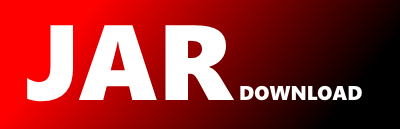
org.joou.Unsigned Maven / Gradle / Ivy
Show all versions of joou-java-6 Show documentation
/*
* Copyright (c) Data Geekery GmbH (http://www.datageekery.com)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.joou;
import java.math.BigInteger;
/**
* A utility class for static access to unsigned number functionality.
*
* It essentially contains factory methods for unsigned number wrappers. In
* future versions, it will also contain some arithmetic methods, handling
* regular arithmetic and bitwise operations
*
* @author Lukas Eder
*/
public final class Unsigned {
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned byte
.
* @see UByte#valueOf(String)
*/
public static UByte ubyte(String value) throws NumberFormatException {
return value == null ? null : UByte.valueOf(value);
}
/**
* Create an unsigned byte
by masking it with 0xFF
* i.e. (byte) -1
becomes (ubyte) 255
*
* @see UByte#valueOf(byte)
*/
public static UByte ubyte(byte value) {
return UByte.valueOf(value);
}
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
* @see UByte#valueOf(short)
*/
public static UByte ubyte(short value) throws NumberFormatException {
return UByte.valueOf(value);
}
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
* @see UByte#valueOf(short)
*/
public static UByte ubyte(int value) throws NumberFormatException {
return UByte.valueOf(value);
}
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
* @see UByte#valueOf(short)
*/
public static UByte ubyte(long value) throws NumberFormatException {
return UByte.valueOf(value);
}
/**
* Create an unsigned short
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned short
.
* @see UShort#valueOf(String)
*/
public static UShort ushort(String value) throws NumberFormatException {
return value == null ? null : UShort.valueOf(value);
}
/**
* Create an unsigned short
by masking it with
* 0xFFFF
i.e. (short) -1
becomes
* (ushort) 65535
*
* @see UShort#valueOf(short)
*/
public static UShort ushort(short value) {
return UShort.valueOf(value);
}
/**
* Create an unsigned short
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned short
* @see UShort#valueOf(int)
*/
public static UShort ushort(int value) throws NumberFormatException {
return UShort.valueOf(value);
}
/**
* Create an unsigned int
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned int
.
* @see UInteger#valueOf(String)
*/
public static UInteger uint(String value) throws NumberFormatException {
return value == null ? null : UInteger.valueOf(value);
}
/**
* Create an unsigned int
by masking it with
* 0xFFFFFFFF
i.e. (int) -1
becomes
* (uint) 4294967295
*
* @see UInteger#valueOf(int)
*/
public static UInteger uint(int value) {
return UInteger.valueOf(value);
}
/**
* Create an unsigned int
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned int
* @see UInteger#valueOf(long)
*/
public static UInteger uint(long value) throws NumberFormatException {
return UInteger.valueOf(value);
}
/**
* Create an unsigned long
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned long
.
* @see ULong#valueOf(String)
*/
public static ULong ulong(String value) throws NumberFormatException {
return value == null ? null : ULong.valueOf(value);
}
/**
* Create an unsigned long
by masking it with
* 0xFFFFFFFFFFFFFFFF
i.e. (long) -1
becomes
* (uint) 18446744073709551615
*
* @see ULong#valueOf(long)
*/
public static ULong ulong(long value) {
return ULong.valueOf(value);
}
/**
* Create an unsigned long
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned long
* @see ULong#valueOf(BigInteger)
*/
public static ULong ulong(BigInteger value) throws NumberFormatException {
return ULong.valueOf(value);
}
/**
* No instances
*/
private Unsigned() {}
}