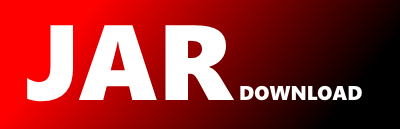
org.jooq.lambda.Seq Maven / Gradle / Ivy
Show all versions of jool Show documentation
/**
* Copyright (c) 2014-2016, Data Geekery GmbH, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jooq.lambda;
import static java.util.Comparator.comparing;
import static java.util.Spliterator.ORDERED;
import static java.util.Spliterators.spliteratorUnknownSize;
import static org.jooq.lambda.SeqUtils.sneakyThrow;
import static org.jooq.lambda.tuple.Tuple.tuple;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.io.Reader;
import java.io.UncheckedIOException;
import java.time.Duration;
import java.time.Instant;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Queue;
import java.util.Random;
import java.util.Set;
import java.util.Spliterator;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.function.ToDoubleFunction;
import java.util.function.ToIntFunction;
import java.util.function.ToLongFunction;
import java.util.function.UnaryOperator;
import java.util.stream.Collector;
import java.util.stream.Collectors;
import java.util.stream.DoubleStream;
import java.util.stream.IntStream;
import java.util.stream.LongStream;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import java.util.NoSuchElementException;
import javax.annotation.Generated;
import org.jooq.lambda.exception.TooManyElementsException;
import org.jooq.lambda.function.Function10;
import org.jooq.lambda.function.Function11;
import org.jooq.lambda.function.Function12;
import org.jooq.lambda.function.Function13;
import org.jooq.lambda.function.Function14;
import org.jooq.lambda.function.Function15;
import org.jooq.lambda.function.Function16;
import org.jooq.lambda.function.Function3;
import org.jooq.lambda.function.Function4;
import org.jooq.lambda.function.Function5;
import org.jooq.lambda.function.Function6;
import org.jooq.lambda.function.Function7;
import org.jooq.lambda.function.Function8;
import org.jooq.lambda.function.Function9;
import org.jooq.lambda.tuple.Tuple;
import org.jooq.lambda.tuple.Tuple1;
import org.jooq.lambda.tuple.Tuple10;
import org.jooq.lambda.tuple.Tuple11;
import org.jooq.lambda.tuple.Tuple12;
import org.jooq.lambda.tuple.Tuple13;
import org.jooq.lambda.tuple.Tuple14;
import org.jooq.lambda.tuple.Tuple15;
import org.jooq.lambda.tuple.Tuple16;
import org.jooq.lambda.tuple.Tuple2;
import org.jooq.lambda.tuple.Tuple3;
import org.jooq.lambda.tuple.Tuple4;
import org.jooq.lambda.tuple.Tuple5;
import org.jooq.lambda.tuple.Tuple6;
import org.jooq.lambda.tuple.Tuple7;
import org.jooq.lambda.tuple.Tuple8;
import org.jooq.lambda.tuple.Tuple9;
/**
* A sequential, ordered {@link Stream} that adds all sorts of useful methods that work only because
* it is sequential and ordered.
*
* @author Lukas Eder
* @author Roman Tkalenko
*/
public interface Seq extends Stream, Iterable, Collectable {
/**
* The underlying {@link Stream} implementation.
*/
Stream stream();
/**
* Transform this stream into a new type.
*
* If certain operations are re-applied frequently to streams, this
* transform operation is very useful for such operations to be applied in a
* fluent style:
*
*
* Function<Seq<Integer>, Seq<String>> toString = s -> s.map(Objects::toString);
* Seq<String> strings =
* Seq.of(1, 2, 3)
* .transform(toString);
*
*/
default U transform(Function super Seq, ? extends U> transformer) {
return transformer.apply(this);
}
/**
* Cross join 2 streams into one.
