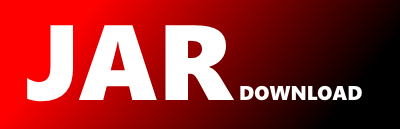
org.jpedal.display.PageOffsets Maven / Gradle / Ivy
/*
* ===========================================
* Java Pdf Extraction Decoding Access Library
* ===========================================
*
* Project Info: http://www.idrsolutions.com
* Help section for developers at http://www.idrsolutions.com/support/
*
* (C) Copyright 1997-2015 IDRsolutions and Contributors.
*
* This file is part of JPedal/JPDF2HTML5
*
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* ---------------
* PageOffsets.java
* ---------------
*/
package org.jpedal.display;
import static org.jpedal.display.Display.CONTINUOUS;
import static org.jpedal.display.Display.CONTINUOUS_FACING;
import static org.jpedal.display.Display.FACING;
import org.jpedal.objects.PdfPageData;
/**
* holds offsets for all multiple pages
*/
public class PageOffsets {
private int width,height;
/**width of all pages*/
private int totalSingleWidth,totalDoubleWidth,gaps,doubleGaps;
/**height of all pages*/
private int totalSingleHeight,totalDoubleHeight;
protected int maxW, maxH;
/**gap between pages*/
public static final int pageGap=10;
/**max widths and heights for facing and continuous pages*/
private int doublePageWidth,doublePageHeight,biggestWidth,biggestHeight,widestPageNR,widestPageR;
public PageOffsets(final int pageCount, final PdfPageData pageData) {
/** calulate sizes for continuous and facing page modes */
int pageH, pageW,rotation;
int facingW = 0, facingH = 0;
int greatestW = 0, greatestH = 0;
totalSingleHeight = 0;
totalSingleWidth = 0;
int widestLeftPage=0,widestRightPage=0,highestLeftPage=0,highestRightPage=0;
widestPageR=0;
widestPageNR=0;
totalDoubleWidth = 0;
totalDoubleHeight = 0;
gaps=0;
doubleGaps=0;
biggestWidth = 0;
biggestHeight = 0;
for (int i = 1; i < pageCount + 1; i++) {
//get page sizes
pageW = pageData.getCropBoxWidth(i);
pageH = pageData.getCropBoxHeight(i);
rotation = pageData.getRotation(i);
//swap if this page rotated and flag
if((rotation==90|| rotation==270)){
final int tmp=pageW;
pageW=pageH;
pageH=tmp;
}
if (pageW > maxW) {
maxW = pageW;
}
if (pageH > maxH) {
maxH = pageH;
}
gaps += pageGap;
totalSingleWidth += pageW;
totalSingleHeight += pageH;
//track page sizes
if(( i & 1)==1){//odd
if(widestRightPage biggestWidth) {
biggestWidth = pageW;
}
if (pageH > biggestHeight) {
biggestHeight = pageH;
}
// track widest and highest combination of facing pages
if ((i & 1) == 1) {
if (greatestW < pageW) {
greatestW = pageW;
}
if (greatestH < pageH) {
greatestH = pageH;
}
if (i == 1) {// first page special case
totalDoubleWidth = pageW;
totalDoubleHeight = pageH;
} else {
totalDoubleWidth += greatestW;
totalDoubleHeight += greatestH;
}
doubleGaps += pageGap;
facingW = pageW;
facingH = pageH;
} else {
facingW += pageW;
facingH += pageH;
greatestW = pageW;
greatestH = pageH;
if (i == pageCount) { // allow for even number of pages
totalDoubleWidth = totalDoubleWidth + greatestW + pageGap;
totalDoubleHeight = totalDoubleHeight + greatestH + pageGap;
}
}
//choose largest (to allow for rotation on specific pages)
//int max=facingW;
//if(max pageCount || (pageNumber==1 && pageCount!=2) ) {
secondW = firstW;
}else {
if ((displayRotation + pageData.getRotation(pageNumber+1))%180==90) {
secondW = pageData.getCropBoxHeight(pageNumber + 1);
} else {
secondW = pageData.getCropBoxWidth(pageNumber + 1);
}
}
//get total width
final int totalW = firstW + secondW;
width=(int)(totalW*scaling)+ PageOffsets.pageGap;
height=(biggestFacingHeight); //NOTE scaled already!
break;
case CONTINUOUS:
if((displayRotation==90)|(displayRotation==270)){
width=(int)(biggestHeight *scaling);
height=(int)((totalSingleWidth)*scaling)+gaps+insetH;
}else{
width=(int)(biggestWidth *scaling);
height=(int)((totalSingleHeight)*scaling)+gaps+insetH;
}
break;
case CONTINUOUS_FACING:
if((displayRotation==90)|(displayRotation==270)){
width=(int)((doublePageHeight)*scaling)+(insetW*2)+doubleGaps;
height=(int)((totalDoubleWidth)*scaling)+doubleGaps+insetH;
}else{
width=(int)((doublePageWidth)*scaling)+(insetW*2);
height=(int)((totalDoubleHeight)*scaling)+doubleGaps+insetH;
}
break;
}
}
public int getPageWidth() {
return width;
}
public int getPageHeight() {
return height;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy