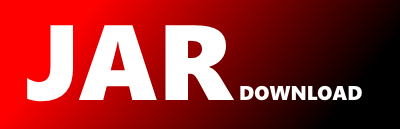
org.jorigin.gui.JDesktopPane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcommon Show documentation
Show all versions of jcommon Show documentation
A java common package that enable to deal with various functionalities
/*
This file is part of JOrigin Common Library.
JOrigin Common is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
JOrigin Common is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JOrigin Common. If not, see .
*/
package org.jorigin.gui;
import java.awt.Container;
import java.awt.Dimension;
import java.beans.PropertyVetoException;
import javax.swing.JInternalFrame;
import org.jorigin.Common;
/**
* This class extends a {@link javax.swing.JDesktopPane JDesktopPane} and provide organization methods for the internal frames.
* @author Julien Seinturier - COMEX S.A. - [email protected] - https://github.com/jorigin/jeometry
* @version {@value Common#version} - b{@value Common#BUILD}
* @since 1.0.1
*/
public class JDesktopPane extends javax.swing.JDesktopPane {
private static final long serialVersionUID = Common.BUILD;
/**
* This flag represent a no organization method for the internal frames.
* @see #MOSAIC
* @see #CASCADE
*/
public static final int NONE = 0;
/**
* This flag represents a mosaic organization for the internal frames.
* @see #NONE
* @see #CASCADE
*/
public static final int MOSAIC = 1;
/**
* This flag represents a cascade organization for the internal frames.
* @see #NONE
* @see #MOSAIC
*/
public static final int CASCADE = 2;
/** No fit is applied to the added components */
public static final int FIT_NONE = 0;
/** Added component are centered */
public static final int FIT_CENTER = 1;
/**
* Added component are centered and resized to fit
* the default. The fit ratio is given by method getFrameDimensionRatio()
*/
public static final int FIT_CENTER_RESIZE = 2;
/**
* Added component are centered then switched in x and y by a delta. The delta is
* available with methods setFitDeltaMax(double delta)
and
* getFitDeltaMax
*/
public static final int FIT_CENTER_DELTA = 3;
/**
* Added component are centered, resized and switched by a delta.
* The fit ratio is given by method getFrameDimensionRatio()
.
* The delta is
* available with methods setFitDeltaMax(double delta)
and
* getFitDeltaMax
*/
public static final int FIT_CENTER_RESIZE_DELTA = 4;
/**
* The current dimension ratio used. By default this ratio is 0.5 of the
* desktop size.
*/
private double frameDimensionRatio = 0.5d;
/**
* The current fit method. By default this method is set to
* FIT_CENTER_DELTA
.
*/
private int fitMethod = FIT_CENTER_DELTA;
/**
* The fit delta max used. By default this delta is set to 50
*/
private double fitDeltaMax = 50;
/**
* The organization method used. By default this is set to NONE
.
*/
private int organizeMethod = NONE;
/**
* Create a new default desktop pane.
*/
public JDesktopPane() {
super();
}
//AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
//AA ACCESSEURS AA
//AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
/**
* Set the organization method used by the desktop to organize components.
* @param method the method to use, can be {@link #CASCADE}, {@link #MOSAIC} or {@link #NONE}.
*/
public void setOrganizeMethod(int method){
this.organizeMethod = method;
}
/**
* Get the organization method used by the desktop to organize components.
* @return the organization method.
*/
public int getOrganizeMethod(){
return this.organizeMethod;
}
/**
* Set the frame dimension ratio for internal frame. All added internal frame
* will be redimensionned to fit the ratio of descktop dimension.
* @param ratio the ratio of desktop dimension.
*/
public void setFrameDimensionRatio(double ratio){
this.frameDimensionRatio = ratio;
}
/**
* Get the frame dimension ratio for internal frame. All added internal frame
* will be redimensionned to fit the ratio of descktop dimension.
* @return ratio the ratio of desktop dimension.
*/
public double getFrameDimensionRatio(){
return this.frameDimensionRatio;
}
/**
* Set the fit method used to initialize space occupation
* of new components.
* @param fitMethod the fit method to use (FIT_CENTER, FIT_CENTER_RESIZE, ...)
*/
public void setFitMethod(int fitMethod){
this.fitMethod = fitMethod;
}
/**
* Get the fit method used to initialize space occupation
* of new components.
* @return the fit method to use (FIT_CENTER, FIT_CENTER_RESIZE, ...)
*/
public int getFitMethod(){
return this.fitMethod;
}
/**
* Set the fit delta maximum value. The delta is used when
* an organization with random delta is processed.
* @param delta the max delta value.
*/
public void setFitDeltaMax(double delta){
this.fitDeltaMax = delta;
}
/**
* Get the fit delta maximum value. The delta is used when
* an organization with random delta is processed.
* @return the max delta value.
*/
public double getFitDeltaMax(){
return this.fitDeltaMax;
}
//AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
//AA FIN ACCESSEURS AA
//AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
/**
* Organize the Internal frames following the default method
*/
public void organize(){
organize(organizeMethod);
}
/**
* Organize the Internal frames following the method given in parameter.
* {@link #MOSAIC}
* @param method the method to use (can be {@link #MOSAIC} or {@link #CASCADE})
*/
public void organize(int method){
switch(method){
case MOSAIC:
mosaic();
break;
case CASCADE:
cascade();
break;
default:
}
}
/**
* Reorganize the frames of the desktop by using cascading
*/
public void cascade(){
// Recuperation des frames du desktop
JInternalFrame[] frames = getAllFrames();
// calcul du nombre de frame internes non icones
int nbInternalFrame = frames.length;
int n = nbInternalFrame;
for( int i = 0 ; i < nbInternalFrame; ++i){
if(frames[i].isIcon()){
n--;
}
}
for(int i = 0, j = n-1 ; i 0.5d){
xDelta *= -1;
}
if (Math.random() > 0.5d){
yDelta *= -1;
}
locationx = (int) xDelta + desktopDimension.width / 2 - (frameDimension.width / 2);
locationy = (int) yDelta + desktopDimension.height / 2 - (frameDimension.height / 2);
break;
case FIT_CENTER_RESIZE_DELTA:
frameDimension = new Dimension(((int)(desktopDimension.getWidth()*0.5d)),
((int)(desktopDimension.getHeight()*0.5d)));
frame.setSize(frameDimension);
frame.setPreferredSize(frameDimension);
// Calcul d'un delta pour le positionnement
xDelta = Math.random()*this.fitDeltaMax;
yDelta = Math.random()*this.fitDeltaMax;
// Changement de signe du delta
if (Math.random() > 0.5d){
xDelta *= -1;
}
if (Math.random() > 0.5d){
yDelta *= -1;
}
locationx = (int) xDelta + desktopDimension.width / 2 - (frameDimension.width / 2);
locationy = (int) yDelta + desktopDimension.height / 2 - (frameDimension.height / 2);
break;
}
// Verification de la cohérence des tailles de fenêtre et correction
// en cas d'erreur
if (frame.getWidth() > this.getWidth()){
frameDimension.setSize(this.getWidth(), frameDimension.getHeight());
}
if (frame.getHeight() > this.getHeight()){
frameDimension.setSize(frameDimension.getWidth(), this.getHeight());
}
frame.setSize(frameDimension);
if ( (locationx >= 0) && (locationx < this.getSize().getWidth())
&& (locationy >= 0) && (locationy < this.getSize().getHeight())){
frame.setLocation(locationx, locationy);
} else {
frame.setLocation(0, 0);
}
}
/**
* Iconify all frames inb the desktop
*/
void iconifyAll(){
JInternalFrame [] frames = getAllFrames();
try{
for( int i = 0; i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy