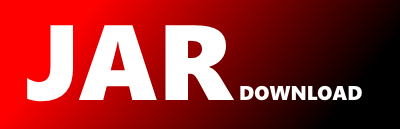
org.jpmml.converter.visitors.ExpressionCompactor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpmml-converter Show documentation
Show all versions of jpmml-converter Show documentation
Java library for authoring PMML
/*
* Copyright (c) 2016 Villu Ruusmann
*
* This file is part of JPMML-Converter
*
* JPMML-Converter is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JPMML-Converter is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with JPMML-Converter. If not, see .
*/
package org.jpmml.converter.visitors;
import java.util.List;
import java.util.ListIterator;
import org.dmg.pmml.Apply;
import org.dmg.pmml.Expression;
import org.dmg.pmml.VisitorAction;
import org.jpmml.model.visitors.AbstractVisitor;
public class ExpressionCompactor extends AbstractVisitor {
@Override
public VisitorAction visit(Apply apply){
String function = apply.getFunction();
switch(function){
case "and":
case "or":
inlineLogicalExpressions(apply);
break;
case "not":
negateExpression(apply);
break;
default:
break;
}
return super.visit(apply);
}
static
private void inlineLogicalExpressions(Apply apply){
String function = apply.getFunction();
List expressions = apply.getExpressions();
if(expressions.size() < 2){
throw new IllegalArgumentException();
}
for(ListIterator expressionIt = expressions.listIterator(); expressionIt.hasNext(); ){
Expression expression = expressionIt.next();
if(expression instanceof Apply){
Apply nestedApply = (Apply)expression;
if((function).equals(nestedApply.getFunction())){
expressionIt.remove();
// Depth first, breadth second
inlineLogicalExpressions(nestedApply);
List nestedExpressions = nestedApply.getExpressions();
for(Expression nestedExpression : nestedExpressions){
expressionIt.add(nestedExpression);
}
}
}
}
}
static
private void negateExpression(Apply apply){
List expressions = apply.getExpressions();
if(expressions.size() != 1){
throw new IllegalArgumentException();
}
ListIterator expressionIt = expressions.listIterator();
Expression expression = expressionIt.next();
if(expression instanceof Apply){
Apply nestedApply = (Apply)expression;
String negatedFunction = negate(nestedApply.getFunction());
if(negatedFunction != null){
expressionIt.remove();
apply.setFunction(negatedFunction);
List nestedExpressions = nestedApply.getExpressions();
for(Expression nestedExpression : nestedExpressions){
expressionIt.add(nestedExpression);
}
}
}
}
static
private String negate(String function){
switch(function){
case "equal":
return "notEqual";
case "greaterOrEqual":
return "lessThan";
case "greaterThan":
return "lessOrEqual";
case "isMissing":
return "isNotMissing";
case "isNotMissing":
return "isMissing";
case "lessOrEqual":
return "greaterThan";
case "lessThan":
return "greaterOrEqual";
case "notEqual":
return "equal";
default:
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy