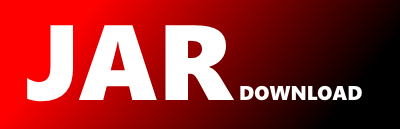
org.jooq.meta.AbstractMetaDatabase Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses for this work are available. These replace the above
* ASL 2.0 and offer limited warranties, support, maintenance, and commercial
* database integrations.
*
* For more information, please visit: http://www.jooq.org/licenses
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*/
package org.jooq.meta;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import org.jooq.Catalog;
import org.jooq.DSLContext;
import org.jooq.Field;
import org.jooq.ForeignKey;
import org.jooq.Meta;
import org.jooq.Schema;
import org.jooq.Sequence;
import org.jooq.Table;
import org.jooq.UniqueKey;
import org.jooq.impl.DSL;
/**
* The base implementation for {@link Meta} based databases.
*
* @author Lukas Eder
*/
public abstract class AbstractMetaDatabase extends AbstractDatabase {
private List catalogs;
private List schemas;
@Override
protected DSLContext create0() {
return DSL.using(getConnection());
}
abstract protected Meta getMeta0();
@Override
protected void loadPrimaryKeys(DefaultRelations relations) throws SQLException {
}
@Override
protected void loadUniqueKeys(DefaultRelations relations) throws SQLException {
for (Schema schema : getSchemasFromMeta()) {
SchemaDefinition s = getSchema(schema.getName());
if (s != null) {
for (Table> table : schema.getTables()) {
TableDefinition t = getTable(s, table.getName());
if (t != null) {
UniqueKey> key = table.getPrimaryKey();
if (key != null)
for (Field> field : key.getFields())
relations.addPrimaryKey("PK_" + key.getTable().getName(), t, t.getColumn(field.getName()));
}
}
}
}
}
@Override
protected void loadForeignKeys(DefaultRelations relations) throws SQLException {
for (Schema referencingS : getSchemasFromMeta()) {
SchemaDefinition referencingSD = getSchema(referencingS.getName());
if (referencingSD != null) {
for (Table> referencingT : referencingS.getTables()) {
TableDefinition referencingTD = getTable(referencingSD, referencingT.getName());
if (referencingTD != null) {
for (ForeignKey, ?> fk : referencingT.getReferences()) {
UniqueKey> uk = fk.getKey();
if (uk != null) {
Table> referencedT = uk.getTable();
if (referencedT != null) {
Schema referencedS = referencedT.getSchema();
if (referencedS == null)
referencedS = referencingS;
SchemaDefinition referencedSD = getSchema(referencedS.getName());
TableDefinition referencedTD = getTable(referencedSD, referencedT.getName());
addForeignKey:
if (referencedTD != null) {
for (Field> fkField : fk.getFields())
if (referencingTD.getColumn(fkField.getName()) == null)
break addForeignKey;
for (Field> fkField : fk.getFields())
relations.addForeignKey(
fk.getName(),
referencingTD,
referencingTD.getColumn(fkField.getName()),
uk.getName(),
referencedTD
);
}
}
}
}
}
}
}
}
}
@Override
protected void loadCheckConstraints(DefaultRelations r) throws SQLException {
}
@Override
protected List getCatalogs0() throws SQLException {
List result = new ArrayList<>();
for (Catalog catalog : getCatalogsFromMeta())
result.add(new CatalogDefinition(this, catalog.getName(), ""));
result.sort(COMP);
return result;
}
private List getCatalogsFromMeta() {
if (catalogs == null)
catalogs = new ArrayList<>(getMeta0().getCatalogs());
return catalogs;
}
@Override
protected List getSchemata0() throws SQLException {
List result = new ArrayList<>();
for (Schema schema : getSchemasFromMeta()) {
if (schema.getCatalog() != null) {
CatalogDefinition catalog = getCatalog(schema.getCatalog().getName());
if (catalog != null)
result.add(new SchemaDefinition(this, schema.getName(), "", catalog));
}
else
result.add(new SchemaDefinition(this, schema.getName(), ""));
}
result.sort(COMP);
return result;
}
private List getSchemasFromMeta() {
if (schemas == null)
schemas = new ArrayList<>(getMeta0().getSchemas());
return schemas;
}
@Override
protected List getSequences0() throws SQLException {
List result = new ArrayList<>();
for (Schema schema : getSchemasFromMeta()) {
for (Sequence> sequence : schema.getSequences()) {
SchemaDefinition sd = getSchema(schema.getName());
DataTypeDefinition type = new DefaultDataTypeDefinition(
this,
sd,
sequence.getDataType().getTypeName()
);
result.add(new DefaultSequenceDefinition(
sd, sequence.getName(), type));
}
}
result.sort(COMP);
return result;
}
@Override
protected List getTables0() throws SQLException {
List result = new ArrayList<>();
for (Schema schema : getSchemasFromMeta()) {
SchemaDefinition sd = getSchema(schema.getName());
if (sd != null)
for (Table> table : schema.getTables())
result.add(new DefaultMetaTableDefinition(sd, table));
}
result.sort(COMP);
return result;
}
@Override
protected List getEnums0() throws SQLException {
List result = new ArrayList<>();
return result;
}
@Override
protected List getDomains0() throws SQLException {
List result = new ArrayList<>();
return result;
}
@Override
protected List getUDTs0() throws SQLException {
List result = new ArrayList<>();
return result;
}
@Override
protected List getArrays0() throws SQLException {
List result = new ArrayList<>();
return result;
}
@Override
protected List getRoutines0() throws SQLException {
List result = new ArrayList<>();
return result;
}
@Override
protected List getPackages0() throws SQLException {
List result = new ArrayList<>();
return result;
}
private static final Comparator COMP = Comparator.comparing(Definition::getQualifiedInputName);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy