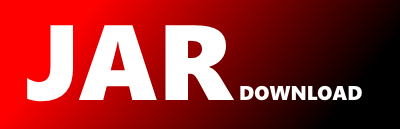
org.jpmml.evaluator.Table Maven / Gradle / Ivy
/*
* Copyright (c) 2024 Villu Ruusmann
*
* This file is part of JPMML-Evaluator
*
* JPMML-Evaluator is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JPMML-Evaluator is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with JPMML-Evaluator. If not, see .
*/
package org.jpmml.evaluator;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
public class Table {
private List columns = null;
private List exceptions = new ArrayList<>();
private Map> values = new HashMap<>();
private Map types = new HashMap<>();
public Table(){
this(new ArrayList<>());
}
public Table(List columns){
setColumns(columns);
}
public int getNumberOfRows(){
List exceptions = getExceptions();
int result = exceptions.size();
Map> columnValues = getValues();
Collection>> entries = columnValues.entrySet();
for(Map.Entry> entry : entries){
List> values = entry.getValue();
result = Math.max(result, values.size());
}
return result;
}
public int getNumberOfColumns(){
List columns = getColumns();
return columns.size();
}
public void canonicalize(){
List columns = getColumns();
int numberOfRows = getNumberOfRows();
List exceptions = getExceptions();
TableUtil.ensureSize(exceptions, numberOfRows);
for(String column : columns){
List> values = getValues(column);
if(values == null){
values = new ArrayList<>(numberOfRows);
setValues(column, values);
}
TableUtil.ensureSize(values, numberOfRows);
}
}
public boolean addColumn(String column){
List columns = getColumns();
if(!columns.contains(column)){
columns.add(column);
return true;
}
return false;
}
public boolean removeColumn(String column){
List columns = getColumns();
boolean result = columns.remove(column);
if(result){
Map> columnValues = getValues();
Map columnTypes = getTypes();
columnValues.remove(column);
columnTypes.remove(column);
}
return result;
}
public Exception getException(int index){
List exceptions = getExceptions();
return TableUtil.get(exceptions, index);
}
public void setException(int index, Exception exception){
List exceptions = getExceptions();
TableUtil.set(exceptions, index, exception);
}
public List> getValues(String column){
Map> columnValues = getValues();
return columnValues.get(column);
}
public void setValues(String column, List> values){
Map> columnValues = getValues();
columnValues.put(column, values);
}
public TypeInfo getType(String column){
Map columnTypes = getTypes();
return columnTypes.get(column);
}
public void setType(String column, TypeInfo typeInfo){
Map columnTypes = getTypes();
columnTypes.put(column, typeInfo);
}
public List getColumns(){
return this.columns;
}
void setColumns(List columns){
this.columns = Objects.requireNonNull(columns);
}
public List getExceptions(){
return this.exceptions;
}
public Map> getValues(){
return this.values;
}
public Map getTypes(){
return this.types;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy