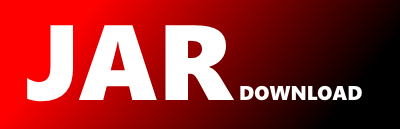
org.jooq.Rows Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Other licenses:
* -----------------------------------------------------------------------------
* Commercial licenses for this work are available. These replace the above
* Apache-2.0 and offer limited warranties, support, maintenance, and commercial
* database integrations.
*
* For more information, please visit: https://www.jooq.org/legal/licensing
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*/
package org.jooq;
import java.util.ArrayList;
import java.util.List;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.stream.Collector;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.jooq.impl.DSL;
/**
* An auxiliary class for constructing {@link Row} collections.
*
* The current implementation is in draft stage. It may be changed incompatibly
* in the future. Use at your own risk.
*
* @author Dmitry Baev
* @author Lukas Eder
*/
public final class Rows {
/**
* Create a collector that can collect into an array of {@link RowN}.
*/
@SafeVarargs
public static final Collector toRowArray(
Function super T, ? extends Field>>... functions
) {
return Collectors.collectingAndThen(toRowList(functions), l -> l.toArray(new RowN[0]));
}
/**
* Create a collector that can collect into a list of {@link RowN}.
*/
@SafeVarargs
public static final Collector> toRowList(
Function super T, ? extends Field>>... functions
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(Stream.of(functions).map(f -> f.apply(t)).toArray())),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row1}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1
) {
return Collectors.collectingAndThen(toRowList(f1), l -> l.toArray(new Row1[0]));
}
/**
* Create a collector that can collect into a list of {@link Row1}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row2}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2
) {
return Collectors.collectingAndThen(toRowList(f1, f2), l -> l.toArray(new Row2[0]));
}
/**
* Create a collector that can collect into a list of {@link Row2}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row3}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3), l -> l.toArray(new Row3[0]));
}
/**
* Create a collector that can collect into a list of {@link Row3}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row4}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4), l -> l.toArray(new Row4[0]));
}
/**
* Create a collector that can collect into a list of {@link Row4}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row5}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5), l -> l.toArray(new Row5[0]));
}
/**
* Create a collector that can collect into a list of {@link Row5}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row6}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6), l -> l.toArray(new Row6[0]));
}
/**
* Create a collector that can collect into a list of {@link Row6}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row7}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6, f7), l -> l.toArray(new Row7[0]));
}
/**
* Create a collector that can collect into a list of {@link Row7}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t), f7.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row8}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6, f7, f8), l -> l.toArray(new Row8[0]));
}
/**
* Create a collector that can collect into a list of {@link Row8}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t), f7.apply(t), f8.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row9}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6, f7, f8, f9), l -> l.toArray(new Row9[0]));
}
/**
* Create a collector that can collect into a list of {@link Row9}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t), f7.apply(t), f8.apply(t), f9.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row10}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6, f7, f8, f9, f10), l -> l.toArray(new Row10[0]));
}
/**
* Create a collector that can collect into a list of {@link Row10}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t), f7.apply(t), f8.apply(t), f9.apply(t), f10.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row11}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10,
Function super T, ? extends Field> f11
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6, f7, f8, f9, f10, f11), l -> l.toArray(new Row11[0]));
}
/**
* Create a collector that can collect into a list of {@link Row11}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10,
Function super T, ? extends Field> f11
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t), f7.apply(t), f8.apply(t), f9.apply(t), f10.apply(t), f11.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row12}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10,
Function super T, ? extends Field> f11,
Function super T, ? extends Field> f12
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6, f7, f8, f9, f10, f11, f12), l -> l.toArray(new Row12[0]));
}
/**
* Create a collector that can collect into a list of {@link Row12}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10,
Function super T, ? extends Field> f11,
Function super T, ? extends Field> f12
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t), f7.apply(t), f8.apply(t), f9.apply(t), f10.apply(t), f11.apply(t), f12.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row13}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10,
Function super T, ? extends Field> f11,
Function super T, ? extends Field> f12,
Function super T, ? extends Field> f13
) {
return Collectors.collectingAndThen(toRowList(f1, f2, f3, f4, f5, f6, f7, f8, f9, f10, f11, f12, f13), l -> l.toArray(new Row13[0]));
}
/**
* Create a collector that can collect into a list of {@link Row13}.
*/
public static final Collector>> toRowList(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field> f10,
Function super T, ? extends Field> f11,
Function super T, ? extends Field> f12,
Function super T, ? extends Field> f13
) {
return Collector.of(
ArrayList::new,
(l, t) -> l.add(DSL.row(f1.apply(t), f2.apply(t), f3.apply(t), f4.apply(t), f5.apply(t), f6.apply(t), f7.apply(t), f8.apply(t), f9.apply(t), f10.apply(t), f11.apply(t), f12.apply(t), f13.apply(t))),
listCombiner()
);
}
/**
* Create a collector that can collect into an array of {@link Row14}.
*/
@SuppressWarnings("unchecked")
public static final Collector[]> toRowArray(
Function super T, ? extends Field> f1,
Function super T, ? extends Field> f2,
Function super T, ? extends Field> f3,
Function super T, ? extends Field> f4,
Function super T, ? extends Field> f5,
Function super T, ? extends Field> f6,
Function super T, ? extends Field> f7,
Function super T, ? extends Field> f8,
Function super T, ? extends Field> f9,
Function super T, ? extends Field