*
*
* // (tuple(1, "a"), tuple(1, "b"), tuple(2, "a"), tuple(2, "b"))
* Seq.of(1, 2).crossJoin(Seq.of("a", "b"))
*
*/
default Seq> crossJoin(Stream extends U> other) {
return Seq.crossJoin(this, other);
}
/**
* Cross join 2 streams into one.
*
*
* // (tuple(1, "a"), tuple(1, "b"), tuple(2, "a"), tuple(2, "b"))
* Seq.of(1, 2).crossJoin(Seq.of("a", "b"))
*
*/
default Seq> crossJoin(Iterable extends U> other) {
return Seq.crossJoin(this, other);
}
/**
* Cross join 2 streams into one.
*
*
* // (tuple(1, "a"), tuple(1, "b"), tuple(2, "a"), tuple(2, "b"))
* Seq.of(1, 2).crossJoin(Seq.of("a", "b"))
*
*/
default Seq> crossJoin(Seq extends U> other) {
return Seq.crossJoin(this, other);
}
/**
* Inner join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2))
* Seq.of(1, 2, 3).innerJoin(Seq.of(1, 2), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> innerJoin(Stream extends U> other, BiPredicate super T, ? super U> predicate) {
return innerJoin(seq(other), predicate);
}
/**
* Inner join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2))
* Seq.of(1, 2, 3).innerJoin(Seq.of(1, 2), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> innerJoin(Iterable extends U> other, BiPredicate super T, ? super U> predicate) {
return innerJoin(seq(other), predicate);
}
/**
* Inner join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2))
* Seq.of(1, 2, 3).innerJoin(Seq.of(1, 2), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> innerJoin(Seq extends U> other, BiPredicate super T, ? super U> predicate) {
// This algorithm isn't lazy and has substantial complexity for large argument streams!
List extends U> list = other.toList();
return flatMap(t -> seq(list)
.filter(u -> predicate.test(t, u))
.map(u -> tuple(t, u)))
.onClose(other::close);
}
/**
* Left outer join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2), tuple(3, null))
* Seq.of(1, 2, 3).leftOuterJoin(Seq.of(1, 2), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> leftOuterJoin(Stream extends U> other, BiPredicate super T, ? super U> predicate) {
return leftOuterJoin(seq(other), predicate);
}
/**
* Left outer join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2), tuple(3, null))
* Seq.of(1, 2, 3).leftOuterJoin(Seq.of(1, 2), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> leftOuterJoin(Iterable extends U> other, BiPredicate super T, ? super U> predicate) {
return leftOuterJoin(seq(other), predicate);
}
/**
* Left outer join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2), tuple(3, null))
* Seq.of(1, 2, 3).leftOuterJoin(Seq.of(1, 2), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> leftOuterJoin(Seq extends U> other, BiPredicate super T, ? super U> predicate) {
// This algorithm isn't lazy and has substantial complexity for large argument streams!
List extends U> list = other.toList();
return flatMap(t -> seq(list)
.filter(u -> predicate.test(t, u))
.onEmpty(null)
.map(u -> tuple(t, u)))
.onClose(other::close);
}
/**
* Right outer join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2), tuple(null, 3))
* Seq.of(1, 2).rightOuterJoin(Seq.of(1, 2, 3), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> rightOuterJoin(Stream extends U> other, BiPredicate super T, ? super U> predicate) {
return rightOuterJoin(seq(other), predicate);
}
/**
* Right outer join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2), tuple(null, 3))
* Seq.of(1, 2).rightOuterJoin(Seq.of(1, 2, 3), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> rightOuterJoin(Iterable extends U> other, BiPredicate super T, ? super U> predicate) {
return rightOuterJoin(seq(other), predicate);
}
/**
* Right outer join 2 streams into one.
*
*
* // (tuple(1, 1), tuple(2, 2), tuple(null, 3))
* Seq.of(1, 2).rightOuterJoin(Seq.of(1, 2, 3), t -> Objects.equals(t.v1, t.v2))
*
*/
default Seq> rightOuterJoin(Seq extends U> other, BiPredicate super T, ? super U> predicate) {
return other
.leftOuterJoin(this, (u, t) -> predicate.test(t, u))
.map(t -> tuple(t.v2, t.v1))
.onClose(other::close);
}
/**
* Produce this stream, or an alternative stream from the
* value
, in case this stream is empty.
*/
default Seq onEmpty(T value) {
return onEmptyGet(() -> value);
}
/**
* Produce this stream, or an alternative stream from the
* supplier
, in case this stream is empty.
*/
default Seq onEmptyGet(Supplier extends T> supplier) {
boolean[] first = { true };
return SeqUtils.transform(this, (delegate, action) -> {
if (first[0]) {
first[0] = false;
if (!delegate.tryAdvance(action))
action.accept(supplier.get());
return true;
} else {
return delegate.tryAdvance(action);
}
});
}
/**
* Produce this stream, or an alternative stream from the
* supplier
, in case this stream is empty.
*/
default Seq onEmptyThrow(Supplier extends X> supplier) {
boolean[] first = { true };
return SeqUtils.transform(this, (delegate, action) -> {
if (first[0]) {
first[0] = false;
if (!delegate.tryAdvance(action))
sneakyThrow(supplier.get());
return true;
} else {
return delegate.tryAdvance(action);
}
});
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).concat(Seq.of(4, 5, 6))
*
*
* @see #concat(Stream[])
*/
default Seq concat(Stream extends T> other) {
return concat(seq(other));
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).concat(Seq.of(4, 5, 6))
*
*
* @see #concat(Stream[])
*/
default Seq concat(Iterable extends T> other) {
return concat(seq(other));
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).concat(Seq.of(4, 5, 6))
*
*
* @see #concat(Stream[])
*/
@SuppressWarnings({ "unchecked" })
default Seq concat(Seq extends T> other) {
return Seq.concat(new Seq[]{this, other});
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4)
* Seq.of(1, 2, 3).concat(4)
*
*
* @see #concat(Stream[])
*/
default Seq concat(T other) {
return concat(Seq.of(other));
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).concat(4, 5, 6)
*
*
* @see #concat(Stream[])
*/
@SuppressWarnings({ "unchecked" })
default Seq concat(T... other) {
return concat(Seq.of(other));
}
/**
* Concatenate an optional value.
*
*
* // (1, 2, 3, 4)
* Seq.of(1, 2, 3).concat(Optional.of(4))
*
* // (1, 2, 3)
* Seq.of(1, 2, 3).concat(Optional.empty())
*
*/
default Seq concat(Optional extends T> other) {
return concat(Seq.seq(other));
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).append(Seq.of(4, 5, 6))
*
*
* @see #concat(Stream[])
*/
default Seq append(Stream extends T> other) {
return concat(other);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).append(Seq.of(4, 5, 6))
*
*
* @see #concat(Stream[])
*/
default Seq append(Iterable extends T> other) {
return concat(other);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).append(Seq.of(4, 5, 6))
*
*
* @see #concat(Stream[])
*/
@SuppressWarnings({ "unchecked" })
default Seq append(Seq extends T> other) {
return concat(other);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4)
* Seq.of(1, 2, 3).append(4)
*
*
* @see #concat(Stream[])
*/
default Seq append(T other) {
return concat(other);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(1, 2, 3).append(4, 5, 6)
*
*
* @see #concat(Stream[])
*/
@SuppressWarnings({ "unchecked" })
default Seq append(T... other) {
return concat(other);
}
/**
* Concatenate an optional value.
*
*
* // (1, 2, 3, 4)
* Seq.of(1, 2, 3).append(Optional.of(4))
*
* // (1, 2, 3)
* Seq.of(1, 2, 3).append(Optional.empty())
*
*/
default Seq append(Optional extends T> other) {
return concat(other);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(4, 5, 6).prepend(Seq.of(1, 2, 3))
*
*
* @see #concat(Stream[])
*/
default Seq prepend(Stream extends T> other) {
return seq(other).concat(this);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(4, 5, 6).prepend(Seq.of(1, 2, 3))
*
*
* @see #concat(Stream[])
*/
default Seq prepend(Iterable extends T> other) {
return seq(other).concat(this);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(4, 5, 6).prepend(Seq.of(1, 2, 3))
*
*
* @see #concat(Stream[])
*/
@SuppressWarnings({ "unchecked" })
default Seq prepend(Seq extends T> other) {
return concat(other, this);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4)
* Seq.of(2, 3, 4).prepend(1)
*
*
* @see #concat(Stream[])
*/
default Seq prepend(T other) {
return Seq.of(other).concat(this);
}
/**
* Concatenate two streams.
*
*
* // (1, 2, 3, 4, 5, 6)
* Seq.of(4, 5, 6).prepend(Seq.of(1, 2, 3))
*
*
* @see #concat(Stream[])
*/
@SuppressWarnings({ "unchecked" })
default Seq prepend(T... other) {
return Seq.of(other).concat(this);
}
/**
* Concatenate an optional value.
*
*
* // (0, 1, 2, 3)
* Seq.of(1, 2, 3).prepend(Optional.of(0))
*
* // (1, 2, 3)
* Seq.of(1, 2, 3).prepend(Optional.empty())
*
*/
default Seq prepend(Optional extends T> other) {
return Seq.seq(other).concat(this);
}
/**
* Check whether this stream contains a given value.
*
*
* // true
* Seq.of(1, 2, 3).contains(2)
*
*/
default boolean contains(T other) {
return anyMatch(Predicate.isEqual(other));
}
/**
* Check whether this stream contains all given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAll(2, 3)
*
*/
default boolean containsAll(T... other) {
return containsAll(of(other));
}
/**
* Check whether this stream contains all given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAll(2, 3)
*
*/
default boolean containsAll(Stream extends T> other) {
return containsAll(seq(other));
}
/**
* Check whether this stream contains all given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAll(2, 3)
*
*/
default boolean containsAll(Iterable extends T> other) {
return containsAll(seq(other));
}
/**
* Check whether this stream contains all given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAll(2, 3)
*
*/
default boolean containsAll(Seq extends T> other) {
Set extends T> set = other.toSet(HashSet::new);
return set.isEmpty() ? true : filter(t -> set.remove(t)).anyMatch(t -> set.isEmpty());
}
/**
* Check whether this stream contains any of the given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAny(2, 4)
*
*/
default boolean containsAny(T... other) {
return containsAny(of(other));
}
/**
* Check whether this stream contains any of the given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAny(2, 4)
*
*/
default boolean containsAny(Stream extends T> other) {
return containsAny(seq(other));
}
/**
* Check whether this stream contains any of the given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAny(2, 4)
*
*/
default boolean containsAny(Iterable extends T> other) {
return containsAny(seq(other));
}
/**
* Check whether this stream contains any of the given values.
*
*
* // true
* Seq.of(1, 2, 3).containsAny(2, 4)
*
*/
default boolean containsAny(Seq extends T> other) {
Set extends T> set = other.toSet(HashSet::new);
return set.isEmpty() ? false : anyMatch(set::contains);
}
/**
* Get a single element from the stream at a given index.
*/
default Optional get(long index) {
if (index < 0L)
return Optional.empty();
else if (index == 0L)
return findFirst();
else
return skip(index).findFirst();
}
/**
* Get the single element from the stream, or throw an exception if the
* stream holds more than one element.
*/
default Optional findSingle() throws TooManyElementsException {
Iterator it = iterator();
if (!it.hasNext())
return Optional.empty();
T result = it.next();
if (!it.hasNext())
return Optional.of(result);
throw new TooManyElementsException("Stream contained more than one element.");
}
/**
* Get a single element from the stream given a predicate.
*/
default Optional findFirst(Predicate super T> predicate) {
return filter(predicate).findFirst();
}
/**
* Return a new stream where the first occurrence of the argument is removed.
*
*
* // 1, 3, 2, 4
* Seq.of(1, 2, 3, 2, 4).remove(2)
*
*/
default Seq remove(T other) {
boolean[] removed = new boolean[1];
return filter(t -> removed[0] || !(removed[0] = Objects.equals(t, other)));
}
/**
* Return a new stream where all occurrences of the arguments are removed.
*
*
* // 1, 4
* Seq.of(1, 2, 3, 2, 4).removeAll(2, 3)
*
*/
default Seq removeAll(T... other) {
return removeAll(of(other));
}
/**
* Return a new stream where all occurrences of the arguments are removed.
*
*
* // 1, 4
* Seq.of(1, 2, 3, 2, 4).removeAll(2, 3)
*
*/
default Seq removeAll(Stream extends T> other) {
return removeAll(seq(other));
}
/**
* Return a new stream where all occurrences of the arguments are removed.
*
*
* // 1, 4
* Seq.of(1, 2, 3, 2, 4).removeAll(2, 3)
*
*/
default Seq removeAll(Iterable extends T> other) {
return removeAll(seq(other));
}
/**
* Return a new stream where all occurrences of the arguments are removed.
*
*
* // 1, 4
* Seq.of(1, 2, 3, 2, 4).removeAll(2, 3)
*
*/
default Seq removeAll(Seq extends T> other) {
Set extends T> set = other.toSet(HashSet::new);
return set.isEmpty() ? this : filter(t -> !set.contains(t)).onClose(other::close);
}
/**
* Return a new stream where only occurrences of the arguments are retained.
*
*
* // 2, 3, 2
* Seq.of(1, 2, 3, 2, 4).retainAll(2, 3)
*
*/
default Seq retainAll(T... other) {
return retainAll(of(other));
}
/**
* Return a new stream where only occurrences of the arguments are retained.
*
*
* // 2, 3, 2
* Seq.of(1, 2, 3, 2, 4).retainAll(2, 3)
*
*/
default Seq retainAll(Stream extends T> other) {
return retainAll(seq(other));
}
/**
* Return a new stream where only occurrences of the arguments are retained.
*
*
* // 2, 3, 2
* Seq.of(1, 2, 3, 2, 4).retainAll(2, 3)
*
*/
default Seq retainAll(Iterable extends T> other) {
return retainAll(seq(other));
}
/**
* Return a new stream where only occurrences of the arguments are retained.
*
*
* // 2, 3, 2
* Seq.of(1, 2, 3, 2, 4).retainAll(2, 3)
*
*/
default Seq retainAll(Seq extends T> other) {
Set extends T> set = other.toSet(HashSet::new);
return set.isEmpty() ? empty() : filter(t -> set.contains(t)).onClose(other::close);
}
/**
* Repeat a stream infinitely.
*
*
* // (1, 2, 3, 1, 2, 3, ...)
* Seq.of(1, 2, 3).cycle();
*
*
* @see #cycle(Stream)
*/
default Seq cycle() {
return cycle(this);
}
/**
* Repeat a stream a certain amount of times.
*
*
* // ()
* Seq.of(1, 2, 3).cycle(0);
*
* // (1, 2, 3)
* Seq.of(1, 2, 3).cycle(1);
*
* // (1, 2, 3, 1, 2, 3, 1, 2, 3)
* Seq.of(1, 2, 3).cycle(3);
*
*
* @see #cycle(Stream, long)
*/
default Seq cycle(long times) {
return cycle(this, times);
}
/**
* Get a stream of distinct keys.
*
*
* // (1, 2, 3)
* Seq.of(1, 1, 2, -2, 3).distinct(Math::abs)
*
*/
default Seq distinct(Function super T, ? extends U> keyExtractor) {
final Map seen = new ConcurrentHashMap<>();
return filter(t -> seen.put(keyExtractor.apply(t), "") == null);
}
/**
* Zip two streams into one.
*
*
* // (tuple(1, "a"), tuple(2, "b"), tuple(3, "c"))
* Seq.of(1, 2, 3).zip(Seq.of("a", "b", "c"))
*
*
* @see #zip(Stream, Stream)
*/
default Seq> zip(Stream extends U> other) {
return zip(seq(other));
}
/**
* Zip two streams into one.
*
*
* // (tuple(1, "a"), tuple(2, "b"), tuple(3, "c"))
* Seq.of(1, 2, 3).zip(Seq.of("a", "b", "c"))
*
*
* @see #zip(Stream, Stream)
*/
default Seq> zip(Iterable extends U> other) {
return zip(seq(other));
}
/**
* Zip two streams into one.
*
*
* // (tuple(1, "a"), tuple(2, "b"), tuple(3, "c"))
* Seq.of(1, 2, 3).zip(Seq.of("a", "b", "c"))
*
*
* @see #zip(Stream, Stream)
*/
default Seq> zip(Seq extends U> other) {
return zip(this, other);
}
/**
* Zip two streams into one using a {@link BiFunction} to produce resulting values.
*
*
* // ("1:a", "2:b", "3:c")
* Seq.of(1, 2, 3).zip(Seq.of("a", "b", "c"), (i, s) -> i + ":" + s)
*
*
* @see #zip(Seq, BiFunction)
*/
default Seq zip(Stream extends U> other, BiFunction super T, ? super U, ? extends R> zipper) {
return zip(seq(other), zipper);
}
/**
* Zip two streams into one using a {@link BiFunction} to produce resulting values.
*
*
* // ("1:a", "2:b", "3:c")
* Seq.of(1, 2, 3).zip(Seq.of("a", "b", "c"), (i, s) -> i + ":" + s)
*
*
* @see #zip(Seq, BiFunction)
*/
default Seq zip(Iterable extends U> other, BiFunction super T, ? super U, ? extends R> zipper) {
return zip(seq(other), zipper);
}
/**
* Zip two streams into one using a {@link BiFunction} to produce resulting values.
*
*
* // ("1:a", "2:b", "3:c")
* Seq.of(1, 2, 3).zip(Seq.of("a", "b", "c"), (i, s) -> i + ":" + s)
*
*
* @see #zip(Seq, BiFunction)
*/
default Seq zip(Seq extends U> other, BiFunction super T, ? super U, ? extends R> zipper) {
return zip(this, other, zipper);
}
// [jooq-tools] START [zip-all-static]
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, T1 default1, T2 default2) {
return zipAll(s1, s2, default1, default2, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, T1 default1, T2 default2, T3 default3) {
return zipAll(s1, s2, s3, default1, default2, default3, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, T1 default1, T2 default2, T3 default3, T4 default4) {
return zipAll(s1, s2, s3, s4, default1, default2, default3, default4, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5) {
return zipAll(s1, s2, s3, s4, s5, default1, default2, default3, default4, default5, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6) {
return zipAll(s1, s2, s3, s4, s5, s6, default1, default2, default3, default4, default5, default6, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, default1, default2, default3, default4, default5, default6, default7, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, default1, default2, default3, default4, default5, default6, default7, default8, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, default1, default2, default3, default4, default5, default6, default7, default8, default9, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, Stream extends T10> s10, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, Stream extends T10> s10, Stream extends T11> s11, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10, T11 default11) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, default11, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, Stream extends T10> s10, Stream extends T11> s11, Stream extends T12> s12, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10, T11 default11, T12 default12) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11, s12, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, default11, default12, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, Stream extends T10> s10, Stream extends T11> s11, Stream extends T12> s12, Stream extends T13> s13, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10, T11 default11, T12 default12, T13 default13) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11, s12, s13, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, default11, default12, default13, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, Stream extends T10> s10, Stream extends T11> s11, Stream extends T12> s12, Stream extends T13> s13, Stream extends T14> s14, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10, T11 default11, T12 default12, T13 default13, T14 default14) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11, s12, s13, s14, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, default11, default12, default13, default14, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, Stream extends T10> s10, Stream extends T11> s11, Stream extends T12> s12, Stream extends T13> s13, Stream extends T14> s14, Stream extends T15> s15, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10, T11 default11, T12 default12, T13 default13, T14 default14, T15 default15) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11, s12, s13, s14, s15, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, default11, default12, default13, default14, default15, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Stream extends T1> s1, Stream extends T2> s2, Stream extends T3> s3, Stream extends T4> s4, Stream extends T5> s5, Stream extends T6> s6, Stream extends T7> s7, Stream extends T8> s8, Stream extends T9> s9, Stream extends T10> s10, Stream extends T11> s11, Stream extends T12> s12, Stream extends T13> s13, Stream extends T14> s14, Stream extends T15> s15, Stream extends T16> s16, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10, T11 default11, T12 default12, T13 default13, T14 default14, T15 default15, T16 default16) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11, s12, s13, s14, s15, s16, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, default11, default12, default13, default14, default15, default16, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, T1 default1, T2 default2) {
return zipAll(s1, s2, default1, default2, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, T1 default1, T2 default2, T3 default3) {
return zipAll(s1, s2, s3, default1, default2, default3, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, Iterable extends T4> s4, T1 default1, T2 default2, T3 default3, T4 default4) {
return zipAll(s1, s2, s3, s4, default1, default2, default3, default4, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, Iterable extends T4> s4, Iterable extends T5> s5, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5) {
return zipAll(s1, s2, s3, s4, s5, default1, default2, default3, default4, default5, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, Iterable extends T4> s4, Iterable extends T5> s5, Iterable extends T6> s6, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6) {
return zipAll(s1, s2, s3, s4, s5, s6, default1, default2, default3, default4, default5, default6, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, Iterable extends T4> s4, Iterable extends T5> s5, Iterable extends T6> s6, Iterable extends T7> s7, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, default1, default2, default3, default4, default5, default6, default7, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, Iterable extends T4> s4, Iterable extends T5> s5, Iterable extends T6> s6, Iterable extends T7> s7, Iterable extends T8> s8, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, default1, default2, default3, default4, default5, default6, default7, default8, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, Iterable extends T4> s4, Iterable extends T5> s5, Iterable extends T6> s6, Iterable extends T7> s7, Iterable extends T8> s8, Iterable extends T9> s9, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, default1, default2, default3, default4, default5, default6, default7, default8, default9, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*
* // (tuple(1, "a"), tuple(2, "x"), tuple(3, "x"))
* Seq.zipAll(Seq.of(1, 2, 3), Seq.of("a"), 0, "x")
*
*/
static Seq> zipAll(Iterable extends T1> s1, Iterable extends T2> s2, Iterable extends T3> s3, Iterable extends T4> s4, Iterable extends T5> s5, Iterable extends T6> s6, Iterable extends T7> s7, Iterable extends T8> s8, Iterable extends T9> s9, Iterable extends T10> s10, T1 default1, T2 default2, T3 default3, T4 default4, T5 default5, T6 default6, T7 default7, T8 default8, T9 default9, T10 default10) {
return zipAll(s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, default1, default2, default3, default4, default5, default6, default7, default8, default9, default10, Tuple::tuple);
}
/**
* Zip two streams into one - by storing the corresponding elements from them in a tuple,
* when one of streams will end - a default value for that stream will be provided instead -
* so the resulting stream will be as long as the longest of the two streams.
*
*