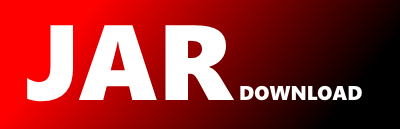
org.jpedal.fonts.tt.FontFile2 Maven / Gradle / Ivy
/*
* ===========================================
* Java Pdf Extraction Decoding Access Library
* ===========================================
*
* Project Info: http://www.idrsolutions.com
* Help section for developers at http://www.idrsolutions.com/support/
*
* (C) Copyright 1997-2015 IDRsolutions and Contributors.
*
* This file is part of JPedal/JPDF2HTML5
*
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* ---------------
* FontFile2.java
* ---------------
*/
package org.jpedal.fonts.tt;
import org.jpedal.fonts.objects.FontData;
import java.io.Serializable;
import java.util.ArrayList;
/**
* @author markee
*
* To change the template for this generated type comment go to
* Window - Preferences - Java - Code Generation - Code and Comments
*/
@SuppressWarnings("UnusedDeclaration")
public class FontFile2 implements Serializable{
private static final long serialVersionUID = -3097990864237320960L;
public static final int HEAD=0;
public static final int MAXP =1;
public static final int CMAP=2;
public static final int LOCA=3;
public static final int GLYF=4;
public static final int HHEA=5;
public static final int HMTX=6;
public static final int NAME=7;
public static final int POST=8;
public static final int CVT=9;
public static final int FPGM=10;
public static final int HDMX=11;
public static final int KERN=12;
public static final int OS2=13;
public static final int PREP=14;
public static final int DSIG=15;
public static final int CFF=16;
public static final int GSUB=17;
public static final int BASE=18;
public static final int EBDT=19;
public static final int EBLC=20;
public static final int GASP=21;
public static final int VHEA=22;
public static final int VMTX=23;
public static final int GDEF=24;
public static final int JSTF=25;
public static final int LTSH=26;
public static final int PCLT=27;
public static final int VDMX=28;
public static final int BSLN=29;
public static final int MORT=30;
public static final int FDSC=31;
public static final int FFTM=32;
public static final int GPOS=33;
public static final int FEAT=34;
public static final int JUST=35;
public static final int PROP=36;
public static final int LCCL=37;
public static final int Zapf=38;
protected int tableCount=39;
//location of tables
protected int checksums[][];
protected int tables[][];
protected int tableLength[][];
/**holds embedded font*/
private FontData fontDataAsObject;
private byte[] fontDataAsArray;
private boolean useArray=true;
protected ArrayList tableList=new ArrayList(32);
/**current location in fontDataArray*/
private int pointer;
public static final int OPENTYPE = 1;
public static final int TRUETYPE = 2;
public static final int TTC = 3;
/**subtypes used in conversion*/
public static final int PS=10;
public static final int TTF=11;
protected int subType=PS;
protected int type=TRUETYPE;
//if several fonts, selects which font
public int currentFontID;
private int fontCount=1;
//defaults are for OTF write
protected int numTables=11,searchRange=128,entrySelector=3,rangeShift=48;
public FontFile2(final FontData data){
useArray=false;
this.fontDataAsObject=data;
readHeader();
}
public FontFile2(final byte[] data){
useArray=true;
this.fontDataAsArray=data;
readHeader();
}
public FontFile2(final byte[] data, final boolean ignoreHeaders){
useArray=true;
this.fontDataAsArray=data;
if(!ignoreHeaders) {
readHeader();
}
}
public FontFile2() {
}
/**
* set selected font as a number in TTC
* ie if 4 fonts, use 0,1,2,3
* if less than fontCount. Otherwise does
* nothing
*/
public void setSelectedFontIndex(final int currentFontID) {
if(currentFontID32768) {
number -= 65536;
}
pointer += 2;
if(useArray) {
dec = ((fontDataAsArray[pointer] & 0xff) * 256) + (fontDataAsArray[pointer + 1] & 0xff);
} else {
dec = ((fontDataAsObject.getByte(pointer) & 0xff) * 256) + (fontDataAsObject.getByte(pointer + 1) & 0xff);
}
pointer += 2;
return (number+(dec/65536f));
}
/**
* get a pascal string
*/
public String getString() {
final int length;
//catch bug in odd file
if(useArray && pointer==fontDataAsArray.length) {
return "";
}
if(useArray) {
length = fontDataAsArray[pointer] & 255;
} else {
length = fontDataAsObject.getByte(pointer) & 255;
}
final char[] chars=new char[length];
//StringBuilder value=new StringBuilder();
//value.setLength(length);
pointer++;
for(int i=0;i=fontDataAsArray.length) {
i = length;
}
}
return String.copyValueOf(chars);
//return value.toString();
}
/**
* get a pascal string
*/
public byte[] getStringBytes() {
final int length;
//catch bug in odd file
if(useArray && pointer==fontDataAsArray.length) {
return new byte[1];
}
if(useArray) {
length = fontDataAsArray[pointer] & 255;
} else {
length = fontDataAsObject.getByte(pointer) & 255;
}
final byte[] value=new byte[length];
pointer++;
for(int i=0;i=fontDataAsArray.length) {
i = length;
}
}
return value;
}
public float getF2Dot14() {
final int firstValue;
if(useArray) {
firstValue = ((fontDataAsArray[pointer] & 0xff) << 8) + (fontDataAsArray[pointer + 1] & 0xff);
} else {
firstValue = ((fontDataAsObject.getByte(pointer) & 0xff) << 8) + (fontDataAsObject.getByte(pointer + 1) & 0xff);
}
pointer += 2;
if(firstValue==49152){
return -1.0f;
}else if(firstValue==16384){
return 1.0f;
}else{
return (firstValue - (2 * (firstValue & 32768))) / 16384f;
}
}
public byte[] readBytes(final int startPointer, final int length) {
if(useArray){
final byte[] block=new byte[length];
System.arraycopy(fontDataAsArray,startPointer,block,0,length);
return block;
}else {
return fontDataAsObject.getBytes(startPointer, length);
}
}
public byte[] getTableBytes(final int tableID){
final int startPointer=tables[tableID][currentFontID];
int length= tableLength[tableID][currentFontID];
if(useArray){
if (fontDataAsArray.length - startPointer < length) {
length = fontDataAsArray.length - startPointer;
}
final byte[] block=new byte[length];
System.arraycopy(fontDataAsArray,startPointer,block,0,length);
return block;
}else {
return fontDataAsObject.getBytes(startPointer, length);
}
}
//number of fonts - 1 for Open/True, can be more for TTC
public int getFontCount() {
return fontCount;
}
/**
* used to test if table too short so need to stop reading
* @return
*/
public boolean hasValuesLeft() {
final int size;
if(useArray) {
size = fontDataAsArray.length;
} else {
size = fontDataAsObject.length();
}
return pointer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy