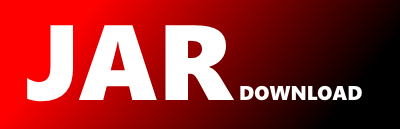
org.dmg.pmml.RegressionTable Maven / Gradle / Ivy
Show all versions of pmml-model-gwt Show documentation
package org.dmg.pmml;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.dmg.org/PMML-4_2}Extension" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.dmg.org/PMML-4_2}NumericPredictor" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.dmg.org/PMML-4_2}CategoricalPredictor" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.dmg.org/PMML-4_2}PredictorTerm" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="intercept" use="required" type="{http://www.dmg.org/PMML-4_2}REAL-NUMBER" />
* <attribute name="targetCategory" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"extensions",
"numericPredictors",
"categoricalPredictors",
"predictorTerms"
})
@XmlRootElement(name = "RegressionTable", namespace = "http://www.dmg.org/PMML-4_2")
public class RegressionTable
extends org.dmg.pmml.PMMLObject
implements HasExtensions
{
@XmlAttribute(name = "intercept", required = true)
private double intercept;
@XmlAttribute(name = "targetCategory")
private String targetCategory;
@XmlElement(name = "Extension", namespace = "http://www.dmg.org/PMML-4_2")
private List extensions;
@XmlElement(name = "NumericPredictor", namespace = "http://www.dmg.org/PMML-4_2")
private List numericPredictors;
@XmlElement(name = "CategoricalPredictor", namespace = "http://www.dmg.org/PMML-4_2")
private List categoricalPredictors;
@XmlElement(name = "PredictorTerm", namespace = "http://www.dmg.org/PMML-4_2")
private List predictorTerms;
public RegressionTable() {
super();
}
public RegressionTable(final double intercept) {
super();
this.intercept = intercept;
}
/**
* Gets the value of the intercept property.
*
*/
public double getIntercept() {
return intercept;
}
/**
* Sets the value of the intercept property.
*
*/
public RegressionTable setIntercept(double intercept) {
this.intercept = intercept;
return this;
}
/**
* Gets the value of the targetCategory property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTargetCategory() {
return targetCategory;
}
/**
* Sets the value of the targetCategory property.
*
* @param targetCategory
* allowed object is
* {@link String }
*
*/
public RegressionTable setTargetCategory(String targetCategory) {
this.targetCategory = targetCategory;
return this;
}
/**
* Gets the value of the extensions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the extensions property.
*
*
* For example, to add a new item, do as follows:
*
* getExtensions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension }
*
*
*/
public List getExtensions() {
if (extensions == null) {
extensions = new ArrayList();
}
return this.extensions;
}
/**
* Gets the value of the numericPredictors property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the numericPredictors property.
*
*
* For example, to add a new item, do as follows:
*
* getNumericPredictors().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NumericPredictor }
*
*
*/
public List getNumericPredictors() {
if (numericPredictors == null) {
numericPredictors = new ArrayList();
}
return this.numericPredictors;
}
/**
* Gets the value of the categoricalPredictors property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the categoricalPredictors property.
*
*
* For example, to add a new item, do as follows:
*
* getCategoricalPredictors().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CategoricalPredictor }
*
*
*/
public List getCategoricalPredictors() {
if (categoricalPredictors == null) {
categoricalPredictors = new ArrayList();
}
return this.categoricalPredictors;
}
/**
* Gets the value of the predictorTerms property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the predictorTerms property.
*
*
* For example, to add a new item, do as follows:
*
* getPredictorTerms().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PredictorTerm }
*
*
*/
public List getPredictorTerms() {
if (predictorTerms == null) {
predictorTerms = new ArrayList();
}
return this.predictorTerms;
}
public boolean hasExtensions() {
return ((this.extensions!= null)&&(this.extensions.size()> 0));
}
public RegressionTable addExtensions(Extension... extensions) {
getExtensions().addAll(Arrays.asList(extensions));
return this;
}
public boolean hasNumericPredictors() {
return ((this.numericPredictors!= null)&&(this.numericPredictors.size()> 0));
}
public RegressionTable addNumericPredictors(NumericPredictor... numericPredictors) {
getNumericPredictors().addAll(Arrays.asList(numericPredictors));
return this;
}
public boolean hasCategoricalPredictors() {
return ((this.categoricalPredictors!= null)&&(this.categoricalPredictors.size()> 0));
}
public RegressionTable addCategoricalPredictors(CategoricalPredictor... categoricalPredictors) {
getCategoricalPredictors().addAll(Arrays.asList(categoricalPredictors));
return this;
}
public boolean hasPredictorTerms() {
return ((this.predictorTerms!= null)&&(this.predictorTerms.size()> 0));
}
public RegressionTable addPredictorTerms(PredictorTerm... predictorTerms) {
getPredictorTerms().addAll(Arrays.asList(predictorTerms));
return this;
}
@Override
public VisitorAction accept(Visitor visitor) {
VisitorAction status = visitor.visit(this);
visitor.pushParent(this);
if ((status == VisitorAction.CONTINUE)&&hasExtensions()) {
status = org.dmg.pmml.PMMLObject.traverse(visitor, getExtensions());
}
if ((status == VisitorAction.CONTINUE)&&hasNumericPredictors()) {
status = org.dmg.pmml.PMMLObject.traverse(visitor, getNumericPredictors());
}
if ((status == VisitorAction.CONTINUE)&&hasCategoricalPredictors()) {
status = org.dmg.pmml.PMMLObject.traverse(visitor, getCategoricalPredictors());
}
if ((status == VisitorAction.CONTINUE)&&hasPredictorTerms()) {
status = org.dmg.pmml.PMMLObject.traverse(visitor, getPredictorTerms());
}
visitor.popParent();
if (status == VisitorAction.TERMINATE) {
return VisitorAction.TERMINATE;
}
return VisitorAction.CONTINUE;
}
}