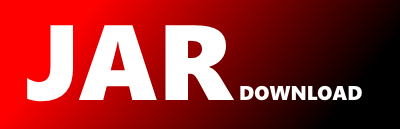
org.jooq.lambda.tuple.Tuple2 Maven / Gradle / Ivy
Show all versions of jool Show documentation
/**
* Copyright (c) 2014-2015, Data Geekery GmbH, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jooq.lambda.tuple;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import org.jooq.lambda.function.Function1;
import org.jooq.lambda.function.Function2;
/**
* A tuple of degree 2.
*
* @author Lukas Eder
*/
public class Tuple2 implements Tuple, Comparable>, Serializable, Cloneable {
private static final long serialVersionUID = 1L;
public final T1 v1;
public final T2 v2;
public T1 v1() {
return v1;
}
public T2 v2() {
return v2;
}
public Tuple2(Tuple2 tuple) {
this.v1 = tuple.v1;
this.v2 = tuple.v2;
}
public Tuple2(T1 v1, T2 v2) {
this.v1 = v1;
this.v2 = v2;
}
/**
* Concatenate a value to this tuple.
*/
public final Tuple3 concat(T3 value) {
return new Tuple3<>(v1, v2, value);
}
/**
* Concatenate a tuple to this tuple.
*/
public final Tuple3 concat(Tuple1 tuple) {
return new Tuple3<>(v1, v2, tuple.v1);
}
/**
* Concatenate a tuple to this tuple.
*/
public final Tuple4 concat(Tuple2 tuple) {
return new Tuple4<>(v1, v2, tuple.v1, tuple.v2);
}
/**
* Concatenate a tuple to this tuple.
*/
public final Tuple5 concat(Tuple3 tuple) {
return new Tuple5<>(v1, v2, tuple.v1, tuple.v2, tuple.v3);
}
/**
* Concatenate a tuple to this tuple.
*/
public final Tuple6 concat(Tuple4 tuple) {
return new Tuple6<>(v1, v2, tuple.v1, tuple.v2, tuple.v3, tuple.v4);
}
/**
* Concatenate a tuple to this tuple.
*/
public final Tuple7 concat(Tuple5 tuple) {
return new Tuple7<>(v1, v2, tuple.v1, tuple.v2, tuple.v3, tuple.v4, tuple.v5);
}
/**
* Concatenate a tuple to this tuple.
*/
public final Tuple8 concat(Tuple6 tuple) {
return new Tuple8<>(v1, v2, tuple.v1, tuple.v2, tuple.v3, tuple.v4, tuple.v5, tuple.v6);
}
/**
* Get a tuple with the two attributes swapped.
*/
public final Tuple2 swap() {
return new Tuple2<>(v2, v1);
}
/**
* Whether two tuples overlap.
*
*
* // true
* range(1, 3).overlaps(range(2, 4))
*
* // false
* range(1, 3).overlaps(range(5, 8))
*
*/
public static final > boolean overlaps(Tuple2 left, Tuple2 right) {
return left.v1.compareTo(right.v2) <= 0
&& left.v2.compareTo(right.v1) >= 0;
}
/**
* The intersection of two ranges.
*
*
* // (2, 3)
* range(1, 3).intersect(range(2, 4))
*
* // none
* range(1, 3).intersect(range(5, 8))
*
*/
public static final > Optional> intersect(Tuple2 left, Tuple2 right) {
if (overlaps(left, right))
return Optional.of(new Tuple2<>(
left.v1.compareTo(right.v1) >= 0 ? left.v1 : right.v1,
left.v2.compareTo(right.v2) <= 0 ? left.v2 : right.v2
));
else
return Optional.empty();
}
/**
* Apply this tuple as arguments to a function.
*/
public final R map(Function2 function) {
return function.apply(this);
}
/**
* Apply attribute 1 as argument to a function and return a new tuple with the substituted argument.
*/
public final Tuple2 map1(Function1 super T1, ? extends U1> function) {
return Tuple.tuple(function.apply(v1), v2);
}
/**
* Apply attribute 2 as argument to a function and return a new tuple with the substituted argument.
*/
public final Tuple2 map2(Function1 super T2, ? extends U2> function) {
return Tuple.tuple(v1, function.apply(v2));
}
@Override
public final Object[] array() {
return new Object[] { v1, v2 };
}
@Override
public final List> list() {
return Arrays.asList(array());
}
/**
* The degree of this tuple: 2.
*/
@Override
public final int degree() {
return 2;
}
@Override
@SuppressWarnings("unchecked")
public final Iterator