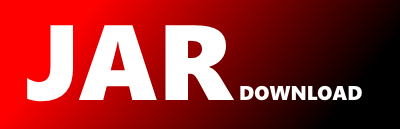
org.jooq.Functions Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses for this work are available. These replace the above
* Apache-2.0 license and offer limited warranties, support, maintenance, and
* commercial database integrations.
*
* For more information, please visit: http://www.jooq.org/licenses
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*/
package org.jooq;
import org.jetbrains.annotations.NotNull;
/**
* Utilities related to the construction of functions.
*
* @author Lukas Eder
*/
public final class Functions {
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function1 nullOnAllNull(Function1 super T1, ? extends R> function) {
return (t1) -> t1 == null ? null : function.apply(t1);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function2 nullOnAllNull(Function2 super T1, ? super T2, ? extends R> function) {
return (t1, t2) -> t1 == null && t2 == null ? null : function.apply(t1, t2);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function3 nullOnAllNull(Function3 super T1, ? super T2, ? super T3, ? extends R> function) {
return (t1, t2, t3) -> t1 == null && t2 == null && t3 == null ? null : function.apply(t1, t2, t3);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function4 nullOnAllNull(Function4 super T1, ? super T2, ? super T3, ? super T4, ? extends R> function) {
return (t1, t2, t3, t4) -> t1 == null && t2 == null && t3 == null && t4 == null ? null : function.apply(t1, t2, t3, t4);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function5 nullOnAllNull(Function5 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? extends R> function) {
return (t1, t2, t3, t4, t5) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null ? null : function.apply(t1, t2, t3, t4, t5);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function6 nullOnAllNull(Function6 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null ? null : function.apply(t1, t2, t3, t4, t5, t6);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function7 nullOnAllNull(Function7 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function8 nullOnAllNull(Function8 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function9 nullOnAllNull(Function9 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function10 nullOnAllNull(Function10 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function11 nullOnAllNull(Function11 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function12 nullOnAllNull(Function12 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function13 nullOnAllNull(Function13 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function14 nullOnAllNull(Function14 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function15 nullOnAllNull(Function15 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function16 nullOnAllNull(Function16 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null && t16 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function17 nullOnAllNull(Function17 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null && t16 == null && t17 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function18 nullOnAllNull(Function18 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null && t16 == null && t17 == null && t18 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function19 nullOnAllNull(Function19 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null && t16 == null && t17 == null && t18 == null && t19 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function20 nullOnAllNull(Function20 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? super T20, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null && t16 == null && t17 == null && t18 == null && t19 == null && t20 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function21 nullOnAllNull(Function21 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? super T20, ? super T21, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null && t16 == null && t17 == null && t18 == null && t19 == null && t20 == null && t21 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21);
}
/**
* A function that short circuits the argument function returning null
* if all arguments are null
.
*/
@NotNull
public static final Function22 nullOnAllNull(Function22 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? super T20, ? super T21, ? super T22, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21, t22) -> t1 == null && t2 == null && t3 == null && t4 == null && t5 == null && t6 == null && t7 == null && t8 == null && t9 == null && t10 == null && t11 == null && t12 == null && t13 == null && t14 == null && t15 == null && t16 == null && t17 == null && t18 == null && t19 == null && t20 == null && t21 == null && t22 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21, t22);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function1 nullOnAnyNull(Function1 super T1, ? extends R> function) {
return (t1) -> t1 == null ? null : function.apply(t1);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function2 nullOnAnyNull(Function2 super T1, ? super T2, ? extends R> function) {
return (t1, t2) -> t1 == null || t2 == null ? null : function.apply(t1, t2);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function3 nullOnAnyNull(Function3 super T1, ? super T2, ? super T3, ? extends R> function) {
return (t1, t2, t3) -> t1 == null || t2 == null || t3 == null ? null : function.apply(t1, t2, t3);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function4 nullOnAnyNull(Function4 super T1, ? super T2, ? super T3, ? super T4, ? extends R> function) {
return (t1, t2, t3, t4) -> t1 == null || t2 == null || t3 == null || t4 == null ? null : function.apply(t1, t2, t3, t4);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function5 nullOnAnyNull(Function5 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? extends R> function) {
return (t1, t2, t3, t4, t5) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null ? null : function.apply(t1, t2, t3, t4, t5);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function6 nullOnAnyNull(Function6 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null ? null : function.apply(t1, t2, t3, t4, t5, t6);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function7 nullOnAnyNull(Function7 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function8 nullOnAnyNull(Function8 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function9 nullOnAnyNull(Function9 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function10 nullOnAnyNull(Function10 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function11 nullOnAnyNull(Function11 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function12 nullOnAnyNull(Function12 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function13 nullOnAnyNull(Function13 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function14 nullOnAnyNull(Function14 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function15 nullOnAnyNull(Function15 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function16 nullOnAnyNull(Function16 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null || t16 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function17 nullOnAnyNull(Function17 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null || t16 == null || t17 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function18 nullOnAnyNull(Function18 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null || t16 == null || t17 == null || t18 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function19 nullOnAnyNull(Function19 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null || t16 == null || t17 == null || t18 == null || t19 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function20 nullOnAnyNull(Function20 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? super T20, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null || t16 == null || t17 == null || t18 == null || t19 == null || t20 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function21 nullOnAnyNull(Function21 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? super T20, ? super T21, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null || t16 == null || t17 == null || t18 == null || t19 == null || t20 == null || t21 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21);
}
/**
* A function that short circuits the argument function returning null
* if any argument is null
.
*/
@NotNull
public static final Function22 nullOnAnyNull(Function22 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? super T10, ? super T11, ? super T12, ? super T13, ? super T14, ? super T15, ? super T16, ? super T17, ? super T18, ? super T19, ? super T20, ? super T21, ? super T22, ? extends R> function) {
return (t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21, t22) -> t1 == null || t2 == null || t3 == null || t4 == null || t5 == null || t6 == null || t7 == null || t8 == null || t9 == null || t10 == null || t11 == null || t12 == null || t13 == null || t14 == null || t15 == null || t16 == null || t17 == null || t18 == null || t19 == null || t20 == null || t21 == null || t22 == null ? null : function.apply(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21, t22);
}
private Functions() {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy