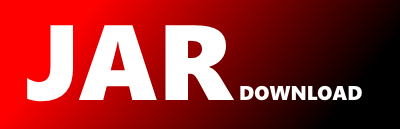
org.jpedal.parser.image.ImageCommands Maven / Gradle / Ivy
/*
* ===========================================
* Java Pdf Extraction Decoding Access Library
* ===========================================
*
* Project Info: http://www.idrsolutions.com
* Help section for developers at http://www.idrsolutions.com/support/
*
* (C) Copyright 1997-2015 IDRsolutions and Contributors.
*
* This file is part of JPedal/JPDF2HTML5
*
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* ---------------
* ImageCommands.java
* ---------------
*/
package org.jpedal.parser.image;
import org.jpedal.color.ColorSpaces;
import org.jpedal.color.GenericColorSpace;
import org.jpedal.constants.PDFflags;
import org.jpedal.function.FunctionFactory;
import org.jpedal.function.PDFFunction;
import org.jpedal.io.*;
import org.jpedal.objects.GraphicsState;
import org.jpedal.objects.raw.*;
import org.jpedal.render.DynamicVectorRenderer;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.awt.image.Raster;
public class ImageCommands {
public static final int ID=0;
public static final int XOBJECT=2;
@SuppressWarnings("CanBeFinal")
static boolean sharpenDownsampledImages;
public static boolean trackImages;
public static boolean rejectSuperimposedImages=true;
static{
final String operlapValue=System.getProperty("org.jpedal.rejectsuperimposedimages");
if(operlapValue!=null) {
ImageCommands.rejectSuperimposedImages = (operlapValue.toLowerCase().contains("true"));
}
//hidden value to turn on function
final String imgSetting=System.getProperty("org.jpedal.trackImages");
if(imgSetting!=null) {
trackImages = (imgSetting.toLowerCase().contains("true"));
}
final String nodownsamplesharpen=System.getProperty("org.jpedal.sharpendownsampledimages");
if(nodownsamplesharpen!=null) {
sharpenDownsampledImages = (nodownsamplesharpen.toLowerCase().contains("true"));
}
}
/**
* make transparent
*/
static BufferedImage makeBlackandWhiteTransparent(final BufferedImage image) {
final Raster ras=image.getRaster();
final int w=ras.getWidth();
final int h=ras.getHeight();
//image=ColorSpaceConvertor.convertToARGB(image);
final BufferedImage newImage=new BufferedImage(w,h,BufferedImage.TYPE_INT_ARGB);
boolean validPixelsFound=false,transparent,isBlack;
final int[] values=new int[3];
final int[] transparentPixel={255,0,0,0};
for(int y=0;y245 && values[1]>245 && values[2]>245);
isBlack=(values[0]<10 && values[1]<10 && values[2]<10);
//if it matched replace and move on
if(transparent || isBlack) {
newImage.getRaster().setPixels(x,y,1,1,transparentPixel);
}else{
validPixelsFound=true;
final int[] newPixel=new int[4];
newPixel[3]=255;
newPixel[0]=values[0];
newPixel[1]=values[1];
newPixel[2]=values[2];
newImage.getRaster().setPixels(x,y,1,1,newPixel);
}
}
}
if(validPixelsFound) {
return newImage;
} else {
return null;
}
}
/**
* CMYK overprint mode
*/
static BufferedImage simulateOP(BufferedImage image, final boolean whiteIs255) {
final Raster ras=image.getRaster();
image= ColorSpaceConvertor.convertToARGB(image);
final int w=image.getWidth();
final int h=image.getHeight();
boolean hasNoTransparent=false;// pixelsSet=false;
//reset
//minX=w;
//minY=h;
//maxX=-1;
//maxY=-1;
final int[] transparentPixel={255,0,0,0};
final int[] values=new int[4];
boolean transparent;
for(int y=0;y243 && values[1]>243 && values[2]>243;
}else{
transparent=values[1]<3 && values[2]<3 && values[3]<3;
}
//if it matched replace and move on
if(transparent){
image.getRaster().setPixel(x,y,transparentPixel);
}else{
hasNoTransparent=true;
}
}
}
if(hasNoTransparent){
return image;
}else {
return null;
}
}
/**
* @param maskCol
*/
static void getMaskColor(final byte[] maskCol, final GraphicsState gs) {
final int foreground =gs.nonstrokeColorSpace.getColor().getRGB();
maskCol[0]=(byte) ((foreground>>16) & 0xFF);
maskCol[1]=(byte) ((foreground>>8) & 0xFF);
maskCol[2]=(byte) ((foreground) & 0xFF);
}
/**
* Test whether the data representing a line is uniform along it height
*/
static boolean isRepeatingLine(final byte[] lineData, final int height)
{
if(lineData.length % height != 0) {
return false;
}
final int step = lineData.length / height;
for(int x = 0; x < (lineData.length / height) - 1; x++) {
int targetIndex = step;
while(targetIndex < lineData.length - 1) {
if(lineData[x] != lineData[targetIndex]) {
return false;
}
targetIndex += step;
}
}
return true;
}
static BufferedImage simulateOverprint(final GenericColorSpace decodeColorData,
final byte[] data, final boolean isDCT, final boolean isJPX, BufferedImage image,
final int colorspaceID, final DynamicVectorRenderer current, final GraphicsState gs) {
//simulate overPrint //currentGraphicsState.getNonStrokeOP() &&
if((colorspaceID==ColorSpaces.DeviceCMYK || colorspaceID==ColorSpaces.ICC) && gs.getOPM()==1.0f){
//if((colorspaceID==ColorSpaces.DeviceCMYK || colorspaceID==ColorSpaces.ICC) && gs.getOPM()==1.0f){
//try to keep as binary if possible
boolean isBlank=false;
//indexed colors
final byte[] index = decodeColorData.getIndexedMap();
//see if allblack
if(index==null && current.hasObjectsBehind(gs.CTM)){
isBlank=true; //assume true and disprove
for(int ii=0;ii4 && possIdent[0]==47 && possIdent[1]==73 && possIdent[2]==100 && possIdent[3]==101)//(/Identity
{
Function = null;
} else {
currentPdfFile.readObject(Function);
}
/** setup the translation function */
if(Function!=null) {
functions[count] = FunctionFactory.getFunction(Function, currentPdfFile);
hasFunction = true;
}
}
if (!hasFunction){
return image;
}
/**
* apply colour transform
*/
final Raster ras=image.getRaster();
//image=ColorSpaceConvertor.convertToARGB(image);
final int[] values=new int[4];
for(int y=0;ydecodeArray[1]){
//if(type!=ColorSpaces.DeviceGray){// || (decodeArray[0]>decodeArray[1] && XObject instanceof MaskObject)){
final int byteCount=data.length;
for(int ii=0;ii1)&&(type==ColorSpaces.DeviceRGB || type==ColorSpaces.CalRGB || type==ColorSpaces.DeviceCMYK)){
int j=0;
for(int ii=0;iidecodeArray[j+1]) {
currentByte = (int) decodeArray[j + 1];
}
j += 2;
if(j==decodeArray.length) {
j = 0;
}
data[ii]=(byte)currentByte;
}
}else{
/**
* apply array
*
* Assumes black and white or gray colorspace
* */
maxValue = (d<< 1);
final int divisor = maxValue - 1;
for(int ii=0;ii-1;bits--){
int current=(currentByte >> bits) & 1;
current =(int)(decodeArray[min]+ (current* ((decodeArray[max] - decodeArray[min])/ (divisor))));
/**check in range and set*/
if (current > maxValue) {
current = maxValue;
}
if (current < 0) {
current = 0;
}
current=((current & 1)<
© 2015 - 2025 Weber Informatics LLC | Privacy Policy