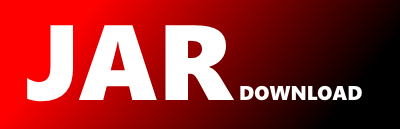
org.jpmml.evaluator.ReportingFloatValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pmml-evaluator Show documentation
Show all versions of pmml-evaluator Show documentation
JPMML class model evaluator
/*
* Copyright (c) 2017 Villu Ruusmann
*
* This file is part of JPMML-Evaluator
*
* JPMML-Evaluator is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JPMML-Evaluator is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with JPMML-Evaluator. If not, see .
*/
package org.jpmml.evaluator;
public class ReportingFloatValue
extends FloatValue
implements HasReport
{
private org.jpmml.evaluator.Report report = null;
public ReportingFloatValue(float value, org.jpmml.evaluator.Report report) {
super(value);
setReport(report);
report(new StringBuilder(256).append("").append(value).append(" ").toString());
}
public ReportingFloatValue(float value, org.jpmml.evaluator.Report report, String expression) {
super(value);
setReport(report);
if (expression!= null) {
report(expression);
}
}
@Override
public ReportingFloatValue copy() {
org.jpmml.evaluator.Report report = getReport();
return new ReportingFloatValue(super.value, report.copy(), null);
}
@Override
public ReportingFloatValue add(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.add(value));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
} else {
report(new StringBuilder(256).append("").append(((float) value)).append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue add(org.jpmml.evaluator.Value extends Number> value) {
ReportingFloatValue result = ((ReportingFloatValue) super.add(value));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(value.floatValue()).append(" ").append(" ").toString());
} else {
report(new StringBuilder(256).append("").append(value.floatValue()).append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue add(double coefficient, Number factor) {
ReportingFloatValue result = ((ReportingFloatValue) super.add(coefficient, factor));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").append("").append(((float) coefficient)).append(" ").append("").append(factor.floatValue()).append(" ").append(" ").toString());
} else {
report(new StringBuilder(256).append(" ").append("").append(((float) coefficient)).append(" ").append("").append(factor.floatValue()).append(" ").append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue add(double coefficient, Number firstFactor, Number secondFactor) {
ReportingFloatValue result = ((ReportingFloatValue) super.add(coefficient, firstFactor, secondFactor));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").append("").append(((float) coefficient)).append(" ").append("").append(firstFactor.floatValue()).append(" ").append("").append(secondFactor.floatValue()).append(" ").append(" ").toString());
} else {
report(new StringBuilder(256).append(" ").append("").append(((float) coefficient)).append(" ").append("").append(firstFactor.floatValue()).append(" ").append("").append(secondFactor.floatValue()).append(" ").append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue add(double coefficient, Number... factors) {
ReportingFloatValue result = ((ReportingFloatValue) super.add(coefficient));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").append("").append(((float) coefficient)).append(" ").append(format(factors)).append(" ").toString());
} else {
report(new StringBuilder(256).append(" ").append("").append(((float) coefficient)).append(" ").append(format(factors)).append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue add(double coefficient, Number factor, int exponent) {
ReportingFloatValue result = ((ReportingFloatValue) super.add(coefficient, factor, exponent));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").append("").append(((float) coefficient)).append(" ").append(" ").append("").append(factor.floatValue()).append(" ").append("").append(((float) exponent)).append(" ").append(" ").toString());
} else {
report(new StringBuilder(256).append(" ").append("").append(((float) coefficient)).append(" ").append(" ").append("").append(factor.floatValue()).append(" ").append("").append(((float) exponent)).append(" ").append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue subtract(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.subtract(value));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
} else {
report(new StringBuilder(256).append(" ").append("").append(((float) value)).append(" ").append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue subtract(org.jpmml.evaluator.Value extends Number> value) {
ReportingFloatValue result = ((ReportingFloatValue) super.subtract(value));
if (hasExpression()) {
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(value.floatValue()).append(" ").append(" ").toString());
} else {
report(new StringBuilder(256).append(" ").append("").append(value.floatValue()).append(" ").append(" ").toString());
}
return result;
}
@Override
public ReportingFloatValue multiply(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.multiply(value));
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue multiply(org.jpmml.evaluator.Value extends Number> value) {
ReportingFloatValue result = ((ReportingFloatValue) super.multiply(value));
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(value.floatValue()).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue multiply(Number factor, double exponent) {
ReportingFloatValue result = ((ReportingFloatValue) super.multiply(factor, exponent));
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").append("").append(factor.floatValue()).append(" ").append("").append(((float) exponent)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue divide(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.divide(value));
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue divide(org.jpmml.evaluator.Value extends Number> value) {
ReportingFloatValue result = ((ReportingFloatValue) super.divide(value));
report(new StringBuilder(256).append(" ").append(getExpression()).append("").append(value.floatValue()).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue residual(org.jpmml.evaluator.Value extends Number> value) {
ReportingFloatValue result = ((ReportingFloatValue) super.residual(value));
report(new StringBuilder(256).append("1 ").append("").append(value.floatValue()).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue square() {
ReportingFloatValue result = ((ReportingFloatValue) super.square());
report(new StringBuilder(256).append(" ").append(getExpression()).append("2 ").toString());
return result;
}
@Override
public ReportingFloatValue power(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.power(value));
report(new StringBuilder(256).append(" ").append(getExpression()).append("{0} ").toString());
return result;
}
@Override
public ReportingFloatValue reciprocal() {
ReportingFloatValue result = ((ReportingFloatValue) super.reciprocal());
report(new StringBuilder(256).append("1 ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue elliott() {
ReportingFloatValue result = ((ReportingFloatValue) super.elliott());
report(new StringBuilder(256).append("elliott ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue exp() {
ReportingFloatValue result = ((ReportingFloatValue) super.exp());
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue gauss() {
ReportingFloatValue result = ((ReportingFloatValue) super.gauss());
report(new StringBuilder(256).append("gauss ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseLogit() {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseLogit());
report(new StringBuilder(256).append("logit ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseCloglog() {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseCloglog());
report(new StringBuilder(256).append("cloglog ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseLoglog() {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseLoglog());
report(new StringBuilder(256).append("loglog ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseLogc() {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseLogc());
report(new StringBuilder(256).append("logc ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseNegbin(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseNegbin(value));
report(new StringBuilder(256).append("negbin ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseOddspower(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseOddspower(value));
report(new StringBuilder(256).append("oddspower ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inversePower(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.inversePower(value));
report(new StringBuilder(256).append(" ").append(getExpression()).append("1 ").append("").append(((float) value)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseCauchit() {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseCauchit());
report(new StringBuilder(256).append("cauchit ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue inverseProbit() {
ReportingFloatValue result = ((ReportingFloatValue) super.inverseProbit());
report(new StringBuilder(256).append("probit ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue sin() {
ReportingFloatValue result = ((ReportingFloatValue) super.sin());
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue cos() {
ReportingFloatValue result = ((ReportingFloatValue) super.cos());
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue arctan() {
ReportingFloatValue result = ((ReportingFloatValue) super.arctan());
report(new StringBuilder(256).append("2 ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue tanh() {
ReportingFloatValue result = ((ReportingFloatValue) super.tanh());
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue threshold(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.threshold(value));
report(new StringBuilder(256).append("threshold ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue relu() {
ReportingFloatValue result = ((ReportingFloatValue) super.relu());
report(new StringBuilder(256).append("0 ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue abs() {
ReportingFloatValue result = ((ReportingFloatValue) super.abs());
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue gaussSim(double value) {
ReportingFloatValue result = ((ReportingFloatValue) super.gaussSim(value));
report(new StringBuilder(256).append("gaussSim ").append(getExpression()).append("").append(((float) value)).append(" ").append(" ").toString());
return result;
}
@Override
public ReportingFloatValue restrict(double lowValue, double highValue) {
ReportingFloatValue result = ((ReportingFloatValue) super.restrict(lowValue, highValue));
report(new StringBuilder(256).append(" ").append("").append(((float) lowValue)).append(" ").append(" ").append("").append(((float) highValue)).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue round() {
ReportingFloatValue result = ((ReportingFloatValue) super.round());
report(new StringBuilder(256).append("round ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue ceiling() {
ReportingFloatValue result = ((ReportingFloatValue) super.ceiling());
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue floor() {
ReportingFloatValue result = ((ReportingFloatValue) super.floor());
report(new StringBuilder(256).append(" ").append(getExpression()).append(" ").toString());
return result;
}
@Override
public ReportingFloatValue denormalize(double leftOrig, double leftNorm, double rightOrig, double rightNorm) {
ReportingFloatValue result = ((ReportingFloatValue) super.denormalize(leftOrig, leftNorm, rightOrig, rightNorm));
report(new StringBuilder(256).append("denormalize ").append(getExpression()).append("").append(((float) leftOrig)).append(" ").append("").append(((float) leftNorm)).append(" ").append("").append(((float) rightOrig)).append(" ").append("").append(((float) rightNorm)).append(" ").append(" ").toString());
return result;
}
private void report(String expression) {
org.jpmml.evaluator.Report report = getReport();
org.jpmml.evaluator.Report.Entry entry = new org.jpmml.evaluator.Report.Entry(expression, getValue());
report.add(entry);
}
private boolean hasExpression() {
org.jpmml.evaluator.Report report = getReport();
return report.hasEntries();
}
private String getExpression() {
org.jpmml.evaluator.Report report = getReport();
org.jpmml.evaluator.Report.Entry entry = report.tailEntry();
return entry.getExpression();
}
@Override
public org.jpmml.evaluator.Report getReport() {
return this.report;
}
private void setReport(org.jpmml.evaluator.Report report) {
this.report = report;
}
private static String format(Number... values) {
StringBuilder sb = new StringBuilder((values.length* 32));
for (Number value: values) {
sb.append("").append(value.floatValue()).append(" ").toString();
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy