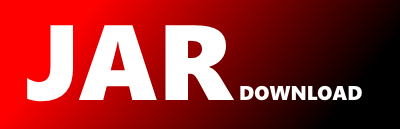
org.jooq.util.mysql.mysql.tables.Proc Maven / Gradle / Ivy
/**
* This class is generated by jOOQ
*/
package org.jooq.util.mysql.mysql.tables;
import java.sql.Timestamp;
import javax.annotation.Generated;
import org.jooq.Field;
import org.jooq.Record;
import org.jooq.Schema;
import org.jooq.Table;
import org.jooq.TableField;
import org.jooq.impl.TableImpl;
import org.jooq.util.mysql.mysql.Mysql;
import org.jooq.util.mysql.mysql.enums.ProcIsDeterministic;
import org.jooq.util.mysql.mysql.enums.ProcLanguage;
import org.jooq.util.mysql.mysql.enums.ProcSecurityType;
import org.jooq.util.mysql.mysql.enums.ProcSqlDataAccess;
import org.jooq.util.mysql.mysql.enums.ProcType;
/**
* Stored Procedures
*/
@Generated(
value = {
"http://www.jooq.org",
"jOOQ version:3.9.0"
},
comments = "This class is generated by jOOQ"
)
@SuppressWarnings({ "all", "unchecked", "rawtypes" })
public class Proc extends TableImpl {
private static final long serialVersionUID = -765641597;
/**
* The reference instance of mysql.proc
*/
public static final Proc PROC = new Proc();
/**
* The class holding records for this type
*/
@Override
public Class getRecordType() {
return Record.class;
}
/**
* The column mysql.proc.db
.
*/
public static final TableField DB = createField("db", org.jooq.impl.SQLDataType.CHAR.length(64).nullable(false).defaultValue(org.jooq.impl.DSL.inline("", org.jooq.impl.SQLDataType.CHAR)), PROC, "");
/**
* The column mysql.proc.name
.
*/
public static final TableField NAME = createField("name", org.jooq.impl.SQLDataType.CHAR.length(64).nullable(false).defaultValue(org.jooq.impl.DSL.inline("", org.jooq.impl.SQLDataType.CHAR)), PROC, "");
/**
* The column mysql.proc.type
.
*/
public static final TableField TYPE = createField("type", org.jooq.util.mysql.MySQLDataType.VARCHAR.asEnumDataType(org.jooq.util.mysql.mysql.enums.ProcType.class), PROC, "");
/**
* The column mysql.proc.specific_name
.
*/
public static final TableField SPECIFIC_NAME = createField("specific_name", org.jooq.impl.SQLDataType.CHAR.length(64).nullable(false).defaultValue(org.jooq.impl.DSL.inline("", org.jooq.impl.SQLDataType.CHAR)), PROC, "");
/**
* The column mysql.proc.language
.
*/
public static final TableField LANGUAGE = createField("language", org.jooq.util.mysql.MySQLDataType.VARCHAR.asEnumDataType(org.jooq.util.mysql.mysql.enums.ProcLanguage.class), PROC, "");
/**
* The column mysql.proc.sql_data_access
.
*/
public static final TableField SQL_DATA_ACCESS = createField("sql_data_access", org.jooq.util.mysql.MySQLDataType.VARCHAR.asEnumDataType(org.jooq.util.mysql.mysql.enums.ProcSqlDataAccess.class), PROC, "");
/**
* The column mysql.proc.is_deterministic
.
*/
public static final TableField IS_DETERMINISTIC = createField("is_deterministic", org.jooq.util.mysql.MySQLDataType.VARCHAR.asEnumDataType(org.jooq.util.mysql.mysql.enums.ProcIsDeterministic.class), PROC, "");
/**
* The column mysql.proc.security_type
.
*/
public static final TableField SECURITY_TYPE = createField("security_type", org.jooq.util.mysql.MySQLDataType.VARCHAR.asEnumDataType(org.jooq.util.mysql.mysql.enums.ProcSecurityType.class), PROC, "");
/**
* The column mysql.proc.param_list
.
*/
public static final TableField PARAM_LIST = createField("param_list", org.jooq.impl.SQLDataType.BLOB.nullable(false), PROC, "");
/**
* The column mysql.proc.returns
.
*/
public static final TableField RETURNS = createField("returns", org.jooq.impl.SQLDataType.BLOB.nullable(false), PROC, "");
/**
* The column mysql.proc.body
.
*/
public static final TableField BODY = createField("body", org.jooq.impl.SQLDataType.BLOB.nullable(false), PROC, "");
/**
* The column mysql.proc.definer
.
*/
public static final TableField DEFINER = createField("definer", org.jooq.impl.SQLDataType.CHAR.length(77).nullable(false).defaultValue(org.jooq.impl.DSL.inline("", org.jooq.impl.SQLDataType.CHAR)), PROC, "");
/**
* The column mysql.proc.created
.
*/
public static final TableField CREATED = createField("created", org.jooq.impl.SQLDataType.TIMESTAMP.nullable(false).defaultValue(org.jooq.impl.DSL.inline("CURRENT_TIMESTAMP", org.jooq.impl.SQLDataType.TIMESTAMP)), PROC, "");
/**
* The column mysql.proc.modified
.
*/
public static final TableField MODIFIED = createField("modified", org.jooq.impl.SQLDataType.TIMESTAMP.nullable(false).defaultValue(org.jooq.impl.DSL.inline("0000-00-00 00:00:00", org.jooq.impl.SQLDataType.TIMESTAMP)), PROC, "");
/**
* The column mysql.proc.sql_mode
.
*/
public static final TableField SQL_MODE = createField("sql_mode", org.jooq.impl.SQLDataType.VARCHAR.length(478).nullable(false).defaultValue(org.jooq.impl.DSL.inline("", org.jooq.impl.SQLDataType.VARCHAR)), PROC, "");
/**
* The column mysql.proc.comment
.
*/
public static final TableField COMMENT = createField("comment", org.jooq.impl.SQLDataType.CLOB.nullable(false), PROC, "");
/**
* The column mysql.proc.character_set_client
.
*/
public static final TableField CHARACTER_SET_CLIENT = createField("character_set_client", org.jooq.impl.SQLDataType.CHAR.length(32), PROC, "");
/**
* The column mysql.proc.collation_connection
.
*/
public static final TableField COLLATION_CONNECTION = createField("collation_connection", org.jooq.impl.SQLDataType.CHAR.length(32), PROC, "");
/**
* The column mysql.proc.db_collation
.
*/
public static final TableField DB_COLLATION = createField("db_collation", org.jooq.impl.SQLDataType.CHAR.length(32), PROC, "");
/**
* The column mysql.proc.body_utf8
.
*/
public static final TableField BODY_UTF8 = createField("body_utf8", org.jooq.impl.SQLDataType.BLOB, PROC, "");
/**
* No further instances allowed
*/
private Proc() {
this("proc", null);
}
private Proc(String alias, Table aliased) {
this(alias, aliased, null);
}
private Proc(String alias, Table aliased, Field>[] parameters) {
super(alias, null, aliased, parameters, "Stored Procedures");
}
/**
* {@inheritDoc}
*/
@Override
public Schema getSchema() {
return Mysql.MYSQL;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy