExecutionException if setting the flag fails.
*
* @since 1.1
*/
protected final void setResourceProcessingEnabled( final boolean value ) throws MojoExecutionException
{
this.resourceProcessingEnabled = value;
}
/**
* Gets a flag indicating the processing of classes is enabled.
*
* @return {@code true}, if processing of classes is enabled; {@code false}, else.
*
* @throws MojoExecutionException if getting the flag fails.
*/
protected final boolean isClassProcessingEnabled() throws MojoExecutionException
{
return this.classProcessingEnabled;
}
/**
* Sets the flag indicating the processing of classes is enabled.
*
* @param value {@code true}, to enable processing of classes; {@code false}, to disable processing of classes.
*
* @throws MojoExecutionException if setting the flag fails.
*
* @since 1.1
*/
protected final void setClassProcessingEnabled( final boolean value ) throws MojoExecutionException
{
this.classProcessingEnabled = value;
}
/**
* Gets a flag indicating the processing of models is enabled.
*
* @return {@code true}, if processing of models is enabled; {@code false}, else.
*
* @throws MojoExecutionException if getting the flag fails.
*/
protected final boolean isModelProcessingEnabled() throws MojoExecutionException
{
return this.modelProcessingEnabled;
}
/**
* Sets the flag indicating the processing of models is enabled.
*
* @param value {@code true}, to enable processing of models; {@code false}, to disable processing of models.
*
* @throws MojoExecutionException if setting the flag fails.
*
* @since 1.1
*/
protected final void setModelProcessingEnabled( final boolean value ) throws MojoExecutionException
{
this.modelProcessingEnabled = value;
}
/**
* Gets a flag indicating model object class path resolution is enabled.
*
* @return {@code true}, if model object class path resolution is enabled; {@code false}, else.
*
* @throws MojoExecutionException if getting the flag fails.
*/
protected final boolean isModelObjectClasspathResolutionEnabled() throws MojoExecutionException
{
return this.modelObjectClasspathResolutionEnabled;
}
/**
* Sets the flag indicating model object class path resolution is enabled.
*
* @param value {@code true}, to enable model object class path resolution; {@code false}, to disable model object
* class path resolution.
*
* @throws MojoExecutionException if setting the flag fails.
*
* @since 1.1
*/
protected final void setModelObjectClasspathResolutionEnabled( final boolean value ) throws MojoExecutionException
{
this.modelObjectClasspathResolutionEnabled = value;
}
/**
* Gets the identifier of the model to process.
*
* @return The identifier of the model to process.
*
* @throws MojoExecutionException if getting the identifier fails.
*/
protected String getModel() throws MojoExecutionException
{
return this.model;
}
/**
* Gets the name of the module to process.
*
* @return The name of the module to process.
*
* @throws MojoExecutionException if getting the name of the module fails.
*/
protected String getModuleName() throws MojoExecutionException
{
return this.moduleName;
}
/**
* Gets the name of the test module to process.
*
* @return The name of the test module to process.
*
* @throws MojoExecutionException if getting the name of the test module fails.
*/
protected String getTestModuleName() throws MojoExecutionException
{
return this.testModuleName;
}
/**
* Gets the model to process.
*
* @param context The model context to get the model to process with.
*
* @return The model to process.
*
* @throws NullPointerException if {@code context} is {@code null}.
* @throws MojoExecutionException if getting the model fails.
*/
protected Model getModel( final ModelContext context ) throws MojoExecutionException
{
if ( context == null )
{
throw new NullPointerException( "context" );
}
try
{
Model m = context.findModel( this.getModel() );
final Modules modules = ModelHelper.getModules( m );
if ( modules != null && this.isModelObjectClasspathResolutionEnabled() )
{
final Module classpathModule =
modules.getClasspathModule( Modules.getDefaultClasspathModuleName(), context.getClassLoader() );
if ( classpathModule != null )
{
modules.getModule().add( classpathModule );
}
}
if ( this.isModelProcessingEnabled() )
{
m = context.processModel( m );
}
return m;
}
catch ( final ModelException e )
{
throw new MojoExecutionException( Messages.getMessage( e ), e );
}
}
/**
* Creates a new model context instance for a given class loader.
*
* @param classLoader The class loader to use for creating the context.
*
* @return A new model context instance for {@code classLoader}.
*
* @throws MojoExecutionException if creating the model context fails.
*
* @see #setupModelContext(org.jomc.modlet.ModelContext)
*/
protected ModelContext createModelContext( final ClassLoader classLoader ) throws MojoExecutionException
{
final ModelContextFactory modelContextFactory;
if ( this.modelContextFactoryClassName != null )
{
modelContextFactory = ModelContextFactory.newInstance( this.modelContextFactoryClassName );
}
else
{
modelContextFactory = ModelContextFactory.newInstance();
}
final ModelContext context = modelContextFactory.newModelContext( classLoader );
this.setupModelContext( context );
return context;
}
/**
* Creates a new tool instance for processing source files.
*
* @param context The context of the tool.
*
* @return A new tool instance for processing source files.
*
* @throws NullPointerException if {@code context} is {@code null}.
* @throws MojoExecutionException if creating a new tool instance fails.
*
* @see #createJomcTool(org.jomc.modlet.ModelContext, java.lang.String, java.lang.Class)
*/
protected SourceFileProcessor createSourceFileProcessor( final ModelContext context ) throws MojoExecutionException
{
if ( context == null )
{
throw new NullPointerException( "context" );
}
return this.createJomcTool( context, this.sourceFileProcessorClassName, SourceFileProcessor.class );
}
/**
* Creates a new tool instance for processing resource files.
*
* @param context The context of the tool.
*
* @return A new tool instance for processing resource files.
*
* @throws NullPointerException if {@code context} is {@code null}.
* @throws MojoExecutionException if creating a new tool instance fails.
*
* @see #createJomcTool(org.jomc.modlet.ModelContext, java.lang.String, java.lang.Class)
*/
protected ResourceFileProcessor createResourceFileProcessor( final ModelContext context )
throws MojoExecutionException
{
if ( context == null )
{
throw new NullPointerException( "context" );
}
return this.createJomcTool( context, this.resourceFileProcessorClassName, ResourceFileProcessor.class );
}
/**
* Creates a new tool instance for processing class files.
*
* @param context The context of the tool.
*
* @return A new tool instance for processing class files.
*
* @throws NullPointerException if {@code context} is {@code null}.
* @throws MojoExecutionException if creating a new tool instance fails.
*
* @see #createJomcTool(org.jomc.modlet.ModelContext, java.lang.String, java.lang.Class)
*/
protected ClassFileProcessor createClassFileProcessor( final ModelContext context ) throws MojoExecutionException
{
if ( context == null )
{
throw new NullPointerException( "context" );
}
return this.createJomcTool( context, this.classFileProcessorClassName, ClassFileProcessor.class );
}
/**
* Creates a new {@code JomcTool} object for a given class name and type.
*
* @param context The context of the tool.
* @param className The name of the class to create an object of.
* @param type The class of the type of object to create.
* @param The type of the object to create.
*
* @return A new instance of the class with name {@code className}.
*
* @throws NullPointerException if {@code context}, {@code className} or {@code type} is {@code null}.
* @throws MojoExecutionException if creating a new {@code JomcTool} object fails.
*
* @see #createObject(org.jomc.modlet.ModelContext, java.lang.String, java.lang.Class)
* @see #setupJomcTool(org.jomc.modlet.ModelContext, org.jomc.tools.JomcTool)
*
* @since 1.2
*/
protected T createJomcTool( final ModelContext context, final String className,
final Class type ) throws MojoExecutionException
{
if ( context == null )
{
throw new NullPointerException( "context" );
}
if ( className == null )
{
throw new NullPointerException( "className" );
}
if ( type == null )
{
throw new NullPointerException( "type" );
}
final T tool = this.createObject( context, className, type );
this.setupJomcTool( context, tool );
return tool;
}
/**
* Creates a new object for a given class name and type.
*
* @param className The name of the class to create an object of.
* @param type The class of the type of object to create.
* @param The type of the object to create.
*
* @return A new instance of the class with name {@code className}.
*
* @throws NullPointerException if {@code className} or {@code type} is {@code null}.
* @throws MojoExecutionException if creating a new object fails.
*
* @since 1.2
* @deprecated As of JOMC 1.8, replaced by method {@link #createObject(org.jomc.modlet.ModelContext, java.lang.String, java.lang.Class)}.
* This method will be removed in JOMC 2.0.
*/
@Deprecated
@SuppressWarnings( "deprecation" )
protected T createObject( final String className, final Class type ) throws MojoExecutionException
{
if ( className == null )
{
throw new NullPointerException( "className" );
}
if ( type == null )
{
throw new NullPointerException( "type" );
}
try
{
return Class.forName( className ).asSubclass( type ).newInstance();
}
catch ( final InstantiationException e )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingObject", className ), e );
}
catch ( final IllegalAccessException e )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingObject", className ), e );
}
catch ( final ClassNotFoundException e )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingObject", className ), e );
}
catch ( final ClassCastException e )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingObject", className ), e );
}
}
/**
* Creates a new object for a given class name and type.
*
* @param modelContext The model context to search.
* @param className The name of the class to create an object of.
* @param type The class of the type of object to create.
* @param The type of the object to create.
*
* @return A new instance of the class with name {@code className}.
*
* @throws NullPointerException if {@code modelContext}, {@code className} or {@code type} is {@code null}.
* @throws MojoExecutionException if creating a new object fails.
*
* @since 1.8
*/
protected T createObject( final ModelContext modelContext, final String className, final Class type )
throws MojoExecutionException
{
if ( modelContext == null )
{
throw new NullPointerException( "modelContext" );
}
if ( className == null )
{
throw new NullPointerException( "className" );
}
if ( type == null )
{
throw new NullPointerException( "type" );
}
try
{
final Class> javaClass = modelContext.findClass( className );
if ( javaClass == null )
{
throw new MojoExecutionException( Messages.getMessage( "classNotFound", className ) );
}
return javaClass.asSubclass( type ).newInstance();
}
catch ( final ModelException e )
{
String m = Messages.getMessage( e );
m = m == null ? "" : " " + m;
throw new MojoExecutionException( Messages.getMessage( "failedSearchingClass", className, m ), e );
}
catch ( final InstantiationException e )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingObject", className ), e );
}
catch ( final IllegalAccessException e )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingObject", className ), e );
}
catch ( final ClassCastException e )
{
throw new MojoExecutionException( Messages.getMessage( "failedCreatingObject", className ), e );
}
}
/**
* Creates an {@code URL} for a given resource location.
* This method first searches the class path of the plugin for a single resource matching {@code location}. If
* such a resource is found, the URL of that resource is returned. If no such resource is found, an attempt is made
* to parse the given location to an URL. On successful parsing, that URL is returned. Failing that, the given
* location is interpreted as a file name relative to the project's base directory. If that file is found, the URL
* of that file is returned. Otherwise {@code null} is returned.
*
* @param location The location to create an {@code URL} from.
*
* @return An {@code URL} for {@code location} or {@code null}, if parsing {@code location} to an URL fails and
* {@code location} points to a non-existent resource.
*
* @throws NullPointerException if {@code location} is {@code null}.
* @throws MojoExecutionException if creating an URL fails.
*
* @since 1.2
* @deprecated As of JOMC 1.8, replaced by method {@link #getResource(org.jomc.modlet.ModelContext, java.lang.String)}.
* This method will be removed in JOMC 2.0.
*/
@Deprecated
@SuppressWarnings( "deprecation" )
protected URL getResource( final String location ) throws MojoExecutionException
{
if ( location == null )
{
throw new NullPointerException( "location" );
}
try
{
String absolute = location;
if ( !absolute.startsWith( "/" ) )
{
absolute = "/" + location;
}
URL resource = this.getClass().getResource( absolute );
if ( resource == null )
{
try
{
resource = new URL( location );
}
catch ( final MalformedURLException e )
{
if ( this.isLoggable( Level.FINEST ) )
{
this.log( Level.FINEST, Messages.getMessage( e ), e );
}
resource = null;
}
}
if ( resource == null )
{
final File f = this.getAbsoluteFile( location );
if ( f.isFile() )
{
resource = f.toURI().toURL();
}
}
return resource;
}
catch ( final MalformedURLException e )
{
String m = Messages.getMessage( e );
m = m == null ? "" : " " + m;
throw new MojoExecutionException( Messages.getMessage( "malformedLocation", location, m ), e );
}
}
/**
* Creates an {@code URL} for a given resource location.
* This method first searches the given model context for a single resource matching {@code location}. If such a
* resource is found, the URL of that resource is returned. If no such resource is found, an attempt is made to
* parse the given location to an URL. On successful parsing, that URL is returned. Failing that, the given location
* is interpreted as a file name relative to the project's base directory. If that file is found, the URL of that
* file is returned. Otherwise {@code null} is returned.
*
* @param modelContext The model conext to search.
* @param location The location to create an {@code URL} from.
*
* @return An {@code URL} for {@code location} or {@code null}, if parsing {@code location} to an URL fails and
* {@code location} points to a non-existent resource.
*
* @throws NullPointerException if {@code modelContext} or {@code location} is {@code null}.
* @throws MojoExecutionException if creating an URL fails.
*
* @since 1.8
*/
protected URL getResource( final ModelContext modelContext, final String location ) throws MojoExecutionException
{
if ( modelContext == null )
{
throw new NullPointerException( "modelContext" );
}
if ( location == null )
{
throw new NullPointerException( "location" );
}
try
{
String absolute = location;
if ( !absolute.startsWith( "/" ) )
{
absolute = "/" + location;
}
URL resource = modelContext.findResource( absolute );
if ( resource == null )
{
try
{
resource = new URL( location );
}
catch ( final MalformedURLException e )
{
if ( this.isLoggable( Level.FINEST ) )
{
this.log( Level.FINEST, Messages.getMessage( e ), e );
}
resource = null;
}
}
if ( resource == null )
{
final File f = this.getAbsoluteFile( location );
if ( f.isFile() )
{
resource = f.toURI().toURL();
}
}
return resource;
}
catch ( final ModelException e )
{
String m = Messages.getMessage( e );
m = m == null ? "" : " " + m;
throw new MojoExecutionException( Messages.getMessage( "failedSearchingResource", location, m ), e );
}
catch ( final MalformedURLException e )
{
String m = Messages.getMessage( e );
m = m == null ? "" : " " + m;
throw new MojoExecutionException( Messages.getMessage( "malformedLocation", location, m ), e );
}
}
/**
* Creates an {@code URL} for a given directory location.
* This method first attempts to parse the given location to an URL. On successful parsing, that URL is returned.
* Failing that, the given location is interpreted as a directory name relative to the project's base directory.
* If that directory is found, the URL of that directory is returned. Otherwise {@code null} is returned.
*
* @param location The directory location to create an {@code URL} from.
*
* @return An {@code URL} for {@code location} or {@code null}, if parsing {@code location} to an URL fails and
* {@code location} points to a non-existent directory.
*
* @throws NullPointerException if {@code location} is {@code null}.
* @throws MojoExecutionException if creating an URL fails.
*
* @since 1.2
*/
protected URL getDirectory( final String location ) throws MojoExecutionException
{
if ( location == null )
{
throw new NullPointerException( "location" );
}
try
{
URL resource = null;
try
{
resource = new URL( location );
}
catch ( final MalformedURLException e )
{
if ( this.isLoggable( Level.FINEST ) )
{
this.log( Level.FINEST, Messages.getMessage( e ), e );
}
resource = null;
}
if ( resource == null )
{
final File f = this.getAbsoluteFile( location );
if ( f.isDirectory() )
{
resource = f.toURI().toURL();
}
}
return resource;
}
catch ( final MalformedURLException e )
{
String m = Messages.getMessage( e );
m = m == null ? "" : " " + m;
throw new MojoExecutionException( Messages.getMessage( "malformedLocation", location, m ), e );
}
}
/**
* Creates a new {@code Transformer} from a given {@code TransformerResourceType}.
*
* @param resource The resource to initialize the transformer with.
*
* @return A {@code Transformer} for {@code resource} or {@code null}, if {@code resource} is not found and flagged
* optional.
*
* @throws NullPointerException if {@code resource} is {@code null}.
* @throws MojoExecutionException if creating a transformer fails.
*
* @see #getResource(java.lang.String)
* @since 1.2
* @deprecated As of JOMC 1.8, replaced by method {@link #getTransformer(org.jomc.modlet.ModelContext, org.jomc.mojo.TransformerResourceType)}.
* This method will be removed in JOMC 2.0.
*/
@Deprecated
@SuppressWarnings( "deprecation" )
protected Transformer getTransformer( final TransformerResourceType resource ) throws MojoExecutionException
{
if ( resource == null )
{
throw new NullPointerException( "resource" );
}
InputStream in = null;
boolean suppressExceptionOnClose = true;
final URL url = this.getResource( resource.getLocation() );
final ErrorListener errorListener = new ErrorListener()
{
public void warning( final TransformerException exception ) throws TransformerException
{
try
{
log( Level.WARNING, Messages.getMessage( exception ), exception );
}
catch ( final MojoExecutionException e )
{
getLog().warn( exception );
getLog().error( e );
}
}
public void error( final TransformerException exception ) throws TransformerException
{
try
{
log( Level.SEVERE, Messages.getMessage( exception ), exception );
}
catch ( final MojoExecutionException e )
{
getLog().error( exception );
getLog().error( e );
}
throw exception;
}
public void fatalError( final TransformerException exception ) throws TransformerException
{
try
{
log( Level.SEVERE, Messages.getMessage( exception ), exception );
}
catch ( final MojoExecutionException e )
{
getLog().error( exception );
getLog().error( e );
}
throw exception;
}
};
try
{
if ( url != null )
{
if ( this.isLoggable( Level.FINER ) )
{
this.log( Level.FINER, Messages.getMessage( "loadingTransformer", url.toExternalForm() ), null );
}
final URLConnection con = url.openConnection();
con.setConnectTimeout( resource.getConnectTimeout() );
con.setReadTimeout( resource.getReadTimeout() );
con.connect();
in = con.getInputStream();
final TransformerFactory transformerFactory = TransformerFactory.newInstance();
transformerFactory.setErrorListener( errorListener );
final Transformer transformer =
transformerFactory.newTransformer( new StreamSource( in, url.toURI().toASCIIString() ) );
transformer.setErrorListener( errorListener );
for ( final Map.Entry