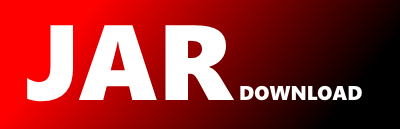
org.jooq.tools.Convert Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses for this work are available. These replace the above
* Apache-2.0 license and offer limited warranties, support, maintenance, and
* commercial database integrations.
*
* For more information, please visit: http://www.jooq.org/licenses
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*/
package org.jooq.tools;
import java.io.File;
import java.net.URI;
import java.net.URL;
import java.nio.ByteBuffer;
import java.sql.Date;
import java.sql.Time;
import java.sql.Timestamp;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.time.temporal.Temporal;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import org.jooq.Converter;
import org.jooq.ConverterProvider;
import org.jooq.DataType;
import org.jooq.Field;
import org.jooq.exception.DataTypeException;
import org.jooq.impl.Internal;
/**
* Utility methods for type conversions
*
* This class provides less type-safety than the general jOOQ API methods. For
* instance, it accepts arbitrary {@link Converter} objects in methods like
* {@link #convert(Collection, Converter)} and {@link #convert(Object, Class)},
* trying to retrofit the Object
argument to the type provided in
* {@link Converter#fromType()} before performing the actual conversion.
*
* @author Lukas Eder
* @deprecated - 3.15.0 - [#11898] This class will be removed in the future. Do
* not reuse. Data type conversion happens using internal
* {@link Converter} implementations, or {@link ConverterProvider}
* provided ones, or implementations attached to fields via
* generated code, or via {@link Field#convert(Converter)}, or
* {@link DataType#asConvertedDataType(Converter)}.
*/
@Deprecated(forRemoval = true, since = "3.15")
public final class Convert {
/**
* All string values that can be transformed into a boolean true
value.
*/
public static final Set TRUE_VALUES;
/**
* All string values that can be transformed into a boolean false
value.
*/
public static final Set FALSE_VALUES;
static {
Set trueValues = new HashSet<>();
Set falseValues = new HashSet<>();
trueValues.add("1");
trueValues.add("1.0");
trueValues.add("y");
trueValues.add("Y");
trueValues.add("yes");
trueValues.add("YES");
trueValues.add("true");
trueValues.add("TRUE");
trueValues.add("t");
trueValues.add("T");
trueValues.add("on");
trueValues.add("ON");
trueValues.add("enabled");
trueValues.add("ENABLED");
falseValues.add("0");
falseValues.add("0.0");
falseValues.add("n");
falseValues.add("N");
falseValues.add("no");
falseValues.add("NO");
falseValues.add("false");
falseValues.add("FALSE");
falseValues.add("f");
falseValues.add("F");
falseValues.add("off");
falseValues.add("OFF");
falseValues.add("disabled");
falseValues.add("DISABLED");
TRUE_VALUES = Collections.unmodifiableSet(trueValues);
FALSE_VALUES = Collections.unmodifiableSet(falseValues);
}
/**
* Convert an array of values to a matching data type
*
* This converts values[i]
to fields[i].getType()
*/
public static final Object[] convert(Object[] values, Field>[] fields) {
return Internal.convert(values, fields);
}
/**
* Convert an array of values to a matching data type
*
* This converts values[i]
to types[i]
*/
public static final Object[] convert(Object[] values, Class>[] types) {
return Internal.convert(values, types);
}
/**
* Convert an array into another one using a converter
*
* This uses {@link #convertArray(Object[], Class)} to convert the array to
* an array of {@link Converter#fromType()} first, before converting that
* array again to {@link Converter#toType()}
*
* @param from The array to convert
* @param converter The data type converter
* @return A converted array
* @throws DataTypeException - When the conversion is not possible
*/
public static final U[] convertArray(Object[] from, Converter, ? extends U> converter) throws DataTypeException {
return Internal.convertArray(from, converter);
}
/**
* Convert an array into another one by these rules
*
*
* - If
toClass
is not an array class, then make it an array
* class first
* - If
toClass
is an array class, then create an instance
* from it, and convert all elements in the from
array one by
* one, using {@link #convert(Object, Class)}
*
*
* @param from The array to convert
* @param toClass The target array type
* @return A converted array
* @throws DataTypeException - When the conversion is not possible
*/
public static final Object[] convertArray(Object[] from, Class> toClass) throws DataTypeException {
return Internal.convertArray(from, toClass);
}
public static final U[] convertCollection(Collection from, Class extends U[]> to){
return Internal.convertCollection(from, to);
}
/**
* Convert an object to a type.
*
* @param from The source object
* @param converter The data type converter
* @return The target type object
* @throws DataTypeException - When the conversion is not possible
*/
public static final U convert(Object from, Converter, ? extends U> converter) throws DataTypeException {
return Internal.convert(from, converter);
}
/**
* Convert an object to a type. These are the conversion rules:
*
* null
is always converted to null
, or the
* primitive default value, or {@link Optional#empty()}, regardless of the
* target type.
* - Identity conversion (converting a value to its own type) is always
* possible.
* - Primitive types can be converted to their wrapper types and vice
* versa
* - All types can be converted to
String
* - All types can be converted to
Object
* - All
Number
types can be converted to other
* Number
types
* - All
Number
or String
types can be converted
* to Boolean
. Possible (case-insensitive) values for
* true
:
*
* 1
* 1.0
* y
* yes
* true
* on
* enabled
*
*
* Possible (case-insensitive) values for false
:
*
* 0
* 0.0
* n
* no
* false
* off
* disabled
*
*
* All other values evaluate to null
* - All {@link java.util.Date} subtypes ({@link Date}, {@link Time},
* {@link Timestamp}), as well as most {@link Temporal} subtypes (
* {@link LocalDate}, {@link LocalTime}, {@link LocalDateTime},
* {@link OffsetTime}, {@link OffsetDateTime}, as well as {@link Instant})
* can be converted into each other.
* - All
String
types can be converted into {@link URI},
* {@link URL} and {@link File}
* byte[]
and {@link ByteBuffer} can be converted into one
* another
* byte[]
can be converted into String
, using
* the platform's default charset
* Object[]
can be converted into any other array type, if
* array elements can be converted, too
* - All types can be converted into types containing a single argument
* constructor whose argument is a type that can be converted to according
* to the above rules.
* - All other combinations that are not listed above will result in a
* {@link DataTypeException}
*
*
* @param from The object to convert
* @param toClass The target type
* @return The converted object
* @throws DataTypeException - When the conversion is not possible
*/
public static final T convert(Object from, Class extends T> toClass) throws DataTypeException {
return Internal.convert(from, toClass);
}
/**
* Convert a collection of objects to a list of T
, using
* {@link #convert(Object, Class)}
*
* @param collection The list of objects
* @param type The target type
* @return The list of converted objects
* @throws DataTypeException - When the conversion is not possible
* @see #convert(Object, Class)
*/
public static final List convert(Collection> collection, Class extends T> type) throws DataTypeException {
return Internal.convert(collection, type);
}
/**
* Convert a collection of objects to a list of T
, using
* {@link #convert(Object, Converter)}
*
* @param collection The collection of objects
* @param converter The data type converter
* @return The list of converted objects
* @throws DataTypeException - When the conversion is not possible
* @see #convert(Object, Converter)
*/
public static final List convert(Collection> collection, Converter, ? extends U> converter) throws DataTypeException {
return Internal.convert(collection, converter);
}
}