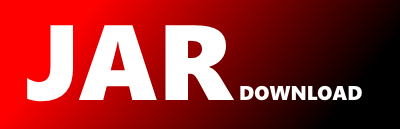
org.dmg.pmml.TextModelNormalization Maven / Gradle / Ivy
Show all versions of pmml-model-gwt Show documentation
package org.dmg.pmml;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.dmg.org/PMML-4_2}Extension" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="localTermWeights" default="termFrequency">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="termFrequency"/>
* <enumeration value="binary"/>
* <enumeration value="logarithmic"/>
* <enumeration value="augmentedNormalizedTermFrequency"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="globalTermWeights" default="inverseDocumentFrequency">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="inverseDocumentFrequency"/>
* <enumeration value="none"/>
* <enumeration value="GFIDF"/>
* <enumeration value="normal"/>
* <enumeration value="probabilisticInverse"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="documentNormalization" default="none">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="none"/>
* <enumeration value="cosine"/>
* </restriction>
* </simpleType>
* </attribute>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"extensions"
})
@XmlRootElement(name = "TextModelNormalization", namespace = "http://www.dmg.org/PMML-4_2")
public class TextModelNormalization
extends org.dmg.pmml.PMMLObject
implements HasExtensions
{
@XmlAttribute(name = "localTermWeights")
private TextModelNormalization.LocalTermWeights localTermWeights;
@XmlAttribute(name = "globalTermWeights")
private TextModelNormalization.GlobalTermWeights globalTermWeights;
@XmlAttribute(name = "documentNormalization")
private TextModelNormalization.DocumentNormalization documentNormalization;
@XmlElement(name = "Extension", namespace = "http://www.dmg.org/PMML-4_2")
private List extensions;
/**
* Gets the value of the localTermWeights property.
*
* @return
* possible object is
* {@link TextModelNormalization.LocalTermWeights }
*
*/
public TextModelNormalization.LocalTermWeights getLocalTermWeights() {
if (localTermWeights == null) {
return TextModelNormalization.LocalTermWeights.TERM_FREQUENCY;
} else {
return localTermWeights;
}
}
/**
* Sets the value of the localTermWeights property.
*
* @param localTermWeights
* allowed object is
* {@link TextModelNormalization.LocalTermWeights }
*
*/
public TextModelNormalization setLocalTermWeights(TextModelNormalization.LocalTermWeights localTermWeights) {
this.localTermWeights = localTermWeights;
return this;
}
/**
* Gets the value of the globalTermWeights property.
*
* @return
* possible object is
* {@link TextModelNormalization.GlobalTermWeights }
*
*/
public TextModelNormalization.GlobalTermWeights getGlobalTermWeights() {
if (globalTermWeights == null) {
return TextModelNormalization.GlobalTermWeights.INVERSE_DOCUMENT_FREQUENCY;
} else {
return globalTermWeights;
}
}
/**
* Sets the value of the globalTermWeights property.
*
* @param globalTermWeights
* allowed object is
* {@link TextModelNormalization.GlobalTermWeights }
*
*/
public TextModelNormalization setGlobalTermWeights(TextModelNormalization.GlobalTermWeights globalTermWeights) {
this.globalTermWeights = globalTermWeights;
return this;
}
/**
* Gets the value of the documentNormalization property.
*
* @return
* possible object is
* {@link TextModelNormalization.DocumentNormalization }
*
*/
public TextModelNormalization.DocumentNormalization getDocumentNormalization() {
if (documentNormalization == null) {
return TextModelNormalization.DocumentNormalization.NONE;
} else {
return documentNormalization;
}
}
/**
* Sets the value of the documentNormalization property.
*
* @param documentNormalization
* allowed object is
* {@link TextModelNormalization.DocumentNormalization }
*
*/
public TextModelNormalization setDocumentNormalization(TextModelNormalization.DocumentNormalization documentNormalization) {
this.documentNormalization = documentNormalization;
return this;
}
/**
* Gets the value of the extensions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the extensions property.
*
*
* For example, to add a new item, do as follows:
*
* getExtensions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension }
*
*
*/
public List getExtensions() {
if (extensions == null) {
extensions = new ArrayList();
}
return this.extensions;
}
public boolean hasExtensions() {
return ((this.extensions!= null)&&(this.extensions.size()> 0));
}
public TextModelNormalization addExtensions(Extension... extensions) {
getExtensions().addAll(Arrays.asList(extensions));
return this;
}
@Override
public VisitorAction accept(Visitor visitor) {
VisitorAction status = visitor.visit(this);
visitor.pushParent(this);
if ((status == VisitorAction.CONTINUE)&&hasExtensions()) {
status = org.dmg.pmml.PMMLObject.traverse(visitor, getExtensions());
}
visitor.popParent();
if (status == VisitorAction.TERMINATE) {
return VisitorAction.TERMINATE;
}
return VisitorAction.CONTINUE;
}
/**
* Java class for null.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="none"/>
* <enumeration value="cosine"/>
* </restriction>
* </simpleType>
*
*
*/
@XmlType(name = "")
@XmlEnum
public enum DocumentNormalization {
@XmlEnumValue("none")
NONE("none"),
@XmlEnumValue("cosine")
COSINE("cosine");
private final String value;
DocumentNormalization(String v) {
value = v;
}
public String value() {
return value;
}
public static TextModelNormalization.DocumentNormalization fromValue(String v) {
for (TextModelNormalization.DocumentNormalization c: TextModelNormalization.DocumentNormalization.values()) {
if (c.value.equals(v)) {
return c;
}
}
throw new IllegalArgumentException(v);
}
}
/**
* Java class for null.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="inverseDocumentFrequency"/>
* <enumeration value="none"/>
* <enumeration value="GFIDF"/>
* <enumeration value="normal"/>
* <enumeration value="probabilisticInverse"/>
* </restriction>
* </simpleType>
*
*
*/
@XmlType(name = "")
@XmlEnum
public enum GlobalTermWeights {
@XmlEnumValue("inverseDocumentFrequency")
INVERSE_DOCUMENT_FREQUENCY("inverseDocumentFrequency"),
@XmlEnumValue("none")
NONE("none"),
GFIDF("GFIDF"),
@XmlEnumValue("normal")
NORMAL("normal"),
@XmlEnumValue("probabilisticInverse")
PROBABILISTIC_INVERSE("probabilisticInverse");
private final String value;
GlobalTermWeights(String v) {
value = v;
}
public String value() {
return value;
}
public static TextModelNormalization.GlobalTermWeights fromValue(String v) {
for (TextModelNormalization.GlobalTermWeights c: TextModelNormalization.GlobalTermWeights.values()) {
if (c.value.equals(v)) {
return c;
}
}
throw new IllegalArgumentException(v);
}
}
/**
* Java class for null.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="termFrequency"/>
* <enumeration value="binary"/>
* <enumeration value="logarithmic"/>
* <enumeration value="augmentedNormalizedTermFrequency"/>
* </restriction>
* </simpleType>
*
*
*/
@XmlType(name = "")
@XmlEnum
public enum LocalTermWeights {
@XmlEnumValue("termFrequency")
TERM_FREQUENCY("termFrequency"),
@XmlEnumValue("binary")
BINARY("binary"),
@XmlEnumValue("logarithmic")
LOGARITHMIC("logarithmic"),
@XmlEnumValue("augmentedNormalizedTermFrequency")
AUGMENTED_NORMALIZED_TERM_FREQUENCY("augmentedNormalizedTermFrequency");
private final String value;
LocalTermWeights(String v) {
value = v;
}
public String value() {
return value;
}
public static TextModelNormalization.LocalTermWeights fromValue(String v) {
for (TextModelNormalization.LocalTermWeights c: TextModelNormalization.LocalTermWeights.values()) {
if (c.value.equals(v)) {
return c;
}
}
throw new IllegalArgumentException(v);
}
}
}