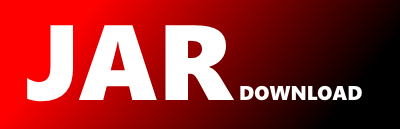
org.jooq.util.jaxb.Database Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.0.5-b02-fcs
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.16 at 11:15:20 AM MEZ
//
package org.jooq.util.jaxb;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for Database complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Database">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <all>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="includes" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="excludes" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="dateAsTimestamp" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="unsignedTypes" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="inputSchema" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="outputSchema" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="schemata" type="{http://www.jooq.org/xsd/jooq-codegen-2.1.0.xsd}Schemata" minOccurs="0"/>
* <element name="masterDataTables" type="{http://www.jooq.org/xsd/jooq-codegen-2.1.0.xsd}MasterDataTables" minOccurs="0"/>
* <element name="customTypes" type="{http://www.jooq.org/xsd/jooq-codegen-2.1.0.xsd}CustomTypes" minOccurs="0"/>
* <element name="enumTypes" type="{http://www.jooq.org/xsd/jooq-codegen-2.1.0.xsd}EnumTypes" minOccurs="0"/>
* <element name="forcedTypes" type="{http://www.jooq.org/xsd/jooq-codegen-2.1.0.xsd}ForcedTypes" minOccurs="0"/>
* </all>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Database", propOrder = {
})
public class Database {
@XmlElement(required = true)
protected String name;
@XmlElement(defaultValue = ".*")
protected String includes = ".*";
@XmlElement(defaultValue = "")
protected String excludes = "";
@XmlElement(defaultValue = "false")
protected Boolean dateAsTimestamp = false;
@XmlElement(defaultValue = "true")
protected Boolean unsignedTypes = true;
@XmlElement(defaultValue = "")
protected String inputSchema = "";
@XmlElement(defaultValue = "")
protected String outputSchema = "";
@XmlElementWrapper(name = "schemata")
@XmlElement(name = "schema")
protected List schemata;
@XmlElementWrapper(name = "masterDataTables")
@XmlElement(name = "masterDataTable")
protected List masterDataTables;
@XmlElementWrapper(name = "customTypes")
@XmlElement(name = "customType")
protected List customTypes;
@XmlElementWrapper(name = "enumTypes")
@XmlElement(name = "enumType")
protected List enumTypes;
@XmlElementWrapper(name = "forcedTypes")
@XmlElement(name = "forcedType")
protected List forcedTypes;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the includes property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIncludes() {
return includes;
}
/**
* Sets the value of the includes property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIncludes(String value) {
this.includes = value;
}
/**
* Gets the value of the excludes property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExcludes() {
return excludes;
}
/**
* Sets the value of the excludes property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExcludes(String value) {
this.excludes = value;
}
/**
* Gets the value of the dateAsTimestamp property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isDateAsTimestamp() {
return dateAsTimestamp;
}
/**
* Sets the value of the dateAsTimestamp property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setDateAsTimestamp(Boolean value) {
this.dateAsTimestamp = value;
}
/**
* Gets the value of the unsignedTypes property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isUnsignedTypes() {
return unsignedTypes;
}
/**
* Sets the value of the unsignedTypes property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setUnsignedTypes(Boolean value) {
this.unsignedTypes = value;
}
/**
* Gets the value of the inputSchema property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInputSchema() {
return inputSchema;
}
/**
* Sets the value of the inputSchema property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setInputSchema(String value) {
this.inputSchema = value;
}
/**
* Gets the value of the outputSchema property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOutputSchema() {
return outputSchema;
}
/**
* Sets the value of the outputSchema property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOutputSchema(String value) {
this.outputSchema = value;
}
public List getSchemata() {
if (schemata == null) {
schemata = new ArrayList();
}
return schemata;
}
public List getMasterDataTables() {
if (masterDataTables == null) {
masterDataTables = new ArrayList();
}
return masterDataTables;
}
public List getCustomTypes() {
if (customTypes == null) {
customTypes = new ArrayList();
}
return customTypes;
}
public List getEnumTypes() {
if (enumTypes == null) {
enumTypes = new ArrayList();
}
return enumTypes;
}
public List getForcedTypes() {
if (forcedTypes == null) {
forcedTypes = new ArrayList();
}
return forcedTypes;
}
public Database withName(String value) {
setName(value);
return this;
}
public Database withIncludes(String value) {
setIncludes(value);
return this;
}
public Database withExcludes(String value) {
setExcludes(value);
return this;
}
public Database withDateAsTimestamp(Boolean value) {
setDateAsTimestamp(value);
return this;
}
public Database withUnsignedTypes(Boolean value) {
setUnsignedTypes(value);
return this;
}
public Database withInputSchema(String value) {
setInputSchema(value);
return this;
}
public Database withOutputSchema(String value) {
setOutputSchema(value);
return this;
}
public Database withSchemata(Schema... values) {
if (values!= null) {
for (Schema value: values) {
getSchemata().add(value);
}
}
return this;
}
public Database withSchemata(Collection values) {
if (values!= null) {
getSchemata().addAll(values);
}
return this;
}
public Database withMasterDataTables(MasterDataTable... values) {
if (values!= null) {
for (MasterDataTable value: values) {
getMasterDataTables().add(value);
}
}
return this;
}
public Database withMasterDataTables(Collection values) {
if (values!= null) {
getMasterDataTables().addAll(values);
}
return this;
}
public Database withCustomTypes(CustomType... values) {
if (values!= null) {
for (CustomType value: values) {
getCustomTypes().add(value);
}
}
return this;
}
public Database withCustomTypes(Collection values) {
if (values!= null) {
getCustomTypes().addAll(values);
}
return this;
}
public Database withEnumTypes(EnumType... values) {
if (values!= null) {
for (EnumType value: values) {
getEnumTypes().add(value);
}
}
return this;
}
public Database withEnumTypes(Collection values) {
if (values!= null) {
getEnumTypes().addAll(values);
}
return this;
}
public Database withForcedTypes(ForcedType... values) {
if (values!= null) {
for (ForcedType value: values) {
getForcedTypes().add(value);
}
}
return this;
}
public Database withForcedTypes(Collection values) {
if (values!= null) {
getForcedTypes().addAll(values);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy