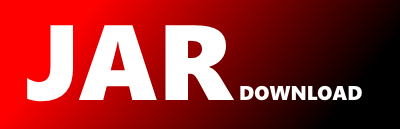
org.jpmml.evaluator.Evaluator Maven / Gradle / Ivy
/*
* Copyright (c) 2012 University of Tartu
*/
package org.jpmml.evaluator;
import java.util.*;
import org.jpmml.manager.*;
import org.dmg.pmml.*;
/**
*
* Performs the evaluation of a {@link Model} in "interpreted mode".
*
*
* Obtaining {@link Evaluator} instance:
*
* PMML pmml = ...;
* PMMLManager pmmlManager = new PMMLManager(pmml);
* Evaluator evaluator = (Evaluator)pmmlManager.getModelManager(null, ModelEvaluatorFactory.getInstance());
*
*
* Preparing {@link Evaluator#getActiveFields() active fields}:
*
* Map<FieldName, Object> arguments = new LinkedHashMap<FieldName, Object>();
* List<FieldName> activeFields = evaluator.getActiveFields();
* for(FieldName activeField : activeFields){
* arguments.put(activeField, evaluator.prepare(activeField, ...));
* }
*
*
* Performing the {@link Evaluator#evaluate(Map) evaluation}:
*
* Map<FieldName, ?> result = evaluator.evaluate(arguments);
*
*
* Retrieving the value of the {@link Evaluator#getTargetField() predicted field} and {@link Evaluator#getOutputFields() output fields}:
*
* FieldName targetField = evaluator.getTargetField();
* Object targetValue = result.get(targetField);
*
* List<FieldName> outputFields = evaluator.getOutputFields();
* for(FieldName outputField : outputFields){
* Object outputValue = result.get(outputField);
* }
*
*
* Decoding {@link Computable complex value} to simple value:
*
* Object value = ...;
* if(value instanceof Computable){
* Computable<?> computable = (Computable<?>)value;
*
* value = computable.getResult();
* }
*
*
* @see EvaluatorUtil
*/
public interface Evaluator extends Consumer {
/**
* Prepares the input value for a field.
*
* First, the value is converted from the user-supplied representation to internal representation.
* Later on, the value is subjected to missing value treatment, invalid value treatment and outlier treatment.
*
* @param name The name of the field
* @param string The input value in user-supplied representation. Use null
to represent missing input value.
*
* @throws EvaluationException If the input value preparation fails
*
* @see #getDataField(FieldName)
* @see #getMiningField(FieldName)
*/
FieldValue prepare(FieldName name, Object value);
/**
* @param arguments Map of {@link #getActiveFields() active field} values.
*
* @return Map of {@link #getPredictedFields() predicted field} and {@link #getOutputFields() output field} values.
* Simple values are represented using the Java equivalents of PMML data types (eg. String, Integer, Float, Double etc.).
* Complex values are represented as instances of {@link Computable} that return simple values.
*
* @throws EvaluationException If the evaluation fails
*
* @see Computable
*/
Map evaluate(Map arguments);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy