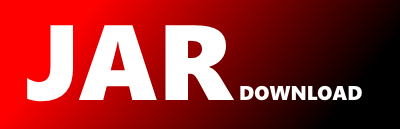
org.jooq.lambda.SeqUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jool Show documentation
Show all versions of jool Show documentation
jOOλ is part of the jOOQ series (along with jOOQ, jOOX, jOOR, jOOU) providing some useful extensions to Java 8 lambdas.
/**
* Copyright (c) 2014-2016, Data Geekery GmbH, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jooq.lambda;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Spliterator;
import java.util.TreeSet;
import java.util.function.Consumer;
import java.util.stream.Collector;
import java.util.stream.Stream;
import org.jooq.lambda.tuple.Tuple2;
import static java.util.Comparator.comparing;
import static org.jooq.lambda.Seq.seq;
/**
* @author Lukas Eder
*/
class SeqUtils {
@SuppressWarnings("unchecked")
static Seq[] seqs(Stream extends T>... streams) {
if (streams == null)
return null;
return Seq.of(streams).map(Seq::seq).toArray(Seq[]::new);
}
@SuppressWarnings("unchecked")
static Seq[] seqs(Iterable extends T>... iterables) {
if (iterables == null)
return null;
return Seq.of(iterables).map(Seq::seq).toArray(Seq[]::new);
}
static Seq transform(Stream extends T> stream, DelegatingSpliterator delegating) {
Spliterator extends T> delegate = stream.spliterator();
return Seq.seq(new Spliterator() {
@Override
public boolean tryAdvance(Consumer super U> action) {
return delegating.tryAdvance(delegate, action);
}
@Override
public Spliterator trySplit() {
return null;
}
@Override
public long estimateSize() {
return Long.MAX_VALUE;
}
@Override
public int characteristics() {
return delegate.characteristics() & Spliterator.ORDERED;
}
@Override
@SuppressWarnings("unchecked")
public Comparator super U> getComparator() {
// This implementation works with the JDK 8, as the information
// is really only used in
// java.util.stream.StreamOpFlag.fromCharacteristics(Spliterator> spliterator)
// Currently, the point of this method is only to be used for
// optimisations (e.g. to avoid sorting a stream twice in a row)
return (Comparator) delegate.getComparator();
}
}).onClose(() -> stream.close());
}
static Map, Partition> partitions(WindowSpecification window, List> input) {
return seq(input).groupBy(
window.partition().compose(t -> t.v1),
Collector.of(
() -> window.order().isPresent()
? new TreeSet>(comparing((Tuple2 t) -> t.v1, window.order().get()).thenComparing(t -> t.v2))
: new ArrayList>(),
(s, t) -> s.add(t),
(s1, s2) -> { s1.addAll(s2); return s1; },
s -> new Partition<>(s instanceof ArrayList ? (List>) s : new ArrayList<>(s))
)
);
}
/**
* Sneaky throw any type of Throwable.
*/
static void sneakyThrow(Throwable throwable) {
SeqUtils.sneakyThrow0(throwable);
}
/**
* Sneaky throw any type of Throwable.
*/
@SuppressWarnings("unchecked")
static void sneakyThrow0(Throwable throwable) throws E {
throw (E) throwable;
}
@FunctionalInterface
interface DelegatingSpliterator {
boolean tryAdvance(Spliterator extends T> delegate, Consumer super U> action);
}
static Runnable closeAll(AutoCloseable... closeables) {
return () -> {
Throwable t = null;
for (AutoCloseable closeable : closeables) {
try {
closeable.close();
}
catch (Throwable t1) {
if (t == null)
t = t1;
else
t.addSuppressed(t1);
}
}
if (t != null)
sneakyThrow(t);
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy