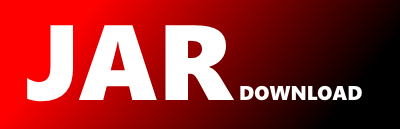
org.jooq.meta.jaxb.Matchers Maven / Gradle / Ivy
package org.jooq.meta.jaxb;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlElementWrapper;
import jakarta.xml.bind.annotation.XmlType;
import org.jooq.util.jaxb.tools.XMLAppendable;
import org.jooq.util.jaxb.tools.XMLBuilder;
/**
* Declarative naming strategy configuration.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Matchers", propOrder = {
})
@SuppressWarnings({
"all"
})
public class Matchers implements Serializable, XMLAppendable
{
private final static long serialVersionUID = 31908L;
@XmlElementWrapper(name = "catalogs")
@XmlElement(name = "catalog")
protected List catalogs;
@XmlElementWrapper(name = "schemas")
@XmlElement(name = "schema")
protected List schemas;
@XmlElementWrapper(name = "tables")
@XmlElement(name = "table")
protected List tables;
@XmlElementWrapper(name = "indexes")
@XmlElement(name = "index")
protected List indexes;
@XmlElementWrapper(name = "primaryKeys")
@XmlElement(name = "primaryKey")
protected List primaryKeys;
@XmlElementWrapper(name = "uniqueKeys")
@XmlElement(name = "uniqueKey")
protected List uniqueKeys;
@XmlElementWrapper(name = "foreignKeys")
@XmlElement(name = "foreignKey")
protected List foreignKeys;
@XmlElementWrapper(name = "fields")
@XmlElement(name = "field")
protected List fields;
@XmlElementWrapper(name = "routines")
@XmlElement(name = "routine")
protected List routines;
@XmlElementWrapper(name = "sequences")
@XmlElement(name = "sequence")
protected List sequences;
@XmlElementWrapper(name = "enums")
@XmlElement(name = "enum")
protected List enums;
@XmlElementWrapper(name = "embeddables")
@XmlElement(name = "embeddable")
protected List embeddables;
@XmlElementWrapper(name = "udts")
@XmlElement(name = "udt")
protected List udts;
@XmlElementWrapper(name = "attributes")
@XmlElement(name = "attribute")
protected List attributes;
public List getCatalogs() {
if (catalogs == null) {
catalogs = new ArrayList();
}
return catalogs;
}
public void setCatalogs(List catalogs) {
this.catalogs = catalogs;
}
public List getSchemas() {
if (schemas == null) {
schemas = new ArrayList();
}
return schemas;
}
public void setSchemas(List schemas) {
this.schemas = schemas;
}
public List getTables() {
if (tables == null) {
tables = new ArrayList();
}
return tables;
}
public void setTables(List tables) {
this.tables = tables;
}
public List getIndexes() {
if (indexes == null) {
indexes = new ArrayList();
}
return indexes;
}
public void setIndexes(List indexes) {
this.indexes = indexes;
}
public List getPrimaryKeys() {
if (primaryKeys == null) {
primaryKeys = new ArrayList();
}
return primaryKeys;
}
public void setPrimaryKeys(List primaryKeys) {
this.primaryKeys = primaryKeys;
}
public List getUniqueKeys() {
if (uniqueKeys == null) {
uniqueKeys = new ArrayList();
}
return uniqueKeys;
}
public void setUniqueKeys(List uniqueKeys) {
this.uniqueKeys = uniqueKeys;
}
public List getForeignKeys() {
if (foreignKeys == null) {
foreignKeys = new ArrayList();
}
return foreignKeys;
}
public void setForeignKeys(List foreignKeys) {
this.foreignKeys = foreignKeys;
}
public List getFields() {
if (fields == null) {
fields = new ArrayList();
}
return fields;
}
public void setFields(List fields) {
this.fields = fields;
}
public List getRoutines() {
if (routines == null) {
routines = new ArrayList();
}
return routines;
}
public void setRoutines(List routines) {
this.routines = routines;
}
public List getSequences() {
if (sequences == null) {
sequences = new ArrayList();
}
return sequences;
}
public void setSequences(List sequences) {
this.sequences = sequences;
}
public List getEnums() {
if (enums == null) {
enums = new ArrayList();
}
return enums;
}
public void setEnums(List enums) {
this.enums = enums;
}
public List getEmbeddables() {
if (embeddables == null) {
embeddables = new ArrayList();
}
return embeddables;
}
public void setEmbeddables(List embeddables) {
this.embeddables = embeddables;
}
public List getUdts() {
if (udts == null) {
udts = new ArrayList();
}
return udts;
}
public void setUdts(List udts) {
this.udts = udts;
}
public List getAttributes() {
if (attributes == null) {
attributes = new ArrayList();
}
return attributes;
}
public void setAttributes(List attributes) {
this.attributes = attributes;
}
public Matchers withCatalogs(MatchersCatalogType... values) {
if (values!= null) {
for (MatchersCatalogType value: values) {
getCatalogs().add(value);
}
}
return this;
}
public Matchers withCatalogs(Collection values) {
if (values!= null) {
getCatalogs().addAll(values);
}
return this;
}
public Matchers withCatalogs(List catalogs) {
setCatalogs(catalogs);
return this;
}
public Matchers withSchemas(MatchersSchemaType... values) {
if (values!= null) {
for (MatchersSchemaType value: values) {
getSchemas().add(value);
}
}
return this;
}
public Matchers withSchemas(Collection values) {
if (values!= null) {
getSchemas().addAll(values);
}
return this;
}
public Matchers withSchemas(List schemas) {
setSchemas(schemas);
return this;
}
public Matchers withTables(MatchersTableType... values) {
if (values!= null) {
for (MatchersTableType value: values) {
getTables().add(value);
}
}
return this;
}
public Matchers withTables(Collection values) {
if (values!= null) {
getTables().addAll(values);
}
return this;
}
public Matchers withTables(List tables) {
setTables(tables);
return this;
}
public Matchers withIndexes(MatchersIndexType... values) {
if (values!= null) {
for (MatchersIndexType value: values) {
getIndexes().add(value);
}
}
return this;
}
public Matchers withIndexes(Collection values) {
if (values!= null) {
getIndexes().addAll(values);
}
return this;
}
public Matchers withIndexes(List indexes) {
setIndexes(indexes);
return this;
}
public Matchers withPrimaryKeys(MatchersPrimaryKeyType... values) {
if (values!= null) {
for (MatchersPrimaryKeyType value: values) {
getPrimaryKeys().add(value);
}
}
return this;
}
public Matchers withPrimaryKeys(Collection values) {
if (values!= null) {
getPrimaryKeys().addAll(values);
}
return this;
}
public Matchers withPrimaryKeys(List primaryKeys) {
setPrimaryKeys(primaryKeys);
return this;
}
public Matchers withUniqueKeys(MatchersUniqueKeyType... values) {
if (values!= null) {
for (MatchersUniqueKeyType value: values) {
getUniqueKeys().add(value);
}
}
return this;
}
public Matchers withUniqueKeys(Collection values) {
if (values!= null) {
getUniqueKeys().addAll(values);
}
return this;
}
public Matchers withUniqueKeys(List uniqueKeys) {
setUniqueKeys(uniqueKeys);
return this;
}
public Matchers withForeignKeys(MatchersForeignKeyType... values) {
if (values!= null) {
for (MatchersForeignKeyType value: values) {
getForeignKeys().add(value);
}
}
return this;
}
public Matchers withForeignKeys(Collection values) {
if (values!= null) {
getForeignKeys().addAll(values);
}
return this;
}
public Matchers withForeignKeys(List foreignKeys) {
setForeignKeys(foreignKeys);
return this;
}
public Matchers withFields(MatchersFieldType... values) {
if (values!= null) {
for (MatchersFieldType value: values) {
getFields().add(value);
}
}
return this;
}
public Matchers withFields(Collection values) {
if (values!= null) {
getFields().addAll(values);
}
return this;
}
public Matchers withFields(List fields) {
setFields(fields);
return this;
}
public Matchers withRoutines(MatchersRoutineType... values) {
if (values!= null) {
for (MatchersRoutineType value: values) {
getRoutines().add(value);
}
}
return this;
}
public Matchers withRoutines(Collection values) {
if (values!= null) {
getRoutines().addAll(values);
}
return this;
}
public Matchers withRoutines(List routines) {
setRoutines(routines);
return this;
}
public Matchers withSequences(MatchersSequenceType... values) {
if (values!= null) {
for (MatchersSequenceType value: values) {
getSequences().add(value);
}
}
return this;
}
public Matchers withSequences(Collection values) {
if (values!= null) {
getSequences().addAll(values);
}
return this;
}
public Matchers withSequences(List sequences) {
setSequences(sequences);
return this;
}
public Matchers withEnums(MatchersEnumType... values) {
if (values!= null) {
for (MatchersEnumType value: values) {
getEnums().add(value);
}
}
return this;
}
public Matchers withEnums(Collection values) {
if (values!= null) {
getEnums().addAll(values);
}
return this;
}
public Matchers withEnums(List enums) {
setEnums(enums);
return this;
}
public Matchers withEmbeddables(MatchersEmbeddableType... values) {
if (values!= null) {
for (MatchersEmbeddableType value: values) {
getEmbeddables().add(value);
}
}
return this;
}
public Matchers withEmbeddables(Collection values) {
if (values!= null) {
getEmbeddables().addAll(values);
}
return this;
}
public Matchers withEmbeddables(List embeddables) {
setEmbeddables(embeddables);
return this;
}
public Matchers withUdts(MatchersUDTType... values) {
if (values!= null) {
for (MatchersUDTType value: values) {
getUdts().add(value);
}
}
return this;
}
public Matchers withUdts(Collection values) {
if (values!= null) {
getUdts().addAll(values);
}
return this;
}
public Matchers withUdts(List udts) {
setUdts(udts);
return this;
}
public Matchers withAttributes(MatchersAttributeType... values) {
if (values!= null) {
for (MatchersAttributeType value: values) {
getAttributes().add(value);
}
}
return this;
}
public Matchers withAttributes(Collection values) {
if (values!= null) {
getAttributes().addAll(values);
}
return this;
}
public Matchers withAttributes(List attributes) {
setAttributes(attributes);
return this;
}
@Override
public final void appendTo(XMLBuilder builder) {
builder.append("catalogs", "catalog", catalogs);
builder.append("schemas", "schema", schemas);
builder.append("tables", "table", tables);
builder.append("indexes", "index", indexes);
builder.append("primaryKeys", "primaryKey", primaryKeys);
builder.append("uniqueKeys", "uniqueKey", uniqueKeys);
builder.append("foreignKeys", "foreignKey", foreignKeys);
builder.append("fields", "field", fields);
builder.append("routines", "routine", routines);
builder.append("sequences", "sequence", sequences);
builder.append("enums", "enum", enums);
builder.append("embeddables", "embeddable", embeddables);
builder.append("udts", "udt", udts);
builder.append("attributes", "attribute", attributes);
}
@Override
public String toString() {
XMLBuilder builder = XMLBuilder.nonFormatting();
appendTo(builder);
return builder.toString();
}
@Override
public boolean equals(Object that) {
if (this == that) {
return true;
}
if (that == null) {
return false;
}
if (getClass()!= that.getClass()) {
return false;
}
Matchers other = ((Matchers) that);
if (catalogs == null) {
if (other.catalogs!= null) {
return false;
}
} else {
if (!catalogs.equals(other.catalogs)) {
return false;
}
}
if (schemas == null) {
if (other.schemas!= null) {
return false;
}
} else {
if (!schemas.equals(other.schemas)) {
return false;
}
}
if (tables == null) {
if (other.tables!= null) {
return false;
}
} else {
if (!tables.equals(other.tables)) {
return false;
}
}
if (indexes == null) {
if (other.indexes!= null) {
return false;
}
} else {
if (!indexes.equals(other.indexes)) {
return false;
}
}
if (primaryKeys == null) {
if (other.primaryKeys!= null) {
return false;
}
} else {
if (!primaryKeys.equals(other.primaryKeys)) {
return false;
}
}
if (uniqueKeys == null) {
if (other.uniqueKeys!= null) {
return false;
}
} else {
if (!uniqueKeys.equals(other.uniqueKeys)) {
return false;
}
}
if (foreignKeys == null) {
if (other.foreignKeys!= null) {
return false;
}
} else {
if (!foreignKeys.equals(other.foreignKeys)) {
return false;
}
}
if (fields == null) {
if (other.fields!= null) {
return false;
}
} else {
if (!fields.equals(other.fields)) {
return false;
}
}
if (routines == null) {
if (other.routines!= null) {
return false;
}
} else {
if (!routines.equals(other.routines)) {
return false;
}
}
if (sequences == null) {
if (other.sequences!= null) {
return false;
}
} else {
if (!sequences.equals(other.sequences)) {
return false;
}
}
if (enums == null) {
if (other.enums!= null) {
return false;
}
} else {
if (!enums.equals(other.enums)) {
return false;
}
}
if (embeddables == null) {
if (other.embeddables!= null) {
return false;
}
} else {
if (!embeddables.equals(other.embeddables)) {
return false;
}
}
if (udts == null) {
if (other.udts!= null) {
return false;
}
} else {
if (!udts.equals(other.udts)) {
return false;
}
}
if (attributes == null) {
if (other.attributes!= null) {
return false;
}
} else {
if (!attributes.equals(other.attributes)) {
return false;
}
}
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = ((prime*result)+((catalogs == null)? 0 :catalogs.hashCode()));
result = ((prime*result)+((schemas == null)? 0 :schemas.hashCode()));
result = ((prime*result)+((tables == null)? 0 :tables.hashCode()));
result = ((prime*result)+((indexes == null)? 0 :indexes.hashCode()));
result = ((prime*result)+((primaryKeys == null)? 0 :primaryKeys.hashCode()));
result = ((prime*result)+((uniqueKeys == null)? 0 :uniqueKeys.hashCode()));
result = ((prime*result)+((foreignKeys == null)? 0 :foreignKeys.hashCode()));
result = ((prime*result)+((fields == null)? 0 :fields.hashCode()));
result = ((prime*result)+((routines == null)? 0 :routines.hashCode()));
result = ((prime*result)+((sequences == null)? 0 :sequences.hashCode()));
result = ((prime*result)+((enums == null)? 0 :enums.hashCode()));
result = ((prime*result)+((embeddables == null)? 0 :embeddables.hashCode()));
result = ((prime*result)+((udts == null)? 0 :udts.hashCode()));
result = ((prime*result)+((attributes == null)? 0 :attributes.hashCode()));
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy