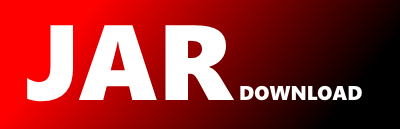
org.jooq.meta.ResultQueryDatabase Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses for this work are available. These replace the above
* Apache-2.0 license and offer limited warranties, support, maintenance, and
* commercial database integrations.
*
* For more information, please visit: https://www.jooq.org/legal/licensing
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*/
package org.jooq.meta;
import java.math.BigDecimal;
import java.util.List;
import org.jooq.Meta;
import org.jooq.Record12;
import org.jooq.Record14;
import org.jooq.Record4;
import org.jooq.Record5;
import org.jooq.Record6;
import org.jooq.ResultQuery;
// ...
// ...
import org.jetbrains.annotations.ApiStatus.Internal;
import org.jetbrains.annotations.Nullable;
/**
* An interface for all {@link AbstractDatabase} implementations that can
* produce {@link ResultQuery} objects to query meta data.
*
* These queries will be used to generate some internal queries in the core
* library's {@link Meta} API. The return types of the various methods are
* subject to change and should not be relied upon.
*
* @author Lukas Eder
*/
@Internal
public interface ResultQueryDatabase extends Database {
/**
* A query that produces primary keys for a set of input schemas.
*
* The resulting columns are:
*
* - Catalog name
* - Schema name
* - Table name
* - Constraint name
* - Column name
* - Column sequence
*
*
* @return The query or null
if this implementation doesn't support the query.
*/
@Internal
@Nullable
ResultQuery> primaryKeys(List schemas);
/**
* A query that produces (non-primary) unique keys for a set of input
* schemas.
*
* The resulting columns are:
*
* - Catalog name
* - Schema name
* - Table name
* - Constraint name
* - Column name
* - Column sequence
*
*
* @return The query or null
if this implementation doesn't support the query.
*/
@Internal
@Nullable
ResultQuery> uniqueKeys(List schemas);
/**
* A query that produces sequences for a set of input schemas.
*
* The resulting columns are:
*
* - Catalog name
* - Schema name
* - Sequence name
* - Data type name
* - Data type precision
* - Data type scale
* - Start value
* - Increment
* - Min value
* - Max value
* - Cycle
* - Cache
*
*
* @return The query or null
if this implementation doesn't support the query.
*/
@Internal
@Nullable
ResultQuery> sequences(List schemas);
/**
* A query that produces enum types and their literals for a set of input schemas.
*
* The resulting columns are:
*
* - Catalog name
* - Schema name
* - Column name (if applicable, e.g. in MySQL style RDBMS)
* - Enum type name (if applicable, e.g. in PostgreSQL style RDBMS)
* - Literal value
* - Literal position
*
*
* @return The query or null
if this implementation doesn't support the query.
*/
@Internal
@Nullable
ResultQuery> enums(List schemas);
/**
* A query that produces source code for a set of input schemas.
*
* The resulting columns are:
*
* - Catalog name
* - Schema name
* - Object name (e.g. table, view, function, package)
* - Source
*
*
* @return The query or null
if this implementation doesn't support the query.
*/
@Internal
@Nullable
ResultQuery> sources(List schemas);
/**
* A query that produces comments for a set of input schemas.
*
* The resulting columns are:
*
* - Catalog name
* - Schema name
* - Object name (e.g. table, view, function, package)
* - Object sub name (e.g. column, package routine)
* - Comment
*
*
* @return The query or null
if this implementation doesn't support the query.
*/
@Internal
@Nullable
ResultQuery> comments(List schemas);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy