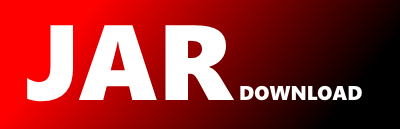
org.jooq.lambda.Window Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jool Show documentation
Show all versions of jool Show documentation
jOOλ is part of the jOOQ series (along with jOOQ, jOOX, jOOR, jOOU) providing some useful extensions to Java 8 lambdas.
/**
* Copyright (c) 2014-2016, Data Geekery GmbH, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jooq.lambda;
import java.util.Comparator;
import java.util.Optional;
import java.util.function.Function;
/**
* A window containing the data for its partition, to perform calculations upon.
*
* Window functions as exposed in this type are inspired by their counterparts
* in SQL. They include:
*
Ranking functions
* Ranking functions are useful to determine the "rank" of any given row within
* the partition, given a specific ordering. The following table explains
* individual ranking functions:
*
*
* Function Description
*
*
* {@link #rowNumber()} The distinct row number of the row within
* the partition, counting from 0
.
*
*
* {@link #rank()} The rank with gaps of a row within the partition,
* counting from 0
.
*
*
* {@link #denseRank()} The rank without gaps of a row within the
* partition, counting from 0
.
*
*
* {@link #percentRank()} Relative rank of a row:
* {@link #rank()} / {@link #count()}.
*
*
* {@link #ntile(long)} Divides the partition in equal buckets and
* assigns values between 0
and buckets - 1
.
*
*
* {@link #lead()} Gets the value after the current row.
*
*
* {@link #lag()} Gets the value before the current row.
*
*
* {@link #firstValue()} Gets the first value in the window.
*
*
* {@link #lastValue()} Gets the last value in the window.
*
*
* {@link #nthValue(long)} Gets the nth value in the window,
* counting from 0
.
*
*
*
* Note: In Java, indexes are always counted from
* 0
, not from 1
as in SQL. This means that the above
* ranking functions also rank rows from zero. This is particularly true for:
*
* - {@link #rowNumber()}
* - {@link #rank()}
* - {@link #denseRank()}
* - {@link #ntile(long)}
* - {@link #nthValue(long)}
*
*
* Aggregate functions
* Each aggregate function from {@link Seq} or from {@link Agg} is also
* available as an aggregate function on the window. For instance,
* {@link #count()} is the same as calling {@link Seq#count()} on
* {@link #window()}
*
* @author Lukas Eder
*/
public interface Window extends Collectable {
static WindowSpecification of() {
return new WindowSpecificationImpl<>(t -> SeqImpl.NULL, null, Long.MIN_VALUE, Long.MAX_VALUE);
}
static WindowSpecification of(long lower, long upper) {
return new WindowSpecificationImpl<>(t -> SeqImpl.NULL, null, lower, upper);
}
static WindowSpecification of(Comparator super T> orderBy) {
return new WindowSpecificationImpl<>(t -> SeqImpl.NULL, orderBy, Long.MIN_VALUE, 0);
}
static WindowSpecification of(Comparator super T> orderBy, long lower, long upper) {
return new WindowSpecificationImpl<>(t -> SeqImpl.NULL, orderBy, lower, upper);
}
static WindowSpecification of(Function super T, ? extends U> partitionBy) {
return new WindowSpecificationImpl<>(partitionBy, null, Long.MIN_VALUE, Long.MAX_VALUE);
}
static WindowSpecification of(Function super T, ? extends U> partitionBy, long lower, long upper) {
return new WindowSpecificationImpl<>(partitionBy, null, lower, upper);
}
static WindowSpecification of(Function super T, ? extends U> partitionBy, Comparator super T> orderBy) {
return new WindowSpecificationImpl<>(partitionBy, orderBy, Long.MIN_VALUE, 0);
}
static WindowSpecification of(Function super T, ? extends U> partitionBy, Comparator super T> orderBy, long lower, long upper) {
return new WindowSpecificationImpl<>(partitionBy, orderBy, lower, upper);
}
/**
* The value of the current row in the window.
*/
T value();
/**
* Stream all elements in the window.
*/
Seq window();
/**
* The row number of the current row within the partition.
*
*
* // (1, 2, 3, 4, 5)
* Seq.of(1, 2, 4, 2, 3).window().map(w -> w.rowNumber());
*
*/
long rowNumber();
/**
* The rank of the current row within the partition.
*
*
* // (1, 2, 2, 4, 5)
* Seq.of(1, 2, 2, 3, 4).window(naturalOrder()).map(w -> w.rank());
*
*/
long rank();
/**
* The dense rank of the current row within the partition.
*
*
* // (1, 2, 2, 3, 4)
* Seq.of(1, 2, 2, 3, 4).window(naturalOrder()).map(w -> w.denseRank());
*
*/
long denseRank();
/**
* The precent rank of the current row within the partition.
*
*
* // (0.0, 0.25, 0.25, 0.75, 1.0)
* Seq.of(1, 2, 2, 3, 4).window(naturalOrder()).map(w -> w.percentRank());
*
*/
double percentRank();
/**
* The bucket number ("ntile") of the current row within the partition.
*
*
* // (0, 0, 1, 1, 2)
* Seq.of(1, 2, 2, 3, 4).window(naturalOrder()).map(w -> w.ntile(3));
*
*/
long ntile(long buckets);
/**
* The next value in the window.
*
* This is the same as calling lead(1)
*
*
* // (2, 2, 3, 4, empty)
* Seq.of(1, 2, 2, 3, 4).window().map(w -> w.lead());
*
*/
Optional lead();
/**
* The next value by lead
in the window.
*
*
* // (2, 2, 3, 4, empty)
* Seq.of(1, 2, 2, 3, 4).window().map(w -> w.lead());
*
*/
Optional lead(long lead);
/**
* The previous value in the window.
*
* This is the same as calling lag(1)
*
*
* // (empty, 1, 2, 2, 3)
* Seq.of(1, 2, 2, 3, 4).window().map(w -> w.lag());
*
*/
Optional lag();
/**
* The previous value by lag
in the window.
*
*
* // (empty, 1, 2, 2, 3)
* Seq.of(1, 2, 2, 3, 4).window().map(w -> w.lag());
*
*/
Optional lag(long lag);
/**
* The first value in the window.
*
*
* // (1, 1, 1, 1, 1)
* Seq.of(1, 2, 4, 2, 3).window().map(w -> w.firstValue());
*
*/
Optional firstValue();
/**
* The first value in the window.
*
*
* // (1, 1, 1, 1, 1)
* Seq.of(1, 2, 4, 2, 3).window().map(w -> w.firstValue());
*
*/
Optional firstValue(Function super T, ? extends U> function);
/**
* The last value in the window.
*
*
* // (3, 3, 3, 3, 3)
* Seq.of(1, 2, 4, 2, 3).window().map(w -> w.lastValue());
*
*/
Optional lastValue();
/**
* The last value in the window.
*
*
* // (3, 3, 3, 3, 3)
* Seq.of(1, 2, 4, 2, 3).window().map(w -> w.lastValue());
*
*/
Optional lastValue(Function super T, ? extends U> function);
/**
* The nth value in the window.
*
*
* // (4, 4, 4, 4, 4)
* Seq.of(1, 2, 4, 2, 3).window().map(w -> w.nthValue(2));
*
*/
Optional nthValue(long n);
/**
* The nth value in the window.
*
*
* // (4, 4, 4, 4, 4)
* Seq.of(1, 2, 4, 2, 3).window().map(w -> w.nthValue(2));
*
*/
Optional nthValue(long n, Function super T, ? extends U> function);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy