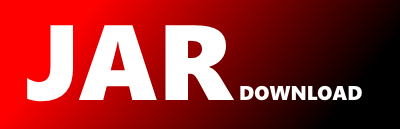
org.jooq.util.maven.example.postgres.Routines Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jooq-codegen-maven-example Show documentation
Show all versions of jooq-codegen-maven-example Show documentation
jOOQ effectively combines complex SQL, typesafety, source code generation, active records, stored procedures, advanced data types, and Java in a fluent, intuitive DSL.
The newest version!
/**
* This class is generated by jOOQ
*/
package org.jooq.util.maven.example.postgres;
/**
* This class is generated by jOOQ.
*
* Convenience access to all stored procedures and functions in public
*/
@javax.annotation.Generated(value = {"http://www.jooq.org", "2.3.3"},
comments = "This class is generated by jOOQ")
public final class Routines {
/**
* Call public.f_arrays
*
* @param inArray
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Integer[] fArrays1(org.jooq.Configuration configuration, java.lang.Integer[] inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays1 f = new org.jooq.util.maven.example.postgres.routines.FArrays1();
f.setInArray(inArray);
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f_arrays as a field
*
* @param inArray
*/
public static org.jooq.Field fArrays1(java.lang.Integer[] inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays1 f = new org.jooq.util.maven.example.postgres.routines.FArrays1();
f.setInArray(inArray);
return f.asField();
}
/**
* Get public.f_arrays as a field
*
* @param inArray
*/
public static org.jooq.Field fArrays1(org.jooq.Field inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays1 f = new org.jooq.util.maven.example.postgres.routines.FArrays1();
f.setInArray(inArray);
return f.asField();
}
/**
* Call public.f_arrays
*
* @param inArray
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Long[] fArrays2(org.jooq.Configuration configuration, java.lang.Long[] inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays2 f = new org.jooq.util.maven.example.postgres.routines.FArrays2();
f.setInArray(inArray);
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f_arrays as a field
*
* @param inArray
*/
public static org.jooq.Field fArrays2(java.lang.Long[] inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays2 f = new org.jooq.util.maven.example.postgres.routines.FArrays2();
f.setInArray(inArray);
return f.asField();
}
/**
* Get public.f_arrays as a field
*
* @param inArray
*/
public static org.jooq.Field fArrays2(org.jooq.Field inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays2 f = new org.jooq.util.maven.example.postgres.routines.FArrays2();
f.setInArray(inArray);
return f.asField();
}
/**
* Call public.f_arrays
*
* @param inArray
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.String[] fArrays3(org.jooq.Configuration configuration, java.lang.String[] inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays3 f = new org.jooq.util.maven.example.postgres.routines.FArrays3();
f.setInArray(inArray);
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f_arrays as a field
*
* @param inArray
*/
public static org.jooq.Field fArrays3(java.lang.String[] inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays3 f = new org.jooq.util.maven.example.postgres.routines.FArrays3();
f.setInArray(inArray);
return f.asField();
}
/**
* Get public.f_arrays as a field
*
* @param inArray
*/
public static org.jooq.Field fArrays3(org.jooq.Field inArray) {
org.jooq.util.maven.example.postgres.routines.FArrays3 f = new org.jooq.util.maven.example.postgres.routines.FArrays3();
f.setInArray(inArray);
return f.asField();
}
/**
* Call public.f_author_exists
*
* @param authorName
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Integer fAuthorExists(org.jooq.Configuration configuration, java.lang.String authorName) {
org.jooq.util.maven.example.postgres.routines.FAuthorExists f = new org.jooq.util.maven.example.postgres.routines.FAuthorExists();
f.setAuthorName(authorName);
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f_author_exists as a field
*
* @param authorName
*/
public static org.jooq.Field fAuthorExists(java.lang.String authorName) {
org.jooq.util.maven.example.postgres.routines.FAuthorExists f = new org.jooq.util.maven.example.postgres.routines.FAuthorExists();
f.setAuthorName(authorName);
return f.asField();
}
/**
* Get public.f_author_exists as a field
*
* @param authorName
*/
public static org.jooq.Field fAuthorExists(org.jooq.Field authorName) {
org.jooq.util.maven.example.postgres.routines.FAuthorExists f = new org.jooq.util.maven.example.postgres.routines.FAuthorExists();
f.setAuthorName(authorName);
return f.asField();
}
/**
* Call public.f_get_one_cursor
*
* @param bookIds
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static org.jooq.Result fGetOneCursor(org.jooq.Configuration configuration, java.lang.Integer[] bookIds) {
org.jooq.util.maven.example.postgres.routines.FGetOneCursor f = new org.jooq.util.maven.example.postgres.routines.FGetOneCursor();
f.setBookIds(bookIds);
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f_get_one_cursor as a field
*
* @param bookIds
*/
public static org.jooq.Field> fGetOneCursor(java.lang.Integer[] bookIds) {
org.jooq.util.maven.example.postgres.routines.FGetOneCursor f = new org.jooq.util.maven.example.postgres.routines.FGetOneCursor();
f.setBookIds(bookIds);
return f.asField();
}
/**
* Get public.f_get_one_cursor as a field
*
* @param bookIds
*/
public static org.jooq.Field> fGetOneCursor(org.jooq.Field bookIds) {
org.jooq.util.maven.example.postgres.routines.FGetOneCursor f = new org.jooq.util.maven.example.postgres.routines.FGetOneCursor();
f.setBookIds(bookIds);
return f.asField();
}
/**
* Call public.f_number
*
* @param n
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Integer fNumber(org.jooq.Configuration configuration, java.lang.Integer n) {
org.jooq.util.maven.example.postgres.routines.FNumber f = new org.jooq.util.maven.example.postgres.routines.FNumber();
f.setN(n);
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f_number as a field
*
* @param n
*/
public static org.jooq.Field fNumber(java.lang.Integer n) {
org.jooq.util.maven.example.postgres.routines.FNumber f = new org.jooq.util.maven.example.postgres.routines.FNumber();
f.setN(n);
return f.asField();
}
/**
* Get public.f_number as a field
*
* @param n
*/
public static org.jooq.Field fNumber(org.jooq.Field n) {
org.jooq.util.maven.example.postgres.routines.FNumber f = new org.jooq.util.maven.example.postgres.routines.FNumber();
f.setN(n);
return f.asField();
}
/**
* Call public.f_one
*
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Integer fOne(org.jooq.Configuration configuration) {
org.jooq.util.maven.example.postgres.routines.FOne f = new org.jooq.util.maven.example.postgres.routines.FOne();
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f_one as a field
*
*/
public static org.jooq.Field fOne() {
org.jooq.util.maven.example.postgres.routines.FOne f = new org.jooq.util.maven.example.postgres.routines.FOne();
return f.asField();
}
/**
* Call public.f317
*
* @param p1
* @param p2
* @param p3
* @param p4
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Integer f317(org.jooq.Configuration configuration, java.lang.Integer p1, java.lang.Integer p2, java.lang.Integer p3, java.lang.Integer p4) {
org.jooq.util.maven.example.postgres.routines.F317 f = new org.jooq.util.maven.example.postgres.routines.F317();
f.setP1(p1);
f.setP2(p2);
f.setP3(p3);
f.setP4(p4);
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.f317 as a field
*
* @param p1
* @param p2
* @param p3
* @param p4
*/
public static org.jooq.Field f317(java.lang.Integer p1, java.lang.Integer p2, java.lang.Integer p3, java.lang.Integer p4) {
org.jooq.util.maven.example.postgres.routines.F317 f = new org.jooq.util.maven.example.postgres.routines.F317();
f.setP1(p1);
f.setP2(p2);
f.setP3(p3);
f.setP4(p4);
return f.asField();
}
/**
* Get public.f317 as a field
*
* @param p1
* @param p2
* @param p3
* @param p4
*/
public static org.jooq.Field f317(org.jooq.Field p1, org.jooq.Field p2, org.jooq.Field p3, org.jooq.Field p4) {
org.jooq.util.maven.example.postgres.routines.F317 f = new org.jooq.util.maven.example.postgres.routines.F317();
f.setP1(p1);
f.setP2(p2);
f.setP3(p3);
f.setP4(p4);
return f.asField();
}
/**
* Call public.p_arrays
*
* @param inArray IN parameter
* @param outArray OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Long[] pArrays2(org.jooq.Configuration configuration, java.lang.Long[] inArray) {
org.jooq.util.maven.example.postgres.routines.PArrays2 p = new org.jooq.util.maven.example.postgres.routines.PArrays2();
p.setInArray(inArray);
p.execute(configuration);
return p.getOutArray();
}
/**
* Call public.p_arrays
*
* @param inArray IN parameter
* @param outArray OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Integer[] pArrays1(org.jooq.Configuration configuration, java.lang.Integer[] inArray) {
org.jooq.util.maven.example.postgres.routines.PArrays1 p = new org.jooq.util.maven.example.postgres.routines.PArrays1();
p.setInArray(inArray);
p.execute(configuration);
return p.getOutArray();
}
/**
* Call public.p_arrays
*
* @param inArray IN parameter
* @param outArray OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.String[] pArrays3(org.jooq.Configuration configuration, java.lang.String[] inArray) {
org.jooq.util.maven.example.postgres.routines.PArrays3 p = new org.jooq.util.maven.example.postgres.routines.PArrays3();
p.setInArray(inArray);
p.execute(configuration);
return p.getOutArray();
}
/**
* Call public.p_author_exists
*
* @param authorName IN parameter
* @param result OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Integer pAuthorExists(org.jooq.Configuration configuration, java.lang.String authorName) {
org.jooq.util.maven.example.postgres.routines.PAuthorExists p = new org.jooq.util.maven.example.postgres.routines.PAuthorExists();
p.setAuthorName(authorName);
p.execute(configuration);
return p.getResult();
}
/**
* Call public.p_create_author
*
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static void pCreateAuthor(org.jooq.Configuration configuration) {
org.jooq.util.maven.example.postgres.routines.PCreateAuthor p = new org.jooq.util.maven.example.postgres.routines.PCreateAuthor();
p.execute(configuration);
}
/**
* Call public.p_create_author_by_name
*
* @param firstName IN parameter
* @param lastName IN parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static void pCreateAuthorByName(org.jooq.Configuration configuration, java.lang.String firstName, java.lang.String lastName) {
org.jooq.util.maven.example.postgres.routines.PCreateAuthorByName p = new org.jooq.util.maven.example.postgres.routines.PCreateAuthorByName();
p.setFirstName(firstName);
p.setLastName(lastName);
p.execute(configuration);
}
/**
* Call public.p_enhance_address1
*
* @param address IN parameter
* @param no OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.String pEnhanceAddress1(org.jooq.Configuration configuration, org.jooq.util.maven.example.postgres.udt.records.UAddressTypeRecord address) {
org.jooq.util.maven.example.postgres.routines.PEnhanceAddress1 p = new org.jooq.util.maven.example.postgres.routines.PEnhanceAddress1();
p.setAddress(address);
p.execute(configuration);
return p.getNo();
}
/**
* Call public.p_enhance_address2
*
* @param address OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static org.jooq.util.maven.example.postgres.udt.records.UAddressTypeRecord pEnhanceAddress2(org.jooq.Configuration configuration) {
org.jooq.util.maven.example.postgres.routines.PEnhanceAddress2 p = new org.jooq.util.maven.example.postgres.routines.PEnhanceAddress2();
p.execute(configuration);
return p.getAddress();
}
/**
* Call public.p_enhance_address3
*
* @param address IN OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static org.jooq.util.maven.example.postgres.udt.records.UAddressTypeRecord pEnhanceAddress3(org.jooq.Configuration configuration, org.jooq.util.maven.example.postgres.udt.records.UAddressTypeRecord address) {
org.jooq.util.maven.example.postgres.routines.PEnhanceAddress3 p = new org.jooq.util.maven.example.postgres.routines.PEnhanceAddress3();
p.setAddress(address);
p.execute(configuration);
return p.getAddress();
}
/**
* Call public.p_get_one_cursor
*
* @param total OUT parameter
* @param books OUT parameter
* @param bookIds IN parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static org.jooq.util.maven.example.postgres.routines.PGetOneCursor pGetOneCursor(org.jooq.Configuration configuration, java.lang.Integer[] bookIds) {
org.jooq.util.maven.example.postgres.routines.PGetOneCursor p = new org.jooq.util.maven.example.postgres.routines.PGetOneCursor();
p.setBookIds(bookIds);
p.execute(configuration);
return p;
}
/**
* Call public.p_get_two_cursors
*
* @param books OUT parameter
* @param authors OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static org.jooq.util.maven.example.postgres.routines.PGetTwoCursors pGetTwoCursors(org.jooq.Configuration configuration) {
org.jooq.util.maven.example.postgres.routines.PGetTwoCursors p = new org.jooq.util.maven.example.postgres.routines.PGetTwoCursors();
p.execute(configuration);
return p;
}
/**
* Call public.p_triggers
*
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static java.lang.Object pTriggers(org.jooq.Configuration configuration) {
org.jooq.util.maven.example.postgres.routines.PTriggers f = new org.jooq.util.maven.example.postgres.routines.PTriggers();
f.execute(configuration);
return f.getReturnValue();
}
/**
* Get public.p_triggers as a field
*
*/
public static org.jooq.Field pTriggers() {
org.jooq.util.maven.example.postgres.routines.PTriggers f = new org.jooq.util.maven.example.postgres.routines.PTriggers();
return f.asField();
}
/**
* Call public.p_unused
*
* @param in1 IN parameter
* @param out1 OUT parameter
* @param out2 IN OUT parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static org.jooq.util.maven.example.postgres.routines.PUnused pUnused(org.jooq.Configuration configuration, java.lang.String in1, java.lang.Integer out2) {
org.jooq.util.maven.example.postgres.routines.PUnused p = new org.jooq.util.maven.example.postgres.routines.PUnused();
p.setIn1(in1);
p.setOut2(out2);
p.execute(configuration);
return p;
}
/**
* Call public.p391
*
* @param i1 IN parameter
* @param io1 IN OUT parameter
* @param o1 OUT parameter
* @param o2 OUT parameter
* @param io2 IN OUT parameter
* @param i2 IN parameter
* @throws org.jooq.exception.DataAccessException if something went wrong executing the query
*/
public static org.jooq.util.maven.example.postgres.routines.P391 p391(org.jooq.Configuration configuration, java.lang.Integer i1, java.lang.Integer io1, java.lang.Integer io2, java.lang.Integer i2) {
org.jooq.util.maven.example.postgres.routines.P391 p = new org.jooq.util.maven.example.postgres.routines.P391();
p.setI1(i1);
p.setIo1(io1);
p.setIo2(io2);
p.setI2(i2);
p.execute(configuration);
return p;
}
/**
* No instances
*/
private Routines() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy